이 기사에서는 JavaScript와 Python의 구문과 기본 프로그래밍 구조를 비교합니다. 이 두 가지 인기 프로그래밍 언어에서 기본 프로그래밍 개념이 구현되는 방식의 유사점을 강조하는 것을 목표로 합니다.
두 언어 모두 많은 공통점을 공유하므로 개발자가 두 언어 간에 전환하거나 다른 언어의 코드를 더 쉽게 이해할 수 있지만, 알아야 할 뚜렷한 구문 및 운영상의 차이점도 있습니다.
JavaScript와 Python의 유사점이나 차이점을 지나치게 강조하지 말고 가벼운 마음으로 비교에 접근하는 것이 중요합니다. 한 언어가 다른 언어보다 우수하다고 선언하려는 것이 아니라 Python에 익숙한 코더가 JavaScript를 더 쉽게 이해하고 전환하는 데 도움이 될 수 있는 리소스를 제공하는 것이 목적입니다.
안녕하세요 세계
자바스크립트
// In codeguppy.com environment println('Hello, World'); // Outside codeguppy.com console.log('Hello, World');
파이썬
print('Hello, World')
변수와 상수
자바스크립트
let myVariable = 100; const MYCONSTANT = 3.14159;
파이썬
myVariable = 100 MYCONSTANT = 3.14159
문자열 보간
자바스크립트
let a = 100; let b = 200; println(`Sum of ${a} and ${b} is ${a + b}`);
파이썬
a = 100 b = 200 print(f'Sum of {a} and {b} is {a + b}')
If 표현식/문
자바스크립트
let age = 18; if (age <p><strong>파이썬</strong><br> </p> <pre class="brush:php;toolbar:false">age = 18 if age <h2> 조건부 </h2> <p><strong>자바스크립트</strong><br> </p> <pre class="brush:php;toolbar:false">let age = 20; let message = age >= 18 ? "Can vote" : "Cannot vote"; println(message); // Output: Can vote
파이썬
age = 20 message = "Can vote" if age >= 18 else "Cannot vote" print(message) # Output: Can vote
배열
자바스크립트
// Creating an array let myArray = [1, 2, 3, 4, 5]; // Accessing elements println(myArray[0]); // Access the first element: 1 println(myArray[3]); // Access the fourth element: 4 // Modifying an element myArray[2] = 30; // Change the third element from 3 to 30 // Adding a new element myArray.push(6); // Add a new element to the end
파이썬
# Creating a list to represent an array my_array = [1, 2, 3, 4, 5] # Accessing elements print(my_array[0]) # Access the first element: 1 print(my_array[3]) # Access the fourth element: 4 # Modifying an element my_array[2] = 30 # Change the third element from 3 to 30 # Adding a new element my_array.append(6) # Add a new element to the end
각각에 대해
자바스크립트
let fruits = ["apple", "banana", "cherry", "date"]; for(let fruit of fruits) println(fruit);
파이썬
fruits = ["apple", "banana", "cherry", "date"] for fruit in fruits: print(fruit)
사전
자바스크립트
// Creating a dictionary fruit_prices = { apple: 0.65, banana: 0.35, cherry: 0.85 }; // Accessing a value by key println(fruit_prices["apple"]); // Output: 0.65
파이썬
# Creating a dictionary fruit_prices = { "apple": 0.65, "banana": 0.35, "cherry": 0.85 } # Accessing a value by key print(fruit_prices["apple"]) # Output: 0.65
기능
자바스크립트
function addNumbers(a, b) { return a + b; } let result = addNumbers(100, 200); println("The sum is: ", result);
파이썬
def add_numbers(a, b): return a + b result = add_numbers(100, 200) print("The sum is: ", result)
튜플 반환
자바스크립트
function getCircleProperties(radius) { const area = Math.PI * radius ** 2; const circumference = 2 * Math.PI * radius; return [area, circumference]; // Return as an array } // Using the function const [area, circumference] = getCircleProperties(5); println(`The area of the circle is: ${area}`); println(`The circumference of the circle is: ${circumference}`);
파이썬
import math def getCircleProperties(radius): """Calculate and return the area and circumference of a circle.""" area = math.pi * radius**2 circumference = 2 * math.pi * radius return (area, circumference) # Using the function radius = 5 area, circumference = getCircleProperties(radius) print(f"The area of the circle is: {area}") print(f"The circumference of the circle is: {circumference}")
가변 개수의 인수
자바스크립트
function sumNumbers(...args) { let sum = 0; for(let i of args) sum += i; return sum; } println(sumNumbers(1, 2, 3)); println(sumNumbers(100, 200));
파이썬
def sum_numbers(*args): sum = 0 for i in args: sum += i return sum print(sum_numbers(1, 2, 3)) print(sum_numbers(100, 200))
람다
자바스크립트
const numbers = [1, 2, 3, 4, 5]; // Use map to apply a function to all elements of the array const squaredNumbers = numbers.map(x => x ** 2); println(squaredNumbers); // Output: [1, 4, 9, 16, 25]
파이썬
numbers = [1, 2, 3, 4, 5] # Use map to apply a function to all elements of the list squared_numbers = map(lambda x: x**2, numbers) # Convert map object to a list to print the results squared_numbers_list = list(squared_numbers) print(squared_numbers_list) # Output: [1, 4, 9, 16, 25]
수업
자바스크립트
class Book { constructor(title, author, pages) { this.title = title; this.author = author; this.pages = pages; } describeBook() { println(`Book Title: ${this.title}`); println(`Author: ${this.author}`); println(`Number of Pages: ${this.pages}`); } }
파이썬
class Book: def __init__(self, title, author, pages): self.title = title self.author = author self.pages = pages def describe_book(self): print(f"Book Title: {self.title}") print(f"Author: {self.author}") print(f"Number of Pages: {self.pages}")
수업의 활용
자바스크립트
// In codeguppy.com environment println('Hello, World'); // Outside codeguppy.com console.log('Hello, World');
파이썬
print('Hello, World')
결론
이 비교를 개선하는 데 참여해 보시기 바랍니다. 수정, 개선, 새로운 추가 등 여러분의 기여는 매우 소중합니다. 협력을 통해 JavaScript 및 Python 학습에 관심이 있는 모든 개발자에게 도움이 되는 보다 정확하고 포괄적인 가이드를 만들 수 있습니다.
