As a developer primarily focused on backend, I've always felt that my frontend skills could use some polishing. To test this, I decided to challenge myself by building a Netflix clone using Vue.js 3 and Vite. In this article, I'll break down the project structure, key components, and share my learning experience.
Project Overview
The goal was to create a responsive web application that mimics the core features of Netflix's user interface. Here's what I initially set out to build:
- A homepage with multiple rows of movies, categorized by genre
- Smooth horizontal scrolling for movie rows
- Lazy loading of images for better performance
- A search functionality to find movies
More to be added in the future.
Tech Stack
For this project, I chose the following tools:
- Vue.js 3: For its reactivity system and component-based architecture
- Vite: As a fast build tool and development server
- Vue Router: For handling navigation
- Pinia: For state management
- Axios: For making API requests to TMDB
- @vueuse/motion: For adding smooth animations
Project Structure
Here's an overview of the project structure:
netflix-clone/ ├── src/ │ ├── components/ │ │ ├── MovieCard.vue │ │ ├── MovieList.vue │ │ ├── MovieRow.vue │ │ └── NavBar.vue │ ├── views/ │ │ ├── HomeView.vue │ │ ├── MovieDetailView.vue │ │ └── SearchView.vue │ ├── router/ │ │ └── index.js │ ├── services/ │ │ └── tmdb.js │ ├── stores/ │ │ └── movies.js │ ├── App.vue │ └── main.js ├── .env.example ├── vite.config.js └── package.json
Key Components Breakdown
MovieCard.vue
This component represents an individual movie. It displays the movie poster and, on hover, shows additional information like the title, rating, and release year.
<template> <div class="movie-card"> <img class="{ lazy" src="/static/imghwm/default1.png" data-src="posterUrl" : :alt="프런트엔드 기술 수준을 높이는 Netflix 복제" true :loaded imageloaded> <div v-if="isHovered" class="movie-info"> <h3 id="프런트엔드-기술-수준을-높이는-Netflix-복제">{{ 프런트엔드 기술 수준을 높이는 Netflix 복제 }}</h3> <p>Rating: {{ movie.vote_average }}/10</p> <p>{{ releaseYear }}</p> </div> </div> </template> <script setup> import { ref, computed } from 'vue'; const props = defineProps(['movie']); const imageLoaded = ref(false); const isHovered = ref(false); const posterUrl = computed(() => `https://image.tmdb.org/t/p/w500${props.movie.poster_path}`); const releaseYear = computed(() => new Date(props.movie.release_date).getFullYear()); // ... hover logic </script>
Key learnings:
- Using computed properties for derived data
- Implementing hover effects with CSS transitions
- Lazy loading images for better performance
MovieRow.vue
This component creates a horizontally scrollable row of movies, typically grouped by genre.
<template> <div class="movie-row"> <h2 id="title">{{ title }}</h2> <div class="movie-list" ref="movieList"> <moviecard v-for="movie in movies" :key="movie.id" :movie="movie"></moviecard> </div> <button class="scroll-btn left"> <button class="scroll-btn right">></button> </button> </div> </template> <script setup> import { ref } from 'vue'; import MovieCard from './MovieCard.vue'; const props = defineProps(['title', 'movies']); const movieList = ref(null); const scroll = (direction) => { const scrollAmount = direction === 'left' ? -300 : 300; movieList.value.scrollBy({ left: scrollAmount, behavior: 'smooth' }); }; </script> ### tmdb.js (API Service) This service handles all API calls to The Movie Database (TMDB) using Axios.
import axios from 'axios'; const API_KEY = import.meta.env.VITE_TMDB_API_KEY; const BASE_URL = 'https://api.themoviedb.org/3'; const tmdbApi = axios.create({ baseURL: BASE_URL, params: { api_key: API_KEY }, }); export const getTrending = () => tmdbApi.get('/trending/all/week'); export const getMoviesByGenre = (genreId) => tmdbApi.get('/discover/movie', { params: { with_genres: genreId } }); export const searchMovies = (query) => tmdbApi.get('/search/movie', { params: { query } });
NavBar.vue
The NavBar component provides navigation for the application and includes a search input for finding movies.
