One really common pattern found in mobile applications is a dual email + Facebook log in system. Facebook is a very popular sign in method, but noteverybodyhas an account and not everybody wants to use their Facebook account to sign in to new services. This makes giving the option to users to use either email or their Facebook account to sign up very attractive.
There seems to be very little (that I could find at least) information out there on how to set up a log in / authentication system in Sencha Touch. So I’m writing a series of blog posts that will cover how to set up a log in system from scratch with the option to sign up with either email or a Facebook account. The application will have a PHP & MySQL backend and will also use PhoneGap Build.
Topics covered over the coming weeks will include:
- Setting up the database and API (this post)
- Creating the application and screens
- Email sign up system
- Authentication and generating sessions for users
- Auto login / remember me for return visits
- Integrating a Facebook signup system
In this first part, I will walk you through setting up your database and setting up some PHP files on your server that are ready to receive calls from your application.
Setting up the database
We’re going to have to create a MySQL database first of course so go ahead and do that, calling it whatever you wish. Once you’ve created the database, add the following table to it:
CREATE TABLE `users` (`id` INT(11) UNSIGNED NOT NULL AUTO_INCREMENT,`fbid` VARCHAR(255),`email` VARCHAR(100) NOT NULL,`password` VARCHAR(255) NOT NULL,`session` VARCHAR(255),PRIMARY KEY (`id`));
This will allow us to store the details the users uses to sign up, as well as any other details we would like to track. You could go ahead and add some other fields like ‘first_name’, ‘phone’ and so on if you wish. If you’re building out a real application, you’re likely going to require other tables as well, but this is all we need to actually get the log in system working.
Setting up the API
Throughout the log in and sign up processes, our application will be making calls to an API hosted on the Internet. This API will be created with PHP files which will interact with our database, and then return data in a JSON format to our application.
Although we are not implementing it just yet, our application will make calls to our server using an Ajax proxy. The problem with this is that we can run into some Cross Origin Resource Sharing errors which might look something like the following:
XMLHttpRequest cannot load [URL]. No ‘Access-Control-Allow-Origin’ header is present on the requested resource. Origin ‘http://localhost’ is therefore not allowed access.
XMLHttpRequest cannot load [URL]. Origin [URL] is not allowed by Access-Control-Allow-Origin.
Request header field Content-Type is not allowed by Access-Control-Allow-Headers
Essentially, we’re running into security problems because we’re making requests to a domain different to the one the application is hosted on. I wrote a blog posts on this recently so if you’d like more information you cancheck it out here.
What we want to do now though is make sure we don’t run into any of these errors by making sure we set our headers (and everything else) correctly from the beginning. Now I need you to create a file called ‘users.php’ and add the following code to it:
<?php $link = mysql_connect("localhost", "db_user", "your_password");mysql_select_db("your_db", $link);$action = $_GET["action"];$result = "{'success':false}";header('Access-Control-Allow-Origin: *');header('Access-Control-Allow-Methods: GET, POST, OPTIONS');header('Access-Control-Allow-Headers: Content-Type,x-prototype-version,x-requested-with');echo($result);?>
What we are doing here is first connecting to the database – you will have to replace these details with your own. Next we are grabbing the ‘action’ which will be passed into the API through the URL. For example: http://www.example.com/api/users.php?action=something. Eventually we will cycle through this ‘action’ variable to perform the appropriate action. When we want to log a user in we could make an Ajax request to ‘users.php?action=login’ or if we wanted to log a user out ‘users.php?action=logout’ and so on.
Before outputting the result, we are also setting our headers here. These headers are necessary to overwrite the default Cross Origin Resource Sharing options and to prevent the errors above. If you read the CORS article I linked above you will notice that you can also set these headers at the server level instead of directly in your PHP files.
At the end of this file we are outputting ‘$result’. If you were to visit this page in your browser you would simply see ‘{‘success’:false}’. This is a JSON formatted string that is telling us that whatever operation was just performed on the server was not successful. In later parts of this tutorial series we will of course be outputting different data through this JSON string that will indicate whether a user successfully logged in, what their session key is and so on. This same format can be used to send in large, complex data including any and all details we had stored about a user in the database.
This concludes the first part of this tutorial series. At this point we have our database set up and our API ready to be added to and interacted with. Stay tuned for the following posts in this series; I’ll update with a link to Part 2 here when it is ready! Feel free to sign up to the fortnightly newsletter in the bar on the right for updates on any new blog posts.
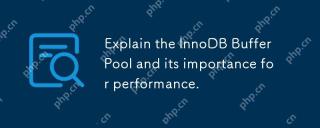
Innodbbufferpoolは、データをキャッシュしてページをインデックス作成することにより、ディスクI/Oを削減し、データベースのパフォーマンスを改善します。その作業原則には次のものが含まれます。1。データ読み取り:Bufferpoolのデータを読む。 2。データの書き込み:データを変更した後、bufferpoolに書き込み、定期的にディスクに更新します。 3.キャッシュ管理:LRUアルゴリズムを使用して、キャッシュページを管理します。 4.読みメカニズム:隣接するデータページを事前にロードします。 BufferPoolのサイジングと複数のインスタンスを使用することにより、データベースのパフォーマンスを最適化できます。
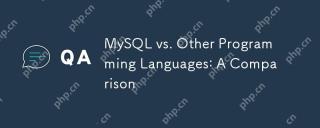
他のプログラミング言語と比較して、MySQLは主にデータの保存と管理に使用されますが、Python、Java、Cなどの他の言語は論理処理とアプリケーション開発に使用されます。 MySQLは、データ管理のニーズに適した高性能、スケーラビリティ、およびクロスプラットフォームサポートで知られていますが、他の言語は、データ分析、エンタープライズアプリケーション、システムプログラミングなどのそれぞれの分野で利点があります。
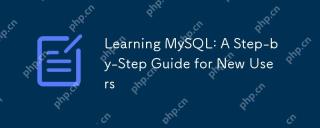
MySQLは、データストレージ、管理、分析に適した強力なオープンソースデータベース管理システムであるため、学習する価値があります。 1)MySQLは、SQLを使用してデータを操作するリレーショナルデータベースであり、構造化されたデータ管理に適しています。 2)SQL言語はMySQLと対話するための鍵であり、CRUD操作をサポートします。 3)MySQLの作業原則には、クライアント/サーバーアーキテクチャ、ストレージエンジン、クエリオプティマイザーが含まれます。 4)基本的な使用には、データベースとテーブルの作成が含まれ、高度な使用にはJoinを使用してテーブルの参加が含まれます。 5)一般的なエラーには、構文エラーと許可の問題が含まれ、デバッグスキルには、構文のチェックと説明コマンドの使用が含まれます。 6)パフォーマンスの最適化には、インデックスの使用、SQLステートメントの最適化、およびデータベースの定期的なメンテナンスが含まれます。
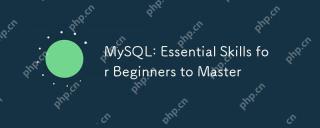
MySQLは、初心者がデータベーススキルを学ぶのに適しています。 1.MySQLサーバーとクライアントツールをインストールします。 2。selectなどの基本的なSQLクエリを理解します。 3。マスターデータ操作:テーブルを作成し、データを挿入、更新、削除します。 4.高度なスキルを学ぶ:サブクエリとウィンドウの関数。 5。デバッグと最適化:構文を確認し、インデックスを使用し、選択*を避け、制限を使用します。
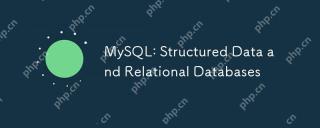
MySQLは、テーブル構造とSQLクエリを介して構造化されたデータを効率的に管理し、外部キーを介してテーブル間関係を実装します。 1.テーブルを作成するときにデータ形式と入力を定義します。 2。外部キーを使用して、テーブル間の関係を確立します。 3。インデックス作成とクエリの最適化により、パフォーマンスを改善します。 4.データベースを定期的にバックアップおよび監視して、データのセキュリティとパフォーマンスの最適化を確保します。
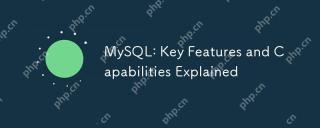
MySQLは、Web開発で広く使用されているオープンソースリレーショナルデータベース管理システムです。その重要な機能には、次のものが含まれます。1。さまざまなシナリオに適したInnodbやMyisamなどの複数のストレージエンジンをサポートします。 2。ロードバランスとデータバックアップを容易にするために、マスタースレーブレプリケーション機能を提供します。 3.クエリの最適化とインデックスの使用により、クエリ効率を改善します。
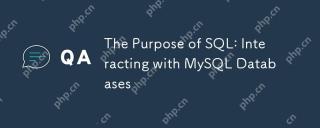
SQLは、MySQLデータベースと対話して、データの追加、削除、変更、検査、データベース設計を実現するために使用されます。 1)SQLは、ステートメントの選択、挿入、更新、削除を介してデータ操作を実行します。 2)データベースの設計と管理に作成、変更、ドロップステートメントを使用します。 3)複雑なクエリとデータ分析は、ビジネス上の意思決定効率を改善するためにSQLを通じて実装されます。
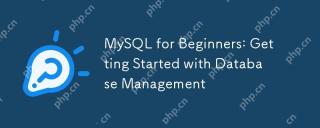
MySQLの基本操作には、データベース、テーブルの作成、およびSQLを使用してデータのCRUD操作を実行することが含まれます。 1.データベースの作成:createdatabasemy_first_db; 2。テーブルの作成:createTableBooks(idintauto_incrementprimarykey、titlevarchary(100)notnull、authorvarchar(100)notnull、published_yearint); 3.データの挿入:InsertIntoBooks(タイトル、著者、公開_year)VA


