본 글은 개인적인 의견이며 어떠한 내용도 언급하려는 것이 아닙니다.
Ruby나 JavaScript와 같은 언어에서 제가 가장 좋아하는 점 중 하나는 해당 언어의 변수가 객체라는 것입니다. 어떤 측면에서는 코드가 훨씬 더 읽기 쉽고 어떤 측면에서는 그렇지 않을 때도 있습니다.
예를 들면 다음과 같습니다.
# Ruby Program for length method. str = "Hello, world!" puts str.length # prints 13 to the console
PHP에서는
$str = 'Hello, world!'; echo strlen($str);
그렇지만 내 관점에서는 변수가 주어이고 메소드가 술어가 되기 때문에 Ruby나 JavaScript 형식이 더 읽기 쉽습니다.
PHP는 이러한 코드 작성 방식을 지원하지 않기 때문에 이를 수행할 수 있는 클래스를 만들었습니다. 그러나 클래스의 목적은 플레이만을 위한 것이며 프로덕션에서는 사용할 수 없습니다. 성능 문제가 있습니다.
<?php namespace Scalar; use Exception; use ReflectionFunction; class Scalar { /** * @var mixed * Value that can only be scalar. */ private $Scalar; /** * Constructor: Initializes the Scalar object. * @param mixed $Scalar * @throws Exception if the value is not scalar. */ public function __construct($Scalar) { if (!is_scalar($Scalar)) { throw new Exception('It\'s not a scalar value'); } $this->Scalar = $Scalar; } /** * Magic method: Dynamically calls a PHP function with the scalar value. * Supports named parameters if provided as an associative array. * @param string $method The name of the function to call. * @param array $arguments Additional arguments for the function. * @return mixed The result of the function call. * @throws Exception if the function does not exist. */ public function __call($method, $arguments) { if (!function_exists($method)) { throw new Exception('The function called ' . $method . ' doesn\'t exist'); } // Verificar si los argumentos son asociativos (named parameters) if (!empty($arguments) && array_keys($arguments) !== range(0, count($arguments) - 1)) { $refFunc = new ReflectionFunction($method); $params = $refFunc->getParameters(); $mappedArgs = []; foreach ($params as $param) { $name = $param->getName(); if (isset($arguments[$name])) { // Asignar el valor proporcionado $mappedArgs[] = $arguments[$name]; } elseif ($name === 'data') { // Insertar $this->Scalar si el parámetro es 'data' $mappedArgs[] = $this->Scalar; } elseif ($param->isDefaultValueAvailable()) { // Usar el valor predeterminado si está disponible $mappedArgs[] = $param->getDefaultValue(); } else { // Parámetro requerido sin valor proporcionado throw new Exception("Missing required parameter: $name for function $method"); } } return $refFunc->invokeArgs($mappedArgs); } else { // Llamada con argumentos posicionales (por defecto, insertar scalar al inicio) array_unshift($arguments, $this->Scalar); return call_user_func_array($method, $arguments); } } /** * Get the scalar value. * @return mixed */ public function getScalar() { return $this->Scalar; } }
잘 작동하려면 변수 이름 앞에 변수를 작성하는 기능을 사용해야 한다는 점을 명심하는 것도 중요합니다
<?PHP $data = new CustomerData( name: $input['name'], email: $input['email'], age: $input['age'], );
단일 매개변수 함수가 있는 클래스와 여러 매개변수가 있는 함수를 사용하는 예:
<?PHP try { $a = 'hola mundo'; $a_object = new Scalar($a); // Llamar a la función hash con named parameters $result = $a_object->hash(algo: 'sha256', binary: true); echo $result; // Hash binario de 'hola mundo' // Llamar a otras funciones echo $a_object->strlen(); // Devuelve 10 (longitud de 'hola mundo') } catch (Exception $e) { echo 'Error: ' . $e->getMessage(); }
위 내용은 마치 객체인 것처럼 변수를 사용하여 작업의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
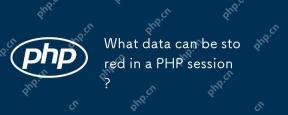
phpsessionscanstorestrings, 숫자, 배열 및 객체 1.Strings : TextDatalikeUsernames.2.numbers : integorfloatsforcounters.3.arrays : listslikeshoppingcarts.4.objects : complexStructuresThatareserialized.
