배열 순회는 모든 개발자가 숙지해야 하는 데이터 구조 및 알고리즘(DSA)의 기본 개념입니다. 이 포괄적인 가이드에서는 기본 접근 방식부터 시작하여 고급 방법까지 진행하면서 JavaScript에서 배열을 탐색하는 다양한 기술을 살펴보겠습니다. 쉬운 수준부터 고급 수준까지 20가지 예시를 다루며, 학습을 강화하기 위한 LeetCode 스타일의 질문도 포함합니다.
목차
- 배열 순회 소개
-
기본 배열 순회
- 예 1: for 루프 사용
- 예 2: while 루프 사용
- 예 3: do-while 루프 사용
- 예 4: 역순회
-
최신 JavaScript 배열 방법
- 예 5: forEach 메소드
- 예 6: 지도 방법
- 예 7: 필터 방법
- 예 8: 축소 방법
-
중간 순회 기술
- 예 9: 투 포인터 기술
- 예시 10: 슬라이딩 윈도우
- 예 11: Kadane의 알고리즘
- 예 12: 네덜란드 국기 알고리즘
-
고급 순회 기술
- 예 13: 재귀 순회
- 예 14: 정렬된 배열의 이진 검색
- 예 15: 두 개의 정렬된 배열 병합
- 예 16: 빠른 선택 알고리즘
-
전문 순회
- 예 17: 2D 배열 탐색
- 예 18: 나선형 행렬 탐색
- 예 19: 대각선 순회
- 예 20: 지그재그 탐색
- 성능 고려 사항
- LeetCode 실습 문제
- 결론
1. 배열 순회 소개
배열 순회는 일부 작업을 수행하기 위해 배열의 각 요소를 방문하는 프로세스입니다. 이는 프로그래밍에서 중요한 기술로, 많은 알고리즘과 데이터 조작의 기초를 형성합니다. JavaScript에서 배열은 데이터를 탐색하고 조작하는 다양한 방법을 제공하는 다목적 데이터 구조입니다.
2. 기본 배열 순회
배열 탐색의 기본 방법부터 시작해 보겠습니다.
예제 1: for 루프 사용
클래식 for 루프는 배열을 순회하는 가장 일반적인 방법 중 하나입니다.
function sumArray(arr) { let sum = 0; for (let i = 0; i <p><strong>시간 복잡도</strong>: O(n), 여기서 n은 배열의 길이입니다.</p> <p></p> <h3> 예제 2: while 루프 사용 </h3> <p> 특히 종료 조건이 더 복잡한 경우 배열 순회에 while 루프를 사용할 수도 있습니다.<br> </p> <pre class="brush:php;toolbar:false">function findFirstNegative(arr) { let i = 0; while (i = 0) { i++; } return i <p><strong>시간 복잡도</strong>: 최악의 경우 O(n)이지만, 음수를 조기에 발견하면 더 작아질 수 있습니다.</p> <p></p> <h3> 예제 3: do-while 루프 사용 </h3> <p>do-while 루프는 배열 순회에서는 덜 일반적이지만 특정 시나리오에서는 유용할 수 있습니다.<br> </p> <pre class="brush:php;toolbar:false">function printReverseUntilZero(arr) { let i = arr.length - 1; do { console.log(arr[i]); i--; } while (i >= 0 && arr[i] !== 0); } const numbers = [1, 3, 0, 5, 7]; printReverseUntilZero(numbers); // Output: 7, 5
시간 복잡도: 최악의 경우 O(n)이지만 초기에 0을 만나면 더 작아질 수 있습니다.
예제 4: 역순회
배열을 역순으로 탐색하는 것은 많은 알고리즘에서 일반적인 작업입니다.
function reverseTraversal(arr) { const result = []; for (let i = arr.length - 1; i >= 0; i--) { result.push(arr[i]); } return result; } const numbers = [1, 2, 3, 4, 5]; console.log(reverseTraversal(numbers)); // Output: [5, 4, 3, 2, 1]
시간 복잡도: O(n), 여기서 n은 배열의 길이입니다.
3. 최신 JavaScript 배열 방법
ES6 및 이후 버전의 JavaScript에는 순회 및 조작을 단순화하는 강력한 배열 방법이 도입되었습니다.
