작가: 트릭스 사이러스
당신의 기기에서 호스팅되는 Python 서버를 만들어 보겠습니다.
시작하기..
server라는 디렉터리를 만듭니다
mkdir server
server.py라는 파일을 만드세요
nano server.py
아래 코드를 붙여넣으세요.
import http.server import socketserver import logging import os import threading from urllib.parse import urlparse, parse_qs PORT = 8080 DIRECTORY = "www" logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(message)s', datefmt='%Y-%m-%d %H:%M:%S') class MyHandler(http.server.SimpleHTTPRequestHandler): def __init__(self, *args, **kwargs): super().__init__(*args, directory=DIRECTORY, **kwargs) def log_message(self, format, *args): logging.info("%s - %s" % (self.client_address[0], format % args)) def do_GET(self): parsed_path = urlparse(self.path) query = parse_qs(parsed_path.query) # Custom logic for different routes if parsed_path.path == '/': self.serve_file("index.html") elif parsed_path.path == '/about': self.respond_with_text("<h1 id="About-Us">About Us</h1><p>This is a custom Python server.</p>") elif parsed_path.path == '/greet': name = query.get('name', ['stranger'])[0] self.respond_with_text(f"<h1 id="Hello-name">Hello, {name}!</h1>") else: self.send_error(404, "File Not Found") def do_POST(self): content_length = int(self.headers['Content-Length']) post_data = self.rfile.read(content_length) logging.info("Received POST data: %s", post_data.decode('utf-8')) self.respond_with_text("<h1 id="POST-request-received">POST request received</h1>") def serve_file(self, filename): if os.path.exists(os.path.join(DIRECTORY, filename)): self.send_response(200) self.send_header("Content-type", "text/html") self.end_headers() with open(os.path.join(DIRECTORY, filename), 'rb') as file: self.wfile.write(file.read()) else: self.send_error(404, "File Not Found") def respond_with_text(self, content): self.send_response(200) self.send_header("Content-type", "text/html") self.end_headers() self.wfile.write(content.encode('utf-8')) class ThreadedHTTPServer(socketserver.ThreadingMixIn, http.server.HTTPServer): daemon_threads = True # Handle requests in separate threads def run_server(): try: with ThreadedHTTPServer(("", PORT), MyHandler) as httpd: logging.info(f"Serving HTTP on port {PORT}") logging.info(f"Serving files from directory: {DIRECTORY}") httpd.serve_forever() except Exception as e: logging.error(f"Error starting server: {e}") except KeyboardInterrupt: logging.info("Server stopped by user") if __name__ == "__main__": server_thread = threading.Thread(target=run_server) server_thread.start() server_thread.join()
www라는 디렉터리를 만듭니다
mkdir www
이제 www 디렉토리로 이동하세요
cd www
index.html이라는 파일을 만듭니다
nano index.html
아래 코드를 붙여넣으세요
<meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Python Simple Server</title> <h1 id="Welcome-to-My-Python-Server">Welcome to My Python Server!</h1> <p>This is a simple web server running on your local device.</p>
2단계: 경로 테스트
수정된 스크립트를 실행한 후 다음으로 이동하세요.
홈페이지를 보려면 http://localhost:8080/
http://localhost:8080/about 정보 페이지를 확인하세요.
http://localhost:8080/greet?name=트릭스
다른 경로의 경우 서버는 404 오류를 반환합니다.
아래는 디렉토리 구조입니다
server/ ├── server.py └── www/ └── index.html
원격기기에서 서버 실행
동일한 네트워크에 있는 다른 장치에서 Python 서버에 액세스하려면 어떻게 해야 합니까? 서버를 실행하는 머신의 로컬 IP 주소를 찾아 localhost 대신 사용하면 쉽게 할 수 있습니다.
1단계: IP 주소 찾기
다음과 같은 명령을 사용하세요
ipconfig
ifconfig
IPv4 주소(예: 192.168.x.x)를 찾으세요.
2단계. 서버 스크립트 수정
서버 스크립트에서 서버가 시작되는 줄을 다음과 같이 바꾸세요.
with ThreadedHTTPServer(("", PORT), MyHandler) as httpd:
다음으로 변경:
with ThreadedHTTPServer(("0.0.0.0", PORT), MyHandler) as httpd:
3단계: 다른 장치에서 서버에 액세스
이제 앞서 찾은 IP 주소를 사용하여 브라우저에서 http://:8080
으로 이동하여 동일한 네트워크에 있는 모든 장치에서 서버에 액세스할 수 있습니다.그리고 모든 설정
~트릭스섹
위 내용은 Python을 사용하여 장치를 간단한 서버로 전환하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
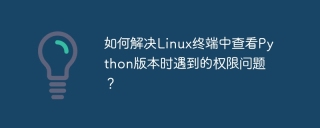
Linux 터미널에서 Python 버전을 보려고 할 때 Linux 터미널에서 Python 버전을 볼 때 권한 문제에 대한 솔루션 ... Python을 입력하십시오 ...
