単純な投票システムを C で記述するにはどうすればよいでしょうか?
テクノロジーの発展に伴い、投票システムは現代社会で広く使用されるツールになりました。投票システムは、選挙、調査、意思決定などのさまざまなシナリオで使用できます。この記事では、C で簡単な投票システムを作成する方法を説明します。
まず、投票システムの基本的な機能を明確にする必要があります。単純な投票システムには次の機能が必要です。
- 有権者の登録: システムでは、ユーザーが投票に参加できるように有権者として登録できる必要があります。
- 投票の作成: システムでは、管理者が投票を作成し、各投票に一意の ID を割り当てることができるようにする必要があります。
- 公開オプション: 管理者は、投票ごとに候補オプションを追加できる必要があります。
- 投票の実施: 登録有権者は、特定の投票用紙を選択して投票できる必要があります。
- 投票結果のカウント: システムは、各投票の投票結果をカウントし、その結果に基づいてランク付けできる必要があります。
上記の基本的な機能が明確になったら、投票システムの作成を開始できます。
まず、有権者を表す Voter クラスを作成する必要があります。このクラスには、投票者の名前、年齢、性別などの基本情報が含まれ、投票者が投票したかどうかを登録および確認するメソッドが必要です。
次に、投票を表す Vote クラスを作成します。このクラスには、投票の名前、ID、候補オプション、および各オプションの投票数を保存する変数が含まれている必要があります。 Vote クラスには、候補者のオプションを追加し、投票結果をカウントするためのメソッドも必要です。
次に、投票システムを管理するための VotingSystem クラスを作成します。このクラスには、すべての投票を格納するベクトルが含まれ、投票者の登録、投票の作成、候補者のオプションの追加、投票の実行、および投票結果の集計を行うメソッドを提供する必要があります。
最後に、シンプルなコンソール インターフェイスを通じてユーザーと対話し、投票システムの機能を実装します。
以下は簡単な例です:
#include <iostream> #include <vector> using namespace std; // Voter class class Voter { string name; int age; string gender; bool voted; public: Voter(string n, int a, string g) { name = n; age = a; gender = g; voted = false; } bool hasVoted() { return voted; } void setVoted() { voted = true; } }; // Vote class class Vote { string name; int id; vector<string> candidates; vector<int> votes; public: Vote(string n, int i) { name = n; id = i; } void addCandidate(string candidate) { candidates.push_back(candidate); votes.push_back(0); } void castVote(int candidateIndex) { votes[candidateIndex]++; } void printResults() { for (int i = 0; i < candidates.size(); i++) { cout << candidates[i] << ": " << votes[i] << " votes" << endl; } } }; // VotingSystem class class VotingSystem { vector<Voter> voters; vector<Vote> votes; public: void registerVoter(string name, int age, string gender) { Voter voter(name, age, gender); voters.push_back(voter); } void createVote(string name, int id) { Vote vote(name, id); votes.push_back(vote); } void addCandidate(int voteIndex, string candidate) { votes[voteIndex].addCandidate(candidate); } void castVote(int voteIndex, int candidateIndex, int voterIndex) { if (!voters[voterIndex].hasVoted()) { votes[voteIndex].castVote(candidateIndex); voters[voterIndex].setVoted(); } } void printVoteResults(int voteIndex) { votes[voteIndex].printResults(); } }; int main() { VotingSystem votingSystem; // Register voters votingSystem.registerVoter("Alice", 25, "female"); votingSystem.registerVoter("Bob", 30, "male"); votingSystem.registerVoter("Charlie", 35, "male"); // Create vote votingSystem.createVote("Favorite color", 1); // Add candidates votingSystem.addCandidate(0, "Red"); votingSystem.addCandidate(0, "Blue"); votingSystem.addCandidate(0, "Green"); // Cast votes votingSystem.castVote(0, 0, 0); votingSystem.castVote(0, 1, 1); votingSystem.castVote(0, 0, 2); // Print vote results votingSystem.printVoteResults(0); return 0; }
