원본 게시: souvikinator.xyz
API 엔드포인트에 대한 단위 테스트를 설정하는 방법은 다음과 같습니다.
우리는 다음 NPM 패키지를 사용할 것입니다:
- 서버용 Express(다른 프레임워크 사용 가능)
- 농담
- 슈퍼테스트
다음을 사용하는 VS 코드 확장:
- 농담
- 제스트러너
종속성 설치
npm install express
npm install -D jest supertest
디렉토리 구조는 다음과 같습니다.
package.json을 잊지 마세요
{ "name": "api-unit-testing", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "jest api.test.js", "start":"node server.js" }, "keywords": [], "author": "", "license": "ISC", "dependencies": { "express": "^4.18.1" }, "devDependencies": { "jest": "^28.1.2", "supertest": "^6.2.3" } }
간단한 익스프레스 서버 만들기
/post 엔드포인트에 본문과 제목이 있는 POST 요청을 수락하는 고속 서버입니다. 둘 중 하나라도 누락되면 상태 400 Bad Request가 반환됩니다.
// app.js const express = require("express"); const app = express(); app.use(express.json()); app.post("/post", (req, res) => { const { title, body } = req.body; if (!title || !body) return res.sendStatus(400).json({ error: "title and body is required" }); return res.sendStatus(200); }); module.exports = app;
const app = require("./app"); const port = 3000; app.listen(port, () => { console.log(`http://localhost:${port} ?`); });
supertest와 함께 사용하기 위해 Express 앱을 내보냅니다. 다른 HTTP 서버 프레임워크에서도 동일한 작업을 수행할 수 있습니다.
테스트 파일 생성
// api.test.js const supertest = require("supertest"); const app = require("./app"); // express app describe("Creating post", () => { // test cases goes here });
Supertest는 서버 운영과 요청을 대신 처리하고 우리는 테스트에 집중합니다.
express 앱은 supertest 에이전트로 전달되고 express를 임의의 사용 가능한 포트에 바인딩합니다. 그런 다음 원하는 API 엔드포인트에 HTTP 요청을 보내고 응답 상태를 예상과 비교할 수 있습니다.
const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", body: "Awesome post body", });
3가지 사례에 대한 테스트를 만들겠습니다.
- 제목과 본문을 모두 제공하는 경우
test("creating new post when both title and body are provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", body: "Awesome post body", }); // comparing response status code with expected status code expect(response.statusCode).toBe(200); });
- 둘 중 하나라도 누락된 경우
test("creating new post when either of the data is not provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", }); expect(response.statusCode).toBe(400); });
- 둘 다 빠졌을 때
test("creating new post when no data is not provided", async () => { const response = await supertest.agent(app).post("/post").send(); expect(response.statusCode).toBe(400); });
최종 코드는 다음과 같습니다.
const supertest = require("supertest"); const app = require("./app"); // express app describe("Creating post", () => { test("creating new post when both title and body are provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", body: "Awesome post body", }); expect(response.statusCode).toBe(200); }); test("creating new post when either of the data is not provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", }); expect(response.statusCode).toBe(400); }); test("creating new post when no data is not provided", async () => { const response = await supertest.agent(app).post("/post").send(); expect(response.statusCode).toBe(400); }); });
테스트 실행
다음 명령을 사용하여 테스트를 실행하세요.
npm run test
jest 및 jest runer와 같은 VS Code 확장을 사용하면 작업이 자동으로 수행됩니다.
이것이 API 엔드포인트를 쉽게 테스트할 수 있는 방법입니다. ?
위 내용은 Jest와 Supertest를 사용하여 REST API 테스트하기 ✅의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
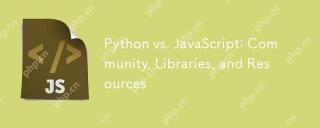
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
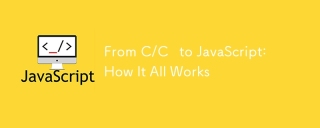
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
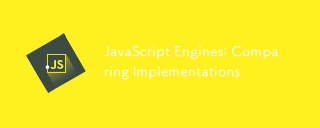
각각의 엔진의 구현 원리 및 최적화 전략이 다르기 때문에 JavaScript 엔진은 JavaScript 코드를 구문 분석하고 실행할 때 다른 영향을 미칩니다. 1. 어휘 분석 : 소스 코드를 어휘 단위로 변환합니다. 2. 문법 분석 : 추상 구문 트리를 생성합니다. 3. 최적화 및 컴파일 : JIT 컴파일러를 통해 기계 코드를 생성합니다. 4. 실행 : 기계 코드를 실행하십시오. V8 엔진은 즉각적인 컴파일 및 숨겨진 클래스를 통해 최적화하여 Spidermonkey는 유형 추론 시스템을 사용하여 동일한 코드에서 성능이 다른 성능을 제공합니다.
