JavaScript에서 '커링'을 접하고 그 목적이 궁금하신가요? 이 게시물에서는 코드 명확성과 유연성을 향상시키는 간단한 예와 실제 응용 프로그램을 통해 카레의 이점을 설명하고 카레의 신비를 풀었습니다.
? 커링이란 무엇인가요?
함수형 프로그래밍 기술인 커링(Currying)에는 함수 인수를 한꺼번에 처리하는 대신 순차적으로 처리하는 방식이 포함됩니다. 카레 함수는 모든 인수가 제공될 때까지 다음 매개변수를 기다리는 또 다른 함수를 생성합니다. 기본적으로 다중 인수 함수를 일련의 단일 인수 함수로 변환합니다.
실제 비유와 코드로 설명해 보겠습니다.
? 버거 만들기
버거를 주문한다고 상상해 보세요. 셰프가 겹겹이 조립합니다:
레이어 1: Bun(첫 번째 인수) 레이어 2: 패티(두 번째 인수) 레이어 3: 토핑(세 번째 인수)
일반 함수와 카레 함수를 사용하는 코드로 변환하는 방법은 다음과 같습니다.
? 일반 기능: 모든 재료가 동시에 통과됩니다.
function makeBurger(bun, patty, topping) { return `Your burger includes: ${bun} bun, ${patty} patty, and ${topping} topping.`; } const myBurger = makeBurger("Sesame", "Beef", "Cheese"); console.log(myBurger); // Output: Your burger includes: Sesame bun, Beef patty, and Cheese topping.
? 카레 기능: 재료를 하나씩 추가합니다.
function makeBurgerCurried(bun) { return function (patty) { return function (topping) { return `Your burger includes: ${bun} bun, ${patty} patty, and ${topping} topping.`; }; }; } // Usage example const selectBun = makeBurgerCurried("Sesame"); const selectPatty = selectBun("Beef"); const myCurriedBurger = selectPatty("Cheese"); console.log(myCurriedBurger); // Output: Your burger includes: Sesame bun, Beef patty, and Cheese topping.
✍️ 설명:
첫 번째 호출: makeBurgerCurried("Sesame")
은 "Sesame"을 수신하고 패티를 기다리는 함수를 반환합니다.
두 번째 호출: selectBun("Beef")
은 "쇠고기"를 수신하고 토핑을 기다리는 함수를 반환합니다.
세 번째 호출: selectPatty("Cheese")
은 "치즈"를 수신하여 시퀀스를 완료하고 버거 설명을 반환합니다.
⭐ 화살표 기능을 사용한 간소화된 커링
화살표 함수는 보다 간결한 접근 방식을 제공합니다.
const curriedArrow = (bun) => (patty) => (topping) => `Your burger includes: ${bun} bun, ${patty} patty, and ${topping} topping`; const myArrowBurger = curriedArrow("Sesame")("Beef")("Cheese"); console.log(myArrowBurger); // Your burger includes: Sesame bun, Beef patty, and Cheese topping
⁉️ 커링을 사용하는 이유는 무엇인가요?
Currying은 미리 정의된 인수를 사용하여 함수를 재사용해야 하는 시나리오에서 탁월합니다. 코드 재사용성, 가독성 및 모듈성을 향상시킵니다.
? 실제 적용: 동적 할인 계산기
단계별 할인이 제공되는 전자상거래 플랫폼을 고려해 보세요.
- 단골고객: 10% 할인
- 프리미엄 고객: 20% 할인
일반 함수 구현과 카레 함수 구현을 비교해 보겠습니다.
? 일반 기능: 유연성이 낮고 재사용성이 낮습니다.
function calculateDiscount(customerType, price) { if (customerType === "Regular") return price * 0.9; else if (customerType === "Premium") return price * 0.8; } console.log(calculateDiscount("Regular", 100)); // Output: 90 console.log(calculateDiscount("Premium", 100)); // Output: 80
? 카레 기능: 재사용성과 적응성이 뛰어납니다.
function createDiscountCalculator(discountRate) { return function (price) { return price * (1 - discountRate); }; } const regularDiscount = createDiscountCalculator(0.1); const premiumDiscount = createDiscountCalculator(0.2); console.log(regularDiscount(100)); // Output: 90 console.log(premiumDiscount(100)); // Output: 80 console.log(regularDiscount(200)); // Output: 180
새 고객 유형을 추가하는 방법은 간단합니다.
const studentDiscount = createDiscountCalculator(0.15); console.log(studentDiscount(100)); // Output: 85
결론
커링은 처음에는 복잡해 보이지만 함수 설계를 단순화하고 코드 명확성을 향상시키며 재사용성을 촉진합니다. 카레를 프로젝트에 포함시켜 그 장점을 직접 경험해보세요.
아래 댓글로 카레에 대한 여러분의 경험을 공유해 주세요! 즐거운 코딩하세요! ✨
위 내용은 실제 애플리케이션으로 JavaScript Currying 이해하기의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
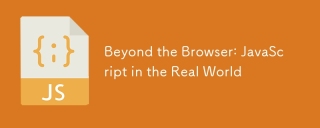
실제 세계에서 JavaScript의 응용 프로그램에는 서버 측 프로그래밍, 모바일 애플리케이션 개발 및 사물 인터넷 제어가 포함됩니다. 1. 서버 측 프로그래밍은 Node.js를 통해 실현되며 동시 요청 처리에 적합합니다. 2. 모바일 애플리케이션 개발은 재교육을 통해 수행되며 크로스 플랫폼 배포를 지원합니다. 3. Johnny-Five 라이브러리를 통한 IoT 장치 제어에 사용되며 하드웨어 상호 작용에 적합합니다.
