Makemoji SDK
Makemojiis a free emoji keyboard for mobile apps.
By installing our keyboard SDK every user of your app will instantly have access to new and trending emojis. Our goal is to increase user engagement as well as provide actionable real time data on sentiment (how users feel) and affinity (what users like). With this extensive data collection your per-user & company valuation will increase along with your user-base.
Features Include
- Extensive library of free emoji
- 722 standard Unicode emoji
- Makemoji Flashtag inline search system
- New emoji load dynamically and does not require a app update
- Analytics Dashboard & CMS
To obtain your SDK key please email: sdk@makemoji.com
Learn More
Library Setup
-
If you are using CocoaPods for dependencies, include the following.
pod "Makemoji-SDK"
-
If your are not using CocoaPods, be sure to include the following libraries.
- AFNetworking 2.6.3
- SDWebImage 3.7.3
-
Drag the MakemojiSDK folder to your project.
-
In Xcode, click on your App Target -> Build Phases -> Link Binary with Libraries and add the following libraries.
libsqlite3 libxml2 libz
- With iOS 9, you will need to include a exception for AWS S3 in your Info.plist for App Transport.
<dict> <key>NSAllowsArbitraryLoads</key> <true/> <key>s3.amazonaws.com</key> <dict> <key>NSExceptionAllowsInsecureHTTPLoads</key> <true/> </dict></dict>
SDK Usage
Initialization
To start using the MakemojiSDK you will first have to add a few lines to your AppDelegate.
Add the Makemoji header file to you AppDelegate.m file.
#import "MakemojiSDK.h"
Then on launch, setup your SDK key.
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { // Override point for customization after application launch. // setup your SDK key [MakemojiSDK setSDKKey:@"YOUR-SDK-KEY"]; return YES; }
Setup a the Makemoji TextInput
Next you will need setup a view controller and add the METextInputView as a property. You will also need to make this conform to the METextInputViewDelegate protocol.
#import <UIKit/UIKit.h> #import "METextInputView.h" @interface ViewController : UIViewController <METextInputViewDelegate> @property (nonatomic, retain) METextInputView * meTextInputView; @end
In your view controller during viewDidLoad or init, initialize the METextInputView. Use the showKeyboard method to make the text input field the first responder.
- (void)viewDidLoad { [super viewDidLoad]; self.meTextInputView = [[METextInputView alloc] initWithFrame:CGRectZero]; self.meTextInputView.delegate = self; [self.view addSubview:self.meTextInputView];}-(void)viewDidAppear:(BOOL)animated { [super viewDidAppear:animated]; [self.meTextInputView showKeyboard];}
Detached Text Input
If you need the Text Input detached from the keyboard, you will need to call the detachTextInputView method and then add textInputContainerView to your view.
[self.meTextInputView detachTextInputView:YES]; [self.view addSubview:self.meTextInputView.textInputContainerView];
Since the Send Button and Camera button are hidden in this mode, you will need to call attach a button to the sendMessage method to trigger capturing the text.
See the included MakemojiSDKDemo app for a full example of how to set this up.
Handling Keyboard & Input Size Changes
You will need to handle keyboard appearance resizing and text input size changes. The didChangeFrame delegate method is called when these events occur.
-(void)meTextInputView:(METextInputView *)inputView didChangeFrame:(CGRect)frame { self.tableView.frame = CGRectMake(self.tableView.frame.origin.x, self.tableView.frame.origin.y, self.tableView.frame.size.width, self.meTextInputView.frame.origin.y);}
Send a Message
The didTapSend delegate callback gives you a dictionary of plaintext and HTML from the MakemojiSDK text view when the Send button is tapped.
-(void)meTextInputView:(METextInputView *)inputView didTapSend:(NSDictionary *)message { NSLog(@"%@", message); // send message to your backend here [self.messages addObject:message]; [self.tableView reloadData];}
The messageDictionary returns the following
{"html" : "Your Message with HTML", "plaintext" : "Your message translated to plaintext"}
You would then send this to your backend to store the message.
You can show or hide the built-in send button by setting the displaySendButton property on METextInputView
self.meTextInputView.displaySendButton = NO;
Camera Button
This is a standard UIButton that can be customized. To handle a action for the camera button use the didTapCameraButton delegate callback.
-(void)meTextInputView:(METextInputView *)inputView didTapCameraButton:(UIButton*)cameraButton { // Present image controller}
You can show or hide the built-in camera by setting the displayCameraButton property on METextInputView
self.meTextInputView.displayCameraButton = NO;
Hypermoji - Emoji with a URL
