以下是十五个最有用的PHP代码片段。您可能还想对任何代码或者你也可以分享你的代码片段在评论部分如果你认为它可能对其他人有用。
1。发送邮件使用邮件函数在PHP
与站长百科 PHP教程同步首发
$to = "viralpatel.net@gmail.com";
$subject = "VIRALPATEL.net";
$body = "Body of your message here you can use HTML too. e.g.
Bold ";
$headers = "From: Peter\r\n";
$headers .= "Reply-To: info@yoursite.com\r\n";
$headers .= "Return-Path: info@yoursite.com\r\n";
$headers .= "X-Mailer: PHP5\n";
$headers .= 'MIME-Version: 1.0' . "\n";
$headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n";
mail($to,$subject,$body,$headers);
?>
2。Base64编码和解码字符串在PHP
function base64url_encode($plainText) {
$base64 = base64_encode($plainText);
$base64url = strtr($base64, '+/=', '-_,');
return $base64url;
}
function base64url_decode($plainText) {
$base64url = strtr($plainText, '-_,', '+/=');
$base64 = base64_decode($base64url);
return $base64;
}
3。在PHP中获得远程IP地址
function getRemoteIPAddress() {
$ip = $_SERVER['REMOTE_ADDR'];
return $ip;
}
上面的代码将无法工作,以防您的客户端代理服务器后面。在这种情况下使用函数来获得真正的IP地址的客户。
function getRealIPAddr()
{
if (!empty($_SERVER['HTTP_CLIENT_IP'])) //check ip from share internet
{
$ip=$_SERVER['HTTP_CLIENT_IP'];
}
elseif (!empty($_SERVER['HTTP_X_FORWARDED_FOR'])) //to check ip is pass from proxy
{
$ip=$_SERVER['HTTP_X_FORWARDED_FOR'];
}
else
{
$ip=$_SERVER['REMOTE_ADDR'];
}
return $ip;
}
4。秒到字符串
这个函数将返回给定时间段期间在天、小时、分钟和秒。
例如secsToStr(1234567)将返回“14天6小时56分钟,7秒”
function secsToStr($secs) {
if($secs>=86400){$days=floor($secs/86400);$secs=$secs%86400;$r=$days.' day';if($days1){$r.='s';}if($secs>0){$r.=', ';}}
if($secs>=3600){$hours=floor($secs/3600);$secs=$secs%3600;$r.=$hours.' hour';if($hours1){$r.='s';}if($secs>0){$r.=', ';}}
if($secs>=60){$minutes=floor($secs/60);$secs=$secs%60;$r.=$minutes.' minute';if($minutes1){$r.='s';}if($secs>0){$r.=', ';}}
$r.=$secs.' second';if($secs1){$r.='s';}
return $r;
}
5。电子邮件验证代码片段在PHP
$email = $_POST['email'];
if(preg_match("~([a-zA-Z0-9!#$%&'*+-/=?^_`{|}~])@([a-zA-Z0-9-]).([a-zA-Z0-9]{2,4})~",$email)) {
echo 'This is a valid email.';
} else{
echo 'This is an invalid email.';
}
6。解析XML的简单的方法使用PHP
所需的扩展:SimpleXML
//this is a sample xml string
$xml_string="
A
K
//load the xml string using simplexml function
$xml = simplexml_load_string($xml_string);
//loop through the each node of molecule
foreach ($xml->molecule as $record)
{
//attribute are accessted by
echo $record['name'], ' ';
//node are accessted by -> operator
echo $record->symbol, ' ';
echo $record->code, '
';
}
7。数据库连接在PHP
if(basename(__FILE__) == basename($_SERVER['PHP_SELF'])) send_404();
$dbHost = "localhost"; //Location Of Database usually its localhost
$dbUser = "xxxx"; //Database User Name
$dbPass = "xxxx"; //Database Password
$dbDatabase = "xxxx"; //Database Name
$db = mysql_connect("$dbHost", "$dbUser", "$dbPass") or die ("Error connecting to database.");
mysql_select_db("$dbDatabase", $db) or die ("Couldn't select the database.");
# This function will send an imitation 404 page if the user
# types in this files filename into the address bar.
# only files connecting with in the same directory as this
# file will be able to use it as well.
function send_404()
{
header('HTTP/1.x 404 Not Found');
print ''."n".