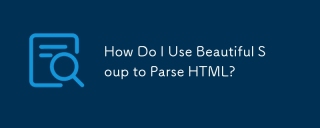
이 기사에서는 HTML을 구문 분석하기 위해 파이썬 라이브러리 인 아름다운 수프를 사용하는 방법을 설명합니다. 데이터 추출, 다양한 HTML 구조 및 오류 처리 및 대안 (SEL과 같은 Find (), find_all (), select () 및 get_text ()와 같은 일반적인 방법을 자세히 설명합니다.
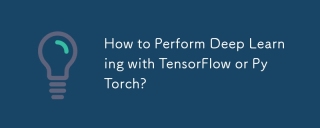
이 기사는 딥 러닝을 위해 텐서 플로와 Pytorch를 비교합니다. 데이터 준비, 모델 구축, 교육, 평가 및 배포와 관련된 단계에 대해 자세히 설명합니다. 프레임 워크, 특히 계산 포도와 관련하여 주요 차이점
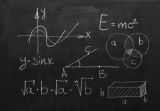
Python의 통계 모듈은 강력한 데이터 통계 분석 기능을 제공하여 생물 통계 및 비즈니스 분석과 같은 데이터의 전반적인 특성을 빠르게 이해할 수 있도록 도와줍니다. 데이터 포인트를 하나씩 보는 대신 평균 또는 분산과 같은 통계를보고 무시할 수있는 원래 데이터에서 트렌드와 기능을 발견하고 대형 데이터 세트를보다 쉽고 효과적으로 비교하십시오. 이 튜토리얼은 평균을 계산하고 데이터 세트의 분산 정도를 측정하는 방법을 설명합니다. 달리 명시되지 않는 한,이 모듈의 모든 함수는 단순히 평균을 합산하는 대신 평균 () 함수의 계산을 지원합니다. 부동 소수점 번호도 사용할 수 있습니다. 무작위로 가져옵니다 수입 통계 Fracti에서
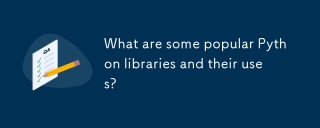
이 기사는 Numpy, Pandas, Matplotlib, Scikit-Learn, Tensorflow, Django, Flask 및 요청과 같은 인기있는 Python 라이브러리에 대해 설명하고 과학 컴퓨팅, 데이터 분석, 시각화, 기계 학습, 웹 개발 및 H에서의 사용에 대해 자세히 설명합니다.
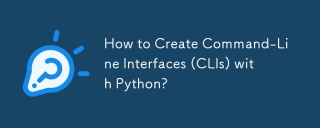
이 기사는 Python 개발자가 CLIS (Command-Line Interfaces) 구축을 안내합니다. Typer, Click 및 Argparse와 같은 라이브러리를 사용하여 입력/출력 처리를 강조하고 CLI 유용성을 향상시키기 위해 사용자 친화적 인 디자인 패턴을 홍보하는 세부 정보.

Python의 Pandas 라이브러리를 사용할 때는 구조가 다른 두 데이터 프레임 사이에서 전체 열을 복사하는 방법이 일반적인 문제입니다. 두 개의 dats가 있다고 가정 해
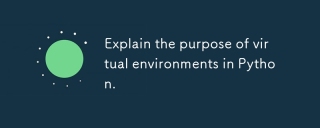
이 기사는 프로젝트 종속성 관리 및 충돌을 피하는 데 중점을 둔 Python에서 가상 환경의 역할에 대해 설명합니다. 프로젝트 관리 개선 및 종속성 문제를 줄이는 데있어 생성, 활성화 및 이점을 자세히 설명합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구
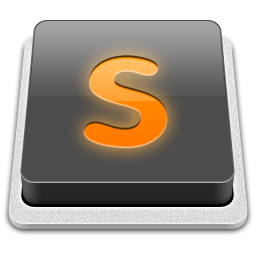
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
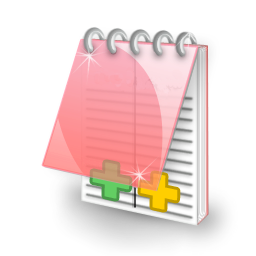
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.
