React의 useRef Hook
useRef 후크는 다시 렌더링하지 않고 렌더링 전반에 걸쳐 값을 유지하는 데 사용되는 내장 React 후크입니다. DOM 요소와 직접 상호작용하고 렌더링 간에 유지되어야 하지만 반드시 다시 렌더링을 트리거할 필요는 없는 값을 저장하는 데 자주 사용됩니다.
useRef란 무엇인가요?
useRef 후크는 주로 두 가지 목적으로 사용됩니다.
- DOM 요소 액세스: DOM 노드 또는 React 요소를 직접 참조하는 방법을 제공하여 명령적으로 상호 작용할 수 있습니다.
- 렌더링 전반에 걸쳐 값 유지: 상태와 달리 업데이트 시 다시 렌더링을 트리거하지 않는 변경 가능한 값을 저장할 수 있습니다.
useRef 구문
const myRef = useRef(initialValue);
- myRef: useRef에 의해 생성된 참조 객체
- initialValue: 참조 객체에 저장될 초기 값입니다. 이는 DOM 노드, 객체 또는 기본 값 등 무엇이든 될 수 있습니다.
useRef가 반환한 참조 객체에는 실제 값이 저장되는 현재 속성이 있습니다.
useRef 작동 방식
- DOM 요소 액세스: 클래스 구성 요소의 ref 속성과 마찬가지로 useRef를 사용하면 기능 구성 요소의 DOM 요소에 직접 액세스할 수 있습니다.
const MyComponent = () => { const inputRef = useRef(null); const focusInput = () => { // Access the DOM node and focus it inputRef.current.focus(); }; return ( <div> <input ref="{inputRef}"> <button onclick="{focusInput}">Focus Input</button> </div> ); };
- 여기서 inputRef는 버튼을 클릭하면 focusInput 함수는 해당 입력 요소에 초점을 맞춥니다.
- 렌더링 전반에 걸쳐 변경 가능한 값 저장: useRef를 사용하면 렌더링 전반에 걸쳐 지속되지만 변경 시 다시 렌더링을 트리거하지 않는 값을 저장할 수 있습니다.
const TimerComponent = () => { const countRef = useRef(0); const increment = () => { countRef.current++; console.log(countRef.current); // This will log the updated value }; return ( <div> <p>Current count (does not trigger re-render): {countRef.current}</p> <button onclick="{increment}">Increment</button> </div> ); };
- 이 예에서 countRef는 변경 가능한 값을 저장합니다. 재렌더링을 트리거하는 useState와 달리 재렌더링을 발생시키지 않고 값을 업데이트할 수 있습니다.
일반적인 사용 사례참고
- DOM 요소 액세스: 예를 들어 입력 필드에 초점을 맞추거나 특정 요소로 스크롤하거나 요소의 크기를 측정합니다.
const ScrollToTop = () => { const topRef = useRef(null); const scrollToTop = () => { topRef.current.scrollIntoView({ behavior: 'smooth' }); }; return ( <div> <div ref="{topRef}"> <ol> <li> <strong>Storing Previous State</strong>: If you need to track the previous value of a prop or state value. </li> </ol> <pre class="brush:php;toolbar:false"> const PreviousState = ({ count }) => { const prevCountRef = useRef(); useEffect(() => { prevCountRef.current = count; // Store the current value in the ref }, [count]); return ( <div> <p>Current Count: {count}</p> <p>Previous Count: {prevCountRef.current}</p> </div> ); };
- 설명: prevCountRef는 다시 렌더링을 트리거하지 않고도 액세스할 수 있는 count의 이전 값을 저장합니다.
복잡한 값에 대한 재렌더링 방지: 재렌더링을 트리거할 필요가 없는 큰 개체나 복잡한 데이터 구조가 있는 경우 useRef는 구성 요소의 값에 영향을 주지 않고 이를 저장할 수 있습니다. 공연.
추적 간격 또는 시간 초과: 시간 초과 또는 간격의 ID를 저장하여 나중에 지울 수 있습니다.
const myRef = useRef(initialValue);
- 설명: IntervalRef는 간격의 ID를 저장하며 구성 요소가 마운트 해제될 때 간격을 지우는 데 사용할 수 있습니다.
useRef와 useState의 주요 차이점
Feature | useRef | useState |
---|---|---|
Triggers re-render | No (does not trigger a re-render) | Yes (triggers a re-render when state changes) |
Use Case | Storing mutable values or DOM references | Storing state that affects rendering |
Value storage | Stored in current property | Stored in state variable |
Persistence across renders | Yes (keeps value across renders without triggering re-render) | Yes (but triggers re-render when updated) |
예: 양식 유효성 검사에 useRef 사용
다음은 유효하지 않은 입력 필드에 초점을 맞춰 양식 유효성 검사에 useRef를 사용하는 예입니다.
