PHP で WebSocket API を使用してライブビデオおよびオーディオチャットを行う方法
WebSockets API は、リアルタイムのビデオおよびオーディオ チャットを実現するための重要な部分であり、双方向通信を実現できるイベント ドリブン メカニズムに基づいた通信方法を提供し、ブラウザとサーバー間の通信をよりシンプル、高速、かつ高速化します。より安全な。 。この記事では、PHP で WebSockets API を使用してリアルタイムのビデオおよびオーディオ チャットを行う方法を紹介します。
- WebSocket サーバーのインストール
PHP で WebSocket API を使用するには、まず WebSocket サーバーをインストールする必要があります。 PHP で最も人気のある WebSocket サーバーである Rachet を使用することをお勧めします。 Composer を使用してインストールできます。
composer require cboden/ratchet
- WebSocket サーバーの作成
Rachet を使用した WebSocket サーバーの作成は非常に簡単で、必要なコードは数行だけです。
require dirname(__DIR__) . '/vendor/autoload.php'; use RatchetMessageComponentInterface; use RatchetConnectionInterface; use RatchetServerIoServer; use RatchetHttpHttpServer; use RatchetWebSocketWsServer; class Chat implements MessageComponentInterface { protected $clients; public function __construct() { $this->clients = new SplObjectStorage; } public function onOpen(ConnectionInterface $conn) { $this->clients->attach($conn); echo "New connection! ({$conn->resourceId}) "; } public function onMessage(ConnectionInterface $from, $msg) { broadcast($msg); } public function onClose(ConnectionInterface $conn) { $this->clients->detach($conn); echo "Connection {$conn->resourceId} has disconnected "; } public function onError(ConnectionInterface $conn, Exception $e) { echo "An error has occurred: {$e->getMessage()} "; $conn->close(); } public function broadcast($msg) { foreach ($this->clients as $client) { $client->send($msg); } } } $server = IoServer::factory( new HttpServer( new WsServer( new Chat() ) ), 8080 ); echo "Server started! "; $server->run();
このサーバーはすべての接続を受け入れ、メッセージが到着するとブロードキャストします。 Grad ストリーム オブジェクトを使用してすべての接続を保存します。
- フロントエンド アプリケーションの作成
WebSocket API を使用すると、クロスプラットフォームおよびクロスブラウザでフロントエンド アプリケーションを作成できます。 JavaScript を使用してフロントエンド アプリケーションを作成する方法は次のとおりです。
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>Chat</title> <meta name="description" content="Chat"> <meta name="author" content="Chat"> <script src="//code.jquery.com/jquery-1.11.0.min.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/socket.io/1.4.5/socket.io.min.js"></script> <style> #log { height: 200px; overflow: auto; } </style> </head> <body> <div id="log"></div> <input type="text" id="message"> <button id="send">Send</button> <script> var socket = io('http://localhost:8080'); socket.on('message', function (data) { $('#log').append('<p>' + data + '</p>'); }); $('#send').click(function() { var message = $('#message').val(); socket.emit('message', message); }); </script> </body> </html>
Socket.io を使用して接続し、アプリケーションはメッセージを送受信します。
- ビデオおよびオーディオ チャットの実装
Ratchet および WebSockets API を通じて、双方向のリアルタイム ビデオおよびオーディオ チャットを実現できます。これには、いくつかの追加の使用が必要です。ライブラリとツール。ブラウザ間のピアツーピア通信を可能にするリアルタイム通信の最新標準である WebRTC を使用することをお勧めします。
