방법 1: 이메일 알림을 위해 JavaMail API 사용
개요:
JavaMail API는 포괄적인 이메일 및 메시징 기능을 수행하는 데 Java 클라이언트 애플리케이션을 지원하도록 설계된 강력하고 플랫폼 독립적이며 프로토콜 독립적인 프레임워크입니다. 이 API는 거래 이메일 시스템에서 생성된 다양한 개체를 나타내는 추상 클래스가 포함된 일반 인터페이스를 제공합니다. 신뢰성과 광범위한 기능이 가장 중요한 엔터프라이즈급 애플리케이션에 특히 유용합니다.
장점:
-
잘 구성된 구조와 널리 채택됨:
- JavaMail API는 견고한 구조와 특히 기업 환경에서 널리 사용되는 것으로 유명합니다.
-
다양한 기능:
- 이메일 읽기, 작성, 보내기 등 다양한 기능을 제공합니다.
-
프로그래밍 방식 통합:
- 다른 프로그램과의 통합을 단순화하여 프로그래밍 방식으로 확인 및 기타 메시지를 더 쉽게 보낼 수 있습니다.
단점:
-
편집 시간 연장:
- 개발자는 API의 복잡성으로 인해 코드 컴파일 시간이 길어질 수 있습니다.
-
메모리 소비:
- JavaMail API를 사용하면 Java 힙 공간이 많이 소모될 수 있습니다.
구현 단계:
1단계: JavaMail API 설치
- CLASSPATH에 JAR 파일(mail.jar 및 activate.jar)을 포함합니다.
- 이메일 전송을 위한 SMTP 서버(예: Pepipost)를 구성하세요.
2단계: 메일 세션 설정
- javax.mail.Session을 사용하여 호스트 정보가 포함된 세션 개체를 생성합니다.
Properties properties = new Properties(); Session session = Session.getDefaultInstance(properties, null);
- 또는:
Properties properties = new Properties(); Session session = Session.getInstance(properties, null);
- 네트워크 인증:
Session session = Session.getInstance(properties, new javax.mail.Authenticator() { protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication("sender@gmail.com", "password"); } });
3단계: 이메일 작성
- javax.mail.internet.MimeMessage 하위 클래스를 사용하여 발신자, 수신자, 제목 및 메시지 본문을 설정합니다.
MimeMessage message = new MimeMessage(session); message.setFrom(new InternetAddress(from)); message.addRecipient(Message.RecipientType.TO, new InternetAddress(to)); message.setSubject("This is the email subject"); message.setText("This is the email body");
4단계: 첨부 파일 추가
- 첨부 파일을 포함하려면 Multipart 개체를 생성하고 각 첨부 파일에 BodyPart를 추가하세요.
Multipart multipart = new MimeMultipart(); BodyPart messageBodyPart = new MimeBodyPart(); messageBodyPart.setText("This is the email body"); multipart.addBodyPart(messageBodyPart); messageBodyPart = new MimeBodyPart(); DataSource source = new FileDataSource("path/to/attachment.txt"); messageBodyPart.setDataHandler(new DataHandler(source)); messageBodyPart.setFileName("attachment.txt"); multipart.addBodyPart(messageBodyPart); message.setContent(multipart);
5단계: 이메일 보내기
- 이메일을 보내려면 javax.mail.Transport 클래스를 사용하세요.
Transport.send(message);
전체 코드 예:
import java.util.Properties; import javax.mail.*; import javax.mail.internet.*; import javax.activation.*; public class SendMail { public static void main(String[] args) { String to = "receiver@gmail.com"; String from = "sender@gmail.com"; String host = "smtp.gmail.com"; Properties properties = System.getProperties(); properties.put("mail.smtp.host", host); properties.put("mail.smtp.port", "465"); properties.put("mail.smtp.ssl.enable", "true"); properties.put("mail.smtp.auth", "true"); Session session = Session.getInstance(properties, new javax.mail.Authenticator(){ protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication("sender@gmail.com", "password"); } }); try { MimeMessage message = new MimeMessage(session); message.setFrom(new InternetAddress(from)); message.addRecipient(Message.RecipientType.TO, new InternetAddress(to)); message.setSubject("Your email subject goes here"); Multipart multipart = new MimeMultipart(); BodyPart messageBodyPart = new MimeBodyPart(); messageBodyPart.setText("You have a new message"); multipart.addBodyPart(messageBodyPart); messageBodyPart = new MimeBodyPart(); DataSource source = new FileDataSource("path/to/attachment.txt"); messageBodyPart.setDataHandler(new DataHandler(source)); messageBodyPart.setFileName("attachment.txt"); multipart.addBodyPart(messageBodyPart); message.setContent(multipart); Transport.send(message); } catch (MessagingException mex) { mex.printStackTrace(); } } }
방법 2: 이메일 알림을 위해 간단한 Java Mail 사용
개요:
Simple Java Mail은 Java로 SMTP 이메일을 보내는 프로세스를 단순화하도록 설계된 사용자 친화적인 메일링 라이브러리입니다. 이는 JavaMail API에 대한 래퍼 역할을 하며 기본 API의 복잡성을 줄여 이메일 전송 프로세스를 간소화합니다.
