희소 행렬은 두 개의 배열 인덱싱 연산만 사용하여 테이블에 요소가 있는지 여부를 계산함으로써 특정 행렬 요소에 대한 빠른 액세스를 제공하는 try를 사용하여 효율적으로 구현할 수 있습니다.
Tries의 주요 기능:
- 기본값에 대한 백업 저장소의 기본 위치를 제공하여 값 테스트가 필요하지 않습니다.
- 빠른 업데이트 지원 백업 저장소 크기를 최적화하기 위해 선택적 "compact()" 작업을 시도합니다.
- 객체 매핑을 활용하여 좌표를 벡터의 정수 위치로 매핑할 수 있습니다.
- 하위 범위의 빠른 검색을 처리합니다. 더 빠른 데이터 액세스를 위해.
장점:
- Trie 구현은 해시맵보다 훨씬 빠르며 복잡한 해싱 기능과 충돌 처리를 방지합니다.
- Java 해시맵은 객체에 대한 인덱스만 수행하므로 잠재적으로 메모리 오버헤드와 가비지 수집 스트레스가 발생할 수 있습니다.
- 시도는 각 소스 인덱스에 대해 객체를 생성할 필요가 없는 효율적인 구현을 제공하여 메모리 작업을 줄입니다.
구현 예:
<code class="java">public class DoubleTrie { // Matrix options private static final int SIZE_I = 1024; private static final int SIZE_J = 1024; private static final double DEFAULT_VALUE = 0.0; // Internal splitting options private static final int SUBRANGEBITS_I = 4; private static final int SUBRANGEBITS_J = 4; // Internal splitting constants private static final int SUBRANGE_I = 1 > SUBRANGEBITS_I) * SUBRANGE_J + (j >> SUBRANGEBITS_J); } private static int positionOffsetOf(int i, int j) { return (i & SUBRANGEMASK_I) * SUBRANGE_J + (j & SUBRANGEMASK_J); } // Fast indexed getter public double getAt(int i, int j) { return values[subrangePositions[subrangeOf(i, j)] + positionOffsetOf(i, j)]; } // Fast indexed setter public double setAt(int i, int j, double value) { final int subrange = subrangeOf(i, j); final int positionOffset = positionOffsetOf(i, j); // Check if the assignment will change something int subrangePosition, valuePosition; if (Double.compare( values[valuePosition = (subrangePosition = subrangePositions[subrange]) + positionOffset], value) != 0) { // Perform the assignment in values if (isSharedValues) { values = values.clone(); isSharedValues = false; } // Scan other subranges to check if the value is shared by another subrange for (int otherSubrange = subrangePositions.length; --otherSubrange >= 0; ) { if (otherSubrange != subrange) continue; // Ignore the target subrange if ((otherSubrangePosition = subrangePositions[otherSubrange]) >= valuePosition && otherSubrangePosition + SUBRANGE_POSITIONS </code>
위 내용은 희소 행렬을 효율적으로 구현하기 위해 시도를 어떻게 사용할 수 있습니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
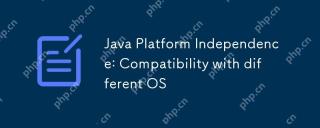
javaachievesplatformincendenceThoughthehoughthejavavirtualmachine (JVM), hittoutModification.thejvmcompileSjavacodeIntOplatform-independentByTecode, whatitTengretsAndexeSontheSpecoS, toplacetSonthecificos, toacketSecificos
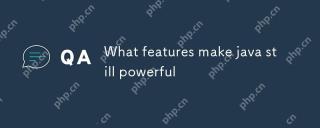
javaispowerfuldueToitsplatformincendence, 객체 지향적, RichandardLibrary, PerformanceCapabilities 및 StrongSecurityFeatures.1) Platform IndependenceAllowsApplicationStorunannyDevicesUpportingjava.2) 대상 지향적 프로그래밍 프로모션 Modulara
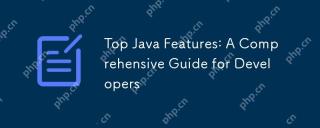
최고 Java 기능에는 다음이 포함됩니다. 1) 객체 지향 프로그래밍, 다형성 지원, 코드 유연성 및 유지 관리 가능성 향상; 2) 예외 처리 메커니즘, 시도 캐치-패치 블록을 통한 코드 견고성 향상; 3) 쓰레기 수집, 메모리 관리 단순화; 4) 제네릭, 유형 안전 강화; 5) 코드를보다 간결하고 표현력있게 만들기위한 AMBDA 표현 및 기능 프로그래밍; 6) 최적화 된 데이터 구조 및 알고리즘을 제공하는 풍부한 표준 라이브러리.
