JavaScript object
JavaScript objects are data that have properties and methods.
Objects, properties and methods in real life
In real life, a car is an object.
The object has its properties, such as weight and color, etc., and the methods include start and stop, etc.:
Object | Properties | Method |
---|
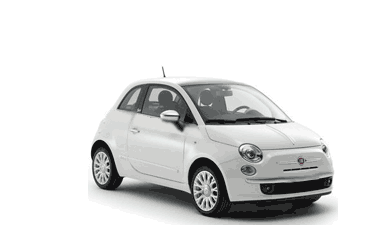 | car.name = Fiat
#car.model = 500
car.weight = 850kg
car.color = white | car.start()
car.drive()
car.brake ()
car.stop() |
#All cars have these attributes, but the attributes of each car are different.
All cars have these methods, but they are executed at different times.
JavaScript Objects
In JavaScript, almost everything is an object.
 | exist
In JavaScript, objects are very important. When you understand objects, you can understand JavaScript. |
---|
#You have learned about JavaScript variable assignment.
The following code sets the value of variable car to "Fiat":
The object is also a variable, but the object can contain multiple values (multiple variables).
var car = {type:"Fiat", model:500, color:"white"};
In the above example, 3 values ("Fiat", 500, "white") are assigned to the variable car.
In the above example, 3 variables (type, model, color) are assigned to the variable car.
 | JavaScript objects are containers for variables. |
---|
Object definition
You can use characters to define and create JavaScript objects:
Examples
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>创建 JavaScript 对象。</p>
<p id="demo"></p>
<script>
var person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
document.getElementById("demo").innerHTML =
person.firstName + " 现在 " + person.age + " 岁.";
</script>
</body>
</html>
Run Example»Click the "Run Example" button to view the online example
Define JavaScript objects can span multiple lines, spaces and line breaks are not required of:
Instance
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>创建 JavaScript 对象。</p>
<p id="demo"></p>
<script>
var person = {
firstName : "John",
lastName : "Doe",
age : 50,
eyeColor : "blue"
};
document.getElementById("demo").innerHTML =
person.firstName + " 现在 " + person.age + " 岁。";
</script>
</body>
</html>
Run Instance»Click the "Run Instance" button to view the online instance
Object properties
It can be said that "JavaScript objects are containers of variables".
However, we usually think of "JavaScript objects as containers of key-value pairs".
The key-value pair is usually written as name : value (key and value are separated by colon).
Key-value pairs in JavaScript objects are usually called Object properties.
 | JavaScript objects are containers for property variables. |
---|
The object key-value pair is written similar to:
Accessing object properties
You can access object properties in two ways:
Instance
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>
有两种方式可以访问对象属性:
</p>
<p>
你可以使用 .property 或 ["property"].
</p>
<p id="demo"></p>
<script>
var person = {
firstName : "John",
lastName : "Doe",
id : 5566
};
document.getElementById("demo").innerHTML =
person.firstName + " " + person.lastName;
</script>
</body>
Run Instance»Click the "Run Instance" button to view the online instance
Instance
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>
有两种方式可以访问对象属性:
</p>
<p>
你可以使用 .property 或 ["property"]。
</p>
<p id="demo"></p>
<script>
var person = {
firstName: "John",
lastName : "Doe",
id : 5566
};
document.getElementById("demo").innerHTML =
person["firstName"] + " " + person["lastName"];
</script>
</body>
</html>
Run Instance»Click the "Run Instance" button to view the online instance
Object methods
The method of an object defines a function and is stored as a property of the object.
Object methods are called (as a function) by adding ().
