현재 스레드에 할당된 작업이 완료될 때까지 다른 스레드의 방해 없이 한 번에 하나의 스레드에서만 리소스를 사용할 수 있는 기술을 C#에서는 동기화라고 합니다. 실제로 멀티스레딩 프로그램에서는 필요한 시간 동안 스레드가 모든 리소스에 액세스할 수 있으며 리소스는 스레드에 의해 비동기적으로 공유 및 실행됩니다. 이는 중요한 작업이며 시스템이 중지될 수 있으므로 스레드는 동기적으로 실행되어야 합니다. 그리고 스레드의 동기화를 통해 스레드의 일관성을 유지하고 하나의 스레드가 실행되는 동안 다른 스레드가 간섭하지 않도록 할 수 있습니다.
C# 스레드 동기화 구문
C#Thread 동기화 구문은 다음과 같습니다.
Thread thread_name = new Thread(method_name); thread_name.Start(); thread_name.Join();
또는
Thread thread_name = new Thread(method_name); thread_name.Start(); method_name() { lock(this) { //thread_name thread is executed } }
여기서 thread_name은 스레드의 이름이고 method_name은 thread_name.Start()가 호출된 시점부터 이 스레드가 단독으로 액세스하는 메서드의 이름이며, thread_name.Join()은 다음에 의해 이 스레드가 완료될 때까지 기다립니다. 다른 모든 스레드의 실행을 중지합니다.
메서드 내의 잠금 키워드인 method_name은 현재 스레드가 완료될 때까지 다른 스레드가 해당 메서드에 액세스할 수 없도록 스레드 실행을 잠급니다.
C# 스레드 동기화 기능
- 멀티스레딩 프로그램에서는 필요한 시간 동안 스레드가 모든 리소스에 액세스할 수 있지만 여러 스레드가 동일한 리소스에 액세스하려고 하면 여러 스레드가 동시에 또는 비동기적으로 리소스를 공유하는 것이 중요한 작업이 되며 시스템 실행이 중단될 수 있습니다.
- 이 문제를 극복하려면 스레드 동기화가 필요합니다. 스레드를 동기화하면 다른 스레드의 방해 없이 특정 스레드만 일정 시간 동안 리소스에 액세스할 수 있습니다.
- 스레드의 동기화는 Join 키워드와 Lock 키워드를 사용하여 수행할 수 있습니다.
- 스레드에 Join 키워드를 사용하면 다른 스레드의 방해 없이 해당 스레드가 실행을 완료할 수 있습니다.
- lock 키워드를 사용하면 스레드가 실행을 완료할 때까지 해당 스레드가 실행 중인 리소스가 잠시 잠겨 있습니다.
C# 스레드 동기화 구현 예
다음은 C# 스레드 동기화의 예입니다.
