이 기사에서는 Perl Weekly Challenge #288의 두 가지 작업, 즉 가장 가까운 회문을 찾고 행렬에서 가장 큰 연속 블록의 크기를 결정하는 작업을 다룰 것입니다. 두 솔루션 모두 Perl과 Go에서 재귀적으로 구현됩니다.
목차
- 가장 가까운 회문
- 인접 블록
- 결론
가장 가까운 회문
첫 번째 작업은 자신을 포함하지 않는 가장 가까운 회문을 찾는 것입니다.
가장 가까운 회문은 두 정수 사이의 절대 차이를 최소화하는 회문으로 정의됩니다.
후보가 여러 개인 경우 가장 작은 후보를 반환해야 합니다.
작업 설명
입력: 정수를 나타내는 문자열 $str.
출력: 문자열로 가장 가까운 회문.
예
입력: "123"
출력: "121"입력: "2"
출력: "1"
가장 가까운 회문 두 개가 있습니다: "1"과 "3". 따라서 가장 작은 "1"을 반환합니다.입력: "1400"
출력: "1441"입력: "1001"
출력: "999"
해결책
펄 구현
이 구현에서는 재귀적 접근 방식을 활용하여 원래 숫자와 동일하지 않은 가장 가까운 회문을 찾습니다. 재귀 함수는 원래 숫자 주변의 하한과 상한을 모두 탐색합니다.
- 현재 후보(하위 및 상위)가 유효한 회문인지(원본과 동일하지 않은지) 확인합니다.
- 두 후보 모두 유효하지 않으면 함수는 유효한 회문을 찾을 때까지 하위 후보를 반복적으로 감소시키고 상위 후보를 증가시킵니다.
이 재귀 전략은 검색 공간을 효과적으로 좁혀 문제의 제약 조건을 준수하면서 가장 가까운 회문을 식별할 수 있도록 합니다.
sub is_palindrome { my ($num) = @_; return $num eq reverse($num); } sub find_closest { my ($lower, $upper, $original) = @_; return $lower if is_palindrome($lower) && $lower != $original; return $upper if is_palindrome($upper) && $upper != $original; return find_closest($lower - 1, $upper + 1, $original) if $lower > 0; return $upper + 1; } sub closest_palindrome { my ($str) = @_; my $num = int($str); return find_closest($num - 1, $num + 1, $num); }
구현으로 이동
Go 구현은 유사한 재귀 전략을 따릅니다. 또한 유효한 회문을 찾을 때까지 경계를 조정하기 위해 재귀를 사용하여 원래 숫자 주변의 후보를 확인합니다.
package main import ( "strconv" ) func isPalindrome(num int) bool { reversed := 0 original := num for num > 0 { digit := num % 10 reversed = reversed*10 + digit num /= 10 } return original == reversed } func findClosest(lower, upper, original int) string { switch { case isPalindrome(lower) && lower != original: return strconv.Itoa(lower) case isPalindrome(upper) && upper != original: return strconv.Itoa(upper) case lower > 0: return findClosest(lower-1, upper+1, original) default: return strconv.Itoa(upper + 1) } } func closestPalindrome(str string) string { num, _ := strconv.Atoi(str) return findClosest(num-1, num+1, num) }
Hier ist die erweiterte Definition für den 인접 블록:
연속 블록
두 번째 작업은 모든 셀에 x 또는 o가 포함된 주어진 행렬에서 가장 큰 연속 블록의 크기를 결정하는 것입니다.
인접 블록은 블록의 다른 요소와 모서리(모서리뿐만 아니라)를 공유하는 동일한 기호를 포함하는 요소로 구성되어 연결된 영역을 만듭니다.
작업 설명
입력: x와 o를 포함하는 직사각형 행렬
출력: 가장 큰 연속 블록의 크기
예
-
입력:
[ ['x', 'x', 'x', 'x', 'o'], ['x', 'o', 'o', 'o', 'o'], ['x', 'o', 'o', 'o', 'o'], ['x', 'x', 'x', 'o', 'o'], ]
출력: 11
x를 포함하는 9개의 연속 셀 블록과 o를 포함하는 11개의 연속 셀 블록이 있습니다.
-
입력:
[ ['x', 'x', 'x', 'x', 'x'], ['x', 'o', 'o', 'o', 'o'], ['x', 'x', 'x', 'x', 'o'], ['x', 'o', 'o', 'o', 'o'], ]
출력: 11
x를 포함하는 11개의 연속 셀 블록과 o를 포함하는 9개의 연속 셀 블록이 있습니다.
