Java를 공부할 때 접하게 되는 기본 개념 중 하나는 생성자입니다. 생성자는 객체가 생성되고 초기화되는 방식에서 중요한 역할을 합니다. 이 게시물에서는 실제 예제를 통해 Java의 생성자, 그 중요성, 다양한 유형 및 사용법을 명확하게 이해할 수 있습니다.
또한 객체를 초기화하고 다양한 방법으로 객체 생성을 처리하는 생성자의 역할을 살펴보겠습니다. 그럼 본격적으로 살펴보겠습니다!
Java의 생성자는 무엇인가요?
Java에서 생성자는 객체가 생성될 때 객체를 초기화하는 데 사용되는 코드 블록입니다. 객체 생성 시 자동으로 호출되어 객체의 초기 상태를 설정합니다. 클래스에 생성자가 명시적으로 정의되어 있지 않으면 Java는 기본 생성자를 호출합니다.
생성자는 두 가지 중요한 점에서 일반 방법과 다릅니다.
- 클래스와 동일한 이름: 생성자는 자신이 속한 클래스와 동일한 이름을 가져야 합니다.
- 반환 없음 유형: 생성자는 void를 비롯한 어떤 값도 반환하지 않습니다.
- 자동 호출: new 키워드를 사용하여 객체가 생성될 때 생성자가 자동으로 호출되므로 명시적으로 호출할 필요가 없습니다.
Java에서 생성자가 왜 중요한가요?
생성자는 일관된 방식으로 새 개체를 초기화하기 위한 프레임워크를 제공하므로 필수적입니다. 모든 객체가 유효하고 의미 있는 데이터로 시작되도록 보장하여 수명 주기 전반에 걸쳐 객체 상태를 더 쉽게 관리할 수 있도록 해줍니다.
생성자를 이해하고 나면 new 키워드를 사용하여 객체를 생성할 때 생성자가 자동으로 호출된다는 점을 이해하게 될 것입니다.
Java의 생성자 유형
Java에는 세 가지 주요 생성자 유형이 있습니다.
- 인수 없는 생성자
- 매개변수화된 생성자
- 기본 생성자
하나하나 자세히 분석해 보겠습니다.
1. 인수 없는 생성자
인수 없는 생성자는 매개변수를 사용하지 않는 생성자입니다. 기본값이나 생성자 내에 정의된 값으로 객체를 초기화합니다.
예:
class Rectangle { double length; double breadth; // No-argument constructor Rectangle() { length = 15.5; breadth = 10.67; } double calculateArea() { return length * breadth; } } class Main { public static void main(String[] args) { Rectangle myRectangle = new Rectangle(); // No-argument constructor is invoked double area = myRectangle.calculateArea(); System.out.println("The area of the Rectangle: " + area); } }
출력: 직사각형의 면적은 165.385입니다.
여기서 인수 없는 생성자는 Rectangle 객체 생성 시 길이와 너비를 기본값으로 초기화합니다.
2. 매개변수화된 생성자
매개변수화된 생성자를 사용하면 인수를 전달하여 특정 값으로 객체를 초기화할 수 있습니다. 이러한 유연성을 통해 초기 상태가 다른 여러 개체를 만들 수 있습니다.
예:
class Rectangle { double length; double breadth; // Parameterized constructor Rectangle(double l, double b) { length = l; breadth = b; } double calculateArea() { return length * breadth; } } class Main { public static void main(String[] args) { Rectangle myRectangle = new Rectangle(20, 30); // Parameterized constructor is invoked double area = myRectangle.calculateArea(); System.out.println("The area of the Rectangle: " + area); } }
출력: 직사각형의 면적은 600.0입니다.
여기서 매개변수화된 생성자는 길이와 너비를 인수로 받아들여 각 객체에 대해 사용자 정의 값을 설정할 수 있습니다.
3. 기본 생성자
클래스에 생성자가 정의되어 있지 않으면 Java는 기본 생성자를 제공합니다. 이 생성자는 인스턴스 변수를 기본값(예: 객체의 경우 null, 숫자의 경우 0)으로 초기화합니다.
