목차
-
소개
- Prisma, Express, TypeScript, PostgreSQL 개요
- Prisma를 ORM으로 사용해야 하는 이유
- 환경설정
-
초기 프로젝트 설정
- 새 Node.js 프로젝트 설정
- TypeScript 구성
- 필수 패키지 설치
-
PostgreSQL 설정
- PostgreSQL 설치
- 새 데이터베이스 생성
- 환경 변수 구성
-
Prisma 설정
- 프리즈마 설치
- 프로젝트에서 Prisma 초기화
- Prisma 스키마 구성
-
데이터 모델 정의
- PSL(Prisma 스키마 언어) 이해
- API용 모델 생성
- Prisma를 사용한 마이그레이션
-
Prisma와 Express 통합
- 익스프레스 서버 설정
- Prisma를 사용하여 CRUD 작업 생성
- 오류 처리 및 유효성 검사
-
유형 안전을 위해 TypeScript 사용
- Prisma로 유형 정의
- 유형이 안전한 쿼리 및 변형
- API 개발에 TypeScript 활용
-
API 테스트
- Prisma 모델을 위한 단위 테스트 작성
- Supertest와 Jest를 이용한 통합 테스트
- Prisma를 이용한 데이터베이스 모의
-
배포 고려 사항
- 프로덕션용 API 준비
- PostgreSQL 배포
- Node.js 애플리케이션 배포
-
결론
- Express 및 TypeScript와 함께 Prisma를 사용할 때의 이점
- 최종 생각 및 다음 단계
1. 소개
Prisma, Express, TypeScript 및 PostgreSQL 개요
현대 웹 개발에서는 강력하고 확장 가능하며 유형이 안전한 API를 구축하는 것이 중요합니다. ORM으로서의 Prisma, 서버측 로직을 위한 Express, 정적 타이핑을 위한 TypeScript, 안정적인 데이터베이스 솔루션인 PostgreSQL을 결합하여 강력한 RESTful API를 만들 수 있습니다.
Prisma는 유형이 안전한 쿼리, 마이그레이션 및 원활한 데이터베이스 스키마 관리를 지원하는 최신 ORM을 제공하여 데이터베이스 관리를 단순화합니다. Express는 웹 및 모바일 애플리케이션을 위한 강력한 기능 세트를 제공하는 최소한의 유연한 Node.js 웹 애플리케이션 프레임워크입니다. TypeScript는 JavaScript에 정적 유형 정의를 추가하여 개발 프로세스 초기에 오류를 포착하는 데 도움을 줍니다. PostgreSQL은 안정성과 기능 세트로 유명한 강력한 오픈 소스 관계형 데이터베이스 시스템입니다.
왜 Prisma를 ORM으로 사용하나요?
Prisma는 Sequelize 및 TypeORM과 같은 기존 ORM에 비해 몇 가지 장점을 제공합니다.
- 유형이 안전한 데이터베이스 쿼리: 자동으로 생성된 유형은 데이터베이스 쿼리가 유형이 안전하고 오류가 없는지 보장합니다.
- 자동 마이그레이션: Prisma는 데이터베이스 스키마를 Prisma 스키마와 동기화된 상태로 유지하는 강력한 마이그레이션 시스템을 제공합니다.
- 직관적인 데이터 모델링: Prisma 스키마 파일(PSL로 작성)은 이해하고 유지 관리하기 쉽습니다.
- 광범위한 생태계: Prisma는 GraphQL, REST API 및 PostgreSQL과 같은 인기 데이터베이스를 포함한 다른 도구 및 서비스와 원활하게 통합됩니다.
환경 설정
코드를 살펴보기 전에 컴퓨터에 다음 도구가 설치되어 있는지 확인하세요.
- Node.js(LTS 버전 권장)
- npm 또는 Yarn(패키지 관리용)
- TypeScript(정적 타이핑용)
- PostgreSQL(데이터베이스로)
이러한 도구가 설치되면 API 구축을 시작할 수 있습니다.
2. 초기 프로젝트 설정
새 Node.js 프로젝트 설정
- 새 프로젝트 디렉토리 만들기:
mkdir prisma-express-api cd prisma-express-api
- 새 Node.js 프로젝트 초기화:
npm init -y
이렇게 하면 프로젝트 디렉터리에 package.json 파일이 생성됩니다.
TypeScript 구성
- TypeScript 및 Node.js 유형 설치:
npm install typescript @types/node --save-dev
- 프로젝트에서 TypeScript 초기화:
npx tsc --init
이 명령은 TypeScript의 구성 파일인 tsconfig.json 파일을 생성합니다. 프로젝트에 필요에 따라 수정하세요. 기본 설정은 다음과 같습니다.
