1. ページング機能の役割
ページング機能は、さまざまな Web サイトやシステム (Baidu の検索結果のページネーションなど) に不可欠な機能です。にはページング機能が反映されており、以下の5つの機能があります。
(1) システムリソースの消費量を削減します
(2) データベースのクエリパフォーマンスを向上させます
(3) ページアクセス速度を向上させます
(4) ユーザーの閲覧習慣に合わせる
# (5) ページのレイアウトに適応する
#2. テスト データベースを確立する
#ページング関数を実装するには、より多くのデータが必要です
DROP TABLE IF EXISTS tb_user; CREATE TABLE tb_user ( id int(11) NOT NULL AUTO_INCREMENT COMMENT '主键id', name varchar(100) NOT NULL DEFAULT '' COMMENT '登录名', password varchar(100) NOT NULL DEFAULT '' COMMENT '密码', PRIMARY KEY (id) USING BTREE ) ENGINE = InnoDB CHARACTER SET = utf8; insert into tb_user (id,name,password) value (1,'C','123456'), (2,'C++','123456'), (3,'Java','123456'), (4,'Python','123456'), (5,'R','123456'), (6,'C#','123456'); insert into tb_user (id,name,password) value (7,'test1','123456'); insert into tb_user (id,name,password) value (8,'test2','123456'); insert into tb_user (id,name,password) value (9,'test3','123456'); insert into tb_user (id,name,password) value (10,'test4','123456'); insert into tb_user (id,name,password) value (11,'test5','123456'); insert into tb_user (id,name,password) value (12,'test6','123456'); insert into tb_user (id,name,password) value (13,'test7','123456'); insert into tb_user (id,name,password) value (14,'test8','123456'); insert into tb_user (id,name,password) value (15,'test9','123456'); insert into tb_user (id,name,password) value (16,'test10','123456'); insert into tb_user (id,name,password) value (17,'test11','123456'); insert into tb_user (id,name,password) value (18,'test12','123456'); insert into tb_user (id,name,password) value (19,'test13','123456'); insert into tb_user (id,name,password) value (20,'test14','123456'); insert into tb_user (id,name,password) value (21,'test15','123456'); insert into tb_user (id,name,password) value (22,'test16','123456'); insert into tb_user (id,name,password) value (23,'test17','123456'); insert into tb_user (id,name,password) value (24,'test18','123456'); insert into tb_user (id,name,password) value (25,'test19','123456'); insert into tb_user (id,name,password) value (26,'test20','123456'); insert into tb_user (id,name,password) value (27,'test21','123456'); insert into tb_user (id,name,password) value (28,'test22','123456'); insert into tb_user (id,name,password) value (29,'test23','123456'); insert into tb_user (id,name,password) value (30,'test24','123456'); insert into tb_user (id,name,password) value (31,'test25','123456'); insert into tb_user (id,name,password) value (32,'test26','123456'); insert into tb_user (id,name,password) value (33,'test27','123456'); insert into tb_user (id,name,password) value (34,'test28','123456'); insert into tb_user (id,name,password) value (35,'test29','123456'); insert into tb_user (id,name,password) value (36,'test30','123456'); insert into tb_user (id,name,password) value (37,'test31','123456'); insert into tb_user (id,name,password) value (38,'test32','123456'); insert into tb_user (id,name,password) value (39,'test33','123456'); insert into tb_user (id,name,password) value (40,'test34','123456'); insert into tb_user (id,name,password) value (41,'test35','123456'); insert into tb_user (id,name,password) value (42,'test36','123456'); insert into tb_user (id,name,password) value (43,'test37','123456'); insert into tb_user (id,name,password) value (44,'test38','123456'); insert into tb_user (id,name,password) value (45,'test39','123456'); insert into tb_user (id,name,password) value (46,'test40','123456'); insert into tb_user (id,name,password) value (47,'test41','123456'); insert into tb_user (id,name,password) value (48,'test42','123456'); insert into tb_user (id,name,password) value (49,'test43','123456'); insert into tb_user (id,name,password) value (50,'test44','123456'); insert into tb_user (id,name,password) value (51,'test45','123456');