'
'
''."n".
'
Not Found
'."n".'
The requested URL '.
str_replace(strstr($_SERVER['REQUEST_URI'], '?'), '', $_SERVER['REQUEST_URI']).
' was not found on this server.
''."n";
exit;
}
# In any file you want to connect to the database,
# and in this case we will name this file db.php
# just add this line of php code (without the pound sign):
# include"db.php";
?>
8。创建和解析JSON数据在PHP
以下是PHP代码创建JSON数据格式,上面的示例使用的PHP数组。
$json_data = array ('id'=>1,'name'=>"rolf",'country'=>'russia',"office"=>array("google","oracle"));
echo json_encode($json_data);
下面的代码将解析JSON数据到PHP数组。
$json_string='{"id":1,"name":"rolf","country":"russia","office":["google","oracle"]} ';
$obj=json_decode($json_string);
//print the parsed data
echo $obj->name; //displays rolf
echo $obj->office[0]; //displays google
9。MySQL在PHP过程时间戳
$query = "select UNIX_TIMESTAMP(date_field) as mydate from mytable where 1=1";
$records = mysql_query($query) or die(mysql_error());
while($row = mysql_fetch_array($records))
{
echo $row;
}
10。在PHP中生成一个身份验证代码
这个基本的代码片段将创建一个随机身份验证代码,或者仅仅是一个随机字符串。
# This particular code will generate a random string
# that is 25 charicters long 25 comes from the number
# that is in the for loop
$string = "abcdefghijklmnopqrstuvwxyz0123456789";
for($i=0;$i $pos = rand(0,36);
$str .= $string{$pos};
}
echo $str;
# If you have a database you can save the string in
# there, and send the user an email with the code in
# it they then can click a link or copy the code
# and you can then verify that that is the correct email
# or verify what ever you want to verify
?>
