이 기사에서는 .map()
루프를 사용하여 해당 기능을 다시 만들어 일반적인 JavaScript 배열 메서드(.includes()
, .concat()
, .flat()
및 for
)를 설명합니다. for
루프는 덜 효율적이고 읽기 어려워 보일 수 있지만 기본 메커니즘을 이해하면 내장 배열 방법에 대한 더 깊은 이해를 제공합니다. 뛰어들어 보세요!
.map()
.map()
메서드는 기존 배열의 각 요소에 함수를 적용하여 새 배열을 생성합니다. 비어 있지 않은 요소만 처리하고 원래 배열은 변경되지 않은 채로 둡니다.
데이터 구조의 고객 이름 목록이 필요한 은행 애플리케이션을 생각해 보세요. .map()
가 가장 효율적인 솔루션이지만 for
루프를 사용하여 이를 달성하는 방법을 살펴보겠습니다.
const bankAccounts = [ { id: 1, name: "Susan", balance: 100.32, deposits: [150, 30, 221], withdrawals: [110, 70.68, 120], }, { id: 2, name: "Morgan", balance: 1100.0, deposits: [1100] }, { id: 3, name: "Joshua", balance: 18456.57, deposits: [4000, 5000, 6000, 9200, 256.57], withdrawals: [1500, 1400, 1500, 1500], }, { id: 4, name: "Candy", balance: 0.0 }, { id: 5, name: "Phil", balance: 18, deposits: [100, 18], withdrawals: [100] }, ]; // Using a 'for' loop: function getAllClientNamesLoop(array) { const acctNames = []; for (const acct of array) { acctNames.push(acct.name); } return acctNames; } // Using .map(): function getAllClientNamesMap(array) { return array.map(acct => acct.name); } console.log(getAllClientNamesLoop(bankAccounts)); // Output: ['Susan', 'Morgan', 'Joshua', 'Candy', 'Phil'] console.log(getAllClientNamesMap(bankAccounts)); // Output: ['Susan', 'Morgan', 'Joshua', 'Candy', 'Phil']
for
루프 접근 방식을 사용하려면 빈 배열을 만들고 반복적으로 이름을 푸시해야 합니다. .map()
방법이 훨씬 더 간결하고 읽기 쉽습니다.
.includes()
이 메서드는 배열에 특정 값이 포함되어 있는지 확인하여 true
또는 false
을 반환합니다.
for
루프와 .includes()
:
// Using a 'for' loop: function doesArrayIncludeLoop(array, value) { let isIncluded = false; for (const elem of array) { if (elem === value) { isIncluded = true; break; // Optimization: Exit loop once value is found } } return isIncluded; } // Using .includes(): function doesArrayIncludeIncludes(array, value) { return array.includes(value); } console.log(doesArrayIncludeLoop([1, 1, 2, 3, 5], 6)); // Output: false console.log(doesArrayIncludeIncludes([1, 1, 2, 3, 5], 3)); // Output: true
for
루프 버전은 플래그를 초기화하고 반복하는 반면 .includes()
은 직접적이고 깔끔한 솔루션을 제공합니다.
.concat()
이 방법은 두 개 이상의 배열을 하나의 새 배열로 병합합니다.
다음은 for
루프와 .concat()
을 사용한 비교입니다.
// Using 'for' loops: function concatArraysLoop(arr1, arr2) { const concatenatedArray = [...arr1, ...arr2]; //Spread syntax for conciseness return concatenatedArray; } // Using .concat(): function concatArraysConcat(arr1, arr2) { return arr1.concat(arr2); } console.log(concatArraysLoop([0, 1, 2], [5, 6, 7])); // Output: [0, 1, 2, 5, 6, 7] console.log(concatArraysConcat([0, 1, 2], [5, 6, 7])); // Output: [0, 1, 2, 5, 6, 7]
다시 말하지만, .concat()
는 뛰어난 간결성과 가독성을 제공합니다.
.flat()
.flat()
메서드는 중첩 배열을 단일 수준 배열로 평면화합니다. 선택적 깊이 매개변수를 허용합니다.
