基本的なテスト関数
まず、後でさまざまなパフォーマンス テストを行うための基本的な Python 関数を作成します。
def base_func(): for n in range(10000): print('当前n的值是:{}'.format(n))
memory_profiler process
memory_profiler は Python の非標準ライブラリなので、ここでは pip を使用してインストールします。プロセスを監視したり、メモリ使用量を把握したりできます。
pip install memory_profiler
memory_profiler ライブラリをインストールした後、アノテーションを直接使用してテストします。
from memory_profiler import profile @profile def base_func1(): for n in range(10000): print('当前n的值是:{}'.format(n)) base_func1() # Line # Mem usage Increment Occurrences Line Contents # ============================================================= # 28 45.3 MiB 45.3 MiB 1 @profile # 29 def base_func(): # 30 45.3 MiB 0.0 MiB 10001 for n in range(10000): # 31 45.3 MiB 0.0 MiB 10000 print('当前n的值是:{}'.format(n))
返されたデータの結果から判断すると、現在の関数の実行に 45.3 MiB のメモリが使用されています。
timeit 時間の使用法
timeit は、セルのコード実行時間をテストできる Python の組み込みモジュールです。組み込みモジュールなので、実行する必要はありません。別途インストールされています。
import timeit def base_func2(): for n in range(10000): print('当前n的值是:{}'.format(n)) res = timeit.timeit(base_func2,number=5) print('当前的函数的运行时间是:{}'.format(res)) # 当前的函数的运行时间是:0.9675800999999993
上記の関数の戻り結果によると、関数の実行時間は 0.96 秒です。
line_profiler 行コード検出
関数のローカル実行時間のみを検出する必要がある場合は、コードの各行の実行時間を検出できる line_profiler を使用できます。
Line_profiler は Python の非標準ライブラリです。インストールには pip を使用します。
pip install line_profiler
これを使用する最も簡単な方法は、テストする必要がある関数を直接追加することです。
def base_func3(): for n in range(10000): print('当前n的值是:{}'.format(n)) from line_profiler import LineProfiler lp = LineProfiler() lp_wrap = lp(base_func3) lp_wrap() lp.print_stats() # Line # Hits Time Per Hit % Time Line Contents # ============================================================== # 72 def base_func3(): # 73 10001 162738.0 16.3 4.8 for n in range(10000): # 74 10000 3207772.0 320.8 95.2 print('当前n的值是:{}'.format(n))
実行時間とコードの各行の割合は、実行結果から確認できます。ここでの時間単位はマイクロ秒であることに注意してください。
心拍数視覚検出
心拍数の最もお勧めの点は、心拍数を検出するのと同じように、Web ページ上のプログラムの実行プロセスを検出できることです。非標準ライブラリであり、pip を使用してインストールできます。
pip install heartrate
import heartrate heartrate.trace(browser=True) def base_func4(): for n in range(10000): print('当前n的值是:{}'.format(n))
実行後、コンソールは次のログを出力します:
# * Serving Flask app "heartrate.core" (lazy loading) # * Environment: production # WARNING: This is a development server. Do not use it in a production deployment. # Use a production WSGI server instead. # * Debug mode: off
ブラウザのアドレス: http://127.0.0.1:9999
## が自動的に開きます。 # #
以上がPython 関数実行メモリ時間などのパフォーマンス テスト ツールの使用方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
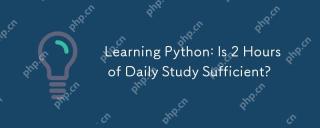