장점:
-
견고함과 가벼움:
- Simple Java Mail은 134kB의 가벼운 설치 공간을 유지하면서 강력합니다.
-
RFC 규정 준수:
- 모든 관련 RFC를 준수하여 다양한 이메일 클라이언트와의 호환성을 보장합니다.
-
인증된 SOCKS 프록시 지원:
- 인증된 SOCKS 프록시를 통해 이메일 전송을 지원합니다.
-
고급 기능:
- HTML 콘텐츠, 이미지, 첨부 파일을 지원하고 동시에 여러 수신자에게 이메일을 보낼 수 있습니다.
단점:
-
제한적인 커뮤니티 지원:
- Simple Java Mail에 대한 커뮤니티 지원은 JavaMail API에 비해 규모가 작습니다.
구현 단계:
1단계: HTML과 첨부 파일이 포함된 이메일 객체 생성
Email email = EmailBuilder.startingBlank() .from("From", "from@example.com") .to("1st Receiver", "rec1@example.com") .to("2nd Receiver", "rec2@example.com") .withSubject("Enhanced Email with HTML and Attachments") .withHTMLText("<h1 id="Hello">Hello!</h1><p>This is an enhanced email with HTML content.</p>") .withAttachment("path/to/attachment.txt") .buildEmail();
2단계: MailerBuilder를 사용하여 메일러 객체 생성
Mailer mailer = MailerBuilder .withSMTPServer("smtp.mailtrap.io", 2525, "username", "password") .withTransportStrategy(TransportStrategy.SMTPS) .buildMailer();
3단계: 강화된 이메일 보내기
mailer.sendMail(email);
전체 코드 예:
import org.simplejavamail.api.email.Email; import org.simplejavamail.email.EmailBuilder; import org.simplejavamail.mailer.Mailer; import org.simplejavamail.mailer.MailerBuilder; import org.simplejavamail.api.mailer.config.TransportStrategy; public class SendEnhancedMail { public static void main(String[] args) { Email email = EmailBuilder.startingBlank() .from("From", "from@example.com") .to("1st Receiver", "case1@example.com") .to("2nd Receiver", "case2@example.com") .withSubject("Enhanced Email with HTML and Attachments") .withHTMLText("<h1 id="Hello">Hello!</h1><p>This is an enhanced email with HTML content.</p>") .withAttachment("path/to/attachment.txt") .buildEmail(); Mailer mailer = MailerBuilder .withSMTPServer("smtp.mailtrap.io", 2525, "username", "password") .withTransportStrategy(TransportStrategy.SMTPS) .buildMailer(); mailer.sendMail(email); } }
방법 3: JAVA SDK를 사용하여 다중 채널 알림에 SuprSend 사용
개요:
SuprSend는 통합 API를 통해 이메일, SMS, 푸시 알림과 같은 다양한 채널을 통해 알림 전송을 지원하는 포괄적인 타사 다중 채널 알림 인프라를 제공합니다. SuprSend를 활용하면 개발자는 복잡한 알림 워크플로우를 원활하게 관리할 수 있습니다.
주요 특징 및 이점:
-
광범위한 통합 옵션:
- 50개 이상의 통신 서비스 제공업체(CSP)와 원활하게 통합되며 Mixpanel, Segment, Twilio, Mailchimp, Slack, Teams, SNS, Vonage, Whatsapp 등을 포함한 여러 채널을 지원합니다.
-
기술 의존성 없음:
- Manages the entire notification lifecycle without heavy reliance on the engineering team. Integrate the JAVA SDK once, and the product team can handle the rest.
-
Intelligent Routing:
- Implements intelligent cross-channel flows across providers without requiring technical dependencies.
-
In-App SDK:
- Provides a developer-ready in-app layer for both web and mobile applications.
-
Granular Template Management:
- Features an intuitive drag & drop editor for designing templates, offering superior control over content.
-
Powerful Workspace:
- Manages multiple projects with distinct integrations, workflows, and templates within each workspace.
-
Unified Analytics:
- Provides a unified view of cross-channel analytics, enabling data-driven decision-making.