두 가지 방법을 모두 사용하여 배열을 한 수준 깊이로 평면화해 보겠습니다.
// Using 'for' loops: function flatArraysLoop(array) { const flattenedArray = []; for (const elem of array) { if (Array.isArray(elem)) { flattenedArray.push(...elem); //Spread syntax } else { flattenedArray.push(elem); } } return flattenedArray; } // Using .flat(): function flatArraysFlat(array) { return array.flat(); } console.log(flatArraysLoop([1, 2, 3, [4, [5, 6]], 10])); // Output: [1, 2, 3, 4, [5, 6], 10] console.log(flatArraysFlat([1, 2, 3, [4, [5, 6]], 10])); // Output: [1, 2, 3, 4, [5, 6], 10]
for
루프를 사용하여 더 깊게 중첩된 배열을 평면화하면 .flat(depth)
의 강력함과 단순성이 강조되면서 점점 더 복잡해집니다.
이 탐색은 JavaScript에 내장된 배열 메소드의 우아함과 효율성을 보여줍니다. 2부에서는 더 많은 배열 방법을 살펴보겠습니다. 여러분의 생각과 질문을 자유롭게 공유해주세요! (2부 링크는 여기에 있습니다)
위 내용은 JavaScript 배열 메서드, 내부(부분)의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
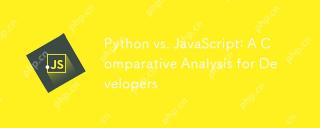
Python과 JavaScript의 주요 차이점은 유형 시스템 및 응용 프로그램 시나리오입니다. 1. Python은 과학 컴퓨팅 및 데이터 분석에 적합한 동적 유형을 사용합니다. 2. JavaScript는 약한 유형을 채택하며 프론트 엔드 및 풀 스택 개발에 널리 사용됩니다. 두 사람은 비동기 프로그래밍 및 성능 최적화에서 고유 한 장점을 가지고 있으며 선택할 때 프로젝트 요구 사항에 따라 결정해야합니다.
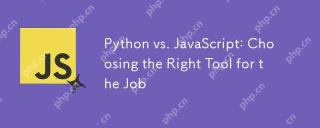
Python 또는 JavaScript를 선택할지 여부는 프로젝트 유형에 따라 다릅니다. 1) 데이터 과학 및 자동화 작업을 위해 Python을 선택하십시오. 2) 프론트 엔드 및 풀 스택 개발을 위해 JavaScript를 선택하십시오. Python은 데이터 처리 및 자동화 분야에서 강력한 라이브러리에 선호되는 반면 JavaScript는 웹 상호 작용 및 전체 스택 개발의 장점에 없어서는 안될 필수입니다.
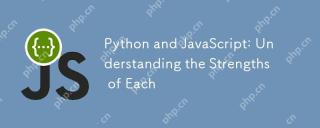
파이썬과 자바 스크립트는 각각 고유 한 장점이 있으며 선택은 프로젝트 요구와 개인 선호도에 따라 다릅니다. 1. Python은 간결한 구문으로 데이터 과학 및 백엔드 개발에 적합하지만 실행 속도가 느립니다. 2. JavaScript는 프론트 엔드 개발의 모든 곳에 있으며 강력한 비동기 프로그래밍 기능을 가지고 있습니다. node.js는 풀 스택 개발에 적합하지만 구문은 복잡하고 오류가 발생할 수 있습니다.
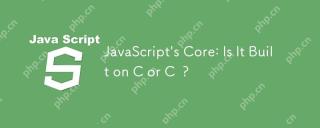
javaScriptisNotBuiltoncorc; it'SangretedLanguageThatrunsonOngineStenWrittenInc .1) javaScriptWasDesignEdasAlightweight, 해석 hanguageforwebbrowsers.2) Endinesevolvedfromsimpleplemporectreterstoccilpilers, 전기적으로 개선된다.