-
입력:
[ ['x', 'x', 'x', 'o', 'o'], ['o', 'o', 'o', 'x', 'x'], ['o', 'x', 'x', 'o', 'o'], ['o', 'o', 'o', 'x', 'x'], ]
출력: 7
o를 포함하는 7개의 연속 셀 블록, o의 다른 2셀 블록 2개, x의 2셀 블록 3개, x의 3셀 블록 1개가 있습니다.
해결책
펄 구현
이 구현에서는 재귀적 깊이 우선 검색(DFS) 접근 방식을 활용하여 행렬에서 가장 큰 연속 블록의 크기를 결정합니다. 주요 함수는 방문 행렬을 초기화하여 탐색된 셀을 추적합니다. 각 셀을 반복하면서 방문하지 않은 셀을 발견할 때마다 재귀 DFS 기능을 호출합니다.
DFS 기능은 현재 셀에서 가능한 네 가지 방향(위, 아래, 왼쪽, 오른쪽)을 모두 탐색합니다. 동일한 기호를 공유하고 방문되지 않은 인접 셀에서 자신을 재귀적으로 호출하여 연속 블록의 크기를 계산합니다. 이 재귀적 방법은 각 셀이 한 번만 계산되도록 하면서 블록 크기를 효과적으로 집계합니다.
sub largest_contiguous_block { my ($matrix) = @_; my $rows = @$matrix; my $cols = @{$matrix->[0]}; my @visited = map { [(0) x $cols] } 1..$rows; my $max_size = 0; for my $r (0 .. $rows - 1) { for my $c (0 .. $cols - 1) { my $symbol = $matrix->[$r][$c]; my $size = dfs($matrix, \@visited, $r, $c, $symbol); $max_size = $size if $size > $max_size; } } return $max_size; } sub dfs { my ($matrix, $visited, $row, $col, $symbol) = @_; return 0 if $row = @$matrix || $col = @{$matrix->[0]} || $visited->[$row][$col] || $matrix->[$row][$col] ne $symbol; $visited->[$row][$col] = 1; my $count = 1; $count += dfs($matrix, $visited, $row + 1, $col, $symbol); $count += dfs($matrix, $visited, $row - 1, $col, $symbol); $count += dfs($matrix, $visited, $row, $col + 1, $symbol); $count += dfs($matrix, $visited, $row, $col - 1, $symbol); return $count; }
구현으로 이동
Go 구현은 이러한 재귀적 DFS 전략을 반영합니다. 마찬가지로 행렬을 순회하고 재귀를 사용하여 동일한 기호가 있는 연속 셀을 탐색합니다.
package main func largestContiguousBlock(matrix [][]rune) int { rows := len(matrix) if rows == 0 { return 0 } cols := len(matrix[0]) visited := make([][]bool, rows) for i := range visited { visited[i] = make([]bool, cols) } maxSize := 0 for r := 0; r maxSize { maxSize = size } } } return maxSize } func dfs(matrix [][]rune, visited [][]bool, row, col int, symbol rune) int { if row = len(matrix) || col = len(matrix[0]) || visited[row][col] || matrix[row][col] != symbol { return 0 } visited[row][col] = true count := 1 count += dfs(matrix, visited, row+1, col, symbol) count += dfs(matrix, visited, row-1, col, symbol) count += dfs(matrix, visited, row, col+1, symbol) count += dfs(matrix, visited, row, col-1, symbol) return count }
Conclusion
In this article, we explored two intriguing challenges from the Perl Weekly Challenge #288: finding the closest palindrome and determining the size of the largest contiguous block in a matrix.
For the first task, both the Perl and Go implementations effectively utilized recursion to navigate around the original number, ensuring the closest palindrome was found efficiently.
In the second task, the recursive depth-first search approach in both languages allowed for a thorough exploration of the matrix, resulting in an accurate count of the largest contiguous block of identical symbols.
These challenges highlight the versatility of recursion as a powerful tool in solving algorithmic problems, showcasing its effectiveness in both Perl and Go. If you're interested in further exploration or have any questions, feel free to reach out!
You can find the complete code, including tests, on GitHub.
위 내용은 심층 분석: 회문 및 연속 블록을 위한 재귀 솔루션의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
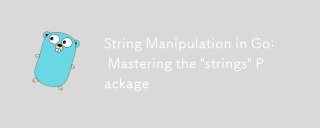
GO 언어로 문자열 패키지를 마스터하면 텍스트 처리 기능과 개발 효율성이 향상 될 수 있습니다. 1) 함유 기능을 사용하여 하위 문자열을 확인하십시오. 2) 인덱스 기능을 사용하여 하위 문자열 위치를 찾으십시오. 빈 문자열을 확인하지 않고 큰 문자열 작동 성능 문제와 같은 일반적인 오류를 피하기 위해주의하십시오.