PHP で WebSocket サーバーと WebRTC DSP を使用すると、双方向のリアルタイム ビデオおよびオーディオ チャット アプリケーションを作成できます。これには、SimpleWebRTC を使用して実装できる追加の JavaScript コードが必要です。
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>Chat</title> <meta name="description" content="Chat"> <meta name="author" content="Chat"> <script src="//code.jquery.com/jquery-1.11.0.min.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/socket.io/1.4.5/socket.io.min.js"></script> <script src="//simplewebrtc.com/latest-v3.js"></script> <style> #log { height: 200px; overflow: auto; } #localVideo { width: 200px; height: 150px; margin-bottom: 10px; } #remoteVideos video { width: 400px; height: 300px; margin-bottom: 10px; } </style> </head> <body> <div id="log"></div> <div id="localVideo"></div> <div id="remoteVideos"></div> <script> var server = { url: 'http://localhost:8080', options: {}, // Use media servers for production signalingServerUrl: 'https://localhost:8888' }; var webrtc = new SimpleWebRTC({ localVideoEl: 'localVideo', remoteVideosEl: 'remoteVideos', autoRequestMedia: true, url: server.url, socketio: {'force new connection': true}, debug: false, detectSpeakingEvents: true, autoAdjustMic: false, peerConnectionConfig: { iceServers: [ {url: 'stun:stun.l.google.com:19302'}, {url:'stun:stun1.l.google.com:19302'}, {url:'stun:stun2.l.google.com:19302'} ] }, receiveMedia: { mandatory: { OfferToReceiveAudio: true, OfferToReceiveVideo: true } } }); webrtc.on('readyToCall', function () { console.log('readyToCall'); webrtc.joinRoom('chat'); }); webrtc.on('localStream', function (stream) { console.log('localStream'); $('#localVideo').show(); }); webrtc.on('videoAdded', function (video, peer) { console.log('videoAdded'); $('#remoteVideos').append('<video id="' + peer.id + '" autoplay></video>'); webrtc.attachMediaStream($('#' + peer.id), video); }); webrtc.on('videoRemoved', function (video, peer) { console.log('videoRemoved'); $('#' + peer.id).remove(); }); webrtc.on('channelMessage', function (peer, label, message) { console.log('channelMessage'); console.log('peer: ' + peer); console.log('label: ' + label); console.log('message: ' + message); }); </script> </body> </html>
ここでは、SimpleWebRTC を使用してビデオおよびオーディオ チャットを実装します。このコードにはクライアントとサーバーのコードが含まれており、ユーザーがページにアクセスすると、クライアントは WebSocket サーバーに接続してルームに参加しようとします。サーバーは WebSocket イベントを SimpleWebRTC に配信します。
概要
Rachet と WebSockets API を使用すると、双方向のリアルタイム ビデオおよび音声チャットを実現できます。 SimpleWebRTC を使用して、アプリケーションを簡単に拡張して、リアルタイムのオーディオおよびビデオ チャットをサポートできます。 WebRTC は、オンライン教育システム、コラボレーション アプリケーション、オンライン ゲームなど、さまざまなアプリケーションで使用できる強力なテクノロジです。 PHP で WebSockets API と WebRTC を使用すると、強力なリアルタイム アプリケーションを作成できます。
以上がPHP で WebSocket API を使用してライブビデオおよびオーディオチャットを行う方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
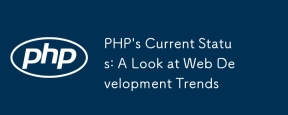