{ "compilerOptions": { "target": "ES2020", "module": "commonjs", "strict": true, "esModuleInterop": true, "skipLibCheck": true, "forceConsistentCasingInFileNames": true, "outDir": "./dist" }, "include": ["src/**/*"] }
- 프로젝트 구조 만들기:
mkdir src touch src/index.ts
필수 패키지 설치
Express 및 Prisma를 시작하려면 몇 가지 필수 패키지를 설치해야 합니다.
npm install express prisma @prisma/client npm install --save-dev ts-node nodemon @types/express
- express: The web framework for Node.js.
- prisma: The Prisma CLI for database management.
- @prisma/client: The Prisma client for querying the database.
- ts-node: Runs TypeScript directly without the need for precompilation.
- nodemon: Automatically restarts the server on file changes.
- @types/express: TypeScript definitions for Express.
3. Setting Up PostgreSQL
Installing PostgreSQL
PostgreSQL can be installed via your operating system’s package manager or directly from the official website. For example, on macOS, you can use Homebrew:
brew install postgresql brew services start postgresql
Creating a New Database
Once PostgreSQL is installed and running, you can create a new database for your project:
psql postgres CREATE DATABASE prisma_express;
Replace prisma_express with your preferred database name.
Configuring Environment Variables
To connect to the PostgreSQL database, create a .env file in your project’s root directory and add the following environment variables:
DATABASE_URL="postgresql://<user>:<password>@localhost:5432/prisma_express" </password></user>
Replace
4. Setting Up Prisma
Installing Prisma
Prisma is already installed in the previous step, so the next step is to initialize it within the project:
npx prisma init
This command will create a prisma directory containing a schema.prisma file and a .env file. The .env file should already contain the DATABASE_URL you specified earlier.
Configuring the Prisma Schema
The schema.prisma file is where you'll define your data models, which will be used to generate database tables.
Here’s a basic example schema:
generator client { provider = "prisma-client-js" } datasource db { provider = "postgresql" url = env("DATABASE_URL") } model User { id Int @id @default(autoincrement()) name String email String @unique createdAt DateTime @default(now()) posts Post[] } model Post { id Int @id @default(autoincrement()) title String content String? published Boolean @default(false) authorId Int author User @relation(fields: [authorId], references: [id]) }
In this schema, we have two models: User and Post. Each model corresponds to a database table. Prisma uses these models to generate type-safe queries for our database.
5. Defining the Data Model
Understanding Prisma Schema Language (PSL)
Prisma Schema Language (PSL) is used to define your database schema. It's intuitive and easy to read, with a focus on simplicity. Each model in the schema represents a table in your database, and each field corresponds to a column.
Creating Models for the API
In the schema defined earlier, we created two models:
- User: Represents users in our application.
- Post: Represents posts created by users.
Migrations with Prisma
To apply your schema changes to the database, you’ll need to run a migration:
npx prisma migrate dev --name init
This command will create a new migration file and apply it to your database, creating the necessary tables.
6. Integrating Prisma with Express
Setting Up the Express Server
In your src/index.ts, set up the basic Express server:
import express, { Request, Response } from 'express'; import { PrismaClient } from '@prisma/client'; const app = express(); const prisma = new PrismaClient(); app.use(express.json()); app.get('/', (req: Request, res: Response) => { res.send('Hello, Prisma with Express!'); }); const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
This code sets up a simple Express server and initializes the Prisma client.
Creating CRUD Operations with Prisma
Next, let’s create some CRUD (Create, Read, Update, Delete) routes for our User model.
Create a new user:
app.post('/user', async (req: Request, res: Response) => { const { name, email } = req.body; const user = await prisma.user.create({ data: { name, email }, }); res.json(user); });
Read all users:
app.get('/users', async (req: Request, res: Response) => { const users = await prisma.user.findMany(); res.json(users); });
Update a user:
app.put('/user/:id', async (req: Request, res: Response) => { const { id } = req.params; const { name, email } = req.body; const user = await prisma.user.update({ where: { id: Number(id) }, data: { name, email }, }); res.json(user); });
Delete a user:
app.delete('/user/:id', async (req: Request, res: Response) => { const { id } = req.params; const user = await prisma.user.delete({ where: { id: Number(id) }, }); res.json(user); });
Error Handling and Validation
To enhance the robustness of your API, consider adding error handling and validation:
app.post('/user', async (req: Request, res: Response) => { try { const { name, email } = req.body; if (!name || !email) { return res.status(400).json({ error: 'Name and email are required' }); } const user = await prisma.user.create({ data: { name, email }, }); res.json(user); } catch (error) { res.status(500).json({ error: 'Internal Server Error' }); } });
7. Using TypeScript for Type Safety
Defining Types with Prisma
Prisma automatically generates TypeScript types for your models based on your schema. This ensures that your database queries are type-safe.