-
Smart Automation:
- Automates synchronization, refreshing, and notification triggers to streamline operations.
-
Scalability:
- Automates scalability, ensuring a hassle-free experience.
Cons:
-
Cost Considerations:
- Managing multiple notification channels may incur costs.
- *Monthly Notification Limit:*
- Though SuprSend provides 10k notifications free every month, which resets every month, you can also buy credits.
Limits:**
- There may be restrictions on the number of notifications per month.
Implementation Steps:
Step 1: Integrating the JAVA SDK
-
Install the SuprSend JAVA SDK:
- Add the SDK to your JAVA project via Maven or Gradle.
Step 2: Configuring the API Key and Workspace Secret
-
Set Up Configuration:
- Obtain the API key and workspace secret from your SuprSend account and configure them in your JAVA project.
Step 3: Creating and Sending Notifications
-
Send Notifications via JAVA SDK:
- Use the SDK to send notifications, specifying the required channel (email, SMS, push, etc.) and the content.
import com.suprsend.Notification; import com.suprsend.NotificationBuilder; import com.suprsend.SuprSendClient; public class SendNotification { public static void main(String[] args) { // Initialize the SuprSendClient with API key and Workspace Secret SuprSendClient client = new SuprSendClient("your_api_key", "your_workspace_secret"); // Build the notification Notification notification = NotificationBuilder.startingBlank() .withRecipientEmail("recipient@example.com") .withRecipientSMS("recipient_phone_number") .withSubject("Notification Subject") .withHTMLBody("<h1 id="Hello">Hello!</h1><p>This is a multichannel notification.</p>") .build(); // Send the notification client.sendNotification(notification); } }
Complete Code Example with JAVA SDK:
import com.suprsend.Notification; import com.suprsend.NotificationBuilder; import com.suprsend.SuprSendClient; public class SuprSendExample { public static void main(String[] args) { // Initialize the SuprSendClient with API key and Workspace Secret SuprSendClient client = new SuprSendClient("your_api_key", "your_workspace_secret"); // Create the notification Notification notification = NotificationBuilder.startingBlank() .withRecipientEmail("receiver@example.com") .withSubject("Subject of the Notification") .withHTMLBody("<h1 id="Hello">Hello!</h1><p>This is a notification from SuprSend.</p>") .withAttachment("path/to/attachment.txt") .build(); // Send the notification client.sendNotification(notification); } }
These methods offer a comprehensive guide to sending email notifications using Java, with varying levels of complexity and integration capabilities to suit different needs and scenarios.
You may want to check out other SuprSend SDKs too. Consider giving us a star after usage. It's free and open.
suprsend
/
suprsend-go
SuprSend SDK for go
suprsend-go
SuprSend Go SDK
Installation
go get github.com/suprsend/suprsend-go
Usage
Initialize the SuprSend SDK
import (
"log"
suprsend "github.com/suprsend/suprsend-go"
)
func main() {
opts := []suprsend.ClientOption{
// suprsend.WithDebug(true),
}
suprClient, err := suprsend.NewClient("__api_key__", "__api_secret__", opts...)
if err != nil {
log.Println(err)
}
}
Trigger Workflow
package main
import (
"log"
suprsend "github.com/suprsend/suprsend-go"
)
func main() {
// Instantiate Client
suprClient, err := suprsend.NewClient("__api_key__", "__api_secret__")
if err != nil {
log.Println(err)
return
}
// Create workflow body
wfBody := map[string]interface{}{
"name": "Workflow Name",
"template": "template slug",
"notification_category": "category",
// "delay": "15m", // Chek duration format in documentation
"users": []map[string]interface{}{
{
"distinct_id": "0f988f74-6982-41c5-8752-facb6911fb08",
…
suprsend
/
suprsend-py-sdk
SuprSend SDK for python3
suprsend-py-sdk
This package can be included in a python3 project to easily integrate with SuprSend platform.
We're working towards creating SDK in other languages as well.
SuprSend SDKs available in following languages
- python3 >= 3.7 (suprsend-py-sdk)
- node (suprsend-node-sdk)
- java (suprsend-java-sdk)
Installation
suprsend-py-sdk is available on PyPI. You can install using pip.
pip install suprsend-py-sdk
This SDK depends on a system package called libmagic. You can install it as follows:
<span class="pl-c"># On debian based systems</span>
sudo apt install libmagic
<span class="pl-c"># If you are using macOS</span>
brew install libmagic
Usage
Initialize the SuprSend SDK
from suprsend import Suprsend
# Initialize SDK
supr_client = Suprsend("workspace_key", "workspace_secret")
Following example shows a sample request for triggering a workflow. It triggers a notification to a user with id: distinct_id, email: user@example.com & androidpush(fcm-token): __android_push_fcm_token__ using template purchase-made and notification_category system
from suprsend import Workflow
…
suprsend
/
suprsend-node-sdk
Official SuprSend SDK for Node.js
suprsend-node-sdk
This package can be included in a node project to easily integrate with SuprSend platform.