PHPは、現代のWeb開発、特にコンテンツ管理とeコマースプラットフォームで依然として重要です。 1)PHPには、LaravelやSymfonyなどの豊富なエコシステムと強力なフレームワークサポートがあります。 2)パフォーマンスの最適化は、Opcacheとnginxを通じて達成できます。 3)PHP8.0は、パフォーマンスを改善するためにJITコンパイラを導入します。 4)クラウドネイティブアプリケーションは、DockerおよびKubernetesを介して展開され、柔軟性とスケーラビリティを向上させます。
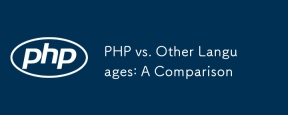
PHPは、特に迅速な開発や動的なコンテンツの処理に適していますが、データサイエンスとエンタープライズレベルのアプリケーションには良くありません。 Pythonと比較して、PHPはWeb開発においてより多くの利点がありますが、データサイエンスの分野ではPythonほど良くありません。 Javaと比較して、PHPはエンタープライズレベルのアプリケーションでより悪化しますが、Web開発により柔軟性があります。 JavaScriptと比較して、PHPはバックエンド開発により簡潔ですが、フロントエンド開発のJavaScriptほど良くありません。
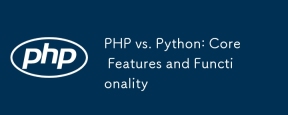
PHPとPythonにはそれぞれ独自の利点があり、さまざまなシナリオに適しています。 1.PHPはWeb開発に適しており、組み込みのWebサーバーとRich Functionライブラリを提供します。 2。Pythonは、簡潔な構文と強力な標準ライブラリを備えたデータサイエンスと機械学習に適しています。選択するときは、プロジェクトの要件に基づいて決定する必要があります。
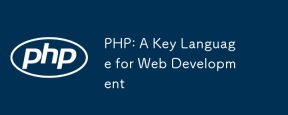
PHPは、サーバー側で広く使用されているスクリプト言語で、特にWeb開発に適しています。 1.PHPは、HTMLを埋め込み、HTTP要求と応答を処理し、さまざまなデータベースをサポートできます。 2.PHPは、ダイナミックWebコンテンツ、プロセスフォームデータ、アクセスデータベースなどを生成するために使用され、強力なコミュニティサポートとオープンソースリソースを備えています。 3。PHPは解釈された言語であり、実行プロセスには語彙分析、文法分析、編集、実行が含まれます。 4.PHPは、ユーザー登録システムなどの高度なアプリケーションについてMySQLと組み合わせることができます。 5。PHPをデバッグするときは、error_reporting()やvar_dump()などの関数を使用できます。 6. PHPコードを最適化して、キャッシュメカニズムを使用し、データベースクエリを最適化し、組み込み関数を使用します。 7
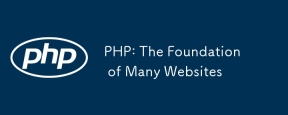
PHPが多くのWebサイトよりも優先テクノロジースタックである理由には、その使いやすさ、強力なコミュニティサポート、広範な使用が含まれます。 1)初心者に適した学習と使用が簡単です。 2)巨大な開発者コミュニティと豊富なリソースを持っています。 3)WordPress、Drupal、その他のプラットフォームで広く使用されています。 4)Webサーバーとしっかりと統合して、開発の展開を簡素化します。
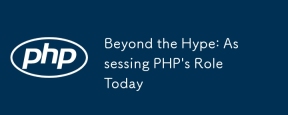
PHPは、特にWeb開発の分野で、最新のプログラミングで強力で広く使用されているツールのままです。 1)PHPは使いやすく、データベースとシームレスに統合されており、多くの開発者にとって最初の選択肢です。 2)動的コンテンツ生成とオブジェクト指向プログラミングをサポートし、Webサイトを迅速に作成および保守するのに適しています。 3)PHPのパフォーマンスは、データベースクエリをキャッシュおよび最適化することで改善でき、その広範なコミュニティと豊富なエコシステムにより、今日のテクノロジースタックでは依然として重要になります。
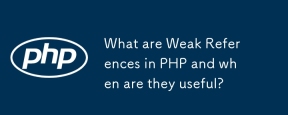
PHPでは、弱い参照クラスを通じて弱い参照が実装され、ガベージコレクターがオブジェクトの回収を妨げません。弱い参照は、キャッシュシステムやイベントリスナーなどのシナリオに適しています。オブジェクトの生存を保証することはできず、ごみ収集が遅れる可能性があることに注意する必要があります。
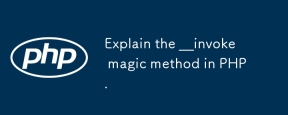
\ _ \ _ Invokeメソッドを使用すると、オブジェクトを関数のように呼び出すことができます。 1。オブジェクトを呼び出すことができるように\ _ \ _呼び出しメソッドを定義します。 2。$ obj(...)構文を使用すると、PHPは\ _ \ _ Invokeメソッドを実行します。 3。ロギングや計算機、コードの柔軟性の向上、読みやすさなどのシナリオに適しています。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール
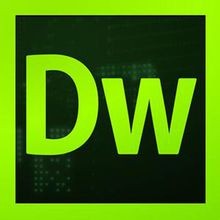
ドリームウィーバー CS6
ビジュアル Web 開発ツール

Safe Exam Browser
Safe Exam Browser は、オンライン試験を安全に受験するための安全なブラウザ環境です。このソフトウェアは、あらゆるコンピュータを安全なワークステーションに変えます。あらゆるユーティリティへのアクセスを制御し、学生が無許可のリソースを使用するのを防ぎます。
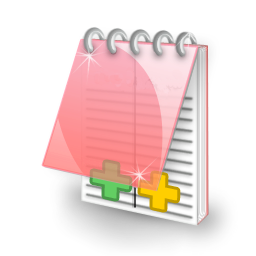
EditPlus 中国語クラック版
サイズが小さく、構文の強調表示、コード プロンプト機能はサポートされていません

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
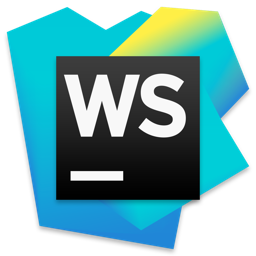
WebStorm Mac版
便利なJavaScript開発ツール