For example, when creating a new user, TypeScript will enforce the shape of the data being passed:
const user = await prisma.user.create({ data: { name, email }, // TypeScript ensures 'name' and 'email' are strings. });
Type-Safe Queries and Mutations
With TypeScript, you get autocomplete and type-checking for all Prisma queries, reducing the chance of runtime errors:
const users: User[] = await prisma.user.findMany();
Leveraging TypeScript in API Development
Using TypeScript throughout your API development helps catch potential bugs early, improves code readability, and enhances overall development experience.
8. Testing the API
Writing Unit Tests for Prisma Models
Testing is an essential part of any application development. You can write unit tests for your Prisma models using a testing framework like Jest:
npm install jest ts-jest @types/jest --save-dev
Create a jest.config.js file:
module.exports = { preset: 'ts-jest', testEnvironment: 'node', };
Example test for creating a user:
import { PrismaClient } from '@prisma/client'; const prisma = new PrismaClient(); test('should create a new user', async () => { const user = await prisma.user.create({ data: { name: 'John Doe', email: 'john.doe@example.com', }, }); expect(user).toHaveProperty('id'); expect(user.name).toBe('John Doe'); });
Integration Testing with Supertest and Jest
You can also write integration tests using Supertest:
npm install supertest --save-dev
Example integration test:
import request from 'supertest'; import app from './app'; // Your Express app test('GET /users should return a list of users', async () => { const response = await request(app).get('/users'); expect(response.status).toBe(200); expect(response.body).toBeInstanceOf(Array); });
Mocking the Database with Prisma
For testing purposes, you might want to mock the Prisma client. You can do this using tools like jest.mock() or by creating a mock instance of the Prisma client.
9. Deployment Considerations
Preparing the API for Production
Before deploying your API, ensure you:
- Remove all development dependencies.
- Set up environment variables correctly.
- Optimize the build process using tools like tsc and webpack.
Deploying PostgreSQL
You can deploy PostgreSQL using cloud services like AWS RDS, Heroku, or DigitalOcean. Make sure to secure your database with proper authentication and network settings.
Deploying the Node.js Application
For deploying the Node.js application, consider using services like:
- Heroku: For simple, straightforward deployments.
- AWS Elastic Beanstalk: For more control over the infrastructure.
- Docker: To containerize the application and deploy it on any cloud platform.
10. Conclusion
Benefits of Using Prisma with Express and TypeScript
Using Prisma as an ORM with Express and TypeScript provides a powerful combination for building scalable, type-safe, and efficient RESTful APIs. With Prisma, you get automated migrations, type-safe queries, and an intuitive schema language, making database management straightforward and reliable.
Congratulations!! You've now built a robust RESTful API using Prisma, Express, TypeScript, and PostgreSQL. From setting up the environment to deploying the application, this guide covered the essential steps to get you started. As next steps, consider exploring advanced Prisma features like nested queries, transactions, and more complex data models.
Happy coding!
위 내용은 Prisma, Express, TypeScript 및 PostgreSQL을 사용하여 RESTful API 구축의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
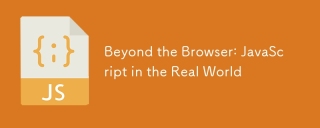
실제 세계에서 JavaScript의 응용 프로그램에는 서버 측 프로그래밍, 모바일 애플리케이션 개발 및 사물 인터넷 제어가 포함됩니다. 1. 서버 측 프로그래밍은 Node.js를 통해 실현되며 동시 요청 처리에 적합합니다. 2. 모바일 애플리케이션 개발은 재교육을 통해 수행되며 크로스 플랫폼 배포를 지원합니다. 3. Johnny-Five 라이브러리를 통한 IoT 장치 제어에 사용되며 하드웨어 상호 작용에 적합합니다.
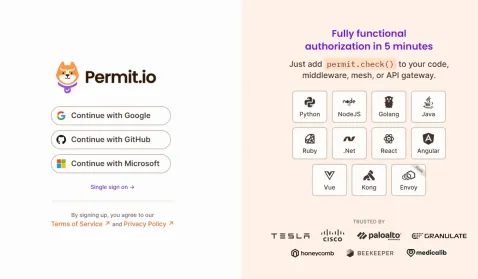
일상적인 기술 도구를 사용하여 기능적 다중 테넌트 SaaS 응용 프로그램 (Edtech 앱)을 구축했으며 동일한 작업을 수행 할 수 있습니다. 먼저, 다중 테넌트 SaaS 응용 프로그램은 무엇입니까? 멀티 테넌트 SAAS 응용 프로그램은 노래에서 여러 고객에게 서비스를 제공 할 수 있습니다.