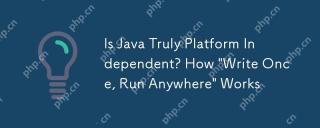
javaisnotentirelyplatformindent의 의존적 duetojvmvariationsandnativecodeintegration
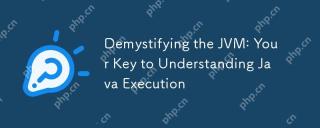
TheJavavirtualMachine (JVM) isanabstractcomputingmachinecrucialforjavaexecutionasitsjavabytecode, "writeonce, runanywhere"기능을 가능하게합니다
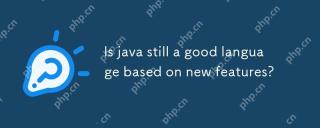
javaremainsagoodlugageedueToitscontinuousevolutionandrobustecosystem.1) lambdaexpressionsenhancececeadeabilitys.2) Streamsallowforefficileddataprocessing, 특히 플레어로드 라트 웨이션
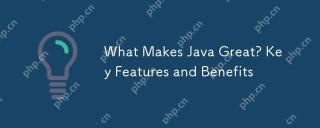
javaisgreatduetoitsplatform incendence, robustoopsupport, extensibraries 및 strongcommunity.1) platforminceptenceviajvmallowscodetorunonvariousplatforms.2) oopeatures inncapsulation, Nheritance, and Polymorphismenblularandscode.3)
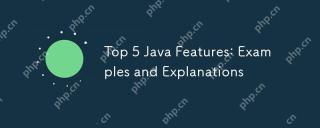
Java의 5 가지 주요 특징은 다형성, Lambda Expressions, Streamsapi, 제네릭 및 예외 처리입니다. 1. 다형성을 사용하면 다른 클래스의 물체가 공통 기본 클래스의 물체로 사용될 수 있습니다. 2. Lambda 표현식은 코드를보다 간결하게 만듭니다. 특히 컬렉션 및 스트림을 처리하는 데 적합합니다. 3.StreamSapi는 대규모 데이터 세트를 효율적으로 처리하고 선언적 작업을 지원합니다. 4. 제네릭은 유형 안전 및 재사용 성을 제공하며 편집 중에 유형 오류가 잡히립니다. 5. 예외 처리는 오류를 우아하게 처리하고 신뢰할 수있는 소프트웨어를 작성하는 데 도움이됩니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구
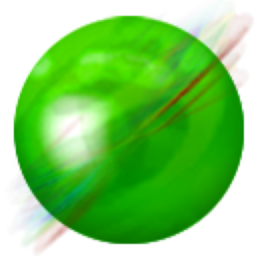
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경

SecList
SecLists는 최고의 보안 테스터의 동반자입니다. 보안 평가 시 자주 사용되는 다양한 유형의 목록을 한 곳에 모아 놓은 것입니다. SecLists는 보안 테스터에게 필요할 수 있는 모든 목록을 편리하게 제공하여 보안 테스트를 더욱 효율적이고 생산적으로 만드는 데 도움이 됩니다. 목록 유형에는 사용자 이름, 비밀번호, URL, 퍼징 페이로드, 민감한 데이터 패턴, 웹 셸 등이 포함됩니다. 테스터는 이 저장소를 새로운 테스트 시스템으로 간단히 가져올 수 있으며 필요한 모든 유형의 목록에 액세스할 수 있습니다.
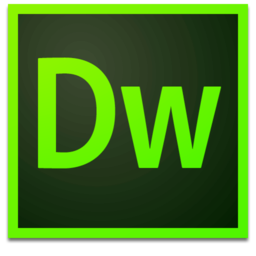
Dreamweaver Mac版
시각적 웹 개발 도구
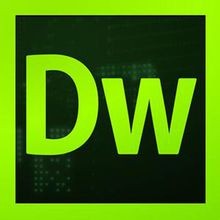
드림위버 CS6
시각적 웹 개발 도구

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.