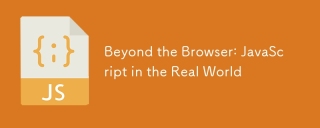
실제 세계에서 JavaScript의 응용 프로그램에는 서버 측 프로그래밍, 모바일 애플리케이션 개발 및 사물 인터넷 제어가 포함됩니다. 1. 서버 측 프로그래밍은 Node.js를 통해 실현되며 동시 요청 처리에 적합합니다. 2. 모바일 애플리케이션 개발은 재교육을 통해 수행되며 크로스 플랫폼 배포를 지원합니다. 3. Johnny-Five 라이브러리를 통한 IoT 장치 제어에 사용되며 하드웨어 상호 작용에 적합합니다.
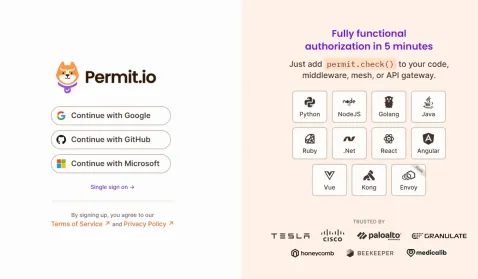
일상적인 기술 도구를 사용하여 기능적 다중 테넌트 SaaS 응용 프로그램 (Edtech 앱)을 구축했으며 동일한 작업을 수행 할 수 있습니다. 먼저, 다중 테넌트 SaaS 응용 프로그램은 무엇입니까? 멀티 테넌트 SAAS 응용 프로그램은 노래에서 여러 고객에게 서비스를 제공 할 수 있습니다.
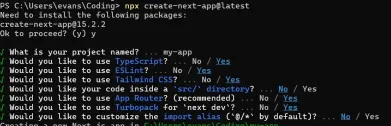
이 기사에서는 Contrim에 의해 확보 된 백엔드와의 프론트 엔드 통합을 보여 주며 Next.js를 사용하여 기능적인 Edtech SaaS 응용 프로그램을 구축합니다. Frontend는 UI 가시성을 제어하기 위해 사용자 권한을 가져오고 API가 역할 기반을 준수하도록합니다.
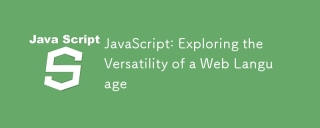
JavaScript는 현대 웹 개발의 핵심 언어이며 다양성과 유연성에 널리 사용됩니다. 1) 프론트 엔드 개발 : DOM 운영 및 최신 프레임 워크 (예 : React, Vue.js, Angular)를 통해 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축합니다. 2) 서버 측 개발 : Node.js는 비 차단 I/O 모델을 사용하여 높은 동시성 및 실시간 응용 프로그램을 처리합니다. 3) 모바일 및 데스크탑 애플리케이션 개발 : 크로스 플랫폼 개발은 개발 효율을 향상시키기 위해 반응 및 전자를 통해 실현됩니다.
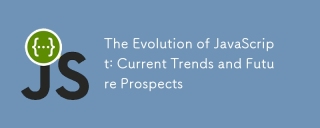
JavaScript의 최신 트렌드에는 Typescript의 Rise, 현대 프레임 워크 및 라이브러리의 인기 및 WebAssembly의 적용이 포함됩니다. 향후 전망은보다 강력한 유형 시스템, 서버 측 JavaScript 개발, 인공 지능 및 기계 학습의 확장, IoT 및 Edge 컴퓨팅의 잠재력을 포함합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.
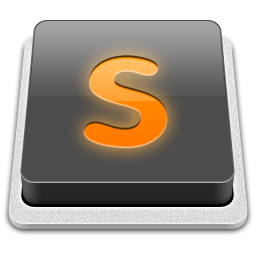
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

SecList
SecLists는 최고의 보안 테스터의 동반자입니다. 보안 평가 시 자주 사용되는 다양한 유형의 목록을 한 곳에 모아 놓은 것입니다. SecLists는 보안 테스터에게 필요할 수 있는 모든 목록을 편리하게 제공하여 보안 테스트를 더욱 효율적이고 생산적으로 만드는 데 도움이 됩니다. 목록 유형에는 사용자 이름, 비밀번호, URL, 퍼징 페이로드, 민감한 데이터 패턴, 웹 셸 등이 포함됩니다. 테스터는 이 저장소를 새로운 테스트 시스템으로 간단히 가져올 수 있으며 필요한 모든 유형의 목록에 액세스할 수 있습니다.
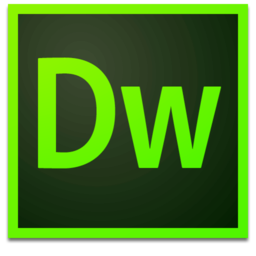
Dreamweaver Mac版
시각적 웹 개발 도구

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구
