32비트 .NET 애플리케이션에서 64비트 레지스트리 항목에 액세스
64비트 Windows 시스템에서 실행되는 32비트 애플리케이션에서 64비트 레지스트리에 액세스하려면 특정 접근 방식이 필요합니다. 다행히 .NET Framework 4.x 이상 버전에서는 이를 기본적으로 지원합니다.
64비트 레지스트리 액세스를 위해 RegistryView 활용
RegistryView
열거형은 32비트와 64비트 레지스트리 액세스를 구별하는 핵심입니다. 사용 방법은 다음과 같습니다.
// Access the 64-bit registry using Microsoft.Win32; RegistryKey localKey64 = RegistryKey.OpenBaseKey(RegistryHive.LocalMachine, RegistryView.Registry64); RegistryKey sqlServerKey64 = localKey64.OpenSubKey(@"SOFTWARE\Microsoft\Microsoft SQL Server\Instance Names\SQL"); // Access the 32-bit registry RegistryKey localKey32 = RegistryKey.OpenBaseKey(RegistryHive.LocalMachine, RegistryView.Registry32); RegistryKey sqlServerKey32 = localKey32.OpenSubKey(@"SOFTWARE\Microsoft\Microsoft SQL Server\Instance Names\SQL");
특정 레지스트리 값 검색
"Instance NamesSQL" 키 아래의 "SQLEXPRESS"와 같은 특정 값을 검색하려면 다음을 사용하세요.
string sqlExpressKeyName = (string)sqlServerKey64.GetValue("SQLEXPRESS");
포괄적인 키 검색: 32비트 및 64비트 결과 결합
32비트 및 64비트 레지스트리 위치 모두의 데이터가 필요한 상황에서는 결합된 쿼리가 유용합니다.
using System.Linq; IEnumerable<string> GetAllRegValueNames(string regPath) { var reg64 = GetRegValueNames(RegistryView.Registry64, regPath); var reg32 = GetRegValueNames(RegistryView.Registry32, regPath); var result = (reg64 != null && reg32 != null) ? reg64.Union(reg32) : (reg64 ?? reg32); return (result ?? new List<string>().AsEnumerable()).OrderBy(x => x); }
여기서 GetRegValueNames
은 지정된 키에서 값 이름을 검색하는 도우미 함수(표시되지 않지만 쉽게 구현됨)입니다. regPath
은 레지스트리 경로를 지정합니다.
사용 예:
var sqlRegPath = @"SOFTWARE\Microsoft\Microsoft SQL Server\Instance Names\SQL"; foreach (var keyName in GetAllRegValueNames(sqlRegPath)) { Console.WriteLine(keyName); } ``` This iterates through all found keys, regardless of whether they reside in the 32-bit or 64-bit registry hive.
위 내용은 32비트 .NET 애플리케이션에서 64비트 레지스트리 키에 액세스하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
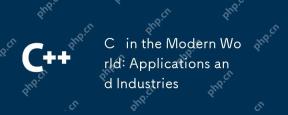
C는 현대 세계에서 널리 사용되고 중요합니다. 1) 게임 개발에서 C는 Unrealengine 및 Unity와 같은 고성능 및 다형성에 널리 사용됩니다. 2) 금융 거래 시스템에서 C의 낮은 대기 시간과 높은 처리량은 고주파 거래 및 실시간 데이터 분석에 적합한 첫 번째 선택입니다.
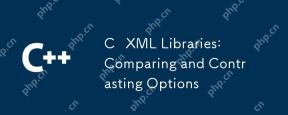
C : Tinyxml-2, Pugixml, XERCES-C 및 RapidXML에는 4 개의 일반적으로 사용되는 XML 라이브러리가 있습니다. 1. TINYXML-2는 자원이 제한적이고 경량이지만 제한된 기능을 가진 환경에 적합합니다. 2. PugixML은 빠르며 복잡한 XML 구조에 적합한 XPath 쿼리를 지원합니다. 3.xerces-c는 강력하고 DOM 및 SAX 해상도를 지원하며 복잡한 처리에 적합합니다. 4. RapidXML은 성능에 중점을두고 매우 빠르게 구문 분석하지만 XPath 쿼리를 지원하지는 않습니다.
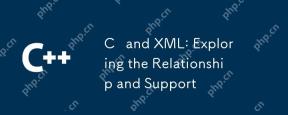
C는 XML과 타사 라이브러리 (예 : TinyXML, Pugixml, Xerces-C)와 상호 작용합니다. 1) 라이브러리를 사용하여 XML 파일을 구문 분석하고 C- 처리 가능한 데이터 구조로 변환하십시오. 2) XML을 생성 할 때 C 데이터 구조를 XML 형식으로 변환하십시오. 3) 실제 애플리케이션에서 XML은 종종 구성 파일 및 데이터 교환에 사용되어 개발 효율성을 향상시킵니다.
