アイデア
そのアイデアは、DEA 捜査官向けのシンプルなプラットフォームを作成し、ブレイキング バッド/ベター コール ソウルの世界のキャラクターに関する情報を管理することでした。 DEA 捜査官の業務を楽にするためには、登場人物に関する情報を名前、生年月日、職業、容疑者であるかどうかなどでフィルタリングできる API エンドポイントが必要です。
DEA は麻薬組織を刑務所に入れようとしているため、麻薬組織とその周囲の人々を追跡しています。タイムスタンプと特定の場所を地理座標として関連テーブルに保存します。データを公開するエンドポイントでは、特定の地理的地点から特定の距離内にある位置エントリ、割り当て先、および記録時の日時範囲のフィルタリングを許可する必要があります。このエンドポイントの順序付けでは、指定された地理的地点からの距離 (昇順と降順の両方) を考慮できるようにする必要があります。
それがどのように行われたかを確認するには、以下のドキュメントに従ってこのプロジェクトをローカルにセットアップし、自分でテストしてください。
コードは私の GitHub リポジトリにあります。
プロジェクトのセットアップ
前提条件として、システムに Docker と docker-compose がインストールされている必要があります。
まず、プロジェクトのフォルダーに移動し、Breaking Bad API リポジトリのクローンを作成します。
git clone git@github.com:drangovski/breaking-bad-api.git
CD ブレイキング・バッド API
次に、次の変数の値を入れる .env ファイルを作成する必要があります:
POSTGRES_USER=heisenberg POSTGRES_PASSWORD=iamthedanger POSTGRES_DB=breakingbad DEBUG=True SECRET_KEY="<secret key>" DJANGO_ALLOWED_HOSTS=localhost 127.0.0.1 [::1] SQL_ENGINE=django.db.backends.postgresql SQL_DATABASE=breakingbad SQL_USER=heisenberg SQL_PASSWORD=iamthedanger SQL_HOST=db<br> SQL_PORT=5432 </secret>
注: 必要に応じて、env_generator.sh ファイルを使用して .env ファイルを作成できます。これにより、SECRET_KEY も自動的に生成されます。このファイルを実行するには、まず chmod x env_generator.sh でアクセス許可を与え、次に ./env_generator.sh
で実行します。
このセットを入手したら、以下を実行できます:
docker-compose ビルド
docker-compose up
これにより、Django アプリケーションが localhost:8000 で起動されます。 API にアクセスするには、URL は localhost:8000/api になります。
模擬ロケーション
これらのプロジェクトのテーマのため (そして最終的にはあなたの生活を少し楽にするため :))、最終的には次の場所とその座標を使用できます:
Location | Longitude | Latitude |
---|---|---|
Los Pollos Hermanos | 35.06534619552971 | -106.64463423464572 |
Walter White House | 35.12625330483283 | -106.53566597939896 |
Saul Goodman Office | 35.12958969793146 | -106.53106126774908 |
Mike Ehrmantraut House | 35.08486667169461 | -106.64115047513016 |
Jessie Pinkman House | 35.078341181544396 | -106.62404891988452 |
Hank & Marrie House | 35.13512843853582 | -106.48159991250327 |
import requests import json url = 'http://localhost:8000/api/characters/' headers = {'Content-Type' : "application/json"} response = requests.get(url, headers=headers, verify=False) if response.status_code == 200: data = response.json() print(json.dumps(data, indent=2)) else: print("Request failed with status code:", response.status_code)
キャラクター
すべての文字を取得する
データベース内のすべての既存の文字を取得します。
GET /api/characters/
[ { "id": 1, "name": "Walter White", "occupation": "Chemistry Professor", "date_of_birth": "1971", "suspect": false }, { "id": 2, "name": "Tuco Salamanca", "occupation": "Grandpa Keeper", "date_of_birth": "1976", "suspect": true } ]
単一の文字を取得する
単一の文字を取得するには、その文字の ID をエンポイントに渡します。
GET /api/characters/{id}
新しいキャラクターを作成する
新しいキャラクターを作成するには、/characters/ エンドポイントへの POST メソッドを使用できます。
POST /api/characters/
作成パラメータ
キャラクターを正常に作成するには、クエリで次のパラメータを渡す必要があります:
{ "name": "string", "occupation": "string", "date_of_birth": "string", "suspect": boolean }
Parameter | Description |
---|---|
name | String value for the name of the character. |
occupation | String value for the occupation of the character. |
date_of_birth | String value for the date of brith. |
suspect | Boolean parameter. True if suspect, False if not. |
Character ordering
Ordering of the characters can be done by two fields as parameters: name and date_of_birth
GET /api/characters/?ordering={name / date_of_birth}
Parameter | Description |
---|---|
name | Order the results by the name field. |
date_of_birth | Order the results by the date_of_birth field. |
Additionally, you can add the parameter ascending with a value 1 or 0 to order the results in ascending or descending order.