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

mPDF
mPDF は、UTF-8 でエンコードされた HTML から PDF ファイルを生成できる PHP ライブラリです。オリジナルの作者である Ian Back は、Web サイトから「オンザフライ」で PDF ファイルを出力し、さまざまな言語を処理するために mPDF を作成しました。 HTML2FPDF などのオリジナルのスクリプトよりも遅く、Unicode フォントを使用すると生成されるファイルが大きくなりますが、CSS スタイルなどをサポートし、多くの機能強化が施されています。 RTL (アラビア語とヘブライ語) や CJK (中国語、日本語、韓国語) を含むほぼすべての言語をサポートします。ネストされたブロックレベル要素 (P、DIV など) をサポートします。

SublimeText3 英語版
推奨: Win バージョン、コードプロンプトをサポート!

SublimeText3 中国語版
中国語版、とても使いやすい
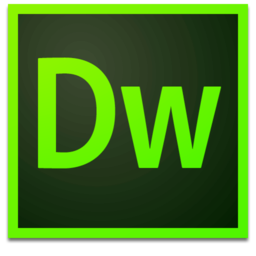
Dreamweaver Mac版
ビジュアル Web 開発ツール
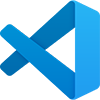
VSCode Windows 64 ビットのダウンロード
Microsoft によって発売された無料で強力な IDE エディター