Installation
npm install @suprsend/node-sdk@latest
Initialization
const { Suprsend } = require("@suprsend/node-sdk");
const supr_client = new Suprsend("workspace_key", "workspace_secret");
Trigger workflow from API
It is a unified API to trigger workflow and doesn't require user creation before hand. If you are using our frontend SDK's to configure notifications and passing events and user properties from third-party data platforms like Segment, then event-based trigger would be a better choice.
const { Suprsend, WorkflowTriggerRequest } = require("@suprsend/node-sdk");
const supr_client = new Suprsend("workspace_key", "workspace_secret");
// workflow payload
const body = {
workflow: "_workflow_slug_",
actor: {
distinct_id: "0fxxx8f74-xxxx-41c5-8752-xxxcb6911fb08",
name: "actor_1",
},
recipients: [
{
distinct_id: "0gxxx9f14-xxxx-23c5-1902-xxxcb6912ab09",
$email: ["abc@example.com"
…
suprsend
/
suprsend-react-inbox
SuprSend SDK for integrating inbox functionality in React applications
@suprsend/react-inbox
Integrating SuprSend Inbox channel in React websites can be done in two ways:
- SuprSendInbox component which comes with UI and customizing props.
- SuprSendProvider headless component and hooks, incase you want to totally take control of UI. (example: Full page notifications).
Detailed documentation can be found here: https://docs.suprsend.com/docs/inbox-react
Installation
You can install SuprSend inbox SDK using npm/yarn
npm install @suprsend/react-inbox
SuprSendInbox Integration
After installing, Import the component in your code and use it as given below. Replace the variables with actual values.
import SuprSendInbox from '@suprsend/react-inbox'
import 'react-toastify/dist/ReactToastify.css' // needed for toast notifications, can be ignored if hideToast=true
// add to your react component;
<suprsendinbox workspacekey="<workspace_key>" subscriberid="<subscriber_id>" distinctid="<distinct_id>"></suprsendinbox>
interface ISuprSendInbox {
workspaceKey: string
distinctId: string | null
subscriberId: string | null
tenantId?: string
stores?: IStore[]
pageSize?: number
pagination?: boolean
…위 내용은 Java(Javamail API, Simple Java Mail 또는 SuprSend Java SDK)를 사용하여 이메일 보내기의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
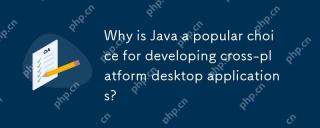
javaispopularforcross-platformdesktopapplicationsduetoits "writeonce, runanywhere"철학
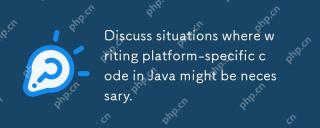
Java에서 플랫폼 별 코드를 작성하는 이유에는 특정 운영 체제 기능에 대한 액세스, 특정 하드웨어와 상호 작용하고 성능 최적화가 포함됩니다. 1) JNA 또는 JNI를 사용하여 Windows 레지스트리에 액세스하십시오. 2) JNI를 통한 Linux 특이 적 하드웨어 드라이버와 상호 작용; 3) 금속을 사용하여 JNI를 통해 MacOS의 게임 성능을 최적화하십시오. 그럼에도 불구하고 플랫폼 별 코드를 작성하면 코드의 이식성에 영향을 미치고 복잡성을 높이며 잠재적으로 성능 오버 헤드 및 보안 위험을 초래할 수 있습니다.
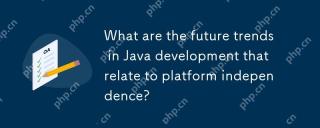
Java는 Cloud-Native Applications, Multi-Platform 배포 및 교차 운용성을 통해 플랫폼 독립성을 더욱 향상시킬 것입니다. 1) Cloud Native Applications는 Graalvm 및 Quarkus를 사용하여 시작 속도를 높입니다. 2) Java는 임베디드 장치, 모바일 장치 및 양자 컴퓨터로 확장됩니다. 3) Graalvm을 통해 Java는 Python 및 JavaScript와 같은 언어와 완벽하게 통합되어 언어 교차 수용 가능성을 향상시킵니다.
