React의 구성요소 및 Prop: 사용자 인터페이스의 구성 요소
React에서 Components와 Props는 개발자가 재사용 가능하고 동적인 사용자 인터페이스를 만들 수 있는 기본 개념입니다. UI를 더 작고 관리 가능한 부분으로 나누고 이러한 부분 간에 데이터를 전달하여 애플리케이션 개발을 단순화합니다.
1. 컴포넌트란 무엇인가요?
React의 컴포넌트는 UI의 일부를 정의하는 재사용 가능하고 독립적인 코드 블록입니다. 구성요소를 애플리케이션 구축을 위한 구성 요소로 생각하세요.
구성품 종류
아. 기능성 구성요소
- 가장 간단한 유형의 React 컴포넌트입니다.
- props를 받아들이고 JSX를 반환하는 JavaScript 함수로 정의됩니다.
예:
const Greeting = (props) => { return <h1 id="Hello-props-name">Hello, {props.name}!</h1>; };
ㄴ. 클래스 구성요소
- ES6 클래스를 사용하여 정의됩니다.
- 상태 및 수명 주기 메서드와 같은 추가 기능을 포함합니다(React Hooks 이전).
- 일반적으로 이전 React 프로젝트에서 사용됩니다.
예:
class Greeting extends React.Component { render() { return <h1 id="Hello-this-props-name">Hello, {this.props.name}!</h1>; } }
컴포넌트를 사용하는 이유
- 재사용성: 한 번 작성하면 동일한 구성 요소를 여러 위치에서 사용할 수 있습니다.
- 유지관리성: UI의 작고 집중된 부분을 관리하고 디버깅합니다.
- 가독성: 복잡한 UI를 더 간단하고 이해하기 쉬운 부분으로 분해하세요.
2. 소품이란 무엇인가요?
Props(properties의 약자)는 상위 구성 요소에서 하위 구성 요소로 데이터를 전달하는 메커니즘입니다. 소품은 읽기 전용이므로 하위 구성 요소에서 수정할 수 없습니다.
소품 작동 방식
- 구성요소에 인수로 전달됩니다.
- 기능적 구성 요소의 props 개체 또는 클래스 구성 요소의 this.props를 통해 액세스할 수 있습니다.
예:
const UserCard = (props) => { return ( <div> <h2 id="props-name">{props.name}</h2> <p>{props.email}</p> </div> ); }; // Usage <usercard name="John Doe" email="john.doe@example.com"></usercard>
3. 소품의 주요 특징
- 단방향 흐름: Prop은 단방향 데이터 흐름으로 상위에서 하위로 흐릅니다.
- 불변: Prop은 수신 컴포넌트에 의해 변경될 수 없습니다.
- 동적: 상위 구성 요소는 동적 값이나 변수를 하위 구성 요소에 전달할 수 있습니다.
동적 소품의 예:
const Greeting = (props) => { return <h1 id="Hello-props-name">Hello, {props.name}!</h1>; };
4. 컴포넌트와 소품 결합
React 애플리케이션은 일반적으로 props를 사용하여 통신하는 여러 구성 요소로 구성됩니다. 이 조합을 통해 계층적이고 동적인 구조를 구축할 수 있습니다.
예: 소품이 포함된 중첩 구성 요소
class Greeting extends React.Component { render() { return <h1 id="Hello-this-props-name">Hello, {this.props.name}!</h1>; } }
5. 기본 소품 및 소품 유형
아. 기본 소품
defaultProps 속성을 사용하여 props의 기본값을 설정할 수 있습니다.
예:
const UserCard = (props) => { return ( <div> <h2 id="props-name">{props.name}</h2> <p>{props.email}</p> </div> ); }; // Usage <usercard name="John Doe" email="john.doe@example.com"></usercard>
ㄴ. 소품 종류
prop-types 라이브러리를 사용하여 구성 요소에 전달된 prop 유형을 확인하세요.
예:
const App = () => { const user = { name: "Alice", email: "alice@example.com" }; return <usercard name="{user.name}" email="{user.email}"></usercard>; };
6. Props와 State의 차이점
Aspect | Props | State |
---|---|---|
Definition | Passed from parent to child. | Local to the component. |
Mutability | Immutable (read-only). | Mutable (can be updated). |
Purpose | Share data between components. | Manage internal component data. |
소품
7. 소품을 사용하는 경우
하위 구성요소에 데이터를 전달합니다.
- 동적 콘텐츠(예: 사용자 프로필, 제품 세부정보)를 렌더링합니다.
재사용 가능하고 사용자 정의 가능한 UI 구성요소(예: 버튼, 카드)를 구축하세요.
- 8. 모범 사례
-
구성요소를 작고 집중적으로 유지
- 각 구성 요소는 단일 목적으로 사용되어야 합니다.
