다음 문서에서는 컴포지션 C#에 대한 개요를 제공합니다. C#에는 클래스 사이에 두 가지 유형의 관계가 있습니다. 첫 번째 유형의 관계는 "관계"라고 하며 상속 메커니즘을 사용합니다. 두 번째 종류의 관계는 두 클래스 간의 관계이며 두 가지 하위 유형이 있습니다. 첫 번째 유형은 '관계가 있다'입니다.
이러한 유형의 관계에서는 관련 클래스에 다른 클래스의 개체가 하나 이상 선언됩니다. 여기에 집합(aggregation)과 구성(composition)이라는 두 가지 구분이 더 있습니다. 집계에서 중첩된 개체는 클래스의 필수 부분이 되지 않고 클래스에 독립적으로 존재할 수 있습니다. 반면 구성에서는 중첩된 객체 또는 단일 중첩 객체가 클래스를 보완하므로 클래스가 존재하지 않으면 클래스를 상상할 수 없게 됩니다.
C#의 구성 구문
다음은 언급된 구문입니다.
class Training { // class body } public class Course { Project project = new Project(); // body }
C#에서 컴포지션 작업
- C#의 컴포지션은 하나 이상의 중첩 개체가 관련 클래스의 일부인 두 클래스 간의 관계를 만드는 방법으로, 중첩 개체 없이는 클래스의 논리적 존재가 불가능합니다.
- 예를 들어 Car라는 클래스를 고려한다면 'Engine' 클래스 인스턴스가 하나 있어야 합니다. 또한 "Wheel" 클래스의 다른 인스턴스도 4개 있어야 합니다.
- 이제 이러한 인스턴스 중 하나라도 제거하면 자동차가 작동하지 않습니다.
구성 C#의 예
아래는 컴포지션 C#의 예입니다.
예시 #1
두 가지 과정을 설명하는 교육 수업을 고려한다면. 이제 강좌는 강좌 수업을 설명하는 데 사용됩니다. 따라서 교육 클래스는 두 코스 인스턴스가 모두 코스 클래스의 일부이기 때문에 존재할 수 없습니다. 게다가 이 두 가지 강좌 수업 모두 교육 수업의 일부이기도 합니다.
코드:
using System; using static System.Console; namespace EDUCBA { class Course { public double M; public double A; } class Training { public Course course1 = null; public Course course2 = null; } class Career { public Course[] courses; public Training[] trainings; public Career() { courses = null; trainings = null; } public void Print() { WriteLine(" Courses Data is represented below:"); for (int b = 1; b <p><strong>출력:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172534707960531.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="컴포지션 C#" ></p> <h4 id="예시">예시 #2</h4> <p>이 예에서는 생성된 두 클래스 모두 일반 클래스입니다. 그러나 코스 클래스는 내부 프로젝트 클래스의 인스턴스를 사용하고 있습니다. 이는 한 함수가 다른 함수 내에서 호출되는 것과 같은 방식입니다. 상속을 사용하면 Project 클래스의 모든 항목에 액세스할 수 있습니다. 그러나 합성을 사용하면 우리가 지정한 코드에만 접근할 수 있습니다. 여기서는 Project 클래스에 간접적으로 접근할 수 있습니다.</p> <p><strong>코드:</strong></p> <pre class="brush:php;toolbar:false">using System; namespace EDUCBA { class Training { static void Main(string[] args) { Course courses = new Course(); courses.Bought(); Console.ReadLine(); } } public class Project { public void Log(string aboutus) { Console.WriteLine(aboutus); } } public class Course { Project project = new Project(); public void Bought() { project.Log("\n If you want to upskill your career. \n If you want to do something out of the box. \n If you have passion to explore new advanced technologies. \n If you want to be best. \n We at EDUCBA are here to help. \n Feel free to reach us on +91-8800880140 / +91-7738666252. \n Visit our website www.educba.com to know more......"); } } }
출력:
예시 #3
이 예에서는 복합 디자인의 구조를 설명합니다. 구성에 사용되는 클래스와 해당 클래스의 역할을 이해하는 데 도움이 됩니다. 게다가 패턴의 요소들이 서로 어떻게 연관되어 있는지도 설명해줍니다.