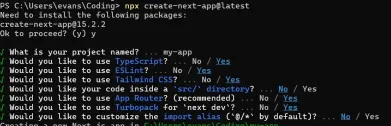
이 기사에서는 Contrim에 의해 확보 된 백엔드와의 프론트 엔드 통합을 보여 주며 Next.js를 사용하여 기능적인 Edtech SaaS 응용 프로그램을 구축합니다. Frontend는 UI 가시성을 제어하기 위해 사용자 권한을 가져오고 API가 역할 기반을 준수하도록합니다.
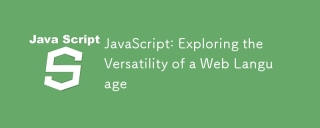
JavaScript는 현대 웹 개발의 핵심 언어이며 다양성과 유연성에 널리 사용됩니다. 1) 프론트 엔드 개발 : DOM 운영 및 최신 프레임 워크 (예 : React, Vue.js, Angular)를 통해 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축합니다. 2) 서버 측 개발 : Node.js는 비 차단 I/O 모델을 사용하여 높은 동시성 및 실시간 응용 프로그램을 처리합니다. 3) 모바일 및 데스크탑 애플리케이션 개발 : 크로스 플랫폼 개발은 개발 효율을 향상시키기 위해 반응 및 전자를 통해 실현됩니다.
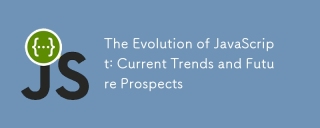
JavaScript의 최신 트렌드에는 Typescript의 Rise, 현대 프레임 워크 및 라이브러리의 인기 및 WebAssembly의 적용이 포함됩니다. 향후 전망은보다 강력한 유형 시스템, 서버 측 JavaScript 개발, 인공 지능 및 기계 학습의 확장, IoT 및 Edge 컴퓨팅의 잠재력을 포함합니다.
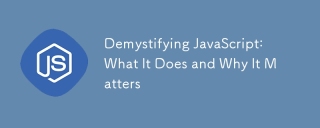
JavaScript는 현대 웹 개발의 초석이며 주요 기능에는 이벤트 중심 프로그래밍, 동적 컨텐츠 생성 및 비동기 프로그래밍이 포함됩니다. 1) 이벤트 중심 프로그래밍을 사용하면 사용자 작업에 따라 웹 페이지가 동적으로 변경 될 수 있습니다. 2) 동적 컨텐츠 생성을 사용하면 조건에 따라 페이지 컨텐츠를 조정할 수 있습니다. 3) 비동기 프로그래밍은 사용자 인터페이스가 차단되지 않도록합니다. JavaScript는 웹 상호 작용, 단일 페이지 응용 프로그램 및 서버 측 개발에 널리 사용되며 사용자 경험 및 크로스 플랫폼 개발의 유연성을 크게 향상시킵니다.
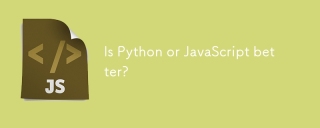
Python은 데이터 과학 및 기계 학습에 더 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 더 적합합니다. 1. Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명하며 데이터 분석 및 웹 개발에 적합합니다. 2. JavaScript는 프론트 엔드 개발의 핵심입니다. Node.js는 서버 측 프로그래밍을 지원하며 풀 스택 개발에 적합합니다.
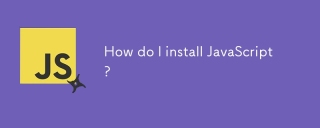
JavaScript는 이미 최신 브라우저에 내장되어 있기 때문에 설치가 필요하지 않습니다. 시작하려면 텍스트 편집기와 브라우저 만 있으면됩니다. 1) 브라우저 환경에서 태그를 통해 HTML 파일을 포함하여 실행하십시오. 2) Node.js 환경에서 Node.js를 다운로드하고 설치 한 후 명령 줄을 통해 JavaScript 파일을 실행하십시오.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기

DVWA
DVWA(Damn Vulnerable Web App)는 매우 취약한 PHP/MySQL 웹 애플리케이션입니다. 주요 목표는 보안 전문가가 법적 환경에서 자신의 기술과 도구를 테스트하고, 웹 개발자가 웹 응용 프로그램 보안 프로세스를 더 잘 이해할 수 있도록 돕고, 교사/학생이 교실 환경 웹 응용 프로그램에서 가르치고 배울 수 있도록 돕는 것입니다. 보안. DVWA의 목표는 다양한 난이도의 간단하고 간단한 인터페이스를 통해 가장 일반적인 웹 취약점 중 일부를 연습하는 것입니다. 이 소프트웨어는
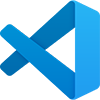
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기