GET /api/characters/?ordering={name / date_of_birth}&ascending={1 / 0}
Parameter | Description |
---|---|
&ascending=1 | Order the results in ascending order by passing 1 as a value. |
&ascending=0 | Order the results in descending order by passing 0 as a value. |
Character filtering
To filter the characters, you can use the parameters in the table below. Case insensitive.
GET /api/characters/?name={text}
Parameter | Description |
---|---|
/?name={text} | Filter the results by name. It can be any length and case insensitive. |
/?occupaton={text} | Filter the results by occupation. It can be any length and case insensitive. |
/?suspect={True / False} | Filter the results by suspect status. It can be True or False. |
Character search
You can also use the search parameter in the query to search characters and retrieve results based on the fields listed below.
GET /api/characters/?search={text}
name
occupation
date_of_birth
Update a character
To update a character, you will need to pass the {id} of a character to the URL and make a PUT method request with the parameters in the table below.
PUT /api/characters/{id}
{ "name": "Mike Ehrmantraut", "occupation": "Retired Officer", "date_of_birth": "1945", "suspect": false }
Parameter | Description |
---|---|
name | String value for the name of the character. |
occupation | String value for the occupation of the character. |
date_of_birth | String value for the date of birth. |
suspect | Boolean parameter. True if suspect, False if not. |
Delete a character
To delete a character, you will need to pass the {id} of a character to the URL and make DELETE method request.
DELETE /api/characters/{id}
Locations
Retrieve all locations
To retrieves all existing locations in the database.
GET /api/locations/
[ { "id": 1, "name": "Los Pollos Hermanos", "longitude": 35.065442792232716, "latitude": -106.6444840309555, "created": "2023-02-09T22:04:32.441106Z", "character": { "id": 2, "name": "Tuco Salamanca", "details": "http://localhost:8000/api/characters/2" } }, ]
Retrieve a single location
To retrieve a single location, pass the locations ID to the endpoint.
GET /api/locations/{id}
Create a new location
You can use the POST method to /locations/ endpoint to create a new location.
POST /api/locations/
Creation parameters
You will need to pass the following parameters in the query, to successfully create a location:
{ "name": "string", "longitude": float, "latitude": float, "character": integer }
Parameter | Description |
---|---|
name | The name of the location. |
longitude | Longitude of the location. |
latitude | Latitude of the location. |
character | This is the id of a character. It is basically ForeignKey relation to the Character model. |
Note: Upon creation of an entry, the Longitude and the Latitude will be converted to a PointField() type of field in the model and stored as a calculated geographical value under the field coordinates, in order for the location coordinates to be eligible for GeoDjango operations.
Location ordering
Ordering of the locations can be done by providing the parameters for the longitude and latitude coordinates for a single point, and a radius (in meters). This will return all of the locations stored in the database, that are in the provided radius from the provided point (coordinates).
GET /api/locations/?longitude={longitude}&latitude={latitude}&radius={radius}
Parameter | Description |
---|---|
longitude | The longitude parameter of the radius point. |
latitude | The latitude parameter of the radius point. |
radius | The radius parameter (in meters). |
Additionally, you can add the parameter ascending with values 1 or 0 to order the results in ascending or descending order.
GET /api/locations/?longitude={longitude}&latitude={latitude}&radius={radius}&ascending={1 / 0}
Parameter | Description |
---|---|
&ascending=1 | Order the results in ascending order by passing 1 as a value. |
&ascending=0 | Order the results in descending order by passing 0 as a value. |
Locaton filtering
To filter the locations, you can use the parameters in the table below. Case insensitive.
GET /api/locations/?character={text}
Parameter | Description |
---|---|
/?name={text} | Filter the results by location name. It can be any length and case insensitive. |
/?character={text} | Filter the results by character. It can be any length and case insensitive. |
/?created={timeframe} | Filter the results by when they were created. Options: today, yesterday, week, month & year. |
Note: You can combine filtering parameters with ordering parameters. Just keep in mind that if you filter by any of these fields above and want to use the ordering parameters, you will always need to pass longitude, latitude and radius altogether. Additionally, if you need to use ascending parameter for ordering, this parameter can't be passed without longitude, latitude and radius as well.
Update a location
To update a location, you will need to pass the {id} of locations to the URL and make a PUT method request with the parameters in the table below.