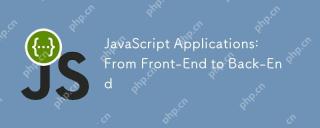
JavaScript는 프론트 엔드 및 백엔드 개발에 사용할 수 있습니다. 프론트 엔드는 DOM 작업을 통해 사용자 경험을 향상시키고 백엔드는 Node.js를 통해 서버 작업을 처리합니다. 1. 프론트 엔드 예 : 웹 페이지 텍스트의 내용을 변경하십시오. 2. 백엔드 예제 : node.js 서버를 만듭니다.
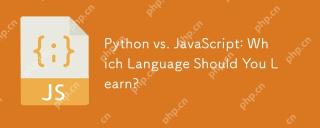
Python 또는 JavaScript는 경력 개발, 학습 곡선 및 생태계를 기반으로해야합니다. 1) 경력 개발 : Python은 데이터 과학 및 백엔드 개발에 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 적합합니다. 2) 학습 곡선 : Python 구문은 간결하며 초보자에게 적합합니다. JavaScript Syntax는 유연합니다. 3) 생태계 : Python에는 풍부한 과학 컴퓨팅 라이브러리가 있으며 JavaScript는 강력한 프론트 엔드 프레임 워크를 가지고 있습니다.
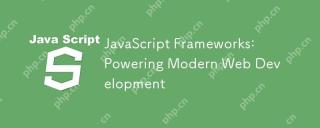
JavaScript 프레임 워크의 힘은 개발 단순화, 사용자 경험 및 응용 프로그램 성능을 향상시키는 데 있습니다. 프레임 워크를 선택할 때 : 1. 프로젝트 규모와 복잡성, 2. 팀 경험, 3. 생태계 및 커뮤니티 지원.
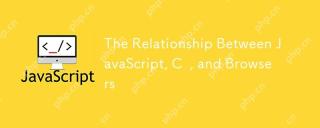
서론 나는 당신이 이상하다는 것을 알고 있습니다. JavaScript, C 및 Browser는 정확히 무엇을해야합니까? 그들은 관련이없는 것처럼 보이지만 실제로는 현대 웹 개발에서 매우 중요한 역할을합니다. 오늘 우리는이 세 가지 사이의 밀접한 관계에 대해 논의 할 것입니다. 이 기사를 통해 브라우저에서 JavaScript가 어떻게 실행되는지, 브라우저 엔진의 C 역할 및 웹 페이지의 렌더링 및 상호 작용을 유도하기 위해 함께 작동하는 방법을 알게됩니다. 우리는 모두 JavaScript와 브라우저의 관계를 알고 있습니다. JavaScript는 프론트 엔드 개발의 핵심 언어입니다. 브라우저에서 직접 실행되므로 웹 페이지를 생생하고 흥미롭게 만듭니다. 왜 Javascr


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전
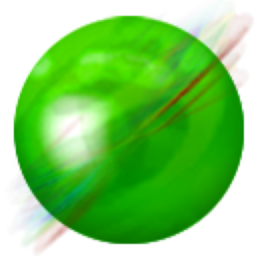
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경

SecList
SecLists는 최고의 보안 테스터의 동반자입니다. 보안 평가 시 자주 사용되는 다양한 유형의 목록을 한 곳에 모아 놓은 것입니다. SecLists는 보안 테스터에게 필요할 수 있는 모든 목록을 편리하게 제공하여 보안 테스트를 더욱 효율적이고 생산적으로 만드는 데 도움이 됩니다. 목록 유형에는 사용자 이름, 비밀번호, URL, 퍼징 페이로드, 민감한 데이터 패턴, 웹 셸 등이 포함됩니다. 테스터는 이 저장소를 새로운 테스트 시스템으로 간단히 가져올 수 있으며 필요한 모든 유형의 목록에 액세스할 수 있습니다.
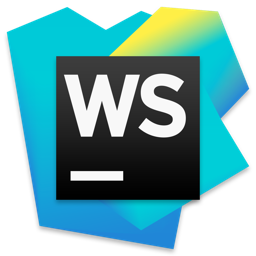
WebStorm Mac 버전
유용한 JavaScript 개발 도구

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구