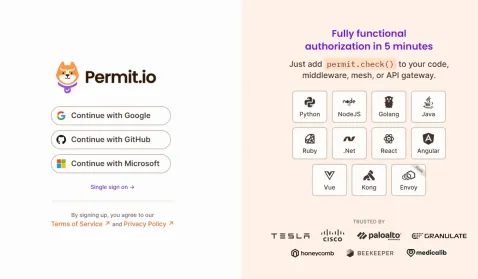
일상적인 기술 도구를 사용하여 기능적 다중 테넌트 SaaS 응용 프로그램 (Edtech 앱)을 구축했으며 동일한 작업을 수행 할 수 있습니다. 먼저, 다중 테넌트 SaaS 응용 프로그램은 무엇입니까? 멀티 테넌트 SAAS 응용 프로그램은 노래에서 여러 고객에게 서비스를 제공 할 수 있습니다.
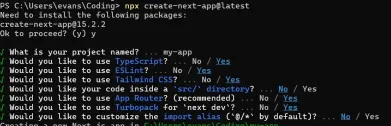
이 기사에서는 Contrim에 의해 확보 된 백엔드와의 프론트 엔드 통합을 보여 주며 Next.js를 사용하여 기능적인 Edtech SaaS 응용 프로그램을 구축합니다. Frontend는 UI 가시성을 제어하기 위해 사용자 권한을 가져오고 API가 역할 기반을 준수하도록합니다.
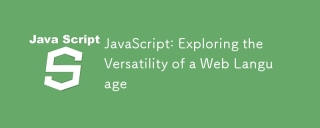
JavaScript는 현대 웹 개발의 핵심 언어이며 다양성과 유연성에 널리 사용됩니다. 1) 프론트 엔드 개발 : DOM 운영 및 최신 프레임 워크 (예 : React, Vue.js, Angular)를 통해 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축합니다. 2) 서버 측 개발 : Node.js는 비 차단 I/O 모델을 사용하여 높은 동시성 및 실시간 응용 프로그램을 처리합니다. 3) 모바일 및 데스크탑 애플리케이션 개발 : 크로스 플랫폼 개발은 개발 효율을 향상시키기 위해 반응 및 전자를 통해 실현됩니다.
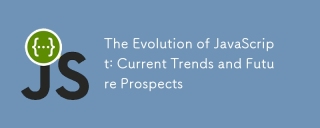
JavaScript의 최신 트렌드에는 Typescript의 Rise, 현대 프레임 워크 및 라이브러리의 인기 및 WebAssembly의 적용이 포함됩니다. 향후 전망은보다 강력한 유형 시스템, 서버 측 JavaScript 개발, 인공 지능 및 기계 학습의 확장, IoT 및 Edge 컴퓨팅의 잠재력을 포함합니다.
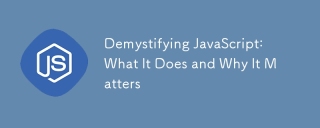
JavaScript는 현대 웹 개발의 초석이며 주요 기능에는 이벤트 중심 프로그래밍, 동적 컨텐츠 생성 및 비동기 프로그래밍이 포함됩니다. 1) 이벤트 중심 프로그래밍을 사용하면 사용자 작업에 따라 웹 페이지가 동적으로 변경 될 수 있습니다. 2) 동적 컨텐츠 생성을 사용하면 조건에 따라 페이지 컨텐츠를 조정할 수 있습니다. 3) 비동기 프로그래밍은 사용자 인터페이스가 차단되지 않도록합니다. JavaScript는 웹 상호 작용, 단일 페이지 응용 프로그램 및 서버 측 개발에 널리 사용되며 사용자 경험 및 크로스 플랫폼 개발의 유연성을 크게 향상시킵니다.
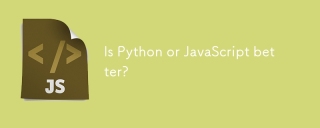
Python은 데이터 과학 및 기계 학습에 더 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 더 적합합니다. 1. Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명하며 데이터 분석 및 웹 개발에 적합합니다. 2. JavaScript는 프론트 엔드 개발의 핵심입니다. Node.js는 서버 측 프로그래밍을 지원하며 풀 스택 개발에 적합합니다.
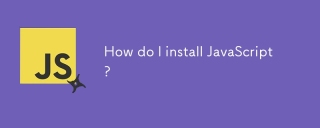
JavaScript는 이미 최신 브라우저에 내장되어 있기 때문에 설치가 필요하지 않습니다. 시작하려면 텍스트 편집기와 브라우저 만 있으면됩니다. 1) 브라우저 환경에서 태그를 통해 HTML 파일을 포함하여 실행하십시오. 2) Node.js 환경에서 Node.js를 다운로드하고 설치 한 후 명령 줄을 통해 JavaScript 파일을 실행하십시오.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기
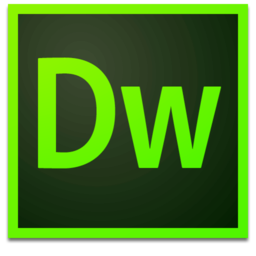
Dreamweaver Mac版
시각적 웹 개발 도구

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전