<template> <nav class="navbar"> <router-link to="/" class="navbar-brand">NetflixClone</router-link> <div class="navbar-links"> <router-link to="/">Home</router-link> <div class="search-container"> <input v-model="searchQuery" placeholder="Search movies..."> </div> </div> </nav> </template> <script setup> import { ref } from 'vue'; import { useRouter } from 'vue-router'; import debounce from 'lodash/debounce'; const router = useRouter(); const searchQuery = ref(''); const debounceSearch = debounce(() => { if (searchQuery.value) { router.push({ name: 'search', query: { q: searchQuery.value } }); } }, 300); </script>
HomeView.vue
The HomeView component serves as the main page of the application, displaying multiple MovieRow components with different genres.
<template> <div class="home-view"> <movierow title="Trending" :movies="trendingMovies"></movierow> <movierow v-for="genre in genres" :key="genre.id" :title="genre.name" :movies="moviesByGenre[genre.id]"></movierow> </div> </template> <script setup> import { ref, onMounted } from 'vue'; import MovieRow from '@/components/MovieRow.vue'; import { getTrending, getGenres, getMoviesByGenre } from '@/services/tmdb'; const trendingMovies = ref([]); const genres = ref([]); const moviesByGenre = ref({}); onMounted(async () => { const [trendingResponse, genresResponse] = await Promise.all([ getTrending(), getGenres() ]); trendingMovies.value = trendingResponse.data.results; genres.value = genresResponse.data.genres.slice(0, 5); // Limit to 5 genres for this example for (const genre of genres.value) { const response = await getMoviesByGenre(genre.id); moviesByGenre.value[genre.id] = response.data.results; } }); </script>
SearchView.vue
The SearchView component displays search results based on the user's query.
<template> <div class="search-view"> <h2 id="Search-Results-for-searchQuery">Search Results for "{{ searchQuery }}"</h2> <div class="search-results"> <moviecard v-for="movie in searchResults" :key="movie.id" :movie="movie"></moviecard> </div> </div> </template> <script setup> import { ref, watch } from 'vue'; import { useRoute } from 'vue-router'; import MovieCard from '@/components/MovieCard.vue'; import { searchMovies } from '@/services/tmdb'; const route = useRoute(); const searchQuery = ref(''); const searchResults = ref([]); const performSearch = async () => { const response = await searchMovies(searchQuery.value); searchResults.value = response.data.results; }; watch(() => route.query.q, (newQuery) => { searchQuery.value = newQuery; performSearch(); }, { immediate: true }); </script>
You can find the full source code for this project on GitHub.
위 내용은 프런트엔드 기술 수준을 높이는 Netflix 복제의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
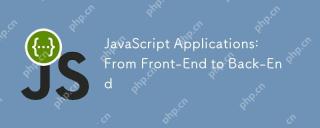
JavaScript는 프론트 엔드 및 백엔드 개발에 사용할 수 있습니다. 프론트 엔드는 DOM 작업을 통해 사용자 경험을 향상시키고 백엔드는 Node.js를 통해 서버 작업을 처리합니다. 1. 프론트 엔드 예 : 웹 페이지 텍스트의 내용을 변경하십시오. 2. 백엔드 예제 : node.js 서버를 만듭니다.
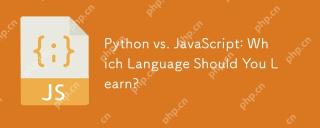
Python 또는 JavaScript는 경력 개발, 학습 곡선 및 생태계를 기반으로해야합니다. 1) 경력 개발 : Python은 데이터 과학 및 백엔드 개발에 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 적합합니다. 2) 학습 곡선 : Python 구문은 간결하며 초보자에게 적합합니다. JavaScript Syntax는 유연합니다. 3) 생태계 : Python에는 풍부한 과학 컴퓨팅 라이브러리가 있으며 JavaScript는 강력한 프론트 엔드 프레임 워크를 가지고 있습니다.
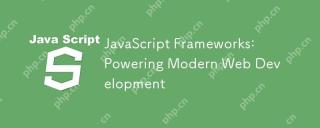
JavaScript 프레임 워크의 힘은 개발 단순화, 사용자 경험 및 응용 프로그램 성능을 향상시키는 데 있습니다. 프레임 워크를 선택할 때 : 1. 프로젝트 규모와 복잡성, 2. 팀 경험, 3. 생태계 및 커뮤니티 지원.