크레딧
이 글은 프리코딩 플랫폼 https://codeguppy.com 플랫폼의 블로그에서 다시 게재되었습니다.
이 기사는 다른 프로그래밍 언어 간의 유사한 비교에 영향을 받았습니다.
- Kotlin은 C#과 같습니다 https://ttu.github.io/kotlin-is-like-csharp/
- Kotlin은 TypeScript와 같습니다 https://gi-no.github.io/kotlin-is-like-typescript/
- Swift는 Kotlin과 같습니다 https://nilhcem.com/swift-is-like-kotlin/
- Swift는 Go와 같습니다 http://repo.tiye.me/jiyinyiyong/swift-is-like-go/
- Swift는 Scala와 같습니다 https://leverich.github.io/swiftislikescala/
위 내용은 자바스크립트는 파이썬과 비슷하다의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
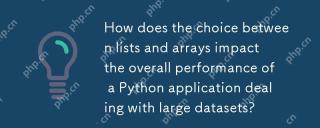
forhandlinglargedatasetsinpython, usenumpyarraysforbetterperformance.1) numpyarraysarememory-effic andfasterfornumericaloperations.2) leveragevectorization foredtimecomplexity.4) managemoryusage withorfications data
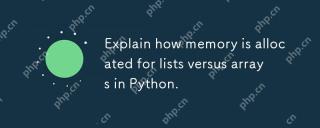
inpython, listsusedyammoryAllocation과 함께 할당하고, whilempyarraysallocatefixedMemory.1) listsAllocatemememorythanneedInitiality.
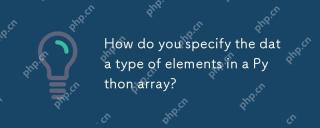
Inpython, youcansspecthedatatypeyfelemeremodelerernspant.1) usenpynernrump.1) usenpynerp.dloatp.ploatm64, 포모 선례 전분자.
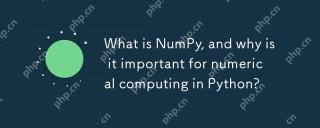
numpyissentialfornumericalcomputinginpythonduetoitsspeed, memory-efficiency 및 comperniveMathematicaticaltions
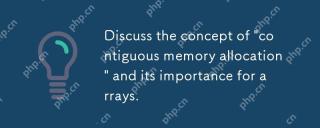
contiguousUousUousUlorAllocationScrucialForraysbecauseItAllowsOfficationAndFastElementAccess.1) ItenableSconstantTimeAccess, o (1), DuetodirectAddressCalculation.2) Itimprovesceeffiency theMultipleementFetchespercacheline.3) Itsimplififiesmomorym
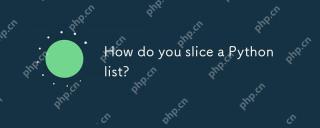
slicepaythonlistisdoneusingthesyntaxlist [start : step : step] .here'showitworks : 1) startistheindexofthefirstelementtoinclude.2) stopistheindexofthefirstelemement.3) stepisincrementbetwetweentractionsoftortionsoflists

NumpyAllowsForVariousOperationsOnArrays : 1) BasicArithmeticLikeadDition, Subtraction, A 및 Division; 2) AdvancedOperationsSuchasmatrixmultiplication; 3) extrayintondsfordatamanipulation; 5) Ag
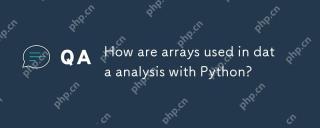
Arraysinpython, 특히 Stroughnumpyandpandas, areestentialfordataanalysis, setingspeedandefficiency


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기
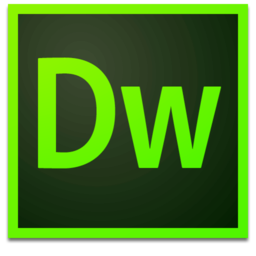
Dreamweaver Mac版
시각적 웹 개발 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기
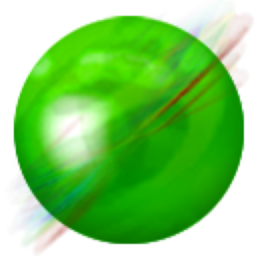
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경