'react'에서 React, { useRef, useState }를 가져옵니다. const FormComponent = () => { const inputRef = useRef(); const [inputValue, setInputValue] = useState(''); const [error, setError] = useState(''); const verifyInput = () => { if (inputValue === '') { setError('입력값은 비워둘 수 없습니다.'); inputRef.current.focus(); // 입력 요소에 초점을 맞춥니다. } 또 다른 { setError(''); } }; 반품 ( <div> setInputValue(e.target.value)} /> {오류 && <p> </p> <ul> <li> <strong>설명</strong>: <ul> <li>inputRef는 입력 값이 비어 있는 경우 입력 요소에 초점을 맞추는 데 사용됩니다.</li> <li>이 기능은 useState에서는 불가능합니다. DOM 요소에 초점을 맞추려면 useState가 제공할 수 없는 요소에 직접 액세스해야 하기 때문입니다.</li> </ul> </li> </ul> <hr> <h3> <strong>사용요약Ref Hook</strong> </h3> <ul> <li> <strong>useRef</strong>는 업데이트 시 다시 렌더링을 트리거하지 않는 DOM 요소 및 변경 가능한 값에 대한 참조를 저장하는 데 사용됩니다.</li> <li>DOM 노드에 직접 액세스하는 데 유용합니다(예: 초점 맞추기, 스크롤 또는 애니메이션).</li> <li> <strong>useRef</strong>는 이전 값을 추적하거나 시간 초과/간격 ID를 저장하는 등 다시 렌더링을 트리거할 필요 없이 렌더링 전반에 걸쳐 지속되는 값을 저장하는 데에도 유용합니다.</li> <li> <strong>주요 차이점</strong>: useState와 달리 useRef를 업데이트해도 다시 렌더링이 실행되지 않습니다.</li> </ul> <hr> <h3> <strong>결론</strong> </h3> <p><strong>useRef</strong> 후크는 React에서 변경 가능한 값과 직접적인 DOM 조작을 처리하는 데 매우 유용합니다. 양식 요소로 작업하든, 이전 상태를 추적하든, 타사 라이브러리와 상호작용하든, useRef는 깔끔하고 효율적인 솔루션을 제공합니다. useRef를 사용하면 불필요한 재렌더링을 트리거하지 않고 지속성을 유지할 수 있으므로 성능에 민감한 작업에 탁월한 선택입니다.</p> <hr> </div>
위 내용은 Reacts useRef Hook 익히기: DOM 및 가변 값 작업의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
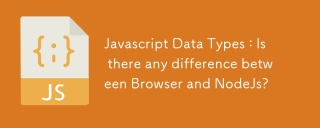
JavaScript 코어 데이터 유형은 브라우저 및 Node.js에서 일관되지만 추가 유형과 다르게 처리됩니다. 1) 글로벌 객체는 브라우저의 창이고 node.js의 글로벌입니다. 2) 이진 데이터를 처리하는 데 사용되는 Node.js의 고유 버퍼 객체. 3) 성능 및 시간 처리에는 차이가 있으며 환경에 따라 코드를 조정해야합니다.
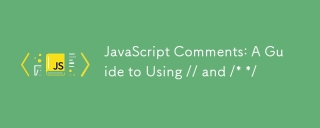
javaScriptUSTWOTYPESOFSOFCOMMENTS : 단일 라인 (//) 및 multi-line (//)
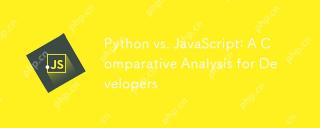
Python과 JavaScript의 주요 차이점은 유형 시스템 및 응용 프로그램 시나리오입니다. 1. Python은 과학 컴퓨팅 및 데이터 분석에 적합한 동적 유형을 사용합니다. 2. JavaScript는 약한 유형을 채택하며 프론트 엔드 및 풀 스택 개발에 널리 사용됩니다. 두 사람은 비동기 프로그래밍 및 성능 최적화에서 고유 한 장점을 가지고 있으며 선택할 때 프로젝트 요구 사항에 따라 결정해야합니다.
