When writing JSP programs, programmers may miss some bugs, and these bugs may appear anywhere in the program. There are usually the following types of exceptions in JSP code:
Checked exception: A checked exception is a typical user error or an error that the programmer cannot foresee. For example, if a file is about to be opened, but the file cannot be found, an exception is thrown. These exceptions cannot simply be ignored at compile time.
Run-time exception: A run-time exception may have been avoided by the programmer. This exception will be ignored at compile time.
Error: There is no exception here, but the problem is that it is beyond the control of the user or programmer. Errors are often ignored in the code and there is little you can do about it. For example, stack overflow error. These errors will be ignored at compile time.
This section will give several simple and elegant ways to handle runtime exceptions and errors.
Using the Exception object
The exception object is an instance of the Throwable subclass and is only available in error pages. The following table lists some important methods in the Throwable class:
Serial number | Method&Description |
---|
##1 | public String getMessage() Return abnormal information. This information is initialized in the Throwable constructor
|
2 | public ThrowablegetCause() returns to cause an exception The reason is that the type is Throwable object
|
3 | public String toString() Return class name
|
4 | public void printStackTrace() Output the exception stack trace to System.err |
5 | public StackTraceElement [] getStackTrace() Returns the exception stack trace in the form of an array of stack trace elements
|
6 | public ThrowablefillInStackTrace() Use the current stack trace to fill the Throwable object
|
JSP provides the option to specify an error page for each JSP page. Whenever the page throws an exception, the JSP container automatically calls the error page.
The following example specifies an error page for main.jsp. Use the <%@page errorPage="XXXXX"%> directive to specify an error page.
<%@ page errorPage="ShowError.jsp" %>
<html>
<head>
<title>Error Handling Example</title>
</head>
<body>
<%
// Throw an exception to invoke the error page
int x = 1;
if (x == 1)
{
throw new RuntimeException("Error condition!!!");
}
%>
</body>
</html>
Now, write the ShowError.jsp file as follows:
<%@ page isErrorPage="true" %>
<html>
<head>
<title>Show Error Page</title>
</head>
<body>
<h1>Opps...</h1>
<p>Sorry, an error occurred.</p>
<p>Here is the exception stack trace: </p>
<pre>
<% exception.printStackTrace(response.getWriter()); %>
Notice that the ShowError.jsp file uses the <%@page isErrorPage="true"%> directive. This directive tells The JSP compiler needs to generate an exception instance variable.
Now try to access the main.jsp page, it will produce the following results:
java.lang.RuntimeException: Error condition!!!
......
Opps...
Sorry, an error occurred.
Here is the exception stack trace:
Using JSTL tags in error pages
You can use JSTL tags to Write the error page ShowError.jsp. The code in this example has almost the same logic as the code in the previous example, but the code in this example has a better structure and can provide more information:
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@page isErrorPage="true" %>
<html>
<head>
<title>Show Error Page</title>
</head>
<body>
<h1>Opps...</h1>
<table width="100%" border="1">
<tr valign="top">
<td width="40%"><b>Error:</b></td>
<td>${pageContext.exception}</td>
</tr>
<tr valign="top">
<td><b>URI:</b></td>
<td>${pageContext.errorData.requestURI}</td>
</tr>
<tr valign="top">
<td><b>Status code:</b></td>
<td>${pageContext.errorData.statusCode}</td>
</tr>
<tr valign="top">
<td><b>Stack trace:</b></td>
<td>
<c:forEach var="trace"
items="${pageContext.exception.stackTrace}">
<p>${trace}</p>
</c:forEach>
</td>
</tr>
</table>
</body>
</html>
The running results are as follows:
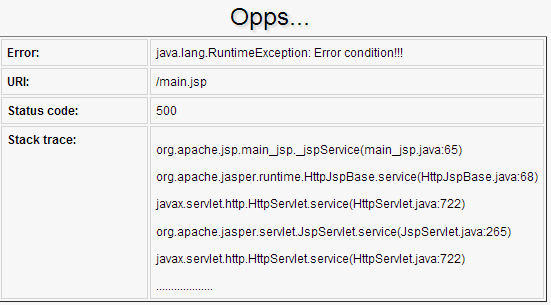
Use try...catch block
If you want to put exception handling in one page and handle different exceptions differently, then you need to use try...catch block.
The following example shows how to use a try...catch block and place this code in main.jsp:
<html>
<head>
<title>Try...Catch Example</title>
</head>
<body>
<%
try{
int i = 1;
i = i / 0;
out.println("The answer is " + i);
}
catch (Exception e){
out.println("An exception occurred: " + e.getMessage());
}
%>
</body>
</html>
Try to access main.jsp, it will produce the following results :
An exception occurred: / by zero