Java의 직렬화는 객체의 상태를 바이트 스트림으로 변환하는 메커니즘입니다. 역직렬화는 반대 프로세스입니다. 역직렬화를 통해 실제 Java 객체가 바이트 스트림의 메모리에 생성됩니다. 이러한 메커니즘은 개체에 지속됩니다. 이렇게 직렬화를 통해 생성된 바이트 스트림은 어떤 플랫폼에도 의존하지 않습니다. 다른 플랫폼에서는 문제 없이 한 플랫폼에서 직렬화된 객체를 역직렬화할 수 있습니다.
무료 소프트웨어 개발 과정 시작
웹 개발, 프로그래밍 언어, 소프트웨어 테스팅 등
따라서 직렬화 및 역직렬화의 전체 프로세스는 JVM에 독립적입니다. 클래스 객체를 직렬화하려면 java.io.Serialized 인터페이스를 구현해야 합니다. Java에서 직렬화 가능한 것은 마커 인터페이스입니다. 구현할 필드나 메서드가 없습니다. 이 프로세스는 옵트인(Opt-In) 프로세스와 유사하게 클래스를 직렬화할 수 있게 만듭니다.
Java의 직렬화는 ObjectInputStream과 ObjectOutputStream 두 클래스에 의해 구현됩니다. 필요한 것은 파일에 저장하거나 네트워크를 통해 전송할 수 있도록 래퍼를 두는 것뿐입니다.
Java의 직렬화 개념
위 섹션에서 언급한 직렬화 클래스인 ObjectOutputStream 클래스에는 다양한 데이터 유형을 작성하기 위한 여러 작성 메소드가 포함되어 있지만 가장 널리 사용되는 메소드는 하나입니다.
public final void writeObject( Object x ) throws IOException
위 방법을 사용하여 객체를 직렬화할 수 있습니다. 이 메서드는 또한 이를 출력 스트림으로 보냅니다. 마찬가지로 ObjectInputStream 클래스에는 객체 역직렬화를 위한 메소드가 포함되어 있습니다.
public final Object readObject() throws IOException, ClassNotFoundException
역직렬화 방법은 스트림에서 객체를 검색하고 이를 역직렬화합니다. 반환 값은 다시 객체이므로 관련 데이터 유형으로 캐스팅하기만 하면 됩니다.
클래스의 성공적인 연재를 위해서는 두 가지 조건이 충족되어야 합니다.
- 아이오. 클래스는 직렬화 가능한 인터페이스를 구현해야 합니다.
- 클래스의 모든 필드는 직렬화 가능해야 합니다. 하나의 필드라도 직렬화할 수 없으면 임시로 표시해야 합니다.
클래스가 직렬화 가능한지 확인해야 하는 경우 간단한 해결책은 클래스가 java.io.Serialized 메서드를 구현하는지 확인하는 것입니다. 그렇다면 직렬화 가능합니다. 그렇지 않다면 그렇지 않습니다. 객체를 파일로 직렬화할 때 표준 관행은 파일에 .ser 확장자를 부여하는 것입니다.
방법
클래스에 이러한 메소드가 포함되어 있으면 Java에서 직렬화에 사용됩니다.
1. Java의 직렬화 방법
Method | Description |
public final void writeObject (Object obj) throws IOException {} | This will write the specified object to the ObjectOutputStream. |
public void flush() throws IOException {} | This will flush the current output stream. |
public void close() throws IOException {} | This will close the current output stream. |
2. Java의 역직렬화 방법
Method | Description |
public final Object readObject() throws IOException, ClassNotFoundException{} | This will read an object from the input stream. |
public void close() throws IOException {} | This will close ObjectInputStream. |
Example of Serialization in Java
An example in Java is provided here to demonstrate how serialization works in Java. We created an Employee class to study some features, and the code is provided below. This employee class implements the Serializable interface.
public class Employee implements java.io.Serializable { public String name; public String address; public transient int SSN; public int number; public void mailCheck() { System.out.println("Mailing a letter to " + name + " " + address); } }
When this program finishes executing, it will create a file named employee.ser. This program does not provide a guaranteed output, rather it is for explanatory purposes only, and the objective is to understand its use and to work.
import java.io.*; public class SerializeDemo { public static void main(String [] args) { Employee e = new Employee(); e.name = "Rahul Jain"; e.address = "epip, Bangalore"; e.SSN = 114433; e.number = 131; try { FileOutputStream fileOut = new FileOutputStream("https://cdn.educba.com/tmp/employee.ser"); ObjectOutputStream out = new ObjectOutputStream(fileOut); out.writeObject(e); out.close(); fileOut.close(); System.out.printf("Serialized data saved in /tmp/employee.ser"); } catch (IOException i) { i.printStackTrace(); } } }
The below-described DeserializeDemo program deserializes the above Employee object created in the Serialize Demo program.
import java.io.*; public class DeserializeDemo { public static void main(String [] args) { Employee e = null; try { FileInputStream fileIn = new FileInputStream("https://cdn.educba.com/tmp/employee.ser"); ObjectInputStream in = new ObjectInputStream(fileIn); e = (Employee) in.readObject(); in.close(); fileIn.close(); } catch (IOException i) { i.printStackTrace(); return; } catch (ClassNotFoundException c) { System.out.println("Employee class is not found"); c.printStackTrace(); return; } System.out.println("Deserialized Employee..."); System.out.println("Name: " + e.name); System.out.println("Address: " + e.address); System.out.println("SSN: " + e.SSN); System.out.println("Number: " + e.number); } }
Output:
Deserialized Employee…
Name: Rahul Jain
Address: epip, Bangalore
SSN: 0
Number:131
Some important points related to the program above are provided below:
- The try/catch block above tries to catch a ClassNotFoundException. This is declared by the readObject() method.
- A JVM can deserialize an object only if it finds the bytecode for the class.
- If the JVM does not find a class during the deserialization, it will throw ClassNotFoundException.
- The readObject () return value is always cast to an Employee reference.
- When the object was serialized, the SSN field had an initial value of 114433, which was not sent to the output stream. Because of the same, the deserialized Employee SSN field object is 0.
Conclusion
Above, we introduced serialization concepts and provided examples. Let’s understand the need for serialization in our concluding remarks.
- Communication: If two machines that are running the same code need to communicate, the easy way out is that one machine should build an object containing information that it would transmit and then serialize that object before sending it to the other machine. The method may not be perfect, but it accomplishes the task.
- Persistence: If you want to store the state of an operation in a database, you first serialize it to a byte array and then store the byte array in the database for retrieval later.
- Deep Copy: If creating a replica of an object is challenging and writing a specialized clone class is difficult, then the goal can be achieved by serializing the object and then de-serializing it into another object.
- Cross JVM Synchronization: JVMs running on different machines and architectures can be synchronized.
위 내용은 Java의 직렬화의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