예시 #1
join 키워드를 사용한 스레드 동기화를 보여주는 C# 프로그램
코드:
using System; using System.Threading; //a namespace called program is created namespace program { //a class called check is defined class check { //main method is called static void Main(string[] args) { //an instance of the thread class is created which operates on a method Thread firstthread = new Thread(secondfunction); //start method is used to begin the execution of the thread firstthread.Start(); //join method stops all other threads while the current thread is executing firstthread.Join(); Thread secondthread = new Thread(firstfunction); secondthread.Start(); secondthread.Join(); } private static void firstfunction(object obj) { for(inti=1;i <p><strong>출력:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172534835714207.png?x-oss-process=image/resize,p_40" class="lazy" alt="C# 스레드 동기화" ></p> <p><strong>설명:</strong> 위 프로그램에서는 program이라는 네임스페이스가 생성됩니다. 그런 다음 메인 메소드가 호출되는 check라는 클래스가 정의됩니다. 그런 다음 메서드에 대해 작동하기 위해 스레드의 인스턴스가 생성됩니다. 이 메서드는 Start() 메서드를 사용하여 시작되고 동일한 스레드에서 Join() 메서드를 사용하여 해당 메서드의 실행이 다른 스레드에 의해 중단되지 않도록 합니다. 따라서 출력은 동기적으로 행에 표시됩니다. 프로그램의 출력은 위의 스냅샷에 표시됩니다.</p> <h4 id="예시">예시 #2</h4> <p>lock 키워드를 사용한 스레드 동기화를 보여주는 C# 프로그램</p> <p><strong>코드: </strong></p> <pre class="brush:php;toolbar:false">using System; using System.Threading; //a class called create is created class create { public void func() { //lock is called on this method lock (this) { for (inti = 1; i <p><strong>출력:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172534835921348.png?x-oss-process=image/resize,p_40" class="lazy" alt="C# 스레드 동기화" ></p> <p><strong>설명:</strong> 위 프로그램에서 create를 호출하는 클래스는 lock 키워드를 사용한 메서드가 정의된 생성됩니다. 즉, 이 메서드에서 작동하는 스레드는 다음이 될 때까지 자체적으로 메서드를 잠급니다. 다른 스레드가 메서드에 액세스하는 것을 허용하지 않고 실행을 완료합니다. 이렇게 하면 스레드가 동기적으로 실행됩니다. 프로그램의 출력은 위의 스냅샷에 표시됩니다.</p> <h3 id="결론">결론</h3> <p>이 튜토리얼에서는 프로그래밍 예제와 그 출력을 통해 스레드 동기화의 정의, 구문, 작동을 통해 C#의 ThreadSynchronization 개념을 이해합니다.</p> <h3 id="추천기사">추천기사</h3> <p>C# 스레드 동기화에 대한 안내입니다. 여기에서는 C# 스레드 동기화 소개와 그 작업, 예제 및 코드 구현에 대해 설명합니다. 더 자세히 알아보려면 다른 추천 기사를 살펴보세요. –</p> <ol> <li>C#의 난수 생성기</li> <li>Java의 정적 생성자 </li> <li>C#의 TextWriter </li> <li>C#의 정적 생성자</li> </ol>
위 내용은 C# 스레드 동기화의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
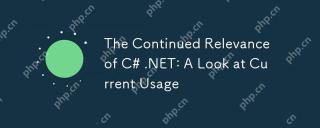
C#.NET은 여러 응용 프로그램 개발을 지원하는 강력한 도구 및 라이브러리를 제공하기 때문에 여전히 중요합니다. 1) C#은 .NET 프레임 워크를 결합하여 개발 효율적이고 편리하게 만듭니다. 2) C#의 타입 안전 및 쓰레기 수집 메커니즘은 장점을 향상시킵니다. 3) .NET은 크로스 플랫폼 실행 환경과 풍부한 API를 제공하여 개발 유연성을 향상시킵니다.
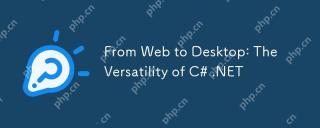
C#.NETISVERSATILEFORBOTHWEBBANDDESKTOPDEVENTROMMENT.1) FORWEB, useASP.NETFORRICHINTERFACES.3) FORDESKTOP.3) USEXAMARINFORCROSS-PLATFORMDEEVENTRIMMENT, LINABILEDEV, MACODEDEV, and MACODEDOWS, 및 MACODEDOWS.
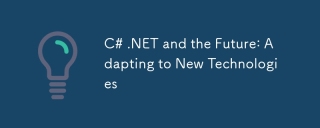
C# 및 .NET는 지속적인 업데이트 및 최적화를 통해 신흥 기술의 요구에 적응합니다. 1) C# 9.0 및 .NET5는 레코드 유형 및 성능 최적화를 소개합니다. 2) .NETCORE는 클라우드 네이티브 및 컨테이너화 된 지원을 향상시킵니다. 3) ASP.NETCORE는 최신 웹 기술과 통합됩니다. 4) ML.NET는 기계 학습 및 인공 지능을 지원합니다. 5) 비동기 프로그래밍 및 모범 사례는 성능을 향상시킵니다.
