JavaScript에서 HTTP 요청을 수행하는 방법에는 여러 가지가 있지만 가장 널리 사용되는 두 가지 방법은 Axios와 기본 fetch() API입니다. 이 게시물에서는 이 두 가지 방법을 비교 및 대조하여 다양한 시나리오에 어떤 방법이 더 적합한지 결정할 것입니다.
HTTP 요청의 필수 역할
HTTP 요청은 웹 애플리케이션에서 서버 및 API와 통신하는 데 기본입니다. Axios와 fetch()는 이러한 요청을 효과적으로 처리하기 위해 널리 사용됩니다. 각 기능을 자세히 살펴보고 어떤 특징이 있는지 살펴보겠습니다.
액시오스(Axios)란 무엇입니까?
Axios는 HTTP 요청을 위한 약속 기반 HTTP 클라이언트를 제공하는 타사 라이브러리입니다. 단순성과 유연성으로 잘 알려져 있으며 JavaScript 커뮤니티에서 널리 사용됩니다.
Axios의 기본 구문
axios(config) .then(response => console.log(response.data)) .catch(error => console.error('Error:', error));
Axios의 주요 기능:
- 구성 유연성: URL과 구성 개체를 모두 허용합니다.
- 자동 데이터 처리: 데이터를 JSON과 자동으로 변환합니다.
- 오류 처리: HTTP 오류 상태 코드를 자동으로 처리하여 catch 블록에 전달합니다.
- 간단한 응답: 응답 개체의 data 속성에 직접 서버 데이터를 반환합니다.
- 간소화된 오류 관리: 더욱 간소화된 오류 처리 메커니즘을 제공합니다.
예:
axios({ method: 'post', url: 'https://api.example.com/data', data: { key: 'value' } }) .then(response => console.log(response.data)) .catch(error => { if (error.response) { console.error('Server responded with:', error.response.status); } else if (error.request) { console.error('No response received'); } else { console.error('Error:', error.message); } });
Axios를 사용하는 이유는 무엇입니까?
- 자동 JSON 데이터 변환: JSON과 데이터를 원활하게 변환합니다.
- 응답 시간 초과: 요청 시간 초과를 설정할 수 있습니다.
- HTTP 인터셉터: 요청과 응답을 가로챌 수 있습니다.
- 다운로드 진행 상황: 다운로드 및 업로드 진행 상황을 추적합니다.
- 동시 요청: 여러 요청을 동시에 처리하고 응답을 결합합니다.
가져오기란 무엇입니까?
fetch()는 모든 최신 브라우저에서 지원되는 최신 JavaScript에 내장된 API입니다. Promise 형태로 데이터를 반환하는 비동기식 웹 API입니다.
가져오기()의 기능:
- 기본 구문: 간단하고 간결하며 URL과 선택적 옵션 개체를 사용합니다.
- 하위 호환성: 폴리필이 있는 이전 브라우저에서 사용할 수 있습니다.
- 사용자 정의 가능: 헤더, 본문, 메소드, 모드, 자격 증명, 캐시, 리디렉션 및 리퍼러 정책을 세부적으로 제어할 수 있습니다.
Axios를 사용하여 HTTP 요청을 만드는 방법
먼저 npm 또는 Yarn을 사용하여 Axios를 설치합니다.
axios(config) .then(response => console.log(response.data)) .catch(error => console.error('Error:', error));
CDN을 통해 Axios를 포함할 수도 있습니다.
axios({ method: 'post', url: 'https://api.example.com/data', data: { key: 'value' } }) .then(response => console.log(response.data)) .catch(error => { if (error.response) { console.error('Server responded with:', error.response.status); } else if (error.request) { console.error('No response received'); } else { console.error('Error:', error.message); } });
Axios를 사용하여 GET 요청을 하는 방법은 다음과 같습니다.
npm install axios # or yarn add axios # or pnpm install axios
Fetch를 사용하여 HTTP 요청 만들기
fetch()가 내장되어 있으므로 아무것도 설치할 필요가 없습니다. fetch()를 사용하여 GET 요청을 만드는 방법은 다음과 같습니다.