11。在PHP的日期格式验证
验证一个日期在“yyyy mm dd”格式。
function checkDateFormat($date)
{
//match the format of the date
if (preg_match ("/^([0-9]{4})-([0-9]{2})-([0-9]{2})$/", $date, $parts))
{
//check weather the date is valid of not
if(checkdate($parts[2],$parts[3],$parts[1]))
return true;
else
return false;
}
else
return false;
}
12。HTTP重定向在PHP
header('Location: http://you_stuff/url.php'); // stick your url here
?>
13。目录清单在PHP
function list_files($dir)
{
if(is_dir($dir))
{
if($handle = opendir($dir))
{
while(($file = readdir($handle)) !== false)
{
if($file != "." && $file != ".." && $file != "Thumbs.db"/*pesky windows, images..*/)
{
echo ''.$file.'
'."\n";
}
}
closedir($handle);
}
}
}
/*
To use:
list_files("images/");
?>
*/
?>
14。在PHP浏览器检测脚本
$useragent = $_SERVER ['HTTP_USER_AGENT'];
echo "Your User Agent is: " . $useragent;
?>
15。Zip文件解压缩一个
function unzip($location,$newLocation){
if(exec("unzip $location",$arr)){
mkdir($newLocation);
for($i = 1;$i $file = trim(preg_replace("~inflating: ~","",$arr[$i]));
copy($location.'/'.$file,$newLocation.'/'.$file);
unlink($location.'/'.$file);
}
return TRUE;
}else{
return FALSE;
}
}
?>
//Use the code as following:
include 'functions.php';
if(unzip('zipedfiles/test.zip','unziped/myNewZip'))
echo 'Success!';
else
echo 'Error';
?>
你怎么这样小的收集的PHP代码片段。你可能想要将您的代码片段在评论中与他人分享。
作者:ssoftware