예시 5: forEach 메서드
forEach 메소드는 배열 요소를 반복하는 깔끔한 방법을 제공합니다.
function logEvenNumbers(arr) { arr.forEach(num => { if (num % 2 === 0) { console.log(num); } }); } const numbers = [1, 2, 3, 4, 5, 6]; logEvenNumbers(numbers); // Output: 2, 4, 6
시간 복잡도: O(n), 여기서 n은 배열의 길이입니다.
예시 6: 지도 방법
map 메소드는 모든 요소에 대해 제공된 함수를 호출한 결과로 새 배열을 생성합니다.
function doubleNumbers(arr) { return arr.map(num => num * 2); } const numbers = [1, 2, 3, 4, 5]; console.log(doubleNumbers(numbers)); // Output: [2, 4, 6, 8, 10]
시간 복잡도: O(n), 여기서 n은 배열의 길이입니다.
실시예 7: 필터 방법
필터 메소드는 특정 조건을 통과하는 모든 요소로 새 배열을 생성합니다.
function filterPrimes(arr) { function isPrime(num) { if (num <p><strong>시간 복잡도</strong>: O(n * sqrt(m)), 여기서 n은 배열의 길이이고 m은 배열에서 가장 큰 숫자입니다.</p> <p></p> <h3> 실시예 8: 감소 방법 </h3> <p>리듀스 메소드는 배열의 각 요소에 리듀서 함수를 적용하여 단일 출력값을 생성합니다.<br> </p> <pre class="brush:php;toolbar:false">function findMax(arr) { return arr.reduce((max, current) => Math.max(max, current), arr[0]); } const numbers = [3, 7, 2, 9, 1, 5]; console.log(findMax(numbers)); // Output: 9
시간 복잡도: O(n), 여기서 n은 배열의 길이입니다.
4. 중간 순회 기법
이제 배열 순회를 위한 몇 가지 중간 기술을 살펴보겠습니다.
예시 9: 두 포인터 기술
두 포인터 기법은 배열 관련 문제를 효율적으로 해결하기 위해 자주 사용됩니다.
function isPalindrome(arr) { let left = 0; let right = arr.length - 1; while (left <p><strong>Time Complexity</strong>: O(n/2) which simplifies to O(n), where n is the length of the array.</p> <p></p> <h3> Example 10: Sliding window </h3> <p>The sliding window technique is useful for solving problems involving subarrays or subsequences.<br> </p> <pre class="brush:php;toolbar:false">function maxSubarraySum(arr, k) { if (k > arr.length) return null; let maxSum = 0; let windowSum = 0; // Calculate sum of first window for (let i = 0; i <p><strong>Time Complexity</strong>: O(n), where n is the length of the array.</p> <p></p> <h3> Example 11: Kadane's Algorithm </h3> <p>Kadane's algorithm is used to find the maximum subarray sum in a one-dimensional array.<br> </p> <pre class="brush:php;toolbar:false">function maxSubarraySum(arr) { let maxSoFar = arr[0]; let maxEndingHere = arr[0]; for (let i = 1; i <p><strong>Time Complexity</strong>: O(n), where n is the length of the array.</p> <p></p> <h3> Example 12: Dutch National Flag Algorithm </h3> <p>This algorithm is used to sort an array containing three distinct elements.<br> </p> <pre class="brush:php;toolbar:false">function dutchFlagSort(arr) { let low = 0, mid = 0, high = arr.length - 1; while (mid <p><strong>Time Complexity</strong>: O(n), where n is the length of the array.</p> <p></p> <h2> 5. Advanced Traversal Techniques </h2> <p>Let's explore some more advanced techniques for array traversal.</p> <p></p> <h3> Example 13: Recursive traversal </h3> <p>Recursive traversal can be powerful for certain types of problems, especially those involving nested structures.<br> </p> <pre class="brush:php;toolbar:false">function sumNestedArray(arr) { let sum = 0; for (let element of arr) { if (Array.isArray(element)) { sum += sumNestedArray(element); } else { sum += element; } } return sum; } const nestedNumbers = [1, [2, 3], [[4, 5], 6]]; console.log(sumNestedArray(nestedNumbers)); // Output: 21
Time Complexity: O(n), where n is the total number of elements including nested ones.
