인메모리 캐시 생성에 대한 이 가이드를 바탕으로 구성 가능한 데이터 지속성을 도입하여 더 나아가겠습니다. 어댑터 및 전략 패턴을 활용하여 캐싱 로직에서 스토리지 메커니즘을 분리하여 필요에 따라 데이터베이스 또는 서비스를 원활하게 통합할 수 있는 확장 가능한 시스템을 설계할 것입니다.
비전: ORM처럼 디커플링
목표는 핵심 로직을 변경하지 않고 캐시를 확장 가능하게 만드는 것입니다. ORM 시스템에서 영감을 받은 우리의 접근 방식에는 공유 API 추상화가 포함됩니다. 이를 통해 localStorage, IndexedDB 또는 원격 데이터베이스와 같은 저장소가 최소한의 코드 변경으로 상호 교환적으로 작동할 수 있습니다.
저장소 어댑터 기본 클래스
지속성 시스템에 대한 API를 정의하는 추상 클래스는 다음과 같습니다.
export abstract class StorageAdapter { abstract connect(): Promise<void>; abstract add(key: string, value: unknown): Promise<void>; abstract get(key: string): Promise<unknown null>; abstract getAll(): Promise<record unknown>>; abstract delete(key: string): Promise<void>; abstract clear(): Promise<void>; } </void></void></record></unknown></void></void>
모든 스토리지 솔루션은 이 기본 클래스를 확장하여 상호 작용의 일관성을 보장해야 합니다. 예를 들어, IndexedDB 구현은 다음과 같습니다.
예: IndexedDB 어댑터
이 어댑터는 StorageAdapter 인터페이스를 구현하여 IndexedDB 저장소에 캐시 데이터를 유지합니다.
import { StorageAdapter } from './storage_adapter'; /** * IndexedDBAdapter is an implementation of the StorageAdapter * interface designed to provide a persistent storage mechanism * using IndexedDB. This adapter can be reused for other cache * implementations or extended for similar use cases, ensuring * flexibility and scalability. */ export class IndexedDBAdapter extends StorageAdapter { private readonly dbName: string; private readonly storeName: string; private db: IDBDatabase | null = null; /** * Initializes the adapter with the specified database and store * names. Defaults are provided to make it easy to set up without * additional configuration. */ constructor(dbName: string = 'cacheDB', storeName: string = 'cacheStore') { super(); this.dbName = dbName; this.storeName = storeName; } /** * Connects to the IndexedDB database and initializes it if * necessary. This asynchronous method ensures that the database * and object store are available before any other operations. * It uses the `onupgradeneeded` event to handle schema creation * or updates, making it a robust solution for versioning. */ async connect(): Promise<void> { return await new Promise((resolve, reject) => { const request = indexedDB.open(this.dbName, 1); request.onupgradeneeded = (event) => { const db = (event.target as IDBOpenDBRequest).result; if (!db.objectStoreNames.contains(this.storeName)) { db.createObjectStore(this.storeName, { keyPath: 'key' }); } }; request.onsuccess = (event) => { this.db = (event.target as IDBOpenDBRequest).result; resolve(); }; request.onerror = () => reject(request.error); }); } /** * Adds or updates a key-value pair in the store. This method is * asynchronous to ensure compatibility with the non-blocking * nature of IndexedDB and to prevent UI thread blocking. Using * the `put` method ensures idempotency: the operation will * insert or replace the entry. */ async add(key: string, value: unknown): Promise<void> { await this._withTransaction('readwrite', (store) => store.put({ key, value })); } /** * Retrieves the value associated with a key. If the key does not * exist, null is returned. This method is designed to integrate * seamlessly with caching mechanisms, enabling fast lookups. */ async get(key: string): Promise<unknown null> { return await this._withTransaction('readonly', (store) => this._promisifyRequest(store.get(key)).then((result) => result ? (result as { key: string; value: unknown }).value : null ) ); } /** * Fetches all key-value pairs from the store. Returns an object * mapping keys to their values, making it suitable for bulk * operations or syncing with in-memory caches. */ async getAll(): Promise<record unknown>> { return