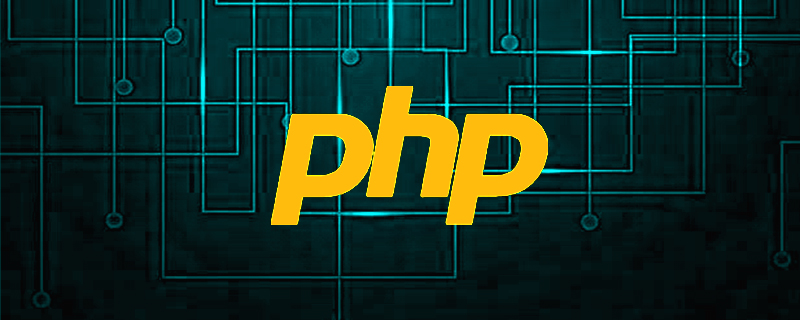
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
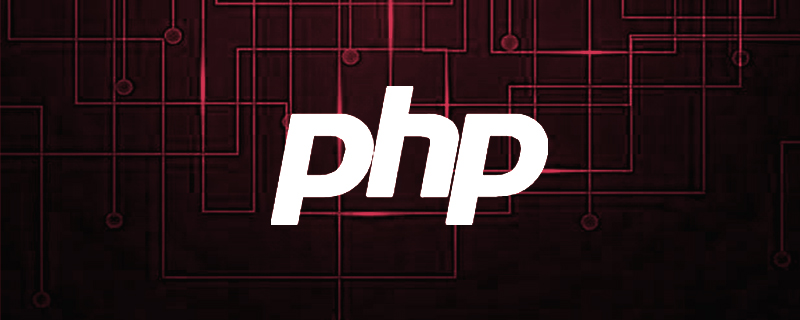
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
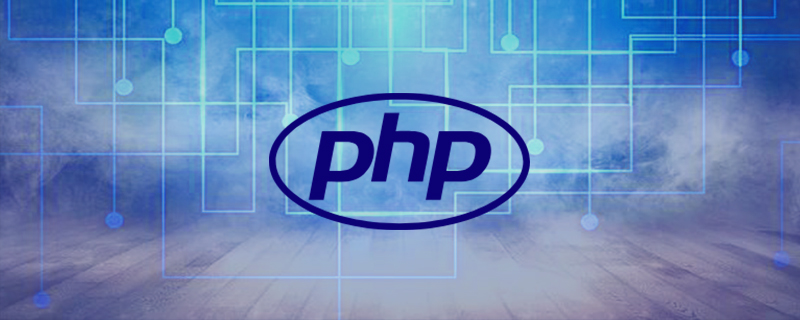
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
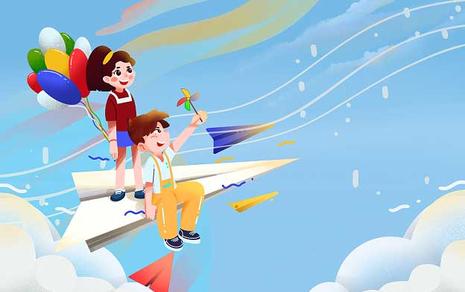
推荐适合地理信息科学专业学生用的电脑1.推荐2.地理信息科学专业学生需要处理大量的地理数据和进行复杂的地理信息分析,因此需要一台性能较强的电脑。一台配置高的电脑可以提供更快的处理速度和更大的存储空间,能够更好地满足专业需求。3.推荐选择一台配备高性能处理器和大容量内存的电脑,这样可以提高数据处理和分析的效率。此外,选择一台具备较大存储空间和高分辨率显示屏的电脑也能更好地展示地理数据和结果。另外,考虑到地理信息科学专业学生可能需要进行地理信息系统(GIS)软件的开发和编程,选择一台支持较好的图形处
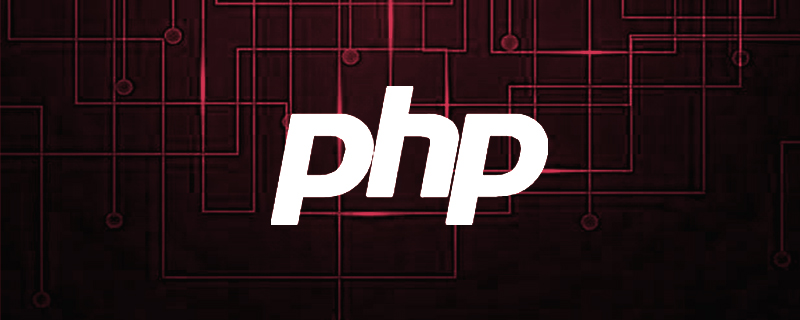
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
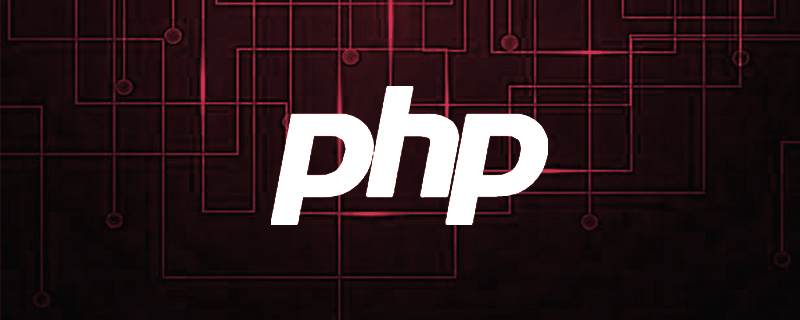
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
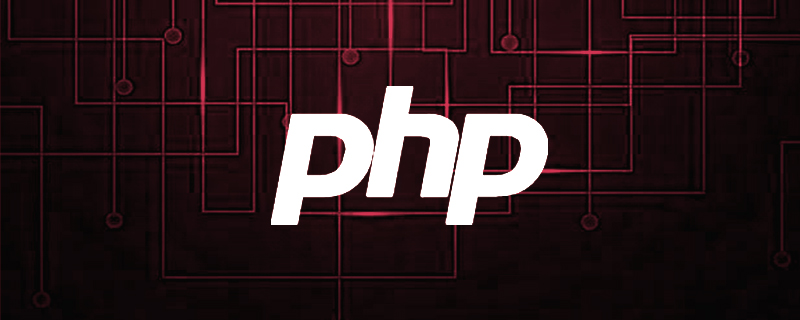
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
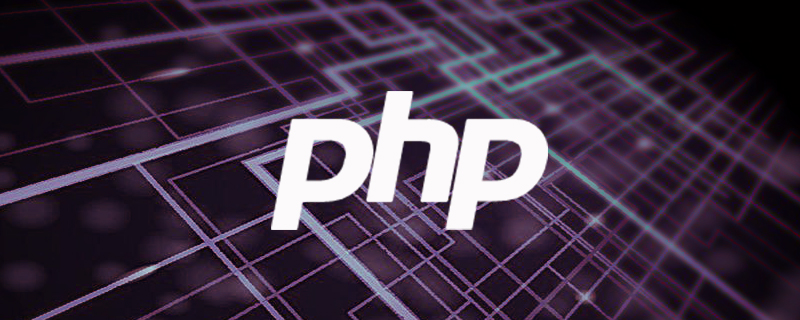
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
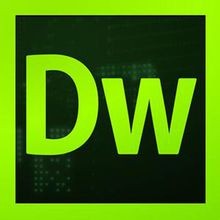
드림위버 CS6
시각적 웹 개발 도구

SecList
SecLists는 최고의 보안 테스터의 동반자입니다. 보안 평가 시 자주 사용되는 다양한 유형의 목록을 한 곳에 모아 놓은 것입니다. SecLists는 보안 테스터에게 필요할 수 있는 모든 목록을 편리하게 제공하여 보안 테스트를 더욱 효율적이고 생산적으로 만드는 데 도움이 됩니다. 목록 유형에는 사용자 이름, 비밀번호, URL, 퍼징 페이로드, 민감한 데이터 패턴, 웹 셸 등이 포함됩니다. 테스터는 이 저장소를 새로운 테스트 시스템으로 간단히 가져올 수 있으며 필요한 모든 유형의 목록에 액세스할 수 있습니다.

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.
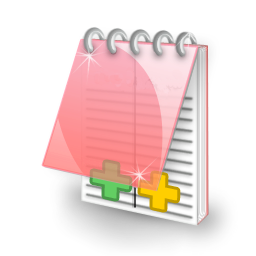
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.