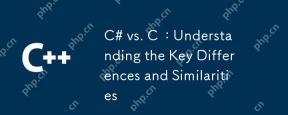
C#과 C의 주요 차이점은 구문, 성능 및 응용 프로그램 시나리오입니다. 1) C# 구문은 더 간결하고 쓰레기 수집을 지원하며 .NET 프레임 워크 개발에 적합합니다. 2) C는 성능이 높고 시스템 프로그래밍 및 게임 개발에 종종 사용되는 수동 메모리 관리가 필요합니다.
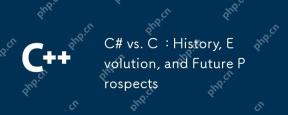
C#과 C의 역사와 진화는 독특하며 미래의 전망도 다릅니다. 1.C는 1983 년 Bjarnestroustrup에 의해 발명되어 객체 지향 프로그래밍을 C 언어에 소개했습니다. Evolution 프로세스에는 자동 키워드 소개 및 Lambda Expressions 소개 C 11, C 20 도입 개념 및 코 루틴과 같은 여러 표준화가 포함되며 향후 성능 및 시스템 수준 프로그래밍에 중점을 둘 것입니다. 2.C#은 2000 년 Microsoft에 의해 출시되었으며 C와 Java의 장점을 결합하여 진화는 단순성과 생산성에 중점을 둡니다. 예를 들어, C#2.0은 제네릭과 C#5.0 도입 된 비동기 프로그래밍을 소개했으며, 이는 향후 개발자의 생산성 및 클라우드 컴퓨팅에 중점을 둘 것입니다.
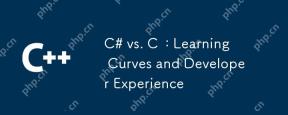
C# 및 C 및 개발자 경험의 학습 곡선에는 상당한 차이가 있습니다. 1) C#의 학습 곡선은 비교적 평평하며 빠른 개발 및 기업 수준의 응용 프로그램에 적합합니다. 2) C의 학습 곡선은 가파르고 고성능 및 저수준 제어 시나리오에 적합합니다.
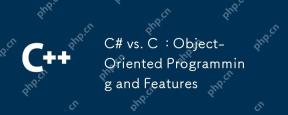
C# 및 C가 객체 지향 프로그래밍 (OOP)의 구현 및 기능에 상당한 차이가 있습니다. 1) C#의 클래스 정의 및 구문은 더 간결하고 LINQ와 같은 고급 기능을 지원합니다. 2) C는 시스템 프로그래밍 및 고성능 요구에 적합한 더 미세한 입상 제어를 제공합니다. 둘 다 고유 한 장점이 있으며 선택은 특정 응용 프로그램 시나리오를 기반으로해야합니다.
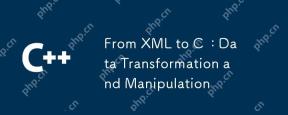
XML에서 C로 변환하고 다음 단계를 통해 수행 할 수 있습니다. 1) TinyxML2 라이브러리를 사용하여 XML 파일을 파싱하는 것은 2) C의 데이터 구조에 데이터를 매핑, 3) 데이터 운영을 위해 std :: 벡터와 같은 C 표준 라이브러리를 사용합니다. 이러한 단계를 통해 XML에서 변환 된 데이터를 효율적으로 처리하고 조작 할 수 있습니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기

SecList
SecLists는 최고의 보안 테스터의 동반자입니다. 보안 평가 시 자주 사용되는 다양한 유형의 목록을 한 곳에 모아 놓은 것입니다. SecLists는 보안 테스터에게 필요할 수 있는 모든 목록을 편리하게 제공하여 보안 테스트를 더욱 효율적이고 생산적으로 만드는 데 도움이 됩니다. 목록 유형에는 사용자 이름, 비밀번호, URL, 퍼징 페이로드, 민감한 데이터 패턴, 웹 셸 등이 포함됩니다. 테스터는 이 저장소를 새로운 테스트 시스템으로 간단히 가져올 수 있으며 필요한 모든 유형의 목록에 액세스할 수 있습니다.

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.
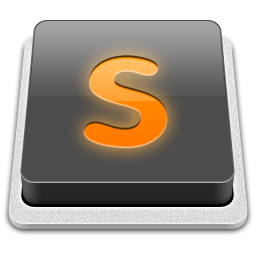
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