-
기본 Prop 및 Prop 유형 사용
- props에 합리적인 기본값이 있는지 확인하고 유형을 검증하세요.
-
소품 남용 방지
- 하위 구성 요소에 광범위한 데이터가 필요한 경우 공유 컨텍스트에서 관리하거나 상태 관리 라이브러리를 사용하는 것이 좋습니다.
명명 규칙을 따르세요
코드 가독성을 유지하려면 소품에 설명이 포함된 이름을 사용하세요.
위 내용은 React의 구성 요소 및 속성 이해: 재사용 가능한 UI의 기초의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
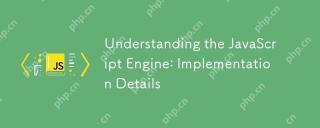
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.

Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
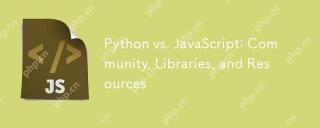
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
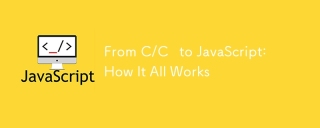
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
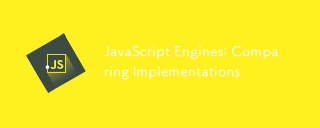
각각의 엔진의 구현 원리 및 최적화 전략이 다르기 때문에 JavaScript 엔진은 JavaScript 코드를 구문 분석하고 실행할 때 다른 영향을 미칩니다. 1. 어휘 분석 : 소스 코드를 어휘 단위로 변환합니다. 2. 문법 분석 : 추상 구문 트리를 생성합니다. 3. 최적화 및 컴파일 : JIT 컴파일러를 통해 기계 코드를 생성합니다. 4. 실행 : 기계 코드를 실행하십시오. V8 엔진은 즉각적인 컴파일 및 숨겨진 클래스를 통해 최적화하여 Spidermonkey는 유형 추론 시스템을 사용하여 동일한 코드에서 성능이 다른 성능을 제공합니다.
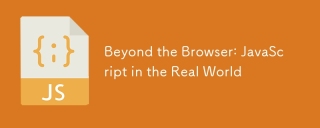
실제 세계에서 JavaScript의 응용 프로그램에는 서버 측 프로그래밍, 모바일 애플리케이션 개발 및 사물 인터넷 제어가 포함됩니다. 1. 서버 측 프로그래밍은 Node.js를 통해 실현되며 동시 요청 처리에 적합합니다. 2. 모바일 애플리케이션 개발은 재교육을 통해 수행되며 크로스 플랫폼 배포를 지원합니다. 3. Johnny-Five 라이브러리를 통한 IoT 장치 제어에 사용되며 하드웨어 상호 작용에 적합합니다.
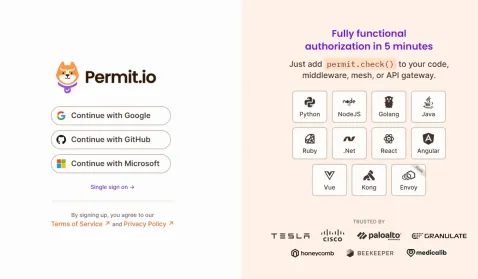
일상적인 기술 도구를 사용하여 기능적 다중 테넌트 SaaS 응용 프로그램 (Edtech 앱)을 구축했으며 동일한 작업을 수행 할 수 있습니다. 먼저, 다중 테넌트 SaaS 응용 프로그램은 무엇입니까? 멀티 테넌트 SAAS 응용 프로그램은 노래에서 여러 고객에게 서비스를 제공 할 수 있습니다.
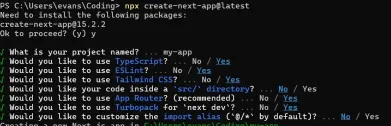
이 기사에서는 Contrim에 의해 확보 된 백엔드와의 프론트 엔드 통합을 보여 주며 Next.js를 사용하여 기능적인 Edtech SaaS 응용 프로그램을 구축합니다. Frontend는 UI 가시성을 제어하기 위해 사용자 권한을 가져오고 API가 역할 기반을 준수하도록합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
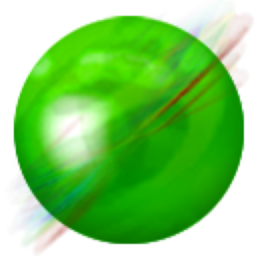
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경
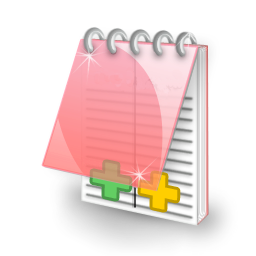
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.
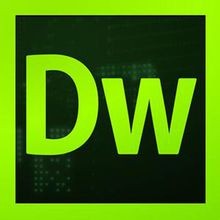
드림위버 CS6
시각적 웹 개발 도구