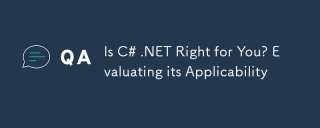
C#.netissuitable forenterprise-levelapplications는 richlibraries, androbustperformance, 그러나 itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical, wherelanguagesslikerustorthightordogrordogrognegrognegrognegrognecross-platformdevelopmentor.
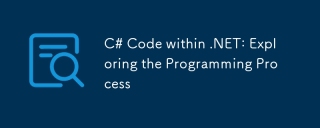
.NET에서 C#의 프로그래밍 프로세스에는 다음 단계가 포함됩니다. 1) C# 코드 작성, 2) 중간 언어 (IL)로 컴파일하고 .NET 런타임 (CLR)에 의해 실행됩니다. .NET에서 C#의 장점은 현대적인 구문, 강력한 유형 시스템 및 .NET 프레임 워크와의 긴밀한 통합으로 데스크탑 응용 프로그램에서 웹 서비스에 이르기까지 다양한 개발 시나리오에 적합합니다.
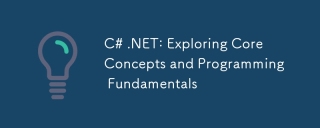
C#은 Microsoft가 개발 한 최신 객체 지향 프로그래밍 언어이며 .NET 프레임 워크의 일부로 개발되었습니다. 1.C#은 캡슐화, 상속 및 다형성을 포함한 객체 지향 프로그래밍 (OOP)을 지원합니다. 2. C#의 비동기 프로그래밍은 응용 프로그램 응답 성을 향상시키기 위해 비동기 및 키워드를 기다리는 키워드를 통해 구현됩니다. 3. LINQ를 사용하여 데이터 컬렉션을 간결하게 처리하십시오. 4. 일반적인 오류에는 NULL 참조 예외 및 인덱스 외 예외가 포함됩니다. 디버깅 기술에는 디버거 사용 및 예외 처리가 포함됩니다. 5. 성능 최적화에는 StringBuilder 사용 및 불필요한 포장 및 Unboxing을 피하는 것이 포함됩니다.
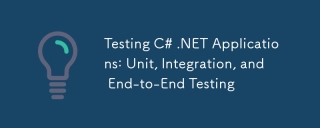
C#.NET 애플리케이션에 대한 테스트 전략에는 단위 테스트, 통합 테스트 및 엔드 투 엔드 테스트가 포함됩니다. 1. 단위 테스트를 통해 MSTEST, NUNIT 또는 XUNIT 프레임 워크를 사용하여 코드의 최소 단위가 독립적으로 작동합니다. 2. 통합 테스트는 일반적으로 사용되는 시뮬레이션 된 데이터 및 외부 서비스를 결합한 여러 장치의 기능을 확인합니다. 3. 엔드 투 엔드 테스트는 사용자의 완전한 작동 프로세스를 시뮬레이션하며 셀레늄은 일반적으로 자동 테스트에 사용됩니다.
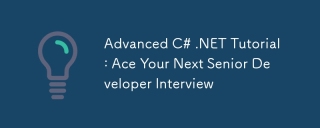
C# 수석 개발자와의 인터뷰에는 비동기 프로그래밍, LINQ 및 .NET 프레임 워크의 내부 작업 원리와 같은 핵심 지식을 마스터하는 것이 필요합니다. 1. 비동기 프로그래밍은 비동기를 통해 작업을 단순화하고 응용 프로그램 응답 성을 향상시키기 위해 기다리고 있습니다. 2.linq는 SQL 스타일로 데이터를 운영하고 성능에주의를 기울입니다. 3. Net Framework의 CLR은 메모리를 관리하며 가비지 컬렉션은주의해서 사용해야합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

SublimeText3 영어 버전
권장 사항: Win 버전, 코드 프롬프트 지원!

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기
