PHP で RESTful API の統合テストを実装する方法
Web アプリケーションの開発と RESTful API の普及に伴い、API の統合テストがますます行われるようになりました。重要。 PHP では、いくつかのツールとテクニックを使用して、このような統合テストを実装できます。この記事では、PHP で RESTful API の統合テストを実装する方法を紹介し、理解を助けるサンプル コードをいくつか紹介します。
- 統合テストでの PHPUnit の使用
PHPUnit は、PHP で最も人気のある単体テスト フレームワークの 1 つです。単体テストだけでなく結合テストにも使用できます。 PHPUnit は、HTTP リクエストをシミュレートし、レスポンスの正確さを検証するためのいくつかの便利なメソッドとアサーションを提供します。以下は、PHPUnit を使用して RESTful API 統合テストを実装する例です。
use PHPUnitFrameworkTestCase; class MyApiTest extends TestCase { private $httpClient; protected function setUp(): void { $this->httpClient = new GuzzleHttpClient([ 'base_uri' => 'http://example.com/api/', ]); } public function testGetUsers() { $response = $this->httpClient->get('users'); $this->assertEquals(200, $response->getStatusCode()); $data = json_decode($response->getBody(), true); $this->assertNotEmpty($data); } public function testUpdateUser() { $response = $this->httpClient->put('users/1', [ 'json' => [ 'name' => 'John Doe', 'email' => 'john.doe@example.com', ], ]); $this->assertEquals(200, $response->getStatusCode()); $data = json_decode($response->getBody(), true); $this->assertEquals('John Doe', $data['name']); $this->assertEquals('john.doe@example.com', $data['email']); } }
- 統合テストにモック HTTP クライアントを使用する
複雑な RESTful API の場合、特定のモックを作成する必要がある場合があります。テストするシナリオまたはデータ。これを行うには、Mock HTTP クライアントを使用して API の応答をシミュレートし、さまざまな状況でコードがどのように動作するかを検証します。以下は、Mock HTTP クライアントを使用して RESTful API 統合テストを実装する例です:
use PHPUnitFrameworkTestCase; use GuzzleHttpHandlerMockHandler; use GuzzleHttpHandlerStack; use GuzzleHttpClient; class MyApiTest extends TestCase { private $httpClient; protected function setUp(): void { $mockHandler = new MockHandler([ new GuzzleHttpPsr7Response(200, [], json_encode(['name' => 'John Doe'])), new GuzzleHttpPsr7Response(404), new GuzzleHttpExceptionConnectException('Connection error', new GuzzleHttpPsr7Request('GET', 'users')), ]); $handlerStack = HandlerStack::create($mockHandler); $this->httpClient = new Client(['handler' => $handlerStack]); } public function testGetUser() { $response = $this->httpClient->get('users/1'); $this->assertEquals(200, $response->getStatusCode()); $data = json_decode($response->getBody(), true); $this->assertEquals('John Doe', $data['name']); } public function testGetNonExistentUser() { $response = $this->httpClient->get('users/999'); $this->assertEquals(404, $response->getStatusCode()); } public function testConnectionError() { $this->expectException(GuzzleHttpExceptionConnectException::class); $this->httpClient->get('users'); } }
Mock HTTP クライアントを使用すると、テストに合わせていつでも API の応答を変更および制御できます。ニーズ。
概要:
PHP での RESTful API の統合テストは、PHPUnit または Mock HTTP クライアントを使用して実行できます。どの方法を選択しても、HTTP リクエストを効果的にシミュレートし、レスポンスの有効性を検証できます。これらの統合テストは、API がさまざまなシナリオで適切に動作することを確認し、API の機能とパフォーマンスを検証するための信頼できる方法を提供するのに役立ちます。
以上がPHPでRESTful APIの統合テストを実装する方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
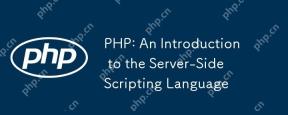
PHPは、動的なWeb開発およびサーバー側のアプリケーションに使用されるサーバー側のスクリプト言語です。 1.PHPは、編集を必要とせず、迅速な発展に適した解釈言語です。 2。PHPコードはHTMLに組み込まれているため、Webページの開発が簡単になりました。 3。PHPプロセスサーバー側のロジック、HTML出力を生成し、ユーザーの相互作用とデータ処理をサポートします。 4。PHPは、データベースと対話し、プロセスフォームの送信、サーバー側のタスクを実行できます。
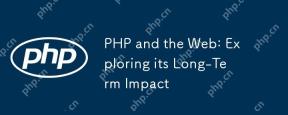
PHPは過去数十年にわたってネットワークを形成しており、Web開発において重要な役割を果たし続けます。 1)PHPは1994年に発信され、MySQLとのシームレスな統合により、開発者にとって最初の選択肢となっています。 2)コア関数には、動的なコンテンツの生成とデータベースとの統合が含まれ、ウェブサイトをリアルタイムで更新し、パーソナライズされた方法で表示できるようにします。 3)PHPの幅広いアプリケーションとエコシステムは、長期的な影響を促進していますが、バージョンの更新とセキュリティの課題にも直面しています。 4)PHP7のリリースなど、近年のパフォーマンスの改善により、現代の言語と競合できるようになりました。 5)将来的には、PHPはコンテナ化やマイクロサービスなどの新しい課題に対処する必要がありますが、その柔軟性とアクティブなコミュニティにより適応性があります。
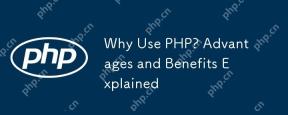
PHPの中心的な利点には、学習の容易さ、強力なWeb開発サポート、豊富なライブラリとフレームワーク、高性能とスケーラビリティ、クロスプラットフォームの互換性、費用対効果が含まれます。 1)初心者に適した学習と使用が簡単。 2)Webサーバーとの適切な統合および複数のデータベースをサポートします。 3)Laravelなどの強力なフレームワークを持っています。 4)最適化を通じて高性能を達成できます。 5)複数のオペレーティングシステムをサポートします。 6)開発コストを削減するためのオープンソース。
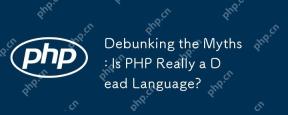
PHPは死んでいません。 1)PHPコミュニティは、パフォーマンスとセキュリティの問題を積極的に解決し、PHP7.xはパフォーマンスを向上させます。 2)PHPは最新のWeb開発に適しており、大規模なWebサイトで広く使用されています。 3)PHPは学習しやすく、サーバーはうまく機能しますが、タイプシステムは静的言語ほど厳格ではありません。 4)PHPは、コンテンツ管理とeコマースの分野で依然として重要であり、エコシステムは進化し続けています。 5)OpcacheとAPCを介してパフォーマンスを最適化し、OOPと設計パターンを使用してコードの品質を向上させます。
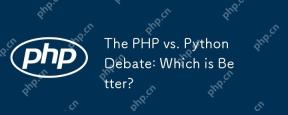
PHPとPythonには独自の利点と短所があり、選択はプロジェクトの要件に依存します。 1)PHPは、Web開発に適しており、学習しやすく、豊富なコミュニティリソースですが、構文は十分に近代的ではなく、パフォーマンスとセキュリティに注意を払う必要があります。 2)Pythonは、簡潔な構文と学習が簡単なデータサイエンスと機械学習に適していますが、実行速度とメモリ管理にはボトルネックがあります。
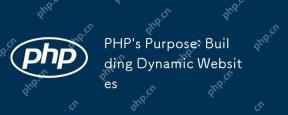
PHPは動的なWebサイトを構築するために使用され、そのコア関数には次のものが含まれます。1。データベースに接続することにより、動的コンテンツを生成し、リアルタイムでWebページを生成します。 2。ユーザーのインタラクションを処理し、提出をフォームし、入力を確認し、操作に応答します。 3.セッションとユーザー認証を管理して、パーソナライズされたエクスペリエンスを提供します。 4.パフォーマンスを最適化し、ベストプラクティスに従って、ウェブサイトの効率とセキュリティを改善します。

PHPはMySQLIおよびPDO拡張機能を使用して、データベース操作とサーバー側のロジック処理で対話し、セッション管理などの関数を介してサーバー側のロジックを処理します。 1)MySQLIまたはPDOを使用してデータベースに接続し、SQLクエリを実行します。 2)セッション管理およびその他の機能を通じて、HTTPリクエストとユーザーステータスを処理します。 3)トランザクションを使用して、データベース操作の原子性を確保します。 4)SQLインジェクションを防ぎ、例外処理とデバッグの閉鎖接続を使用します。 5)インデックスとキャッシュを通じてパフォーマンスを最適化し、読みやすいコードを書き、エラー処理を実行します。
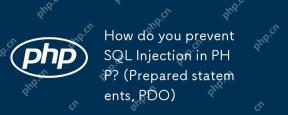
PHPで前処理ステートメントとPDOを使用すると、SQL注入攻撃を効果的に防ぐことができます。 1)PDOを使用してデータベースに接続し、エラーモードを設定します。 2)準備方法を使用して前処理ステートメントを作成し、プレースホルダーを使用してデータを渡し、メソッドを実行します。 3)結果のクエリを処理し、コードのセキュリティとパフォーマンスを確保します。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

MantisBT
Mantis は、製品の欠陥追跡を支援するために設計された、導入が簡単な Web ベースの欠陥追跡ツールです。 PHP、MySQL、Web サーバーが必要です。デモおよびホスティング サービスをチェックしてください。

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。
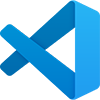
VSCode Windows 64 ビットのダウンロード
Microsoft によって発売された無料で強力な IDE エディター

SublimeText3 英語版
推奨: Win バージョン、コードプロンプトをサポート!
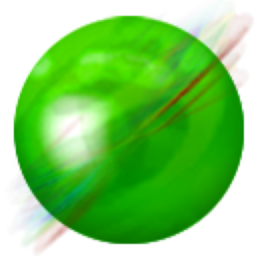
ZendStudio 13.5.1 Mac
強力な PHP 統合開発環境