await this._withTransaction('readonly', (store) => this._promisifyRequest(store.getAll()).then((results) => results.reduce((acc: Record<string unknown>, item: { key: string; value: unknown }) => { acc[item.key] = item.value; return acc; }, {}) ) ); } /** * Deletes a key-value pair by its key. This method is crucial * for managing cache size and removing expired entries. The * `readwrite` mode is used to ensure proper deletion. */ async delete(key: string): Promise<void> { await this._withTransaction('readwrite', (store) => store.delete(key)); } /** * Clears all entries from the store. This method is ideal for * scenarios where the entire cache needs to be invalidated, such * as during application updates or environment resets. */ async clear(): Promise<void> { await this._withTransaction('readwrite', (store) => store.clear()); } /** * Handles transactions in a reusable way. Ensures the database * is connected and abstracts the transaction logic. By * centralizing transaction handling, this method reduces * boilerplate code and ensures consistency across all operations. */ private async _withTransaction<t>( mode: IDBTransactionMode, callback: (store: IDBObjectStore) => IDBRequest | Promise<t> ): Promise<t> { if (!this.db) throw new Error('IndexedDB is not connected'); const transaction = this.db.transaction([this.storeName], mode); const store = transaction.objectStore(this.storeName); const result = callback(store); return result instanceof IDBRequest ? await this._promisifyRequest(result) : await result; } /** * Converts IndexedDB request events into Promises, allowing for * cleaner and more modern asynchronous handling. This is * essential for making IndexedDB operations fit seamlessly into * the Promise-based architecture of JavaScript applications. */ private async _promisifyRequest<t>(request: IDBRequest): Promise<t> { return await new Promise((resolve, reject) => { request.onsuccess = () => resolve(request.result as T); request.onerror = () => reject(request.error); }); } } </t></t></t></t></t></void></void></string></record></unknown></void></void>
캐시에 어댑터 통합
캐시는 선택적 StorageAdapter를 허용합니다. 제공된 경우 데이터베이스 연결을 초기화하고 데이터를 메모리에 로드하며 캐시와 스토리지를 동기화된 상태로 유지합니다.
private constructor(capacity: number, storageAdapter?: StorageAdapter) { this.capacity = capacity; this.storageAdapter = storageAdapter; if (this.storageAdapter) { this.storageAdapter.connect().catch((error) => { throw new Error(error); }); this.storageAdapter.getAll().then((data) => { for (const key in data) { this.put(key, data[key] as T); } }).catch((error) => { throw new Error(error); }); } this.hash = new Map(); this.head = this.tail = undefined; this.hitCount = this.missCount = this.evictionCount = 0; }
왜 어댑터와 전략 패턴인가?
어댑터 패턴 사용:
- 특정 저장 메커니즘에서 캐시를 분리합니다.
- 새로운 스토리지 백엔드에 대한 확장성을 보장합니다.
전략 패턴과 결합:
- 지속성 레이어의 런타임 선택을 활성화합니다.
- 다양한 어댑터를 모의하여 테스트를 단순화합니다.
주요 설계 사례
- 추상 API: 스토리지 세부정보에 관계없이 캐시 로직을 유지합니다.
- 싱글턴 캐시: 공유 상태 일관성을 보장합니다.
- 비동기 초기화: 설정 중 작업 차단을 방지합니다.
- 지연 로딩: 스토리지 어댑터가 제공되는 경우에만 지속형 데이터를 로드합니다.
다음 단계
이 디자인은 견고하지만 개선의 여지가 있습니다.
- 더 나은 성능을 위해 동기화 로직을 최적화하세요.
- Redis 또는 SQLite와 같은 추가 어댑터를 사용해 실험해 보세요.
사용해 보세요! ?
캐시가 실제로 작동하는지 테스트하고 싶다면 npm 패키지(adev-lru)로 사용할 수 있습니다. GitHub: adev-lru 저장소에서 전체 소스 코드를 탐색할 수도 있습니다. 더 나은 앱을 만들기 위한 추천, 건설적인 피드백 또는 기여를 환영합니다! ?
즐거운 코딩하세요! ?
위 내용은 구성 가능한 데이터 지속성을 통해 LRU 캐시 향상의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
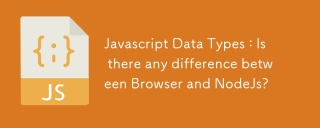
JavaScript 코어 데이터 유형은 브라우저 및 Node.js에서 일관되지만 추가 유형과 다르게 처리됩니다. 1) 글로벌 객체는 브라우저의 창이고 node.js의 글로벌입니다. 2) 이진 데이터를 처리하는 데 사용되는 Node.js의 고유 버퍼 객체. 3) 성능 및 시간 처리에는 차이가 있으며 환경에 따라 코드를 조정해야합니다.