예:
class Circle { double radius; double calculateArea() { return Math.PI * radius * radius; } } class Main { public static void main(String[] args) { Circle myCircle = new Circle(); // Default constructor is invoked System.out.println("Radius: " + myCircle.radius); // Output will be 0.0, the default value } }
Circle 클래스는 생성자를 명시적으로 정의하지 않으므로 Java에서는 반경을 0.0으로 초기화하는 기본 생성자를 제공합니다.
Java의 생성자 오버로딩
Java는 클래스가 서로 다른 인수 목록을 가진 여러 생성자를 가질 수 있는 생성자 오버로드를 허용합니다. 각 생성자는 전달된 매개변수에 따라 고유한 작업을 수행합니다.
예:
class Student { String name; int age; // No-argument constructor Student() { name = "Unknown"; age = 0; } // Parameterized constructor Student(String n, int a) { name = n; age = a; } void displayInfo() { System.out.println("Name: " + name + ", Age: " + age); } } class Main { public static void main(String[] args) { Student student1 = new Student(); // Calls no-argument constructor Student student2 = new Student("Alice", 20); // Calls parameterized constructor student1.displayInfo(); // Output: Name: Unknown, Age: 0 student2.displayInfo(); // Output: Name: Alice, Age: 20 } }
이 경우 Student 클래스에는 두 개의 생성자가 있습니다. 하나는 인수가 없고 다른 하나는 매개변수(이름 및 나이)가 있습니다. Java는 객체 생성 시 전달되는 인수의 수와 유형에 따라 이를 구별합니다.
생성자의 this 키워드
Java에서 this 키워드는 클래스의 현재 인스턴스를 참조하는 데 사용됩니다. 생성자 매개변수가 인스턴스 변수와 동일한 이름을 가질 때 모호성을 방지하는 데 유용합니다.
예:
class Employee { String name; double salary; // Parameterized constructor Employee(String name, double salary) { this.name = name; // 'this' refers to the current object's instance variable this.salary = salary; } void display() { System.out.println("Employee Name: " + name); System.out.println("Salary: " + salary); } } class Main { public static void main(String[] args) { Employee emp = new Employee("John", 50000); // Using parameterized constructor emp.display(); } }
이 예에서 this.name은 인스턴스 변수를 참조하고, this가 없는 name은 생성자에 전달된 매개변수를 참조합니다.
생성자와 메소드: 차이점은 무엇인가요?
Constructor | Method |
---|---|
Must have the same name as the class | Can have any name |
No return type (not even void) | Must have a return type |
Invoked automatically when an object is created | Called explicitly by the programmer |
Used to initialize objects | Used to perform actions or computations |
방법
시공자와의 도전
- Java의 생성자는 장점에도 불구하고 다음과 같은 몇 가지 과제를 안고 있습니다.
- 값을 반환할 수 없음: 생성자는 아무것도 반환할 수 없으므로 특정 상황에서 사용이 제한될 수 있습니다.
: 생성자는 상속될 수 없으므로 하위 클래스에 추가 생성자 정의가 필요할 수 있습니다.
결론
생성자는 Java 프로그래밍의 기본 부분입니다. 객체가 적절한 값으로 초기화되도록 보장하고 오버로드를 통해 유연성을 제공합니다. 인수 없음, 매개변수화 또는 기본값 등 생성자를 효과적으로 사용하는 방법을 이해하는 것은 Java를 마스터하는 데 중요합니다.
당신은 어떻습니까? 어떤 종류의 생성자를 사용하는 것을 선호하시나요?
