C# 글로벌 단축키 설정 안내
글로벌 단축키를 사용하면 프로그램에 초점이 맞지 않을 때에도 키 입력을 캡처할 수 있습니다. 이는 바로 가기 키를 구현하거나 키 조합으로 트리거되는 특정 작업을 수행하는 데 유용합니다. 다음은 C#을 사용하여 전역 단축키를 설정하는 방법에 대한 자세한 지침입니다.
단축키 처리 클래스 만들기
먼저 단축키 등록 및 캡처를 처리하기 위해 KeyboardHook
이라는 클래스를 만듭니다.
public sealed class KeyboardHook : IDisposable { // 导入必要的Win32 API函数 [DllImport("user32.dll")] private static extern bool RegisterHotKey(IntPtr hWnd, int id, uint fsModifiers, uint vk); [DllImport("user32.dll")] private static extern bool UnregisterHotKey(IntPtr hWnd, int id); // 定义一个类来处理窗口消息 private class Window : NativeWindow, IDisposable { ... // 处理Win32热键消息 protected override void WndProc(ref Message m) { ... // 调用事件以通知父级 if (KeyPressed != null) KeyPressed(this, new KeyPressedEventArgs(modifier, key)); } } private Window _window = new Window(); private int _currentId; // 注册热键 public void RegisterHotKey(ModifierKeys modifier, Keys key) { ... // 注册热键 if (!RegisterHotKey(_window.Handle, _currentId, (uint)modifier, (uint)key)) throw new InvalidOperationException("无法注册热键。"); } }
단축키 등록
KeyboardHook
클래스를 인스턴스화하고 RegisterHotKey
메서드를 사용하여 원하는 단축키 조합을 등록합니다. 예를 들어 Ctrl, Alt 및 F12 키를 단축키로 등록하려면:
hook.RegisterHotKey(ModifierKeys.Control | ModifierKeys.Alt, Keys.F12);
단축키 이벤트 처리
핫키를 눌렀을 때 코드를 실행하려면 KeyboardHook
클래스 KeyPressed
이벤트
hook.KeyPressed += new EventHandler<KeyPressedEventArgs>(hook_KeyPressed);
이벤트 핸들러에서는 원하는 작업이나 논리를 수행할 수 있습니다.
로그아웃 단축키
메모리 누수를 방지하려면 프로그램이 종료되거나 단축키가 더 이상 필요하지 않은 경우 단축키를 로그아웃하세요.
hook.Dispose();
중요 팁
- 이 기술을 사용하려면 콘솔 애플리케이션 프로젝트가 아닌 Windows Forms 프로젝트가 필요합니다.
- 적절한 동기화 메커니즘이 없는 다중 스레드 환경에서는
NativeWindow
클래스를 사용하지 마세요. -
RegisterHotKey
기능 애플리케이션당 단축키 조합은 256개로 제한됩니다.
위 내용은 Win32 API를 사용하여 C#에서 전역 단축키를 설정하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
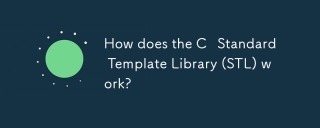
이 기사에서는 컨테이너, 반복자, 알고리즘 및 함수 인 핵심 구성 요소에 중점을 둔 C 표준 템플릿 라이브러리 (STL)에 대해 설명합니다. 일반적인 프로그래밍을 가능하게하기 위해 이러한 상호 작용, 코드 효율성 및 가독성 개선 방법에 대해 자세히 설명합니다.
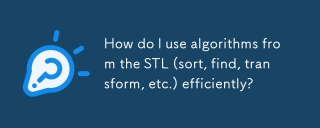
이 기사는 효율적인 STL 알고리즘 사용을 자세히 설명합니다. 데이터 구조 선택 (벡터 대 목록), 알고리즘 복잡성 분석 (예 : std :: sort vs. std :: partial_sort), 반복자 사용 및 병렬 실행을 강조합니다. 일반적인 함정과 같은
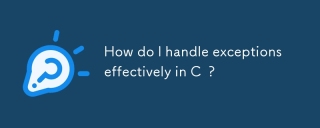
이 기사는 C에서 효과적인 예외 처리를 자세히 설명하고, 시도, 캐치 및 던지기 메커니즘을 다룹니다. RAII와 같은 모범 사례, 불필요한 캐치 블록을 피하고 강력한 코드에 대한 예외를 기록합니다. 이 기사는 또한 Perf를 다룹니다
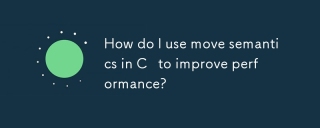
이 기사는 C에서 Move Semantics를 사용하여 불필요한 복사를 피함으로써 성능을 향상시키는 것에 대해 논의합니다. STD :: MOVE를 사용하여 이동 생성자 및 할당 연산자 구현을 다루고 효과적인 APPL을위한 주요 시나리오 및 함정을 식별합니다.
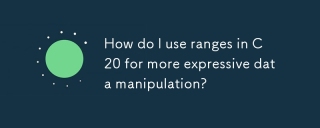
C 20 범위는 표현성, 합성 가능성 및 효율성으로 데이터 조작을 향상시킵니다. 더 나은 성능과 유지 관리를 위해 복잡한 변환을 단순화하고 기존 코드베이스에 통합합니다.
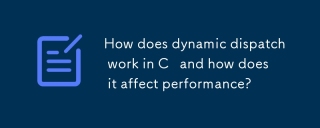
이 기사는 C의 동적 파견, 성능 비용 및 최적화 전략에 대해 설명합니다. 동적 파견이 성능에 영향을 미치는 시나리오를 강조하고이를 정적 파견과 비교하여 성능과 성능 간의 트레이드 오프를 강조합니다.
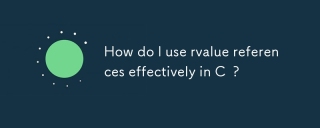
기사는 Move Semantics, Perfect Forwarding 및 Resource Management에 대한 C에서 RValue 참조의 효과적인 사용에 대해 논의하여 모범 사례 및 성능 향상을 강조합니다 (159 자).
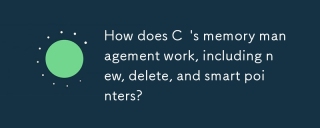
C 메모리 관리는 새로운, 삭제 및 스마트 포인터를 사용합니다. 이 기사는 매뉴얼 대 자동화 된 관리 및 스마트 포인터가 메모리 누출을 방지하는 방법에 대해 설명합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
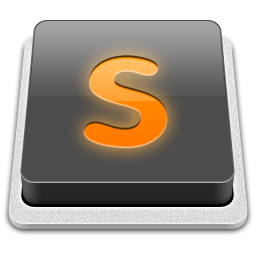
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.
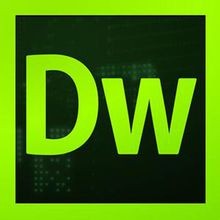
드림위버 CS6
시각적 웹 개발 도구
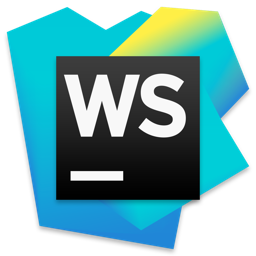
WebStorm Mac 버전
유용한 JavaScript 개발 도구