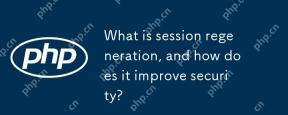
세션 재생은 세션 고정 공격의 경우 사용자가 민감한 작업을 수행 할 때 새 세션 ID를 생성하고 이전 ID를 무효화하는 것을 말합니다. 구현 단계에는 다음이 포함됩니다. 1. 민감한 작업 감지, 2. 새 세션 ID 생성, 3. 오래된 세션 ID 파괴, 4. 사용자 측 세션 정보 업데이트.
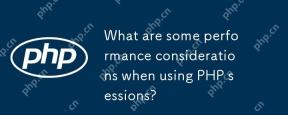
PHP 세션은 응용 프로그램 성능에 큰 영향을 미칩니다. 최적화 방법은 다음과 같습니다. 1. 데이터베이스를 사용하여 세션 데이터를 저장하여 응답 속도를 향상시킵니다. 2. 세션 데이터 사용을 줄이고 필요한 정보 만 저장하십시오. 3. 비 차단 세션 프로세서를 사용하여 동시성 기능을 향상시킵니다. 4. 사용자 경험과 서버 부담의 균형을 맞추기 위해 세션 만료 시간을 조정하십시오. 5. 영구 세션을 사용하여 데이터 읽기 및 쓰기 시간의 수를 줄입니다.
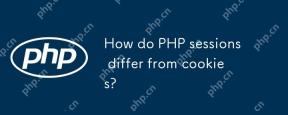
phpsessionsareser-side, whilecookiesareclient-side.1) sessions stessoredataontheserver, andhandlargerdata.2) cookiesstoredataonthecure, andlimitedinsize.usesessionsforsensitivestataondcookiesfornon-sensistive, client-sensation.
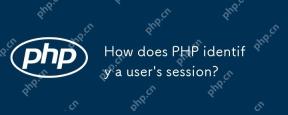
phpidifiesauser의 sssessionusessessioncookiesandssessionids.1) whensession_start () iscalled, phpgeneratesauniquessessionStoredInacookienamedPhpsSessIdonSeuser 'sbrowser.2) thisidallowsphptoretrievessessionDataTromServer.
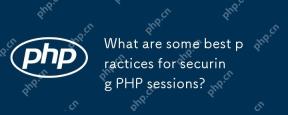
PHP 세션의 보안은 다음 측정을 통해 달성 할 수 있습니다. 1. Session_REGENEREAT_ID ()를 사용하여 사용자가 로그인하거나 중요한 작업 일 때 세션 ID를 재생합니다. 2. HTTPS 프로토콜을 통해 전송 세션 ID를 암호화합니다. 3. 세션 _save_path ()를 사용하여 세션 데이터를 저장하고 권한을 올바르게 설정할 보안 디렉토리를 지정하십시오.
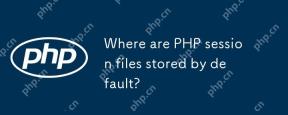
phpsessionfilesarestoredInTheRectorySpecifiedBysession.save_path, 일반적으로/tmponunix-likesystemsorc : \ windows \ temponwindows.tocustomizethis : 1) austession_save_path () toSetacustomDirectory, verlyTeCustory-swritation;


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.
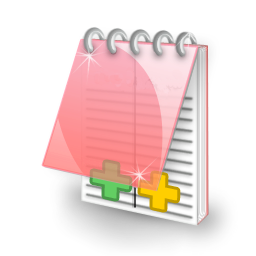
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 영어 버전
권장 사항: Win 버전, 코드 프롬프트 지원!