To handle the display of a webpage when tapping on a Hypermoji ( a emoji with a URL link), use the didTapHypermoji delegate callback
// handle tapping of links (Hypermoji) -(void)meTextInputView:(METextInputView *)inputView didTapHypermoji:(NSString*)urlString { // open webview here }
Displaying Messages
We have included a optimized UITableViewCells for displaying HTML messages. MEChatTableViewCell mimics iMessage display behavior and includes a simple image attachment feature. MESimpleTableViewCell is provided for extensive customization options.
Use the cellHeightForHTML method to give you the row height for a html message. This method caches cell heights for increased performance.
// determine row height with HTML- (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath { if (self.meTextInputView == nil) { return 0; } NSDictionary * message = [self.messages objectAtIndex:indexPath.row]; return [self.meTextInputView cellHeightForHTML:[message objectForKey:@"html"] atIndexPath:indexPath maxCellWidth:self.tableView.frame.size.width cellStyle:MECellStyleChat];}
You can set the MEChatTableViewCell to display on the left or right hand side using setCellDisplay. This should happen before setting your HTML for each message.
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath { static NSString *CellIdentifier = @"Cell"; MEChatTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier]; if (cell == nil) { cell = [[MEChatTableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:CellIdentifier]; } // display chat cell on right side [cell setCellDisplay:MECellDisplayRight]; // display chat cell on left side if (indexPath.row % 2) { [cell setCellDisplay:MECellDisplayLeft]; } NSDictionary * message = [self.messages objectAtIndex:indexPath.row]; [cell setHTMLString:[message objectForKey:@"html"]]; return cell; }
Emoji Wall
The Emoji Wall is a View Controller that allows your users to select one emoji from the makemoji library or the built-in iOS emoji.
To display the emoji wall, use the following:
// initialize the emoji wall view controller MEEmojiWall * emojiWall = [[MEEmojiWall alloc] init]; emojiWall.delegate = self; emojiWall.modalPresentationStyle = UIModalPresentationOverCurrentContext; // wrap view controller in navigation controller UINavigationController *navigationController = [[UINavigationController alloc] initWithRootViewController:emojiWall]; [navigationController.navigationBar setBarTintColor:[UIColor blackColor]]; [navigationController.navigationBar setBarStyle:UIBarStyleBlackTranslucent]; [navigationController.navigationBar setTintColor:[UIColor whiteColor]]; // present the emoji wall as a modal [self presentViewController:navigationController animated:YES completion:nil];
The search bar can be disabled by using the following when instantiating the controller
emojiWall.shouldDisplaySearch = NO;
When a user selects an emoji from the wall, the following NSDictionary is returned to the Emoji Wall delegate.
For Makemoji emoji:
{ "emoji_id" = 935; "emoji_type" = makemoji; "image_object" = "56debbdf1cb0fbf041c63c015fb19ac9, {110, 110}"; "image_url" = "http://d1tvcfe0bfyi6u.cloudfront.net/emoji/935-large@2x.png"; name = Amused; }
For iOS emoji:
{ "emoji_id" = 18; "emoji_type" = native; name = "pensive face"; "unicode_character" = "\Ud83d\Ude14"; }
Reactions
Makemoji reactions allow you to add inline emoji reactions to any view. Here's how you set this up.
You will first want to init the reaction view and give it a frame, typically a height of 30 is the best, but you can use anyting from 25 to 40.
self.reactionView = [[MEReactionView alloc] initWithFrame:CGRectMake(0, 0, your_width, 30)];
You will then want to provide the reaction view with a content id, which is a NSString. THis should be unique to the content you want to associate these reactions to.
self.reactionView.contentId = @"article123";
Setting this contentId will retrieve available reaction data as well as retrieve your default reaction set.
If you want to listen for user reacting to this view, observe the MEReactionNotification key.
The notification will include the reaction data that was selected.
FAQ
-
The Makemoji SDK is completely free.
-
All emojis are served from AWS S3.
-
We do not store your messages. Your app backend will have to process and serve messages created with our SDK.
-
We do not send push notifications.
-
Your app's message volume does not affect the performance of our SDK.
-
Messages are composed of simple HTML containing image and paragraph tags. Formatting is presented as inline CSS.
-
Will work with any built-in iOS keyboard or return type
-
All network operations happen asyncronously and do not block the User Interface
Service Performance
-
Avg Service Repsonse Time: 100ms
-
Hosted with AWS using Elastic Beanstalk & RDS
-
Scales seamlessly to meet traffic demands

