가변 템플릿 함수와 함께 Dectype을 사용하는 후행 반환 유형
다양한 유형의 인수를 합산하여 반환하는 변형 템플릿 함수를 만들려고 할 때 합계를 적절하게 입력하면 일반적인 문제가 발생합니다.
문제 공식화
decltype을 후행 반환 유형으로 사용하는 기본 구현에서는 2개를 초과하는 인수에 대해 정의되지 않은 동작이 발생합니다. 이를 방지하기 위해 함수를 명시적으로 선언할 수 있지만 이로 인해 여러 인수에 대한 잘못된 유형 추론이 발생합니다.
사용자 정의 특성 클래스를 사용한 솔루션
이러한 문제를 극복하려면, sum_type이라는 사용자 정의 특성 클래스가 활용됩니다. std::add_rvalue_reference 및 std::val을 사용하여 반환 유형을 재귀적으로 계산합니다.
template<class t class... p> struct sum_type; template<class t> struct sum_type<t> : id<t> {}; template<class t class u class... p> struct sum_type<t> : sum_type() + val<const u>() ), P... > {};</const></t></class></t></t></class></class>
수정된 구현
decltype을 유형 이름 sum_type
template <class t class... p> auto sum(const T& t, const P&... p) -> typename sum_type<t>::type { return t + sum(p...); }</t></class>
향상된 유형 추론
또한 sum_type의 마지막 특수화에 대한 수정은 향상된 유형 추론을 제공합니다.
template<class t class u class... p> struct sum_type<t> : id<decltype val>() + val<typename sum_type>::type>() )>{};</typename></decltype></t></class>
이렇게 하면 반환 유형이 decltype(a (b c))와 일치하여 예상 추가 순서에 맞춰 정렬됩니다.
위 내용은 다양한 유형의 인수를 합하는 함수에 대한 올바른 반환 유형을 추론하기 위해 후행 반환 유형을 가변 템플릿 함수와 함께 어떻게 사용할 수 있습니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
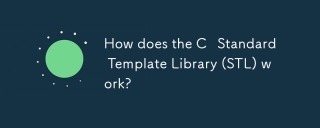
이 기사에서는 컨테이너, 반복자, 알고리즘 및 함수 인 핵심 구성 요소에 중점을 둔 C 표준 템플릿 라이브러리 (STL)에 대해 설명합니다. 일반적인 프로그래밍을 가능하게하기 위해 이러한 상호 작용, 코드 효율성 및 가독성 개선 방법에 대해 자세히 설명합니다.
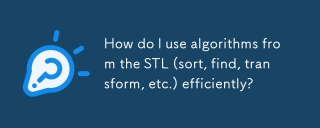
이 기사는 효율적인 STL 알고리즘 사용을 자세히 설명합니다. 데이터 구조 선택 (벡터 대 목록), 알고리즘 복잡성 분석 (예 : std :: sort vs. std :: partial_sort), 반복자 사용 및 병렬 실행을 강조합니다. 일반적인 함정과 같은
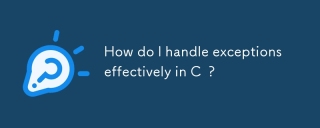
이 기사는 C에서 효과적인 예외 처리를 자세히 설명하고, 시도, 캐치 및 던지기 메커니즘을 다룹니다. RAII와 같은 모범 사례, 불필요한 캐치 블록을 피하고 강력한 코드에 대한 예외를 기록합니다. 이 기사는 또한 Perf를 다룹니다
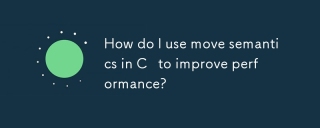
이 기사는 C에서 Move Semantics를 사용하여 불필요한 복사를 피함으로써 성능을 향상시키는 것에 대해 논의합니다. STD :: MOVE를 사용하여 이동 생성자 및 할당 연산자 구현을 다루고 효과적인 APPL을위한 주요 시나리오 및 함정을 식별합니다.
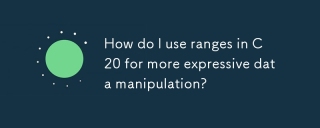
C 20 범위는 표현성, 합성 가능성 및 효율성으로 데이터 조작을 향상시킵니다. 더 나은 성능과 유지 관리를 위해 복잡한 변환을 단순화하고 기존 코드베이스에 통합합니다.
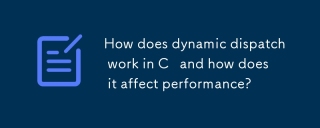
이 기사는 C의 동적 파견, 성능 비용 및 최적화 전략에 대해 설명합니다. 동적 파견이 성능에 영향을 미치는 시나리오를 강조하고이를 정적 파견과 비교하여 성능과 성능 간의 트레이드 오프를 강조합니다.
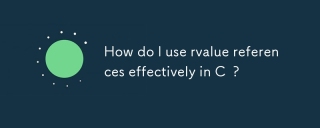
기사는 Move Semantics, Perfect Forwarding 및 Resource Management에 대한 C에서 RValue 참조의 효과적인 사용에 대해 논의하여 모범 사례 및 성능 향상을 강조합니다 (159 자).
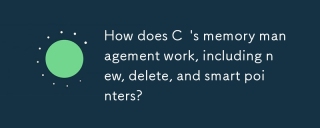
C 메모리 관리는 새로운, 삭제 및 스마트 포인터를 사용합니다. 이 기사는 매뉴얼 대 자동화 된 관리 및 스마트 포인터가 메모리 누출을 방지하는 방법에 대해 설명합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.
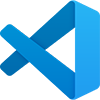
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.