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
다음 사항에 유의하세요.
- 데이터 처리: Axios는 자동으로 데이터를 JSON으로 변환하고, fetch()를 사용하면 response.json()을 수동으로 호출해야 합니다.
- 오류 처리: Axios는 catch 블록 내의 오류를 처리하는 반면, fetch()는 HTTP 상태 오류가 아닌 네트워크 오류에 대한 약속만 거부합니다.
Fetch의 기본 구문
import axios from 'axios'; axios.get('https://example.com/api') .then(response => console.log(response.data)) .catch(error => console.error(error));
주요 특징:
- 간단한 인수: URL과 선택적 구성 개체를 사용합니다.
- 수동 데이터 처리: 데이터를 문자열로 수동 변환해야 합니다.
- 응답 객체: 완전한 응답 정보가 포함된 응답 객체를 반환합니다.
- 오류 처리: HTTP 오류에 대한 응답 상태 코드를 수동으로 확인해야 합니다.
예:
fetch('https://example.com/api') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
Axios와 Fetch의 비교
쿼리 매개변수와 함께 GET 요청 보내기
액시오스:
fetch(url, options) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
가져오기:
fetch('https://api.example.com/data', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ key: 'value' }) }) .then(response => { if (!response.ok) throw new Error('HTTP error ' + response.status); return response.json(); }) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
JSON 본문을 사용하여 POST 요청 보내기
액시오스:
axios.get('/api/data', { params: { name: 'Alice', age: 25 } }) .then(response => { /* handle response */ }) .catch(error => { /* handle error */ });
가져오기:
const url = new URL('/api/data'); url.searchParams.append('name', 'Alice'); url.searchParams.append('age', 25); fetch(url) .then(response => response.json()) .then(data => { /* handle data */ }) .catch(error => { /* handle error */ });
요청에 대한 시간 초과 설정
액시오스:
axios.post('/api/data', { name: 'Bob', age: 30 }) .then(response => { /* handle response */ }) .catch(error => { /* handle error */ });
가져오기:
fetch('/api/data', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ name: 'Bob', age: 30 }) }) .then(response => response.json()) .then(data => { /* handle data */ }) .catch(error => { /* handle error */ });
async/await 구문 사용
액시오스:
axios.get('/api/data', { timeout: 5000 }) // 5 seconds .then(response => { /* handle response */ }) .catch(error => { /* handle error */ });
가져오기:
const controller = new AbortController(); const signal = controller.signal; setTimeout(() => controller.abort(), 5000); // abort after 5 seconds fetch('/api/data', { signal }) .then(response => response.json()) .then(data => { /* handle data */ }) .catch(error => { /* handle error */ });
이전 버전과의 호환성
액시오스:
- 프로젝트에 설치하고 포함해야 합니다.
- Promise 및 기타 최신 JavaScript 기능에 대한 폴리필을 사용하여 이전 브라우저를 지원합니다.
- 새로운 환경과의 호환성을 위해 적극적으로 유지관리합니다.
가져오기:
- 최신 브라우저에서 기본 지원
- 이전 브라우저를 지원하기 위해 폴리필이 가능합니다.
- 브라우저 공급업체에 의해 자동으로 업데이트됩니다.
오류 처리
액시오스:
catch 블록의 오류를 처리하고 2xx 외부의 모든 상태 코드를 오류로 간주합니다.
async function getData() { try { const response = await axios.get('/api/data'); // handle response } catch (error) { // handle error } }
가져오기:
수동 상태 확인 필요:
async function getData() { try { const response = await fetch('/api/data'); const data = await response.json(); // handle data } catch (error) { // handle error } }
Axios vs Fetch: 어느 것이 가장 좋나요?
귀하의 요구 사항에 따라 다르기 때문에 정해진 답은 없습니다.