Example 14: Binary search on sorted array
Binary search is an efficient algorithm for searching a sorted array.
function binarySearch(arr, target) { let left = 0; let right = arr.length - 1; while (left <p><strong>Time Complexity</strong>: O(log n), where n is the length of the array.</p> <p></p> <h3> Example 15: Merge two sorted arrays </h3> <p>This technique is often used in merge sort and other algorithms.<br> </p> <pre class="brush:php;toolbar:false">function mergeSortedArrays(arr1, arr2) { const mergedArray = []; let i = 0, j = 0; while (i <p><strong>Time Complexity</strong>: O(n + m), where n and m are the lengths of the input arrays.</p> <p></p> <h3> Example 16: Quick Select Algorithm </h3> <p>Quick Select is used to find the kth smallest element in an unsorted array.<br> </p> <pre class="brush:php;toolbar:false">function quickSelect(arr, k) { if (k arr.length) { return null; } function partition(low, high) { const pivot = arr[high]; let i = low - 1; for (let j = low; j k - 1) { return select(low, pivotIndex - 1, k); } else { return select(pivotIndex + 1, high, k); } } return select(0, arr.length - 1, k); } const numbers = [3, 2, 1, 5, 6, 4]; console.log(quickSelect(numbers, 2)); // Output: 2 (2nd smallest element)
Time Complexity: Average case O(n), worst case O(n^2), where n is the length of the array.
6. Specialized Traversals
Some scenarios require specialized traversal techniques, especially when dealing with multi-dimensional arrays.
Example 17: Traversing a 2D array
Traversing 2D arrays (matrices) is a common operation in many algorithms.
function traverse2DArray(matrix) { const result = []; for (let i = 0; i <p><strong>Time Complexity</strong>: O(m * n), where m is the number of rows and n is the number of columns in the matrix.</p> <p></p> <h3> Example 18: Spiral Matrix Traversal </h3> <p>Spiral traversal is a more complex pattern often used in coding interviews and specific algorithms.<br> </p> <pre class="brush:php;toolbar:false">function spiralTraversal(matrix) { const result = []; if (matrix.length === 0) return result; let top = 0, bottom = matrix.length - 1; let left = 0, right = matrix[0].length - 1; while (top = left; i--) { result.push(matrix[bottom][i]); } bottom--; } if (left = top; i--) { result.push(matrix[i][left]); } left++; } } return result; } const matrix = [ [1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12] ]; console.log(spiralTraversal(matrix)); // Output: [1, 2, 3, 4, 8, 12, 11, 10, 9, 5, 6, 7]
Time Complexity: O(m * n), where m is the number of rows and n is the number of columns in the matrix.
Example 19: Diagonal Traversal
Diagonal traversal of a matrix is another interesting pattern.
function diagonalTraversal(matrix) { const m = matrix.length; const n = matrix[0].length; const result = []; for (let d = 0; d = 0 && j <p><strong>Time Complexity</strong>: O(m * n), where m is the number of rows and n is the number of columns in the matrix.</p> <p></p> <h3> Example 20: Zigzag Traversal </h3> <p>Zigzag traversal is a pattern where we traverse the array in a zigzag manner.<br> </p> <pre class="brush:php;toolbar:false">function zigzagTraversal(matrix) { const m = matrix.length; const n = matrix[0].length; const result = []; let row = 0, col = 0; let goingDown = true; for (let i = 0; i <p><strong>Time Complexity</strong>: O(m * n), where m is the number of rows and n is the number of columns in the matrix.