This instance accesses the fullName() method of the person object:
Instance
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>创建和使用对象方法。</p>
<p>对象方法作为一个函数定义存储在对象属性中。</p>
<p id="demo"></p>
<script>
var person = {
firstName: "John",
lastName : "Doe",
id : 5566,
fullName : function()
{
return this.firstName + " " + this.lastName;
}
};
document.getElementById("demo").innerHTML = person.fullName();
</script>
</body>
</html>
Running instance» Click the "Run Instance" button to view the online instance
If you want to access the fullName property of the person object, it will be returned as a string defining the function:
Instance
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>创建和使用对象方法。</p>
<p>对象方法是一个函数定义,并作为一个属性值存储。</p>
<p id="demo"></p>
<script>
var person = {
firstName: "John",
lastName : "Doe",
id : 5566,
fullName : function()
{
return this.firstName + " " + this.lastName;
}
};
document.getElementById("demo").innerHTML = person.fullName;
</script>
</body>
</html>
Run instance»Click the "Run instance" button to view the online instance
 | ##JavaScript objects are containers for properties and methods. |
---|
You will learn more about functions, properties and methods in the following tutorials.
Accessing object methodsYou can create object methods using the following syntax: ##methodName : function() { code lines }
You can access object methods using the following syntax:
objectName.methodName()
Usually fullName () is a method of the person object,
fullName is available as an attribute.
There are many ways to create, use and modify JavaScript objects.
There are also many ways to create, use and modify properties and methods.
In the following tutorials, you will learn more about objects. | |
---|
More examples
Create JavaScript object I
Instance
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>创建 JavaScript 对象。</p>
<p id="demo"></p>
<script>
var person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
document.getElementById("demo").innerHTML =
person.firstName + " 现在 " + person.age + " 岁.";
</script>
</body>
</html>
Run Example»Click the "Run Example" button to view the online example
Create JavaScript Object II
Example
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>创建 JavaScript 对象。</p>
<p id="demo"></p>
<script>
var person = {
firstName : "John",
lastName : "Doe",
age : 50,
eyeColor : "blue"
};
document.getElementById("demo").innerHTML =
person.firstName + " 现在 " + person.age + " 岁。";
</script>
</body>
</html>
Run instance»Click the "Run instance" button to view the online instance
Access object propertiesI
Instance
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>
有两种方式可以访问对象属性:
</p>
<p>
你可以使用 .property 或 ["property"].
</p>
<p id="demo"></p>
<script>
var person = {
firstName : "John",
lastName : "Doe",
id : 5566
};
document.getElementById("demo").innerHTML =
person.firstName + " " + person.lastName;
</script>
</body>
</html>
Run instance»Click the "Run instance" button to view the online instance
Access object properties II
Instance
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>phn.cn</title>
</head>
<body>
<p>
有两种方式可以访问对象属性:
</p>
<p>
你可以使用 .property 或 ["property"]。
</p>
<p id="demo"></p>
<script>
var person = {
firstName: "John",
lastName : "Doe",
id : 5566
};
document.getElementById("demo").innerHTML =
person["firstName"] + " " + person["lastName"];
</script>
</body>
</html>
Run Instance»Click the "Run Instance" button to view the online instance
Function attribute accessed as a method
Instance
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>创建和使用对象方法。</p>
<p>对象方法作为一个函数定义存储在对象属性中。</p>
<p id="demo"></p>
<script>
var person = {
firstName: "John",
lastName : "Doe",
id : 5566,
fullName : function()
{
return this.firstName + " " + this.lastName;
}
};
document.getElementById("demo").innerHTML = person.fullName();
</script>
</body>
</html>
Run instance»Click the "Run instance" button to view Online instance
Function attribute accessed as a property
Instance
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php.cn</title>
</head>
<body>
<p>创建和使用对象方法。</p>
<p>对象方法是一个函数定义,并作为一个属性值存储。</p>
<p id="demo"></p>
<script>
var person = {
firstName: "John",
lastName : "Doe",
id : 5566,
fullName : function()
{
return this.firstName + " " + this.lastName;
}
};
document.getElementById("demo").innerHTML = person.fullName;
</script>
</body>
</html>
Running instance»Click the "Run Instance" button to view the online instance