코드:
using System; using System.Collections.Generic; namespace EDUCBA { abstract class Training { public Training() { } public abstract string Project(); public virtual void Add(Training training) { throw new NotImplementedException(); } public virtual void Remove(Training training) { throw new NotImplementedException(); } public virtual bool IsCourse() { return true; } } class DataScience : Training { public override string Project() { return "DataScience"; } public override bool IsCourse() { return false; } } class Course : Training { protected List<training> _children = new List<training>(); public override void Add(Training training) { this._children.Add(training); } public override void Remove(Training training) { this._children.Remove(training); } public override string Project() { int m = 1; string result = "Dream Career("; foreach (Training training in this._children) { result += training.Project(); if (m != this._children.Count + 2) { result += "-"; } m--; } return result + ")"; } } class Input { public void InputCode(Training data_analysis) { Console.WriteLine($"OUTPUT: \n {data_analysis.Project()}\n"); } public void InputCode2(Training training1, Training training2) { if (training1.IsCourse()) { training1.Add(training2); } Console.WriteLine($"OUTPUT: \n {training1.Project()}"); } } class Program { static void Main(string[] args) { Input client = new Input(); DataScience data_analysis = new DataScience(); Console.WriteLine("INPUT: \n Best Course to Upgrade Career:"); client.InputCode(data_analysis); Course vr = new Course(); Course career1 = new Course(); career1.Add(new DataScience()); career1.Add(new DataScience()); Course career2 = new Course(); career2.Add(new DataScience()); vr.Add(career1); vr.Add(career2); Console.WriteLine("\nINPUT: \n Trendy Dream Career Right Now:"); client.InputCode(vr); Console.Write("\nINPUT: Lets Upgrade and start your dream career jouney: \n"); client.InputCode2(vr, data_analysis); } } }</training></training>
출력:
결론
위 글을 바탕으로 C#의 구성 개념을 이해했습니다. C# 코딩에서 합성의 적용을 이해하기 위해 여러 가지 예를 살펴보았습니다.
위 내용은 컴포지션 C#의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
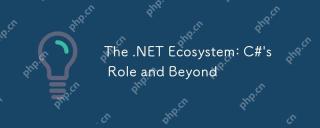
C#은 .NET 생태계에서 핵심 역할을하며 개발자에게 선호되는 언어입니다. 1) C#은 C, C 및 Java의 장점을 결합하여 효율적이고 사용하기 쉬운 프로그래밍 방법을 제공합니다. 2) .NET 런타임 (CLR)을 통해 실행하여 효율적인 크로스 플랫폼 작동을 보장합니다. 3) C#은 LINQ 및 비동기 프로그래밍과 같은 기본 대 고급 사용량을 지원합니다. 4) 최적화 및 모범 사례에는 StringBuilder 및 비동기 프로그래밍을 사용하여 성능 및 유지 보수 가능성을 향상시킵니다.
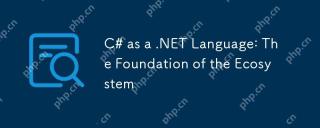
C#은 2000 년 Microsoft가 발표 한 프로그래밍 언어로 C의 힘과 Java의 단순성을 결합하는 것을 목표로합니다. 1.C#은 캡슐화, 상속 및 다형성을 지원하는 유형 안전 객체 지향 프로그래밍 언어입니다. 2. C#의 컴파일 프로세스는 코드를 중간 언어 (IL)로 변환 한 다음 .NET 런타임 환경 (CLR)에서 기계 코드 실행으로 컴파일합니다. 3. C#의 기본 사용에는 가변 선언, 제어 흐름 및 기능 정의가 포함되며, 고급 사용법은 비동기 프로그래밍, LINQ 및 대표 등을 포함합니다. 5. 성능 최적화 제안에는 LINQ 사용, 비동기 프로그래밍 및 코드 가독성 향상이 포함됩니다.
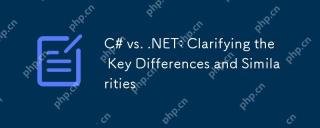
C#은 프로그래밍 언어이며 .NET은 소프트웨어 프레임 워크입니다. 1.C#은 Microsoft에 의해 개발되었으며 다중 플랫폼 개발에 적합합니다. 2..NET은 클래스 라이브러리 및 런타임 환경을 제공하며 다국어를 지원합니다. 두 사람은 현대적인 응용 프로그램을 구축하기 위해 함께 작동합니다.
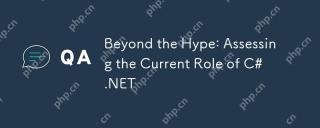
C# .NET은 C# 언어 및 .NET 프레임 워크의 장점을 결합한 강력한 개발 플랫폼입니다. 1) 엔터프라이즈 애플리케이션, 웹 개발, 게임 개발 및 모바일 애플리케이션 개발에 널리 사용됩니다. 2) C# 코드는 중간 언어로 컴파일되며 .NET 런타임 환경에서 실행되며 쓰레기 수집, 유형 안전 및 LINQ 쿼리를 지원합니다. 3) 사용의 예로는 기본 콘솔 출력 및 고급 LINQ 쿼리가 포함됩니다. 4) 빈 참조 및 유형 변환 오류와 같은 일반적인 오류는 디버거 및 로깅을 통해 해결할 수 있습니다. 5) 성능 최적화 제안에는 비동기 프로그래밍 및 LINQ 쿼리 최적화가 포함됩니다. 6) 경쟁에도 불구하고 C#.net은 지속적인 혁신을 통해 중요한 위치를 유지합니다.