PUT /api/locations/{id}
{ "id": 1, "name": "Los Pollos Hermanos", "longitude": 35.065442792232716, "latitude": -106.6444840309555, "created": "2023-02-09T22:04:32.441106Z", "character": { "id": 2, "name": "Tuco Salamanca", "occupation": "Grandpa Keeper", "date_of_birth": "1975", "suspect": true } }
Parameter | Description |
---|---|
name | String value for the name of the location. |
longitude | Float value for the longitude of the location. |
latitude | Float value for the latitude of the location. |
Delete a location
To delete a location, you will need to pass the {id} of a location to the URL and make a DELETE method request.
DELETE /api/locations/{id}
위 내용은 Django Rest Framework로 Heisenberg 사냥하기의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
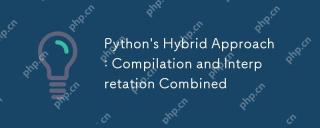
PythonuseSahybrideactroach, combingingcompytobytecodeandingretation.1) codeiscompiledToplatform-IndependentBecode.2) bytecodeistredbythepythonvirtonmachine, enterancingefficiency andportability.
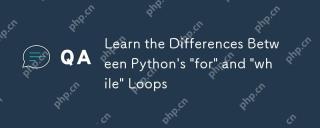
"for"and "while"loopsare : 1) "에 대한"loopsareIdealforitertatingOverSorkNowniterations, whide2) "weekepindiTeRations.Un
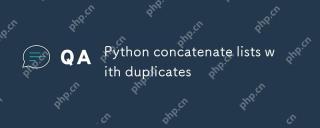
Python에서는 다양한 방법을 통해 목록을 연결하고 중복 요소를 관리 할 수 있습니다. 1) 연산자를 사용하거나 ()을 사용하여 모든 중복 요소를 유지합니다. 2) 세트로 변환 한 다음 모든 중복 요소를 제거하기 위해 목록으로 돌아가지 만 원래 순서는 손실됩니다. 3) 루프 또는 목록 이해를 사용하여 세트를 결합하여 중복 요소를 제거하고 원래 순서를 유지하십시오.
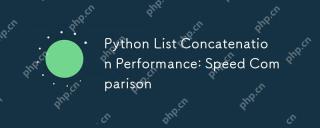
fastestestestedforListCancatenationInpythondSpendsonListsize : 1) Forsmalllist, OperatoriseFficient.2) ForlargerLists, list.extend () OrlistComprehensionIsfaster, withextend () morememory-efficientBymodingListsin-splace.
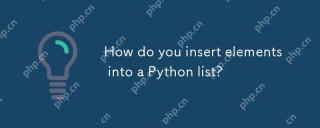
toInsertElmentsIntoapyThonList, useAppend () toaddtotheend, insert () foraspecificposition, andextend () andextend () formultipleElements.1) useappend () foraddingsingleitemstotheend.2) useinsert () toaddatespecificindex, 그러나)
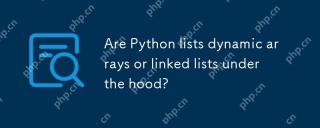
pythonlistsareimplementedesdynamicarrays, notlinkedlists.1) thearestoredIntIguousUousUousUousUousUousUousUousUousUousInSeripendExeDaccess, LeadingSpyTHOCESS, ImpactingEperformance
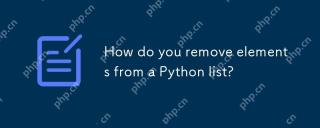
PythonoffersfourmainmethodstoremoveElementsfromalist : 1) 제거 (값) 제거 (값) removesthefirstoccurrencefavalue, 2) pop (index) 제거 elementatAspecifiedIndex, 3) delstatemeveselementsByindexorSlice, 4) RemovesAllestemsfromTheChmetho
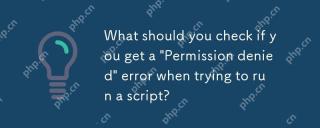
Toresolvea "permissionDenied"오류가 발생할 때 오류가 발생합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구
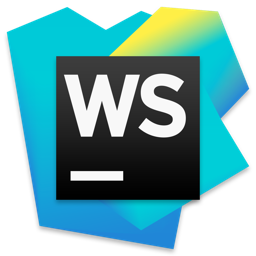
WebStorm Mac 버전
유용한 JavaScript 개발 도구
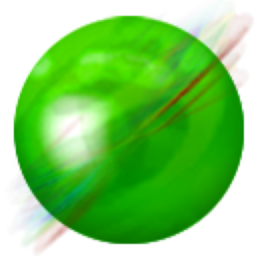
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경
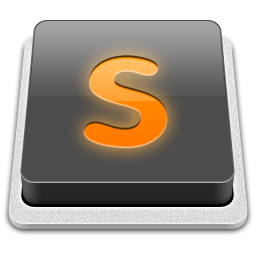
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전