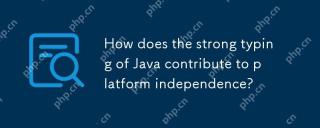
Java의 강력한 유형 시스템은 유형 안전, 통합 유형 변환 및 다형성을 통해 플랫폼 독립성을 보장합니다. 1) 유형 안전성 런타임 오류를 피하기 위해 컴파일 시간에 유형 검사를 수행합니다. 2) 통합 유형 변환 규칙은 모든 플랫폼에서 일관성이 있습니다. 3) 다형성 및 인터페이스 메커니즘은 코드가 다른 플랫폼에서 일관되게 행동하게 만듭니다.
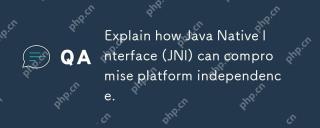
JNI는 Java의 플랫폼 독립성을 파괴 할 것입니다. 1) JNI는 특정 플랫폼에 대한 로컬 라이브러리를 요구합니다. 2) 대상 플랫폼에서 로컬 코드를 컴파일하고 연결해야합니다. 3) 운영 체제 또는 JVM의 다른 버전은 다른 로컬 라이브러리 버전을 필요로 할 수 있습니다.
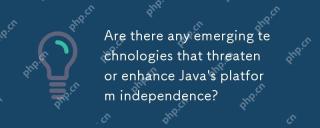
신흥 기술은 위협을 일으키고 Java의 플랫폼 독립성을 향상시킵니다. 1) Docker와 같은 클라우드 컴퓨팅 및 컨테이너화 기술은 Java의 플랫폼 독립성을 향상 시키지만 다양한 클라우드 환경에 적응하도록 최적화되어야합니다. 2) WebAssembly는 Graalvm을 통해 Java 코드를 컴파일하여 플랫폼 독립성을 확장하지만 성능을 위해 다른 언어와 경쟁해야합니다.
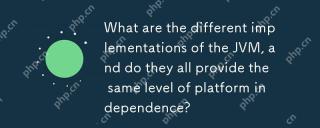
다른 JVM 구현은 플랫폼 독립성을 제공 할 수 있지만 성능은 약간 다릅니다. 1. OracleHotspot 및 OpenJDKJVM 플랫폼 독립성에서 유사하게 수행되지만 OpenJDK에는 추가 구성이 필요할 수 있습니다. 2. IBMJ9JVM은 특정 운영 체제에서 최적화를 수행합니다. 3. Graalvm은 여러 언어를 지원하며 추가 구성이 필요합니다. 4. AzulzingJVM에는 특정 플랫폼 조정이 필요합니다.
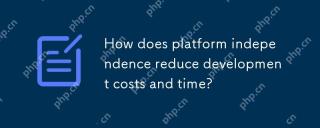
플랫폼 독립성은 여러 운영 체제에서 동일한 코드 세트를 실행하여 개발 비용을 줄이고 개발 시간을 단축시킵니다. 구체적으로, 그것은 다음과 같이 나타납니다. 1. 개발 시간을 줄이면 하나의 코드 세트 만 필요합니다. 2. 유지 보수 비용을 줄이고 테스트 프로세스를 통합합니다. 3. 배포 프로세스를 단순화하기위한 빠른 반복 및 팀 협업.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구
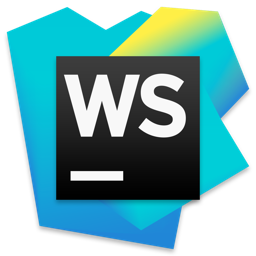
WebStorm Mac 버전
유용한 JavaScript 개발 도구

DVWA
DVWA(Damn Vulnerable Web App)는 매우 취약한 PHP/MySQL 웹 애플리케이션입니다. 주요 목표는 보안 전문가가 법적 환경에서 자신의 기술과 도구를 테스트하고, 웹 개발자가 웹 응용 프로그램 보안 프로세스를 더 잘 이해할 수 있도록 돕고, 교사/학생이 교실 환경 웹 응용 프로그램에서 가르치고 배울 수 있도록 돕는 것입니다. 보안. DVWA의 목표는 다양한 난이도의 간단하고 간단한 인터페이스를 통해 가장 일반적인 웹 취약점 중 일부를 연습하는 것입니다. 이 소프트웨어는

SublimeText3 영어 버전
권장 사항: Win 버전, 코드 프롬프트 지원!
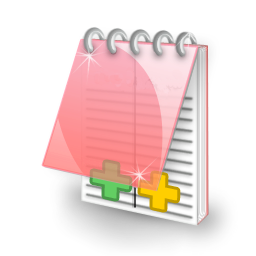
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기