- 자동 JSON 데이터 변환, HTTP 인터셉터, 고급 오류 처리와 같은 기능이 필요한 경우 Axios를 사용하세요.
- 광범위한 사용자 정의 옵션을 갖춘 기본적이고 가벼운 솔루션을 원한다면 fetch()를 사용하세요.
EchoAPI를 사용하여 Axios/Fetch 코드 생성
EchoAPI는 API 설계, 디버깅, 테스트 및 모의를 위한 도구를 제공하는 올인원 협업 API 개발 플랫폼입니다. EchoAPI는 HTTP 요청을 위한 Axios 코드를 자동으로 생성할 수 있습니다.
EchoAPI를 사용하여 Axios 코드를 생성하는 단계:
1. EchoAPI를 열고 새 요청을 생성합니다.
2. API 엔드포인트, 헤더 및 매개변수를 입력한 후 "코드 조각"을 클릭합니다.
3. "클라이언트 코드 생성"을 선택하십시오.
4. 생성된 Axios 코드를 복사하여 프로젝트에 붙여넣습니다.
결론
Axios와 fetch()는 모두 JavaScript에서 HTTP 요청을 만드는 강력한 방법입니다. 프로젝트의 필요와 선호도에 가장 적합한 것을 선택하세요. EchoAPI와 같은 도구를 활용하면 개발 워크플로우를 향상시켜 코드가 정확하고 효율적이도록 보장할 수 있습니다. 즐거운 코딩하세요!
위 내용은 Axios와 Fetch: HTTP 요청에 가장 적합한 것은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
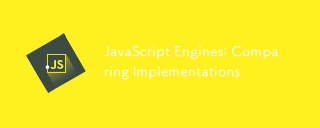
각각의 엔진의 구현 원리 및 최적화 전략이 다르기 때문에 JavaScript 엔진은 JavaScript 코드를 구문 분석하고 실행할 때 다른 영향을 미칩니다. 1. 어휘 분석 : 소스 코드를 어휘 단위로 변환합니다. 2. 문법 분석 : 추상 구문 트리를 생성합니다. 3. 최적화 및 컴파일 : JIT 컴파일러를 통해 기계 코드를 생성합니다. 4. 실행 : 기계 코드를 실행하십시오. V8 엔진은 즉각적인 컴파일 및 숨겨진 클래스를 통해 최적화하여 Spidermonkey는 유형 추론 시스템을 사용하여 동일한 코드에서 성능이 다른 성능을 제공합니다.
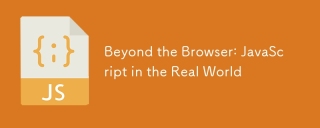
실제 세계에서 JavaScript의 응용 프로그램에는 서버 측 프로그래밍, 모바일 애플리케이션 개발 및 사물 인터넷 제어가 포함됩니다. 1. 서버 측 프로그래밍은 Node.js를 통해 실현되며 동시 요청 처리에 적합합니다. 2. 모바일 애플리케이션 개발은 재교육을 통해 수행되며 크로스 플랫폼 배포를 지원합니다. 3. Johnny-Five 라이브러리를 통한 IoT 장치 제어에 사용되며 하드웨어 상호 작용에 적합합니다.
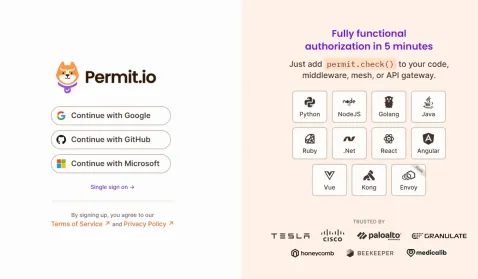
일상적인 기술 도구를 사용하여 기능적 다중 테넌트 SaaS 응용 프로그램 (Edtech 앱)을 구축했으며 동일한 작업을 수행 할 수 있습니다. 먼저, 다중 테넌트 SaaS 응용 프로그램은 무엇입니까? 멀티 테넌트 SAAS 응용 프로그램은 노래에서 여러 고객에게 서비스를 제공 할 수 있습니다.
