Golang에서 멀티파트 파일 업로드를 처리하려면 multipart/form-data 콘텐츠 유형을 사용하여 요청을 여러 부분으로 나누는 작업이 포함됩니다. FormFile 및 ParseMultipartForm 함수를 사용하여 요청을 구문 분석합니다. 업로드된 파일을 가져와서 처리하세요. 실제 사례: HTML 양식에 파일 입력 필드 추가. Go 코드를 사용하여 Echo 프레임워크 및 spew 라이브러리를 가져오고 파일 업로드 핸들러를 정의하세요. 요청 양식을 구문 분석하고 파일을 가져옵니다. 파일 세부정보를 인쇄합니다. 서버를 실행하고 업로드 기능을 테스트합니다.
Golang에서 다중 부분 파일 업로드 처리
소개
다중 부분 파일 업로드는 파일을 더 작은 덩어리로 나누고 HTTP 요청으로 전송하는 기술입니다. 일반적으로 대용량 파일을 업로드하거나 청크로 업로드하는 데 사용됩니다. 이 글은 Golang에서 여러 부분으로 구성된 파일 업로드를 처리하는 방법을 안내하고 간단한 실제 사례를 제공합니다.
Multipart/Form-Data
Multipart 파일 업로드는 요청을 여러 부분으로 나누는 multipart/form-data 콘텐츠 유형을 사용합니다. 각 섹션에는 제목, 콘텐츠 유형 및 실제 파일 콘텐츠를 가리키는 양식 필드가 있습니다.
요청 구문 분석
Golang에서 다중 부분 요청을 구문 분석하려면 FormFile
및 ParseMultipartForm
함수를 사용할 수 있습니다. FormFile
和 ParseMultipartForm
函数:
import ( "fmt" "log" "github.com/labstack/echo/v4" ) func upload(c echo.Context) error { // Read the form data form, err := c.MultipartForm() if err != nil { return err } // Retrieve the uploaded file file, err := form.File("file") if err != nil { return err } // Do something with the file return nil }
实战案例
下面是一个简单的实战案例,展示如何在 Golang 中实现多部分文件上传:
HTML 表单:
<form action="/upload" method="POST" enctype="multipart/form-data"> <input type="file" name="file"> <button type="submit">Upload</button> </form>
Go 代码:
// Install echo/v4 and github.com/go-spew/spew // main.go package main import ( "fmt" "github.com/labstack/echo/v4" "github.com/labstack/echo/v4/middleware" "github.com/go-spew/spew" "net/http" ) func main() { e := echo.New() e.Use(middleware.Logger()) e.POST("/upload", upload) e.Logger.Fatal(e.Start(":8080")) } func upload(c echo.Context) error { // Read the form data form, err := c.MultipartForm() if err != nil { return err } // Retrieve the uploaded file file, err := form.File("file") if err != nil { return err } // Print the file details spew.Dump(file) return c.JSON(http.StatusOK, map[string]interface{}{ "message": "File uploaded successfully", }) }
测试上传
访问 /upload
表单并选择一个文件进行上传。成功上传后,控制台将打印已上传文件的详细信息。
提示
- 使用
FormFile
函数可以获取单个文件。 - 使用
ParseMultipartForm
函数可以获取多个文件和其他表单字段。 -
multipart/form-data
rrreee
/upload를 방문하세요. 코드 > 양식을 선택하고 업로드할 파일을 선택하세요. 업로드가 성공적으로 완료되면 콘솔은 업로드된 파일의 세부 정보를 인쇄합니다. 🎜🎜🎜Tips🎜🎜<ul>
<li>단일 파일을 얻으려면 <code>FormFile
기능을 사용하세요. 🎜
ParseMultipartForm
함수를 사용하세요. 🎜multipart/form-data
는 이미지, 동영상 등 다른 유형의 파일 업로드에도 사용할 수 있습니다. 🎜🎜위 내용은 Golang에서 멀티파트 파일 업로드를 처리하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
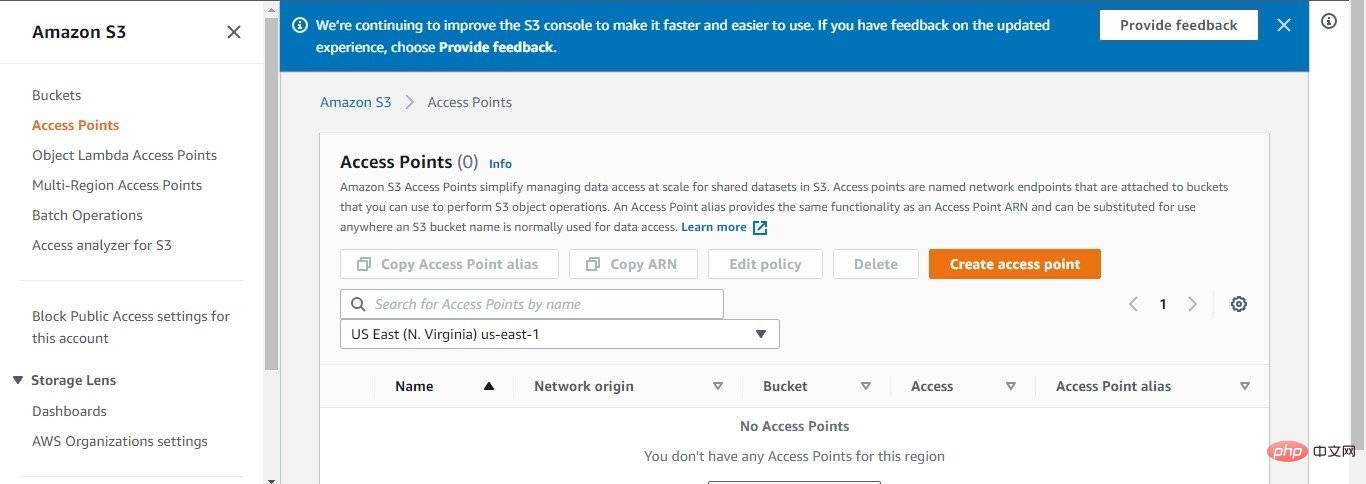
Amazon Simple Storage Service,简称Amazon S3,是一种使用 Web 界面提供存储对象的存储服务。Amazon S3 存储对象可以存储不同类型和大小的数据,从应用程序到数据存档、备份、云存储、灾难恢复等等。该服务具有可扩展性,用户只需为存储空间付费。Amazon S3 有四个基于可用性、性能率和持久性的存储类别。这些类包括 Amazon S3 Standard、Amazon S3 Standard Infrequent Access、Amazon S3 One
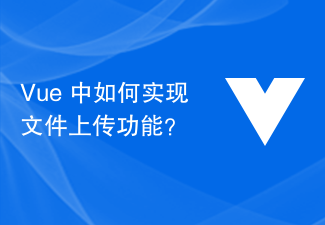
Vue作为目前前端开发最流行的框架之一,其实现文件上传功能的方式也十分简单优雅。本文将为大家介绍在Vue中如何实现文件上传功能。HTML部分在HTML文件中添加如下代码,创建上传表单:<template><div><formref="uploadForm"enc
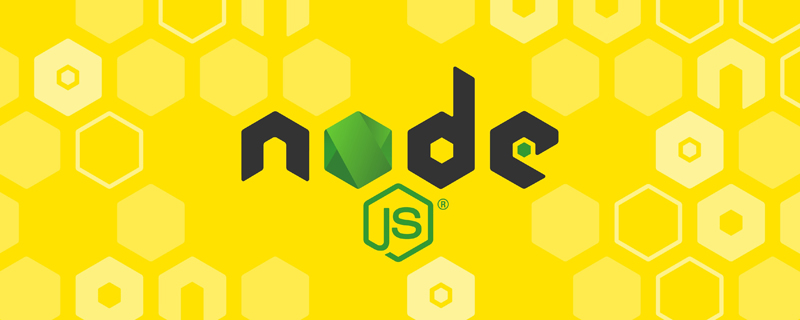
怎么处理文件上传?下面本篇文章给大家介绍一下node项目中如何使用express来处理文件的上传,希望对大家有所帮助!