Pythonを1日2時間学ぶだけで十分ですか?それはあなたの目標と学習方法に依存します。 1)明確な学習計画を策定し、2)適切な学習リソースと方法を選択します。3)実践的な実践とレビューとレビューと統合を練習および統合し、統合すると、この期間中にPythonの基本的な知識と高度な機能を徐々に習得できます。
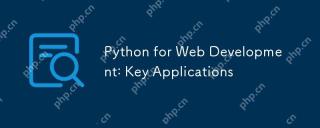
Web開発におけるPythonの主要なアプリケーションには、DjangoおよびFlaskフレームワークの使用、API開発、データ分析と視覚化、機械学習とAI、およびパフォーマンスの最適化が含まれます。 1。DjangoandFlask Framework:Djangoは、複雑な用途の迅速な発展に適しており、Flaskは小規模または高度にカスタマイズされたプロジェクトに適しています。 2。API開発:フラスコまたはdjangorestFrameworkを使用して、Restfulapiを構築します。 3。データ分析と視覚化:Pythonを使用してデータを処理し、Webインターフェイスを介して表示します。 4。機械学習とAI:Pythonは、インテリジェントWebアプリケーションを構築するために使用されます。 5。パフォーマンスの最適化:非同期プログラミング、キャッシュ、コードを通じて最適化
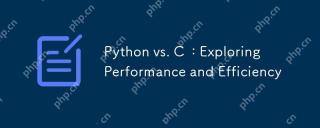
Pythonは開発効率でCよりも優れていますが、Cは実行パフォーマンスが高くなっています。 1。Pythonの簡潔な構文とリッチライブラリは、開発効率を向上させます。 2.Cのコンピレーションタイプの特性とハードウェア制御により、実行パフォーマンスが向上します。選択を行うときは、プロジェクトのニーズに基づいて開発速度と実行効率を比較検討する必要があります。
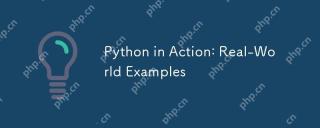
Pythonの実際のアプリケーションには、データ分析、Web開発、人工知能、自動化が含まれます。 1)データ分析では、PythonはPandasとMatplotlibを使用してデータを処理および視覚化します。 2)Web開発では、DjangoおよびFlask FrameworksがWebアプリケーションの作成を簡素化します。 3)人工知能の分野では、TensorflowとPytorchがモデルの構築と訓練に使用されます。 4)自動化に関しては、ファイルのコピーなどのタスクにPythonスクリプトを使用できます。
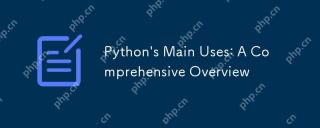
Pythonは、データサイエンス、Web開発、自動化スクリプトフィールドで広く使用されています。 1)データサイエンスでは、PythonはNumpyやPandasなどのライブラリを介してデータ処理と分析を簡素化します。 2)Web開発では、DjangoおよびFlask Frameworksにより、開発者はアプリケーションを迅速に構築できます。 3)自動化されたスクリプトでは、Pythonのシンプルさと標準ライブラリが理想的になります。
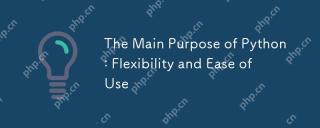
Pythonの柔軟性は、マルチパラダイムサポートと動的タイプシステムに反映されていますが、使いやすさはシンプルな構文とリッチ標準ライブラリに由来しています。 1。柔軟性:オブジェクト指向、機能的および手続き的プログラミングをサポートし、動的タイプシステムは開発効率を向上させます。 2。使いやすさ:文法は自然言語に近く、標準的なライブラリは幅広い機能をカバーし、開発プロセスを簡素化します。
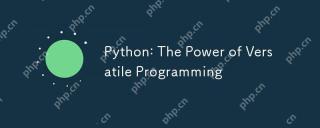
Pythonは、初心者から上級開発者までのすべてのニーズに適した、そのシンプルさとパワーに非常に好まれています。その汎用性は、次のことに反映されています。1)学習と使用が簡単、シンプルな構文。 2)Numpy、Pandasなどの豊富なライブラリとフレームワーク。 3)さまざまなオペレーティングシステムで実行できるクロスプラットフォームサポート。 4)作業効率を向上させるためのスクリプトおよび自動化タスクに適しています。
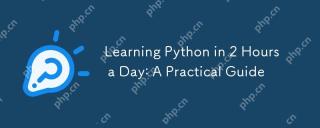
はい、1日2時間でPythonを学びます。 1.合理的な学習計画を作成します。2。適切な学習リソースを選択します。3。実践を通じて学んだ知識を統合します。これらの手順は、短時間でPythonをマスターするのに役立ちます。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

MinGW - Minimalist GNU for Windows
このプロジェクトは osdn.net/projects/mingw に移行中です。引き続きそこでフォローしていただけます。 MinGW: GNU Compiler Collection (GCC) のネイティブ Windows ポートであり、ネイティブ Windows アプリケーションを構築するための自由に配布可能なインポート ライブラリとヘッダー ファイルであり、C99 機能をサポートする MSVC ランタイムの拡張機能が含まれています。すべての MinGW ソフトウェアは 64 ビット Windows プラットフォームで実行できます。

SublimeText3 英語版
推奨: Win バージョン、コードプロンプトをサポート!

SublimeText3 中国語版
中国語版、とても使いやすい

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。

PhpStorm Mac バージョン
最新(2018.2.1)のプロフェッショナル向けPHP統合開発ツール