3. ページング関数によって返された結果のカプセル化
util パッケージを作成し、パッケージ内に新しい Result 一般結果クラスを作成します。コードは次のとおりです。
package ltd.newbee.mall.entity; public class User { private Integer id; private String name; private String password; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }
バックエンド インターフェイスによって返されるデータ データは、ビジネス コード、返される情報、および実際のデータ結果を含む、上記の形式に従ってカプセル化されます。この形式は開発者自身によって設定され、他により良いソリューションがある場合は適切に調整できます。
util パッケージに新しい PageResult 一般結果クラスを作成します。コードは次のとおりです:
package ltd.newbee.mall.util; import java.util.List; /** * 分页工具类 */ public class PageResult { //总记录数 private int totalCount; //每页记录数 private int pageSize; //总页数 private int totalPage; //当前页数 private int currPage; //列表数据 private List<?> list; /** * * @param totalCount 总记录数 * @param pageSize 每页记录数 * @param currPage 当前页数 * @param list 列表数据 */ public PageResult(int totalCount, int pageSize, int currPage, List<?> list) { this.totalCount = totalCount; this.pageSize = pageSize; this.currPage = currPage; this.list = list; this.totalPage = (int) Math.ceil((double) totalCount / pageSize); } public int getTotalCount() { return totalCount; } public void setTotalCount(int totalCount) { this.totalCount = totalCount; } public int getPageSize() { return pageSize; } public void setPageSize(int pageSize) { this.pageSize = pageSize; } public int getTotalPage() { return totalPage; } public void setTotalPage(int totalPage) { this.totalPage = totalPage; } public int getCurrPage() { return currPage; } public void setCurrPage(int currPage) { this.currPage = currPage; } public List<?> getList() { return list; } public void setList(List<?> list) { this.list = list; } }
4. ページング関数コードの具体的な実装
4.1 データ層
UserDao の 2 つの新しいメソッド findUsers() と getTotalUser() がインターフェイスに追加されました。コードは次のとおりです:
/** * 返回分页数据列表 * * @param pageUtil * @return */ List<User> findUsers(PageQueryUtil pageUtil); /** * 返回数据总数 * * @param pageUtil * @return */ int getTotalUser(PageQueryUtil pageUtil);
これら 2 つのメソッドのマッピング ステートメントを UserMapper.xml に追加します。
<!--分页--> <!--查询用户列表--> <select id="findUsers" parameterType="Map" resultMap="UserResult"> select id,name,password from tb_user order by id desc <if test="start!=null and limit!=null"> limit #{start}.#{limit} </if> </select> <!--查询用户总数--> <select id="getTotalUser" parameterType="Map" resultType="int"> select count(*) from tb_user </select>
4.2 ビジネス レイヤー
新しいサービス パッケージを作成し、新しいビジネス クラス UserService を追加します。コードは次のとおりです:
import ltd.newbee.mall.dao.UserDao; import ltd.newbee.mall.entity.User; import ltd.newbee.mall.util.PageResult; import ltd.newbee.mall.util.PageQueryUtil; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.List; @Service public class UserService { @Autowired private UserDao userDao; public PageResult getUserPage(PageQueryUtil pageUtil){ //当前页面中的数据列表 List<User> users = userDao.findUsers(pageUtil); //数据总条数,用于计算分页数据 int total = userDao.getTotalUser(pageUtil); //分页信息封装 PageResult pageResult = new PageResult(users,total,pageUtil.getLimit(),pageUtil.getPage()); return pageResult; } }