HTML의 미래는 무한한 가능성으로 가득합니다. 1) 새로운 기능과 표준에는 더 많은 의미 론적 태그와 WebComponents의 인기가 포함됩니다. 2) 웹 디자인 트렌드는 반응적이고 접근 가능한 디자인을 향해 계속 발전 할 것입니다. 3) 성능 최적화는 반응 형 이미지 로딩 및 게으른로드 기술을 통해 사용자 경험을 향상시킬 것입니다.

웹 개발에서 HTML, CSS 및 JavaScript의 역할은 다음과 같습니다. HTML은 컨텐츠 구조를 담당하고 CSS는 스타일을 담당하며 JavaScript는 동적 동작을 담당합니다. 1. HTML은 태그를 통해 웹 페이지 구조와 컨텐츠를 정의하여 의미를 보장합니다. 2. CSS는 선택기와 속성을 통해 웹 페이지 스타일을 제어하여 아름답고 읽기 쉽게 만듭니다. 3. JavaScript는 스크립트를 통해 웹 페이지 동작을 제어하여 동적 및 대화식 기능을 달성합니다.

Htmlisnotaprogramminglanguage; itisamarkuplanguage.1) htmlstructuresandformatswebcontentusingtags.2) itworksporstylingandjavaScriptOfforIncincivity, WebDevelopment 향상.

HTML은 웹 페이지 구조를 구축하는 초석입니다. 1. HTML은 컨텐츠 구조와 의미론 및 사용 등을 정의합니다. 태그. 2. SEO 효과를 향상시키기 위해 시맨틱 마커 등을 제공합니다. 3. 태그를 통한 사용자 상호 작용을 실현하려면 형식 검증에주의를 기울이십시오. 4. 자바 스크립트와 결합하여 동적 효과를 달성하기 위해 고급 요소를 사용하십시오. 5. 일반적인 오류에는 탈수 된 레이블과 인용되지 않은 속성 값이 포함되며 검증 도구가 필요합니다. 6. 최적화 전략에는 HTTP 요청 감소, HTML 압축, 시맨틱 태그 사용 등이 포함됩니다.

HTML은 웹 페이지를 작성하는 데 사용되는 언어로, 태그 및 속성을 통해 웹 페이지 구조 및 컨텐츠를 정의합니다. 1) HTML과 같은 태그를 통해 문서 구조를 구성합니다. 2) 브라우저는 HTML을 구문 분석하여 DOM을 빌드하고 웹 페이지를 렌더링합니다. 3) 멀티미디어 기능을 향상시키는 HTML5의 새로운 기능. 4) 일반적인 오류에는 탈수 된 레이블과 인용되지 않은 속성 값이 포함됩니다. 5) 최적화 제안에는 시맨틱 태그 사용 및 파일 크기 감소가 포함됩니다.

WebDevelopmentReliesonHtml, CSS 및 JavaScript : 1) HtmlStructuresContent, 2) CSSSTYLESIT, 및 3) JAVASCRIPTADDSINGINTERACTIVITY, BASISOFMODERNWEBEXPERIENCES를 형성합니다.

HTML의 역할은 태그 및 속성을 통해 웹 페이지의 구조와 내용을 정의하는 것입니다. 1. HTML은 읽기 쉽고 이해하기 쉽게하는 태그를 통해 컨텐츠를 구성합니다. 2. 접근성 및 SEO와 같은 시맨틱 태그 등을 사용하십시오. 3. HTML 코드를 최적화하면 웹 페이지로드 속도 및 사용자 경험이 향상 될 수 있습니다.

"Code"는 "Code"BroadlyIncludeLugageslikeJavaScriptandPyThonforFunctureS (htMlisAspecificTypeofCodeFocudecturecturingWebContent)


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
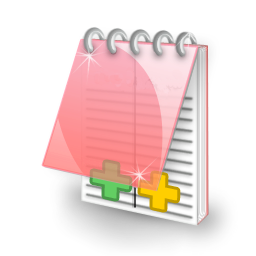
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음
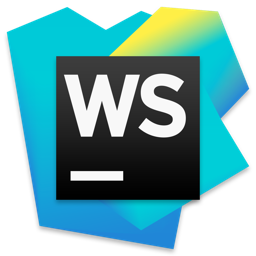
WebStorm Mac 버전
유용한 JavaScript 개발 도구

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.

SublimeText3 영어 버전
권장 사항: Win 버전, 코드 프롬프트 지원!

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경