</p> <p></p> <h2> 7. Performance Considerations </h2> <p>When working with array traversals, it's important to consider performance implications:</p> <ol> <li><p><strong>Time Complexity</strong>: Most basic traversals have O(n) time complexity, where n is the number of elements. However, nested loops or recursive calls can increase this to O(n^2) or higher.</p></li> <li><p><strong>Space Complexity</strong>: Methods like map and filter create new arrays, potentially doubling memory usage. In-place algorithms are more memory-efficient.</p></li> <li><p><strong>Iterator Methods vs. For Loops</strong>: Modern methods like forEach, map, and filter are generally slower than traditional for loops but offer cleaner, more readable code.</p></li> <li><p><strong>Early Termination</strong>: for and while loops allow for early termination, which can be more efficient when you're searching for a specific element.</p></li> <li><p><strong>Large Arrays</strong>: For very large arrays, consider using for loops for better performance, especially if you need to break the loop early.</p></li> <li><p><strong>Caching Array Length</strong>: In performance-critical situations, caching the array length in a variable before the loop can provide a slight speed improvement.</p></li> <li><p><strong>Avoiding Array Resizing</strong>: When building an array dynamically, initializing it with a predetermined size (if possible) can improve performance by avoiding multiple array resizing operations.</p></li> </ol> <p></p><h2> 8. LeetCode 실습 문제 </h2> <p>배열 순회 기술에 대한 이해를 더욱 강화하기 위해 연습할 수 있는 15가지 LeetCode 문제가 있습니다.</p> <ol> <li>투썸</li> <li>주식을 사고 파는 가장 좋은 시기</li> <li>중복 내용이 포함되어 있습니다</li> <li>Self를 제외한 배열의 곱</li> <li>최대 하위 배열</li> <li>0 이동</li> <li>3썸</li> <li>물이 가장 많은 용기</li> <li>배열 회전</li> <li>회전 정렬 배열에서 최소값 찾기</li> <li>회전 정렬 배열에서 검색</li> <li>병합 간격</li> <li>나선형 매트릭스</li> <li>행렬 0 설정</li> <li>가장 긴 연속 시퀀스</li> </ol> <p>이러한 문제는 광범위한 배열 순회 기술을 다루며 이 블로그 게시물에서 논의한 개념을 적용하는 데 도움이 됩니다.</p> <p></p> <h2> 9. 결론 </h2> <p>배열 순회는 많은 알고리즘과 데이터 조작의 기초를 형성하는 프로그래밍의 기본 기술입니다. 기본 for 루프부터 슬라이딩 윈도우 및 특수 행렬 탐색과 같은 고급 기술에 이르기까지 이러한 방법을 익히면 복잡한 문제를 효율적으로 해결하는 능력이 크게 향상됩니다.</p> <p>이 20가지 예를 통해 살펴본 것처럼 JavaScript는 배열 순회를 위한 풍부한 도구 세트를 제공하며 각 도구에는 고유한 장점과 사용 사례가 있습니다. 각 기술을 적용하는 시기와 방법을 이해하면 다양한 프로그래밍 문제를 처리할 수 있는 준비를 갖추게 됩니다.</p> <p>숙련의 열쇠는 연습이라는 점을 기억하세요. 자신의 프로젝트에서 이러한 순회 방법을 구현해 보고, 기본 사항에 익숙해지면 주저하지 말고 고급 기술을 탐색해 보세요. 제공된 LeetCode 문제는 이러한 개념을 다양한 시나리오에 적용할 수 있는 충분한 기회를 제공합니다.</p> <p>계속 기술을 개발하면서 선택한 순회 방법이 성능에 미치는 영향을 항상 염두에 두세요. 때로는 간단한 for 루프가 가장 효율적인 솔루션일 수도 있지만, 다른 경우에는 슬라이딩 윈도우나 2포인터 방법과 같은 보다 전문적인 기술이 최적일 수 있습니다.</p> <p>즐거운 코딩 되시기 바랍니다. 배열이 항상 효율적으로 탐색되기를 바랍니다!</p>
위 내용은 JavaScript를 사용한 DSA의 배열 탐색: 기본부터 고급 기술까지의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
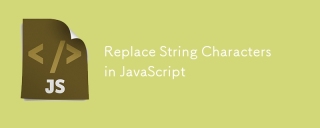
JavaScript 문자열 교체 방법 및 FAQ에 대한 자세한 설명 이 기사는 JavaScript에서 문자열 문자를 대체하는 두 가지 방법 인 내부 JavaScript 코드와 웹 페이지의 내부 HTML을 탐색합니다. JavaScript 코드 내부의 문자열을 교체하십시오 가장 직접적인 방법은 대체 () 메소드를 사용하는 것입니다. str = str.replace ( "find", "replace"); 이 메소드는 첫 번째 일치 만 대체합니다. 모든 경기를 교체하려면 정규 표현식을 사용하고 전역 플래그 g를 추가하십시오. str = str.replace (/fi
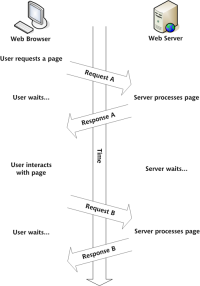
그래서 여기 당신은 Ajax라는이 일에 대해 배울 준비가되어 있습니다. 그러나 정확히 무엇입니까? Ajax라는 용어는 역동적이고 대화식 웹 컨텐츠를 만드는 데 사용되는 느슨한 기술 그룹을 나타냅니다. 원래 Jesse J에 의해 만들어진 Ajax라는 용어
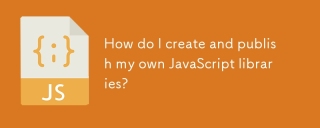
기사는 JavaScript 라이브러리 작성, 게시 및 유지 관리, 계획, 개발, 테스트, 문서 및 홍보 전략에 중점을 둡니다.