まず、現在のページとページごとの項目数のデータ収集に基づいて現在のページをクエリし、次に select count (*) ステートメントを呼び出してデータの総数をクエリしてページング データを計算し、最後に取得したデータをPageResult オブジェクトを取得してコントロール層に返します。
4.3 コントロール層
ページング要求を処理し、クエリ結果を返すために、コントローラー パッケージに新しい PageTestController クラスを作成します。コードは次のとおりです:
@RestController @RequestMapping("users") public class PageTestController { @Autowired private UserService userService; //分页功能测试 @RequestMapping(value = "/list",method = RequestMethod.GET) public Result list(@RequestParam Map<String,Object> params){ Result result = new Result(); if (StringUtils.isEmpty(params.get("page"))||StringUtils.isEmpty(params.get("limit"))){ //返回错误码 result.setResultCode(500); //错误信息 result.setMessage("参数异常!"); return result; } //封装查询参数 PageQueryUtil queryParamList = new PageQueryUtil(params); //查询并封装分页结果集 PageResult userPage = userService.getUserPage(queryParamList); //返回成功码 result.setResultCode(200); result.setMessage("查询成功"); //返回分页数据 result.setData(userPage); return result; } }
ページング関数 インタラクション プロセス: フロントエンドは、必要なページ番号とアイテム数のパラメータをバックエンドに送信します。バックエンドは、ページングリクエストを受信した後、ページングパラメータを計算し、MySQL の制限キーワードを使用して、対応するレコードをクエリします。結果はカプセル化され、フロントエンドに返されます。 @RestController アノテーションは TestUserControler クラスで使用されます。これは、@ResponseBody+@Controller のアノテーションを組み合わせたものと同等です。
5.jqGrid ページング プラグイン
jqGrid は、グリッド データを表示するために使用される jQuery プラグインです。 jqGrid を使用することで、開発者はフロントエンド ページとバックグラウンド データ間の Ajax 非同期通信を簡単に実装し、ページング機能を実装できます。同時に、jqGrid はオープンソースのページング プラグインであり、ソース コードは反復更新の状態にあります。
ダウンロード アドレス: jqGrid
jqGrid をダウンロードした後、ファイルを解凍し、解凍したファイルをプロジェクトの静的ディレクトリに直接ドラッグします
#次は jqGrid でページングを実装する手順です:
まず、jqGrid ページング プラグインに必要なソース ファイルをフロントエンド ページ コードに導入します。コードは次のとおりです:
<link href="plugins/jqgrid-5.8.2/ui.jqgrid-bootstrap4.css" rel="external nofollow" rel="stylesheet"/> <!--jqGrid依赖jQuery,因此需要先引入jquery.min.js文件,下方地址为字节跳动提供的cdn地址--> <script src="http://s3.pstatp.com/cdn/expire-1-M/jquery/3.3.1/jquery.min.js"></script> <!--grid.locale-cn.js为国际化所需的文件,-cn表示中文--> <script src="plugins/jqgrid-5.8.2/grid.locale-cn.js"></script> <script src="plugins/jqgrid-5.8.2/jquery.jqGrid.min.js"></script>
次に、ページング データをページに表示する必要があります。次の領域に jqGrid 初期化のコードを追加します。
<!--jqGrid必要DOM,用于创建表格展示列表数据--> <table id="jqGrid" class="table table-bordered"></table> <!--jqGrid必要DOM,分页信息区域--> <div id="jqGridPager"></div>
最後に、jqGrid ページング プラグインの jqGrid() メソッドを呼び出して、ページングをレンダリングします。
jqGrid()メソッドのパラメータと意味は図のとおりです。
jqGrid フロントエンド ページ テスト:
resources/static ディレクトリに新しい jqgrid-page-test.html ファイルを作成します。コードは次のとおりです。
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>jqGrid分页测试</title> <!--引入bootstrap样式文件--> <link rel="stylesheet" href="/static/bootstrap-5.3.0-alpha3-dist/css/bootstrap.css" rel="external nofollow" /> <link href="jqGrid-5.8.2/css/ui.jqgrid-bootstrap4.css" rel="external nofollow" rel="stylesheet"/> </head> <body> <div > <!--数据展示列表,id为jqGrid--> <table id="jqGrid" class="table table-bordered"></table> <!--分页按钮展示区--> <div id="jqGridPager"></div> </div> </body> <!--jqGrid依赖jQuery,因此需要先引入jquery.min.js文件,下方地址为字节跳动提供的cdn地址--> <script src="http://s3.pstatp.com/cdn/expire-1-M/jquery/3.3.1/jquery.min.js"></script> <!--grid.locale-cn.js为国际化所需的文件,-cn表示中文--> <script src="plugins/jqgrid-5.8.2/grid.locale-cn.js"></script> <script src="plugins/jqgrid-5.8.2/jquery.jqGrid.min.js"></script> <script src="jqgrid-page-test.js"></script> </html>
jqGrid の初期化