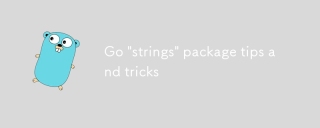
문자열 조작을 단순화하고 코드를보다 명확하고 효율적으로 만들 수 있기 때문에 이동중인 문자열 패키지에주의해야합니다. 1) strings.join을 사용하여 줄을 효율적으로 스플 라이스; 2) strings.fields를 사용하여 빈 문자로 문자열을 나눕니다. 3) 문자열을 통해 기판 위치를 찾으십시오. 4) 문자열을 대체하려면 strings.replaceall을 사용하십시오. 5) 현악기를 효율적으로 스플 라이스로 사용하여 strings.builder를 사용하십시오. 6) 예상치 못한 결과를 피하기 위해 항상 입력을 확인하십시오.
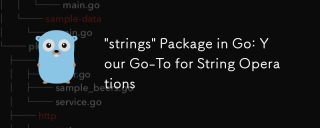
thestringspackageoisessentialponderfficientstringmanipulation.1) itofferssimpleyetpowerfultionsfortaskslikecheckingsubstringsandjoiningstrings.2) ithandlesunicodewell, withFunctionsLikestrings.fieldsforwhitespace-separatedValues.3) forperformance, st
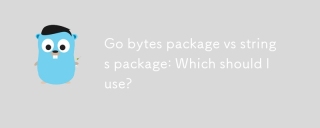
whendecidingbetweengo'sbytespackageandstringspackage, usebytes.bufferforbinarydataandstrings.builderfortringoperations.1) audeBytes.bufferforworkingwhithbyteslices, binarydata, 첨부 DifferentDatatypes, andwritingtoio.2) useastrons
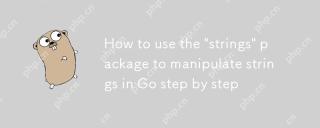
GO의 문자열 패키지는 다양한 문자열 조작 기능을 제공합니다. 1) 문자열을 사용하여 기판을 확인하십시오. 2) strings.split을 사용하여 문자열을 서브 스트링 슬라이스로 분할하십시오. 3) 문자열을 통해 문자열을 병합합니다. 4) 문자열의 시작과 끝에서 strings.trimspace 또는 strings.trim을 사용하여 공백 또는 지정된 문자를 제거하십시오. 5) 지정된 모든 하위 문구를 문자열로 교체하십시오. 6) strings.hasprefix 또는 strings.hassuffix를 사용하여 문자열의 접두사 또는 접미사를 확인하십시오.
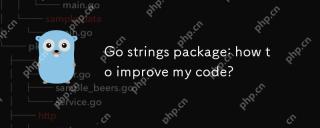
Go Language Strings 패키지를 사용하면 코드 품질이 향상 될 수 있습니다. 1) strings.join ()을 사용하여 성능 오버 헤드를 피하기 위해 문자열 배열을 우아하게 연결하십시오. 2) strings.split () 및 strings.contains ()를 결합하여 텍스트를 처리하고 사례 민감도 문제에주의를 기울입니다. 3) 문자열의 남용을 피하고 ()을 replace ()하고 많은 수의 대체에 정규 표현식을 사용하는 것을 고려하십시오. 4) strings.builder를 사용하여 자주 스 플라이 싱 스트링의 성능을 향상시킵니다.
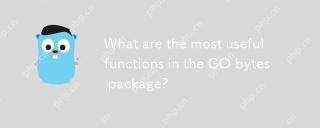
GO의 바이트 패키지는 바이트 슬라이싱을 처리하기위한 다양한 실용적인 기능을 제공합니다. 1. BYTES는 바이트 슬라이스에 특정 시퀀스가 포함되어 있는지 확인하는 데 사용됩니다. 2.Bytes.split은 바이트 슬라이스를 작은 피스로 분할하는 데 사용됩니다. 3.Bytes.join은 여러 바이트 슬라이스를 하나로 연결하는 데 사용됩니다. 4.bytes.trimspace는 바이트 슬라이스의 전면 및 후면 블랭크를 제거하는 데 사용됩니다. 5.Bytes.equal은 두 바이트 슬라이스가 동일인지 비교하는 데 사용됩니다. 6.bytes.index는 LargersLices에서 하위 슬라이스의 시작 지수를 찾는 데 사용됩니다.
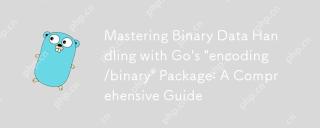
Theencoding/BinaryPackageInsentialBecauseItProvideAstandAdizedWayStandwriteBinaryData, Cross-PlatformCompatibility 및 HandshandlingDifferentendianness.ItoffersFunctionsLikeRead, Write, andwriteUvarIntForPrecisControloverbinary


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경
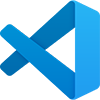
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.