上記は簡単な投票システムの実装例です。 Voter、Vote、および VotingSystem クラスを作成することで、投票者の登録、投票の作成、候補者の選択肢の追加、投票、投票結果の集計などの機能を実装できます。 main 関数では、これらの関数を使用して投票を作成および管理する方法を示します。出力には、各候補オプションの投票数が表示されます。
上記の例を通じて、C 言語を使用して簡単な投票システムを作成する方法を確認できます。もちろん、この例には、投票者認証の追加、同時複数投票のサポート、その他の機能の追加など、改善の余地がまだたくさんあります。ただし、この例は、単純な投票システムの作成を開始する方法を理解するのに十分です。
この記事があなたのお役に立てば幸いです。そして、あなたが完璧な投票システムを作成できることを願っています。
以上がC++ で簡単な投票システムを作成するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
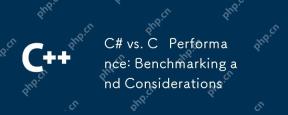
C#とCのパフォーマンスの違いは、主に実行速度とリソース管理に反映されます。1)Cは通常、ハードウェアに近く、ガベージコレクションなどの追加のオーバーヘッドがないため、数値計算と文字列操作でより良いパフォーマンスを発揮します。 2)C#はマルチスレッドプログラミングでより簡潔ですが、そのパフォーマンスはCよりもわずかに劣っています。 3)プロジェクトの要件とチームテクノロジースタックに基づいて、どの言語を選択するかを決定する必要があります。
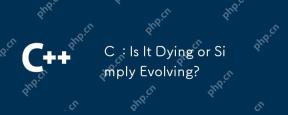
c isnotdying; it'sevolving.1)c relelevantdueToitsversitileSileSixivisityinperformance-criticalApplications.2)thelanguageSlikeModulesandCoroutoUtoimveUsablive.3)despiteChallen
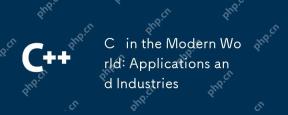
Cは、現代世界で広く使用され、重要です。 1)ゲーム開発において、Cは、非現実的や統一など、その高性能と多型に広く使用されています。 2)金融取引システムでは、Cの低レイテンシと高スループットが最初の選択となり、高周波取引とリアルタイムのデータ分析に適しています。
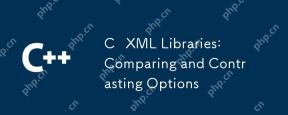
C:tinyxml-2、pugixml、xerces-c、およびrapidxmlには、一般的に使用される4つのXMLライブラリがあります。 1.TinyXML-2は、リソースが限られている環境、軽量ではあるが機能が限られていることに適しています。 2。PUGIXMLは高速で、複雑なXML構造に適したXPathクエリをサポートしています。 3.Xerces-Cは強力で、DOMとSAXの解像度をサポートし、複雑な処理に適しています。 4。RapidXMLはパフォーマンスと分割に非常に高速に焦点を当てていますが、XPathクエリをサポートしていません。
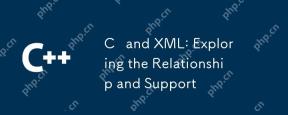
Cは、サードパーティライブラリ(TinyXML、PUGIXML、XERCES-Cなど)を介してXMLと相互作用します。 1)ライブラリを使用してXMLファイルを解析し、それらをC処理可能なデータ構造に変換します。 2)XMLを生成するときは、Cデータ構造をXML形式に変換します。 3)実際のアプリケーションでは、XMLが構成ファイルとデータ交換に使用されることがよくあり、開発効率を向上させます。
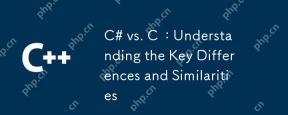
C#とCの主な違いは、構文、パフォーマンス、アプリケーションシナリオです。 1)C#構文はより簡潔で、ガベージコレクションをサポートし、.NETフレームワーク開発に適しています。 2)Cはパフォーマンスが高く、手動メモリ管理が必要であり、システムプログラミングとゲーム開発でよく使用されます。
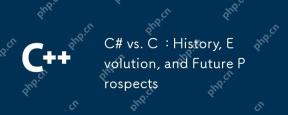
C#とCの歴史と進化はユニークであり、将来の見通しも異なります。 1.Cは、1983年にBjarnestrostrupによって発明され、オブジェクト指向のプログラミングをC言語に導入しました。その進化プロセスには、C 11の自動キーワードとラムダ式の導入など、複数の標準化が含まれます。C20概念とコルーチンの導入、将来のパフォーマンスとシステムレベルのプログラミングに焦点を当てます。 2.C#は2000年にMicrosoftによってリリースされました。CとJavaの利点を組み合わせて、その進化はシンプルさと生産性に焦点を当てています。たとえば、C#2.0はジェネリックを導入し、C#5.0は非同期プログラミングを導入しました。これは、将来の開発者の生産性とクラウドコンピューティングに焦点を当てます。
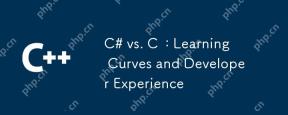
C#とCおよび開発者の経験の学習曲線には大きな違いがあります。 1)C#の学習曲線は比較的フラットであり、迅速な開発およびエンタープライズレベルのアプリケーションに適しています。 2)Cの学習曲線は急勾配であり、高性能および低レベルの制御シナリオに適しています。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、
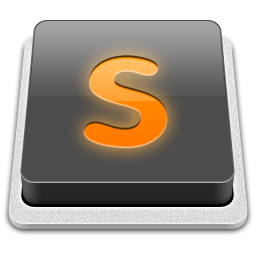
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

メモ帳++7.3.1
使いやすく無料のコードエディター
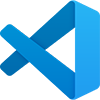
VSCode Windows 64 ビットのダウンロード
Microsoft によって発売された無料で強力な IDE エディター

ホットトピック