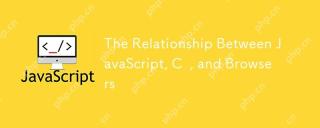
서론 나는 당신이 이상하다는 것을 알고 있습니다. JavaScript, C 및 Browser는 정확히 무엇을해야합니까? 그들은 관련이없는 것처럼 보이지만 실제로는 현대 웹 개발에서 매우 중요한 역할을합니다. 오늘 우리는이 세 가지 사이의 밀접한 관계에 대해 논의 할 것입니다. 이 기사를 통해 브라우저에서 JavaScript가 어떻게 실행되는지, 브라우저 엔진의 C 역할 및 웹 페이지의 렌더링 및 상호 작용을 유도하기 위해 함께 작동하는 방법을 알게됩니다. 우리는 모두 JavaScript와 브라우저의 관계를 알고 있습니다. JavaScript는 프론트 엔드 개발의 핵심 언어입니다. 브라우저에서 직접 실행되므로 웹 페이지를 생생하고 흥미롭게 만듭니다. 왜 Javascr
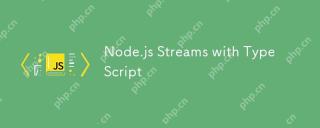
Node.js는 크림 덕분에 효율적인 I/O에서 탁월합니다. 스트림은 메모리 오버로드를 피하고 큰 파일, 네트워크 작업 및 실시간 애플리케이션을위한 메모리 과부하를 피하기 위해 데이터를 점차적으로 처리합니다. 스트림을 TypeScript의 유형 안전과 결합하면 Powe가 생성됩니다
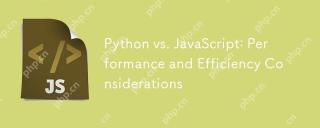
파이썬과 자바 스크립트 간의 성능과 효율성의 차이는 주로 다음과 같이 반영됩니다. 1) 해석 된 언어로서, 파이썬은 느리게 실행되지만 개발 효율이 높고 빠른 프로토 타입 개발에 적합합니다. 2) JavaScript는 브라우저의 단일 스레드로 제한되지만 멀티 스레딩 및 비동기 I/O는 Node.js의 성능을 향상시키는 데 사용될 수 있으며 실제 프로젝트에서는 이점이 있습니다.
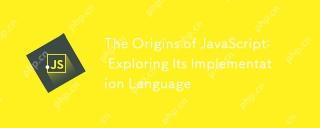
JavaScript는 1995 년에 시작하여 Brandon Ike에 의해 만들어졌으며 언어를 C로 실현했습니다. 1.C Language는 JavaScript의 고성능 및 시스템 수준 프로그래밍 기능을 제공합니다. 2. JavaScript의 메모리 관리 및 성능 최적화는 C 언어에 의존합니다. 3. C 언어의 크로스 플랫폼 기능은 자바 스크립트가 다른 운영 체제에서 효율적으로 실행하는 데 도움이됩니다.
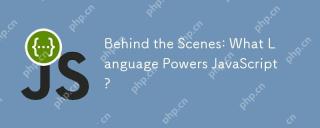
JavaScript는 브라우저 및 Node.js 환경에서 실행되며 JavaScript 엔진을 사용하여 코드를 구문 분석하고 실행합니다. 1) 구문 분석 단계에서 초록 구문 트리 (AST)를 생성합니다. 2) 컴파일 단계에서 AST를 바이트 코드 또는 기계 코드로 변환합니다. 3) 실행 단계에서 컴파일 된 코드를 실행하십시오.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경
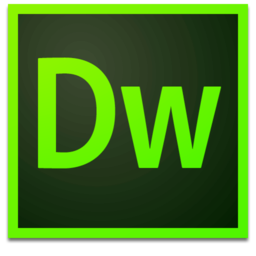
Dreamweaver Mac版
시각적 웹 개발 도구
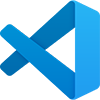
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기
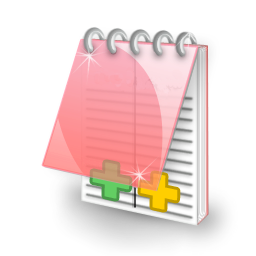
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기