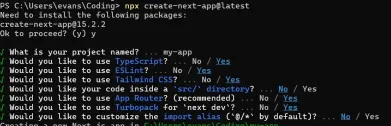
이 기사에서는 Contrim에 의해 확보 된 백엔드와의 프론트 엔드 통합을 보여 주며 Next.js를 사용하여 기능적인 Edtech SaaS 응용 프로그램을 구축합니다. Frontend는 UI 가시성을 제어하기 위해 사용자 권한을 가져오고 API가 역할 기반을 준수하도록합니다.
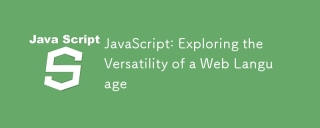
JavaScript는 현대 웹 개발의 핵심 언어이며 다양성과 유연성에 널리 사용됩니다. 1) 프론트 엔드 개발 : DOM 운영 및 최신 프레임 워크 (예 : React, Vue.js, Angular)를 통해 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축합니다. 2) 서버 측 개발 : Node.js는 비 차단 I/O 모델을 사용하여 높은 동시성 및 실시간 응용 프로그램을 처리합니다. 3) 모바일 및 데스크탑 애플리케이션 개발 : 크로스 플랫폼 개발은 개발 효율을 향상시키기 위해 반응 및 전자를 통해 실현됩니다.
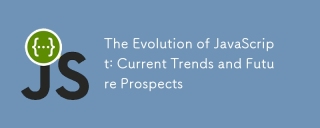
JavaScript의 최신 트렌드에는 Typescript의 Rise, 현대 프레임 워크 및 라이브러리의 인기 및 WebAssembly의 적용이 포함됩니다. 향후 전망은보다 강력한 유형 시스템, 서버 측 JavaScript 개발, 인공 지능 및 기계 학습의 확장, IoT 및 Edge 컴퓨팅의 잠재력을 포함합니다.
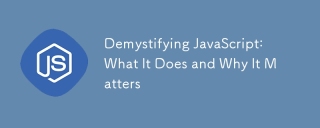
JavaScript는 현대 웹 개발의 초석이며 주요 기능에는 이벤트 중심 프로그래밍, 동적 컨텐츠 생성 및 비동기 프로그래밍이 포함됩니다. 1) 이벤트 중심 프로그래밍을 사용하면 사용자 작업에 따라 웹 페이지가 동적으로 변경 될 수 있습니다. 2) 동적 컨텐츠 생성을 사용하면 조건에 따라 페이지 컨텐츠를 조정할 수 있습니다. 3) 비동기 프로그래밍은 사용자 인터페이스가 차단되지 않도록합니다. JavaScript는 웹 상호 작용, 단일 페이지 응용 프로그램 및 서버 측 개발에 널리 사용되며 사용자 경험 및 크로스 플랫폼 개발의 유연성을 크게 향상시킵니다.
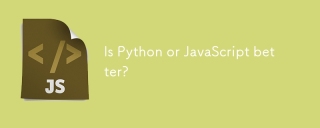
Python은 데이터 과학 및 기계 학습에 더 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 더 적합합니다. 1. Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명하며 데이터 분석 및 웹 개발에 적합합니다. 2. JavaScript는 프론트 엔드 개발의 핵심입니다. Node.js는 서버 측 프로그래밍을 지원하며 풀 스택 개발에 적합합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
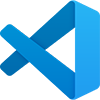
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전
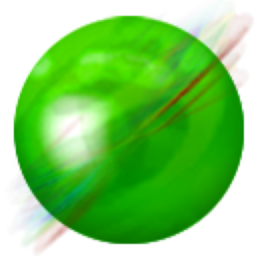
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경

SublimeText3 영어 버전
권장 사항: Win 버전, 코드 프롬프트 지원!

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기