이 기사에서는 Java 프로젝트 관리, 구축 자동화 및 종속성 해상도에 Maven 및 Gradle을 사용하여 접근 방식과 최적화 전략을 비교합니다.
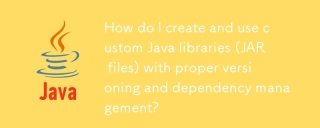
이 기사에서는 Maven 및 Gradle과 같은 도구를 사용하여 적절한 버전 및 종속성 관리로 사용자 정의 Java 라이브러리 (JAR Files)를 작성하고 사용하는 것에 대해 설명합니다.
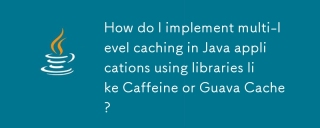
이 기사는 카페인 및 구아바 캐시를 사용하여 자바에서 다단계 캐싱을 구현하여 응용 프로그램 성능을 향상시키는 것에 대해 설명합니다. 구성 및 퇴거 정책 관리 Best Pra와 함께 설정, 통합 및 성능 이점을 다룹니다.

이 기사는 캐싱 및 게으른 하중과 같은 고급 기능을 사용하여 객체 관계 매핑에 JPA를 사용하는 것에 대해 설명합니다. 잠재적 인 함정을 강조하면서 성능을 최적화하기위한 설정, 엔티티 매핑 및 모범 사례를 다룹니다. [159 문자]
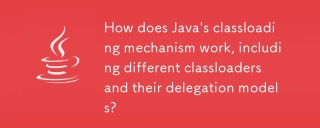
Java의 클래스 로딩에는 부트 스트랩, 확장 및 응용 프로그램 클래스 로더가있는 계층 적 시스템을 사용하여 클래스로드, 링크 및 초기화 클래스가 포함됩니다. 학부모 위임 모델은 핵심 클래스가 먼저로드되어 사용자 정의 클래스 LOA에 영향을 미치도록합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
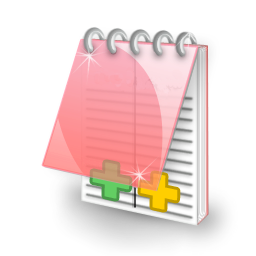
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SecList
SecLists는 최고의 보안 테스터의 동반자입니다. 보안 평가 시 자주 사용되는 다양한 유형의 목록을 한 곳에 모아 놓은 것입니다. SecLists는 보안 테스터에게 필요할 수 있는 모든 목록을 편리하게 제공하여 보안 테스트를 더욱 효율적이고 생산적으로 만드는 데 도움이 됩니다. 목록 유형에는 사용자 이름, 비밀번호, URL, 퍼징 페이로드, 민감한 데이터 패턴, 웹 셸 등이 포함됩니다. 테스터는 이 저장소를 새로운 테스트 시스템으로 간단히 가져올 수 있으며 필요한 모든 유형의 목록에 액세스할 수 있습니다.

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.
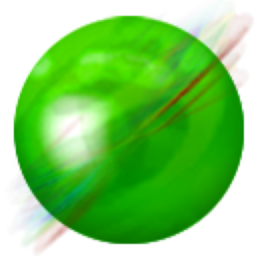
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경