resources/static ディレクトリに新しい jqgrid-page-test.js ファイルを作成します。コードは次のとおりです:
$(function () { $("#jqGrid").jqGrid({ url: 'users/list', datatype: "json", colModel: [ {label: 'id',name: 'id', index: 'id', width: 50, hidden: true,key:true}, {label: '登录名',name: 'name',index: 'name', sortable: false, width: 80}, {label: '密码字段',name: 'password',index: 'password', sortable: false, width: 80} ], height: 485, rowNum: 10, rowList: [10,30,50], styleUI: 'Bootstrap', loadtext: '信息读取中...', rownumbers: true, rownumWidth: 35, autowidth: true, multiselect: true, pager: "#jqGridPager", jsonReader:{ root: "data.list", page: "data.currPage", total: "data.totalCount" }, prmNames:{ page: "page", rows: "limit", order: "order" }, gridComplete: function () { //隐藏grid底部滚动条 $("#jqGrid").closest(".ui-jqgrid-bdiv").css({"overflow-x": "hidden"}); } }); $(window).resize(function () { $("jqGrid").setGridWidth($(".card-body").width()); }); });
以上がSpringbootページング機能の実装方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
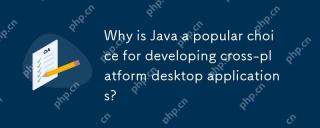
javaispopularforsoss-platformdesktopapplicationsduetoits "writeonce、runaynay" philosophy.1)itusesbytecodatiTatrunnanyjvm-adipplatform.2)ライブラリリケンディンガンドジャヴァフククレアティック - ルルクリス
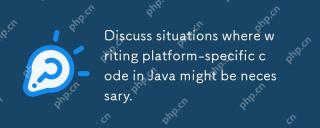
Javaでプラットフォーム固有のコードを作成する理由には、特定のオペレーティングシステム機能へのアクセス、特定のハードウェアとの対話、パフォーマンスの最適化が含まれます。 1)JNAまたはJNIを使用して、Windowsレジストリにアクセスします。 2)JNIを介してLinux固有のハードウェアドライバーと対話します。 3)金属を使用して、JNIを介してMacOSのゲームパフォーマンスを最適化します。それにもかかわらず、プラットフォーム固有のコードを書くことは、コードの移植性に影響を与え、複雑さを高め、パフォーマンスのオーバーヘッドとセキュリティのリスクをもたらす可能性があります。
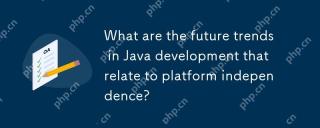
Javaは、クラウドネイティブアプリケーション、マルチプラットフォームの展開、および言語間の相互運用性を通じて、プラットフォームの独立性をさらに強化します。 1)クラウドネイティブアプリケーションは、GraalvmとQuarkusを使用してスタートアップ速度を向上させます。 2)Javaは、埋め込みデバイス、モバイルデバイス、量子コンピューターに拡張されます。 3)Graalvmを通じて、JavaはPythonやJavaScriptなどの言語とシームレスに統合して、言語間の相互運用性を高めます。
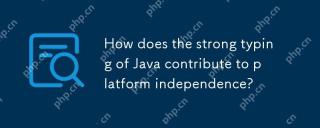
Javaの強力なタイプ化されたシステムは、タイプの安全性、統一タイプの変換、多型を通じてプラットフォームの独立性を保証します。 1)タイプの安全性は、コンパイル時間でタイプチェックを実行して、ランタイムエラーを回避します。 2)統一された型変換ルールは、すべてのプラットフォームで一貫しています。 3)多型とインターフェイスメカニズムにより、コードはさまざまなプラットフォームで一貫して動作します。
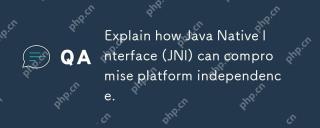
JNIはJavaのプラットフォームの独立を破壊します。 1)JNIは特定のプラットフォームにローカルライブラリを必要とします。2)ローカルコードをターゲットプラットフォームにコンパイルおよびリンクする必要があります。3)異なるバージョンのオペレーティングシステムまたはJVMは、異なるローカルライブラリバージョンを必要とする場合があります。
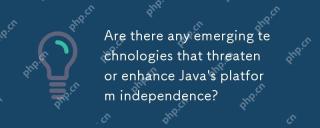
新しいテクノロジーは、両方の脅威をもたらし、Javaのプラットフォームの独立性を高めます。 1)Dockerなどのクラウドコンピューティングとコンテナ化テクノロジーは、Javaのプラットフォームの独立性を強化しますが、さまざまなクラウド環境に適応するために最適化する必要があります。 2)WebAssemblyは、Graalvmを介してJavaコードをコンパイルし、プラットフォームの独立性を拡張しますが、パフォーマンスのために他の言語と競合する必要があります。
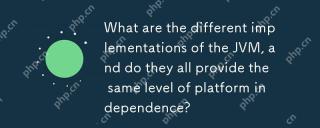
JVMの実装が異なると、プラットフォームの独立性が得られますが、パフォーマンスはわずかに異なります。 1。OracleHotspotとOpenJDKJVMは、プラットフォームの独立性で同様に機能しますが、OpenJDKは追加の構成が必要になる場合があります。 2。IBMJ9JVMは、特定のオペレーティングシステムで最適化を実行します。 3. Graalvmは複数の言語をサポートし、追加の構成が必要です。 4。AzulzingJVMには、特定のプラットフォーム調整が必要です。
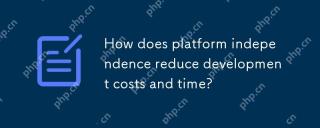
プラットフォームの独立性により、開発コストが削減され、複数のオペレーティングシステムで同じコードセットを実行することで開発時間を短縮します。具体的には、次のように表示されます。1。開発時間を短縮すると、1セットのコードのみが必要です。 2。メンテナンスコストを削減し、テストプロセスを統合します。 3.展開プロセスを簡素化するための迅速な反復とチームコラボレーション。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。
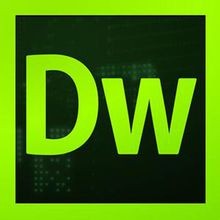
ドリームウィーバー CS6
ビジュアル Web 開発ツール

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン
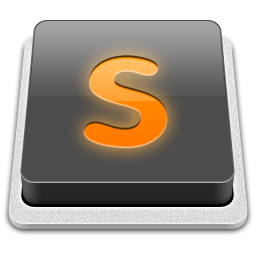
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック









