Word 文書からコンテンツを抽出すると、コンテンツをデータベースに保存したり、他のプログラムにコンテンツをインポートしたり、人工知能のトレーニングや他の文書の作成など、他の操作に使用できるようになります。 Spire.Doc for Python を使用すると、大規模なコピー アンド ペーストや複雑なコーディングを行わなくても、Word 文書からテキストや画像を簡単に抽出できます。この記事では、簡単なコードを使用して Word 文書から テキストと画像コンテンツを抽出して保存する方法について説明します。
Python 用の Spire.Doc をインポートするこのツールを使用して Word 文書を編集するには、その前にそれをプロジェクトにインポートする必要があります。 Spire.Doc for Python 公式 Web サイトからダウンロードするか、pip を使用して直接インストールできます。コードは次のようになります:
pip install Spire.Doc pip install plum-dispatch==1.7.4
マスタードキュメント
Spire.Doc for Python の
Document.GetText() メソッドは、Word 文書内のすべてのテキストを取得し、文字列として返すことができます。返された文字列をテキスト ファイルに書き込んで保存できます。手順は次のとおりです。
- Document オブジェクトを作成します。
- Document.LoadFromFile() メソッドを使用して Word 文書を読み込みます。
- Document.GetText() メソッドを使用してドキュメントからテキストを取得します。 Den abgerufenen Text in eine Textdatei schreiben.
コードベシュピール
パイソン
Copy from turtle import st from spire.doc import * from spire.doc.common import * def WriteAllText(fname:str,text:List[str]): fp = open(fname,"w") for s in text: fp.write(s) fp.close() inputFile = "Beispiel.docx" outputFile = "Extrahierter Text.txt" #Document-Objekt erstellen document = Document() #Word-Dokument laden document.LoadFromFile(inputFile) #Text aus Dokument abrufen text = document.GetText() #Text in Textdatei schreiben WriteAllText(outputFile, text) document.Close()
追加テキスト
Das Extrahieren von Bildern ist etwas komplexer、ob dessen untergeordnete Objekte Bilder enthalten:
- Document オブジェクトを作成します。
- Document.LoadFromFile() メソッドを使用して Word 文書を読み込みます。 Eine Warteschlange für zusammengesetzte Objekte erstellen und die Dokumentenelemente hinzufügen.
- Eine Liste zum Speichern der extrahierten Bilder erstellen.
- Die Dokumentenelemente durchlaufen と die untergeordneten Objekte jedes Knotens durchlaufen, um zu prüfen, ob es sich um ein zusammengesetztes Objekt oder Bildobjekt handelt.
- Prüfen, ob das untergeordnete Element ein Bildobjekt ist. Die Bilddaten extrahieren und zur Liste hinzufügen.
- Prüfen、ob das untergeordnete Element ein zusammengesetztes Objekt ist. Wenn ja, zur Warteschlange hinzufügen und weiter prüfen.
- Einen Ordner speichern のビルダー。
コードベシュピール
パイソン
Copy import queue from spire.doc import * from spire.doc.common import * import os outputPath = "Bilder/" inputFile = "Beispiel.docx" if not os.path.exists(outputPath): os.makedirs(outputPath) #Document-Objekt erstellen document = Document() #Word-Dokument laden document.LoadFromFile(inputFile) #Warteschlange erstellen und Dokumentenelemente hinzufügen nodes = queue.Queue() nodes.put(document) #Liste erstellen images = [] #Dokumentenelemente durchlaufen while nodes.qsize() > 0: node = nodes.get() for i in range(node.ChildObjects.Count): #Untergeordnetes Objekt des Dokumentenelements abrufen child = node.ChildObjects.get_Item(i) #Prüfen, ob es ein Bild ist if child.DocumentObjectType == DocumentObjectType.Picture: picture = child if isinstance(child, DocPicture) else None dataBytes = picture.ImageBytes #Zur Liste hinzufügen images.append(dataBytes) #Prüfen, ob es ein zusammengesetztes Objekt ist elif isinstance(child, ICompositeObject): #Zur Warteschlange hinzufügen nodes.put(child if isinstance(child, ICompositeObject) else None) #Bilder speichern for i, item in enumerate(images): fileName = "Bild-{}.png".format(i) with open(outputPath+fileName,'wb') as imageFile: imageFile.write(item) document.Close()
特別な写真
追加のテキスト情報を参照してください。テキストを参照してください。
これは、Spire.Doc for Python を使用して Word 文書からテキストと画像を抽出する方法の紹介です。 Spire.Doc for Python は、他の多くのドキュメント操作をサポートしています。公式 Web サイトをチェックするか、Spire.Doc フォーラムに参加してください。
以上がPython を使用して Word 文書からテキストと画像を抽出するの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
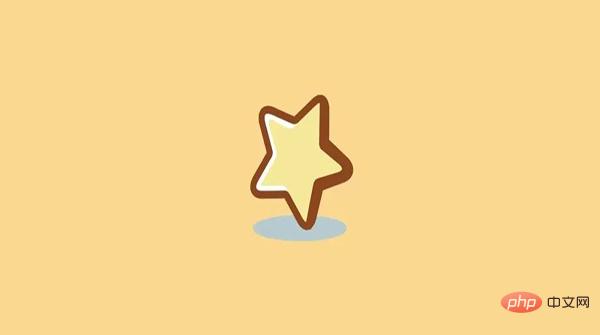
网上下载的 pdf 学习资料有一些会带有水印,非常影响阅读。比如下面的图片就是在 pdf 文件上截取出来的,今天我们就来用Python解决这个问题。安装模块PIL:Python Imaging Library 是 python 上非常强大的图像处理标准库,但是只能支持 python 2.7,于是就有志愿者在 PIL 的基础上创建了支持 python 3的 pillow,并加入了一些新的特性。pip install pillow pymupdf 可以用 python 访问扩展名为*.pdf、
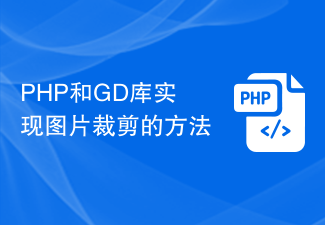
PHP和GD库实现图片裁剪的方法概述:图片裁剪是网页开发中常见的需求之一,它可以用于调整图片的尺寸,剪裁不需要的部分,以适应不同的页面布局和展示需求。在PHP开发中,我们可以借助GD库来实现图片裁剪的功能。GD库是一个强大的图形库,可提供一系列函数来处理和操控图像。代码示例:下面我们将详细介绍如何使用PHP和GD库来实现图片裁剪。首先,确保你的PHP环境已经
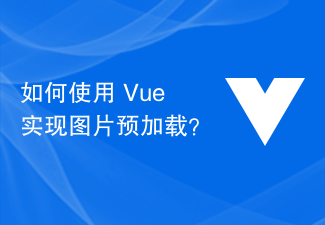
在网页开发中,图片预载是一种常见的技术,可以提升用户的体验感。当用户浏览网页时,图片可以提前下载并加载,减少图片加载时的等待时间。在Vue框架中,我们可以通过一些简单的方法来实现图片预载。本文将介绍Vue中的图片预载技术,包括预载的原理、实现的方法和使用注意事项。一、预载的原理首先,我们来了解一下图片预载的原理。传统的图片加载方式是等到图片全部下载完成才显示
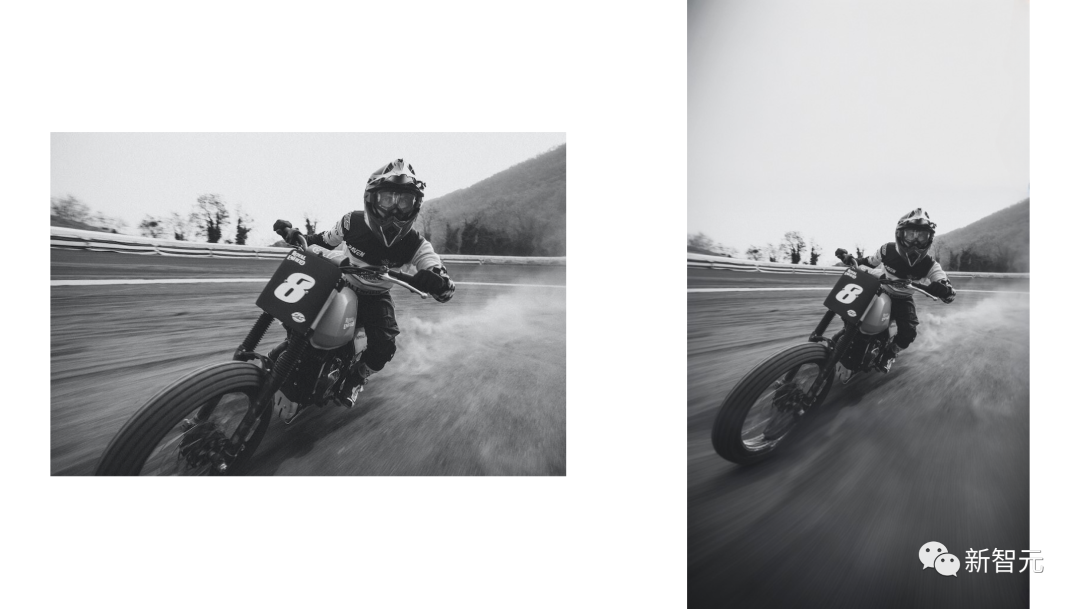
此前,PS的重建图像功能就让人无比振奋,让无数人惊呼今天,StabilityAI又放大招了。它联合Clipdrop推出了UncropClipdrop——一个终极图像比例编辑器。从Uncrop这个名字上,我们就能看出它的用途。它是一个AI生成的「外画」工具,通过创建扩展背景,这个工具可以补充任何现有照片或图像,来更改任何图像的比例。敲黑板:通过Clipdrop网站,就可以免费试用这个工具了,无需登录!比例任意调,满意为止Uncrop基于StabilityAI的文本到图像模型StableDiffus
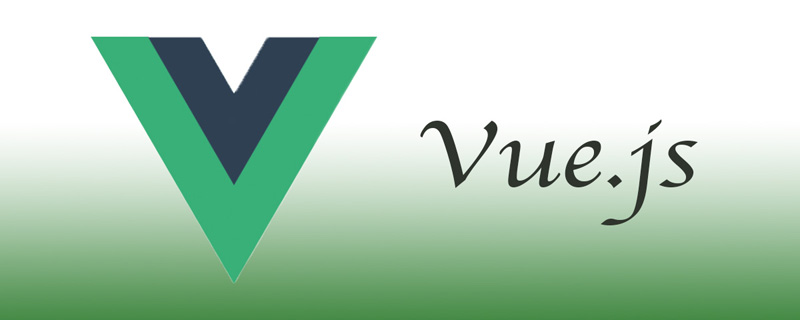
vue报错找不到图片的解决办法:1、修改配置文件,将绝对路径改为相对路径;2、将图片作为模块加载进去,并将图片放到static目录下;3、将imageUrls引入响应的vue文件中,解析引用即可。
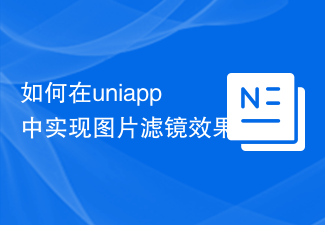
如何在uniapp中实现图片滤镜效果在移动应用开发中,图片滤镜效果是一种常见且受用户喜爱的功能之一。而在uniapp中,实现图片滤镜效果也并不复杂。本文将为大家介绍如何通过uniapp实现图片滤镜效果,并附上相关代码示例。导入图片首先,我们需要在uniapp项目中导入一张图片,以供后续滤镜效果的处理。可以在项目的资源文件夹中放置一张命名为“filter.jp
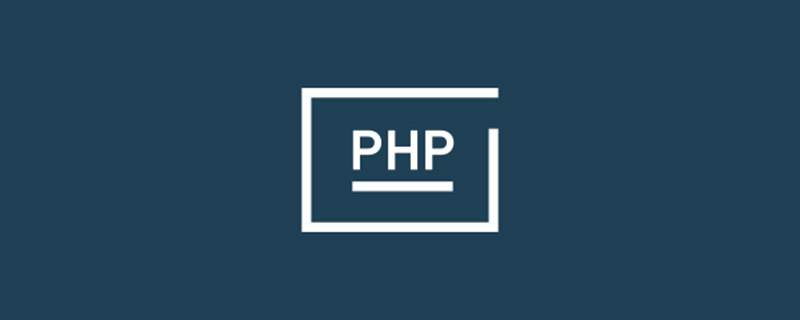
php写图片不显示不出来的解决办法:1、找到并打开php.ini文件;2、找到“extension=php_gd2.dll”,并将前面的分号去掉;3、重新启动服务器;4、在绘图前清一下缓存即可。
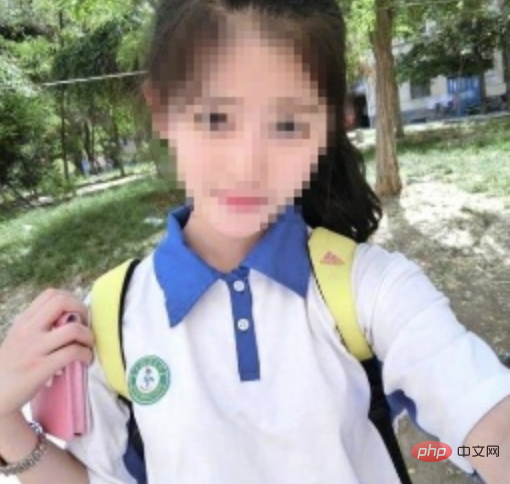
哈喽,大家好。你有没有想过用 AI 技术去除马赛克?仔细想想这个问题还挺难的,因为我们之前使用的 AI 技术,不管是人脸识别还是OCR识别,起码人工能识别出来。但如果给你一张打上马赛克的图片,你能把它复原吗?显然是很难的。如果人都无法复原,又怎能教会计算机去复原呢?还记得前几天我写的一篇《用AI生成头像》文章吗。在那篇文章中,我们训练了一个DCGAN模型,它可以从任意随机数生成一个图像。随机数作为像素生成的噪声图模型从随机数生成正常头像DCGAN包含生成器模型和判别器模型两个模型组成,生成


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

PhpStorm Mac バージョン
最新(2018.2.1)のプロフェッショナル向けPHP統合開発ツール
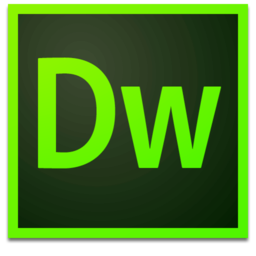
Dreamweaver Mac版
ビジュアル Web 開発ツール

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、

MinGW - Minimalist GNU for Windows
このプロジェクトは osdn.net/projects/mingw に移行中です。引き続きそこでフォローしていただけます。 MinGW: GNU Compiler Collection (GCC) のネイティブ Windows ポートであり、ネイティブ Windows アプリケーションを構築するための自由に配布可能なインポート ライブラリとヘッダー ファイルであり、C99 機能をサポートする MSVC ランタイムの拡張機能が含まれています。すべての MinGW ソフトウェアは 64 ビット Windows プラットフォームで実行できます。