위 내용은 Java의 생성자 마스터하기: 유형 및 예의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
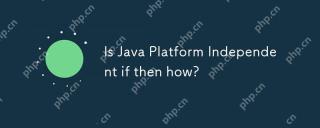
Java는 JVM (Java Virtual Machines) 및 바이트 코드에 의존하는 "Write Once, Everywhere 어디에서나 Run Everywhere"디자인 철학으로 인해 플랫폼 독립적입니다. 1) Java Code는 JVM에 의해 해석되거나 로컬로 계산 된 바이트 코드로 컴파일됩니다. 2) 라이브러리 의존성, 성능 차이 및 환경 구성에주의하십시오. 3) 표준 라이브러리를 사용하여 크로스 플랫폼 테스트 및 버전 관리가 플랫폼 독립성을 보장하기위한 모범 사례입니다.
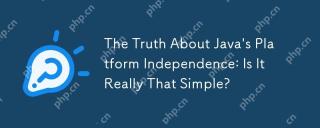
java'splatformincceldenceisisnotsimple; itinvolvescomplex
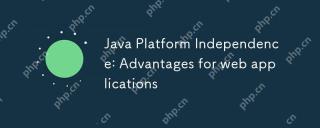
Java'SplatformIndenceBenefitsWebApplicationScodetorUnonySystemwithajvm, simplifyingDeploymentandScaling.Itenables : 1) EasyDeploymentAcrossDifferentservers, 2) SeamlessScalingAcrossCloudPlatforms, 3))
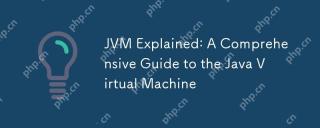
thejvmistheruntimeenvironmenmentforexecutingjavabytecode, Crucialforjava의 "WriteOnce, runanywhere"capability.itmanagesmemory, executesThreads, andensuressecurity, makingestement ofjavadeveloperStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandStandSmetsmentsMemory
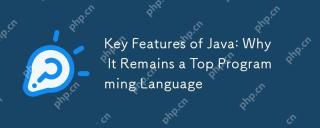
javaremainsatopchoicefordevelopersdueToitsplatformindence, 객체 지향 데 디자인, 강력한, 자동 메모리 관리 및 compehensiveStandardlibrary
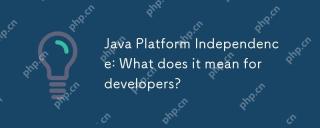
Java'splatforminceldenceMeansdeveloperscanwriteCodeOnceAndrunitonAnyDevicewithoutRecompiling.thisiSocievedTheRoughthejavirtualMachine (JVM), thisTecodeIntomachine-specificinstructions, hallyslatslatsplatforms.howev
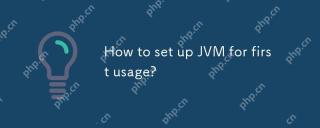
JVM을 설정하려면 다음 단계를 따라야합니다. 1) JDK 다운로드 및 설치, 2) 환경 변수 설정, 3) 설치 확인, 4) IDE 설정, 5) 러너 프로그램 테스트. JVM을 설정하는 것은 단순히 작동하는 것이 아니라 메모리 할당, 쓰레기 수집, 성능 튜닝 및 오류 처리를 최적화하여 최적의 작동을 보장하는 것도 포함됩니다.
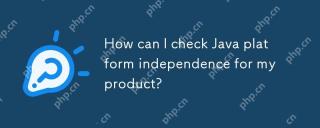
ToensureJavaplatform Independence, followthesesteps : 1) CompileIndrunyourApplicationOnMultiplePlatformsUsingDifferentOnsandjvMversions.2) Utilizeci/CDPIPELINES LICKINSORTIBACTIONSFORAUTOMATES-PLATFORMTESTING


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구
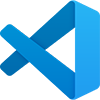
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기
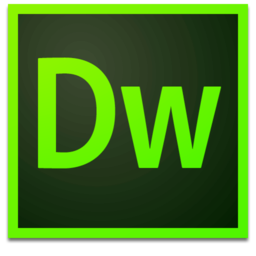
Dreamweaver Mac版
시각적 웹 개발 도구

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경
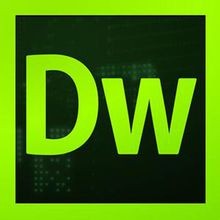
드림위버 CS6
시각적 웹 개발 도구