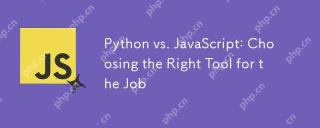
Python 또는 JavaScript를 선택할지 여부는 프로젝트 유형에 따라 다릅니다. 1) 데이터 과학 및 자동화 작업을 위해 Python을 선택하십시오. 2) 프론트 엔드 및 풀 스택 개발을 위해 JavaScript를 선택하십시오. Python은 데이터 처리 및 자동화 분야에서 강력한 라이브러리에 선호되는 반면 JavaScript는 웹 상호 작용 및 전체 스택 개발의 장점에 없어서는 안될 필수입니다.
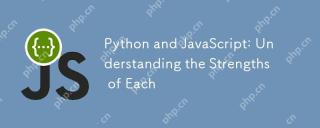
파이썬과 자바 스크립트는 각각 고유 한 장점이 있으며 선택은 프로젝트 요구와 개인 선호도에 따라 다릅니다. 1. Python은 간결한 구문으로 데이터 과학 및 백엔드 개발에 적합하지만 실행 속도가 느립니다. 2. JavaScript는 프론트 엔드 개발의 모든 곳에 있으며 강력한 비동기 프로그래밍 기능을 가지고 있습니다. node.js는 풀 스택 개발에 적합하지만 구문은 복잡하고 오류가 발생할 수 있습니다.
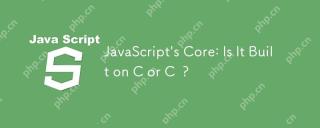
javaScriptisNotBuiltoncorc; it'SangretedLanguageThatrunsonOngineStenWrittenInc .1) javaScriptWasDesignEdasAlightweight, 해석 hanguageforwebbrowsers.2) Endinesevolvedfromsimpleplemporectreterstoccilpilers, 전기적으로 개선된다.
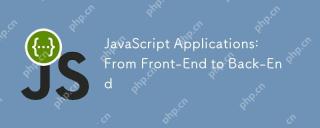
JavaScript는 프론트 엔드 및 백엔드 개발에 사용할 수 있습니다. 프론트 엔드는 DOM 작업을 통해 사용자 경험을 향상시키고 백엔드는 Node.js를 통해 서버 작업을 처리합니다. 1. 프론트 엔드 예 : 웹 페이지 텍스트의 내용을 변경하십시오. 2. 백엔드 예제 : node.js 서버를 만듭니다.
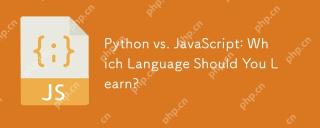
Python 또는 JavaScript는 경력 개발, 학습 곡선 및 생태계를 기반으로해야합니다. 1) 경력 개발 : Python은 데이터 과학 및 백엔드 개발에 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 적합합니다. 2) 학습 곡선 : Python 구문은 간결하며 초보자에게 적합합니다. JavaScript Syntax는 유연합니다. 3) 생태계 : Python에는 풍부한 과학 컴퓨팅 라이브러리가 있으며 JavaScript는 강력한 프론트 엔드 프레임 워크를 가지고 있습니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.

DVWA
DVWA(Damn Vulnerable Web App)는 매우 취약한 PHP/MySQL 웹 애플리케이션입니다. 주요 목표는 보안 전문가가 법적 환경에서 자신의 기술과 도구를 테스트하고, 웹 개발자가 웹 응용 프로그램 보안 프로세스를 더 잘 이해할 수 있도록 돕고, 교사/학생이 교실 환경 웹 응용 프로그램에서 가르치고 배울 수 있도록 돕는 것입니다. 보안. DVWA의 목표는 다양한 난이도의 간단하고 간단한 인터페이스를 통해 가장 일반적인 웹 취약점 중 일부를 연습하는 것입니다. 이 소프트웨어는
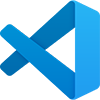
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.
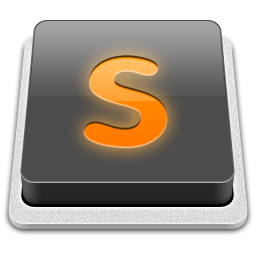
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)