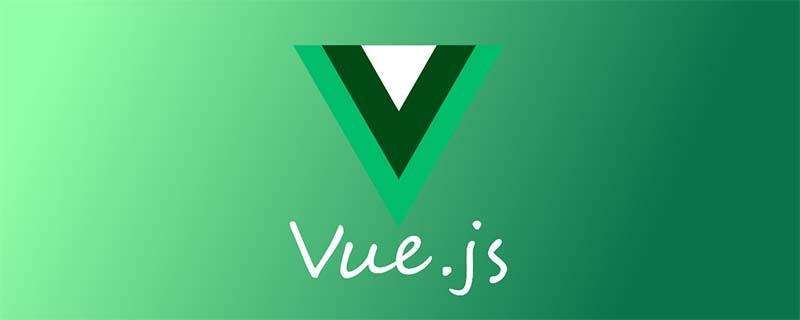
在实际开发项目过程中有时候需要上传比较大的文件,然后呢,上传的时候相对来说就会慢一些,so,后台可能会要求前端进行文件切片上传,很简单哈,就是把比如说1个G的文件流切割成若干个小的文件流,然后分别请求接口传递这个小的文件流。
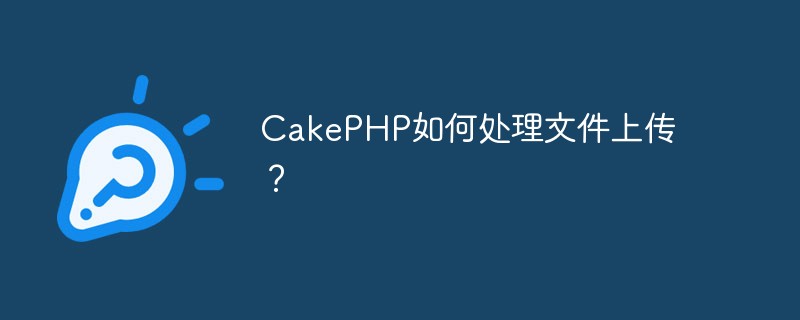
CakePHP是一个开源的Web应用程序框架,它基于PHP语言构建,可以简化Web应用程序的开发过程。在CakePHP中,处理文件上传是一个常见的需求,无论是上传头像、图片还是文档,都需要在程序中实现相应的功能。本文将介绍CakePHP中如何处理文件上传的方法和一些注意事项。在Controller中处理上传文件在CakePHP中,上传文件的处理通常在Cont
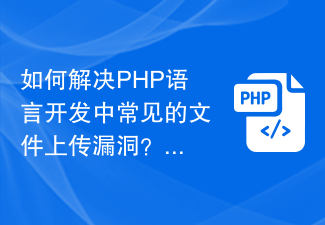
在Web应用程序的开发中,文件上传功能已经成为了基本的需求。这个功能允许用户向服务器上传自己的文件,然后在服务器上进行存储或处理。然而,这个功能也使得开发者更需要注意一个安全漏洞:文件上传漏洞。攻击者可以通过上传恶意文件来攻击服务器,从而导致服务器遭受不同程度的破坏。PHP语言作为广泛应用于Web开发中的语言之一,文件上传漏洞也是常见的安全问题之一。本文将介
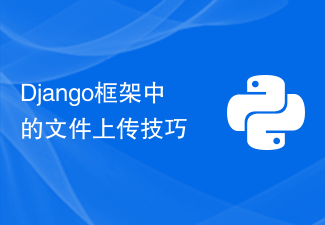
近年来,Web应用程序逐渐流行,而其中许多应用程序都需要文件上传功能。在Django框架中,实现上传文件功能并不困难,但是在实际开发中,我们还需要处理上传的文件,其他操作包括更改文件名、限制文件大小等问题。本文将分享一些Django框架中的文件上传技巧。一、配置文件上传项在Django项目中,要配置文件上传需要在settings.py文件中进
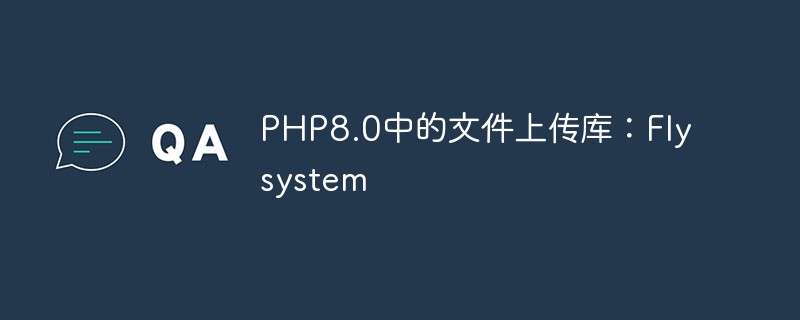
随着互联网的发展和普及,文件上传功能已经成为现代网站开发的必备功能之一。不论是网盘还是社交平台,文件上传都是必不可少的一环。而在PHP领域,由于其广泛的应用和易用性,文件上传的需求也非常常见。在PHP8.0中,一个名为Flysystem的文件上传库正式出现,它为PHP开发人员提供了更加高效、灵活且易于使用的文件上传和管理解决方案。Flysystem是一个轻量


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
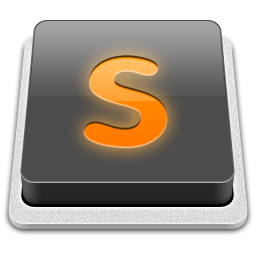
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.
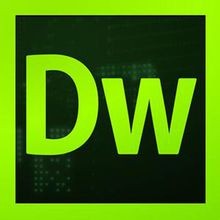
드림위버 CS6
시각적 웹 개발 도구
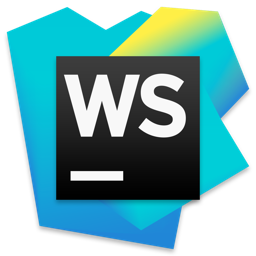
WebStorm Mac 버전
유용한 JavaScript 개발 도구