이 기사는 브라우저에서 JavaScript 성능을 최적화하기위한 전략에 대해 설명하고 실행 시간을 줄이고 페이지로드 속도에 미치는 영향을 최소화하는 데 중점을 둡니다.
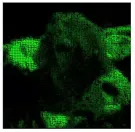
매트릭스 영화 효과를 페이지에 가져 오십시오! 이것은 유명한 영화 "The Matrix"를 기반으로 한 멋진 jQuery 플러그인입니다. 플러그인은 영화에서 클래식 그린 캐릭터 효과를 시뮬레이션하고 사진을 선택하면 플러그인이 숫자로 채워진 매트릭스 스타일 사진으로 변환합니다. 와서 시도해보세요. 매우 흥미 롭습니다! 작동 방식 플러그인은 이미지를 캔버스에로드하고 픽셀 및 색상 값을 읽습니다. data = ctx.getImageData (x, y, settings.grainsize, settings.grainsize) .data 플러그인은 그림의 직사각형 영역을 영리하게 읽고 jQuery를 사용하여 각 영역의 평균 색상을 계산합니다. 그런 다음 사용하십시오
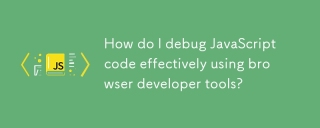
이 기사는 브라우저 개발자 도구를 사용하여 효과적인 JavaScript 디버깅, 중단 점 설정, 콘솔 사용 및 성능 분석에 중점을 둡니다.
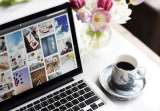
이 기사에서는 jQuery 라이브러리를 사용하여 간단한 사진 회전 목마를 만들도록 안내합니다. jQuery를 기반으로 구축 된 BXSLIDER 라이브러리를 사용하고 회전 목마를 설정하기위한 많은 구성 옵션을 제공합니다. 요즘 그림 회전 목마는 웹 사이트에서 필수 기능이되었습니다. 한 사진은 천 단어보다 낫습니다! 그림 회전 목마를 사용하기로 결정한 후 다음 질문은 그것을 만드는 방법입니다. 먼저 고품질 고해상도 사진을 수집해야합니다. 다음으로 HTML과 일부 JavaScript 코드를 사용하여 사진 회전 목마를 만들어야합니다. 웹에는 다양한 방식으로 회전 목마를 만드는 데 도움이되는 라이브러리가 많이 있습니다. 오픈 소스 BXSLIDER 라이브러리를 사용할 것입니다. BXSLIDER 라이브러리는 반응 형 디자인을 지원 하므로이 라이브러리로 제작 된 회전 목마는
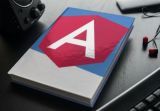
데이터 세트는 API 모델 및 다양한 비즈니스 프로세스를 구축하는 데 매우 필수적입니다. 그렇기 때문에 CSV 가져 오기 및 내보내기가 자주 필요한 기능인 이유입니다.이 자습서에서는 각도 내에서 CSV 파일을 다운로드하고 가져 오는 방법을 배웁니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구
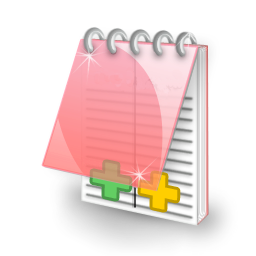
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

맨티스BT
Mantis는 제품 결함 추적을 돕기 위해 설계된 배포하기 쉬운 웹 기반 결함 추적 도구입니다. PHP, MySQL 및 웹 서버가 필요합니다. 데모 및 호스팅 서비스를 확인해 보세요.

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.