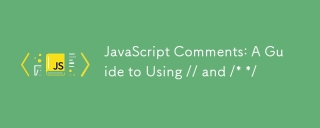
javaScriptUSTWOTYPESOFSOFCOMMENTS : 단일 라인 (//) 및 multi-line (//)
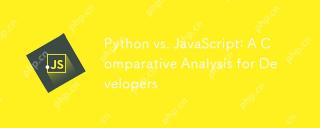
Python과 JavaScript의 주요 차이점은 유형 시스템 및 응용 프로그램 시나리오입니다. 1. Python은 과학 컴퓨팅 및 데이터 분석에 적합한 동적 유형을 사용합니다. 2. JavaScript는 약한 유형을 채택하며 프론트 엔드 및 풀 스택 개발에 널리 사용됩니다. 두 사람은 비동기 프로그래밍 및 성능 최적화에서 고유 한 장점을 가지고 있으며 선택할 때 프로젝트 요구 사항에 따라 결정해야합니다.
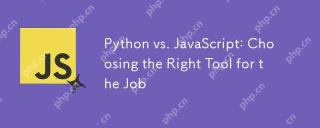
Python 또는 JavaScript를 선택할지 여부는 프로젝트 유형에 따라 다릅니다. 1) 데이터 과학 및 자동화 작업을 위해 Python을 선택하십시오. 2) 프론트 엔드 및 풀 스택 개발을 위해 JavaScript를 선택하십시오. Python은 데이터 처리 및 자동화 분야에서 강력한 라이브러리에 선호되는 반면 JavaScript는 웹 상호 작용 및 전체 스택 개발의 장점에 없어서는 안될 필수입니다.
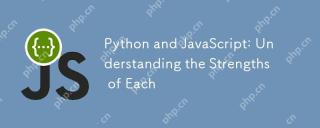
파이썬과 자바 스크립트는 각각 고유 한 장점이 있으며 선택은 프로젝트 요구와 개인 선호도에 따라 다릅니다. 1. Python은 간결한 구문으로 데이터 과학 및 백엔드 개발에 적합하지만 실행 속도가 느립니다. 2. JavaScript는 프론트 엔드 개발의 모든 곳에 있으며 강력한 비동기 프로그래밍 기능을 가지고 있습니다. node.js는 풀 스택 개발에 적합하지만 구문은 복잡하고 오류가 발생할 수 있습니다.
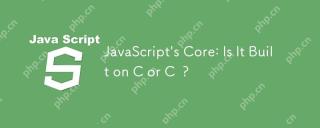
javaScriptisNotBuiltoncorc; it'SangretedLanguageThatrunsonOngineStenWrittenInc .1) javaScriptWasDesignEdasAlightweight, 해석 hanguageforwebbrowsers.2) Endinesevolvedfromsimpleplemporectreterstoccilpilers, 전기적으로 개선된다.
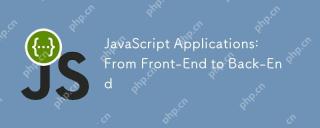
JavaScript는 프론트 엔드 및 백엔드 개발에 사용할 수 있습니다. 프론트 엔드는 DOM 작업을 통해 사용자 경험을 향상시키고 백엔드는 Node.js를 통해 서버 작업을 처리합니다. 1. 프론트 엔드 예 : 웹 페이지 텍스트의 내용을 변경하십시오. 2. 백엔드 예제 : node.js 서버를 만듭니다.
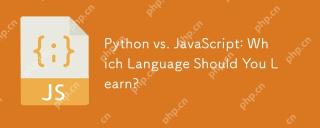
Python 또는 JavaScript는 경력 개발, 학습 곡선 및 생태계를 기반으로해야합니다. 1) 경력 개발 : Python은 데이터 과학 및 백엔드 개발에 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 적합합니다. 2) 학습 곡선 : Python 구문은 간결하며 초보자에게 적합합니다. JavaScript Syntax는 유연합니다. 3) 생태계 : Python에는 풍부한 과학 컴퓨팅 라이브러리가 있으며 JavaScript는 강력한 프론트 엔드 프레임 워크를 가지고 있습니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.
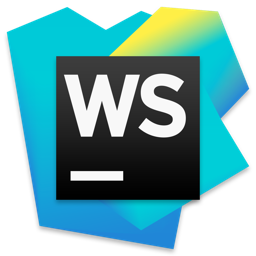
WebStorm Mac 버전
유용한 JavaScript 개발 도구

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경
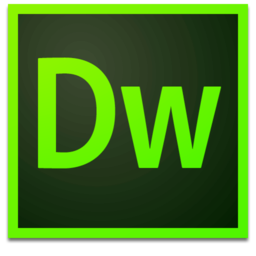
Dreamweaver Mac版
시각적 웹 개발 도구