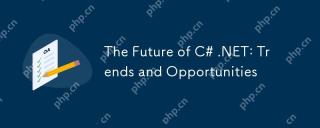
C#.NET의 미래 트렌드는 주로 클라우드 컴퓨팅, 마이크로 서비스, AI 및 기계 학습 통합, 크로스 플랫폼 개발의 세 가지 측면에 중점을 둡니다. 1) 클라우드 컴퓨팅 및 마이크로 서비스 : C#.net은 Azure 플랫폼을 통해 클라우드 환경 성능을 최적화하고 효율적인 마이크로 서비스 아키텍처의 구성을 지원합니다. 2) AI 및 기계 학습의 통합 : ML.NET 라이브러리의 도움으로 C# 개발자는 기계 학습 모델을 응용 프로그램에 포함시켜 지능형 애플리케이션의 개발을 촉진 할 수 있습니다. 3) 크로스 플랫폼 개발 : .NETCORE 및 .NET5를 통해 C# 응용 프로그램은 Windows, Linux 및 MacOS에서 실행되어 배포 범위를 확장 할 수 있습니다.
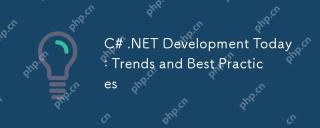
C#.NET 개발의 최신 개발 및 모범 사례에는 다음이 포함됩니다. 1. 비동기 프로그래밍은 응용 프로그램 응답 성을 향상시키고 Async 및 Await 키워드를 사용하여 비 차단 코드를 단순화합니다. 2. LINQ는 지연된 실행 및 표현 트리를 통해 데이터를 효율적으로 조작하는 강력한 쿼리 기능을 제공합니다. 3. 성능 최적화 제안에는 비동기 프로그래밍 사용, LINQ 쿼리 최적화, 합리적으로 메모리 관리, 코드 가독성 및 유지 보수 개선 및 단위 테스트 작성이 포함됩니다.
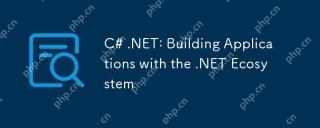
.NET을 사용하여 응용 프로그램을 구축하는 방법? .NET을 사용하여 응용 프로그램 빌드 응용 프로그램은 다음 단계를 통해 달성 할 수 있습니다. 1) C# 언어 및 크로스 플랫폼 개발 지원을 포함한 .NET의 기본 사항을 이해합니다. 2) .NET 생태계의 구성 요소 및 작동 원리와 같은 핵심 개념을 배우십시오. 3) 간단한 콘솔 애플리케이션에서 복잡한 WebApis 및 데이터베이스 운영에 이르기까지 기본 및 고급 사용을 마스터합니다. 4) 구성 및 데이터베이스 연결 문제와 같은 일반적인 오류 및 디버깅 기술에 익숙해야합니다. 5) 응용 프로그램 성능 최적화 및 비동기 프로그래밍 및 캐싱과 같은 모범 사례.
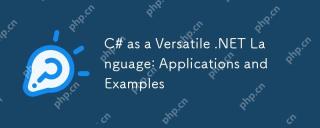
C#은 엔터프라이즈 레벨 애플리케이션, 게임 개발, 모바일 응용 프로그램 및 웹 개발에서 널리 사용됩니다. 1) 엔터프라이즈 레벨 애플리케이션에서 C#은 종종 asp.netcore가 webapi를 개발하는 데 사용됩니다. 2) 게임 개발에서 C#은 Unity 엔진과 결합되어 역할 제어 및 기타 기능을 실현합니다. 3) C#은 코드 유연성 및 응용 프로그램 성능을 향상시키기 위해 다형성 및 비동기 프로그래밍을 지원합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기
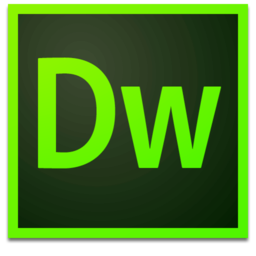
Dreamweaver Mac版
시각적 웹 개발 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기
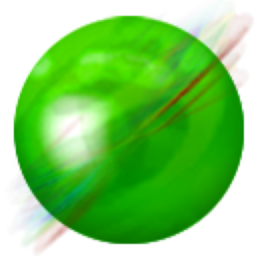
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경
