この記事では、Node.js カスタム モジュールの内容を紹介します (コード付き)。必要な方は参考にしていただければ幸いです。
エクスポート
方法 1:
エクスポート。属性名 = 値/変数;
メインモジュールをエクスポートできます。 / module.js
const myModule = require("./自定义模块.js"); console.log(myModule.username); console.log(myModule.userage); myModule.getInfo(); console.log(myModule.address);
カスタム module.js
// 自定义模块 // =========1.变量============ let username = "Jack"; let userInfo = {age:10, grade:"H5"}; // 导出/ 暴露内容 exports.username = username; exports.userObj = userInfo; //直接赋值 // =========2.变量============ exports.userage = 18; // =========3.方法============ exports.getInfo = function () { console.log(userInfo,username); } //另一个方法表示 module.exports.address = "北京"; //module.exports 等同于exports console.log(module.exports === exports);//输出true
メソッド 2: module.exports = object;
moudule.exports = class/constructor/function;
注: 複数の module.exports を記述します。 = オブジェクトは前のオブジェクトを上書きし、その値を変更します。
エクスポートされたクラス/コンストラクターは new を通じてエクスポートする必要があり、オブジェクトは new にすることはできません。
カスタム モジュール 2-2.js
//自定义模块2 module.exports = { user:"丽丽", tag: 100 } //方法 //此时user和tag输出的为undefined,因为这个exports方法会直接覆盖上面的exports module.exports = function () { console.log("自定义模块2");//主模块调用:myModule2()或new myModule2() } // 导出===类(构造函数) module.exports = class UserName{ // console.log("我是个类/构造函数");//导进的模块必须通过new 下进行导出,对象不能new } //这样不能进行导出,相当于又声明了一个exports,exports添加属性和方法是可以进行导出,修改属性或者方法就不能进行导出 // exports = { // a:100 // }
メイン モジュール.js
// 自定义模块2 console.log("=============自定义模块2==============="); const myModule2 = require("./自定义模块2-2.js"); // console.log(myModule2); console.log(myModule2.user); console.log(myModule2.tag); // myModule2();//还可以new myModule2()表示 new myModule2();
例: 円と正方形の面積と周囲長を計算します
main.js
// 引入模块 let square = require("./square.js"); let circle = require("./circle.js"); // 计算正方形的面积和周长 let squareClass = new square(10); console.log("正方形的面积是:"+squareClass.area()); console.log("正方形的周长是:"+squareClass.circumference()); // 计算圆的面积和周长 console.log("圆的面积是:"+circle.area(5)); console.log("圆的周长是:"+circle.circumference(5));
square.js
//计算正方形的面积和周长,使用模块 module.exports = class { constructor(w){ this.w = w; } area(){ return this.w ** 2; } circumference(){ return this.w * 4; } }
circle.js
//计算圆的面积和周长 exports.area = function (r) { return Math.PI * (r ** 2); } exports.circumference = function (r) { return 2 * Math.PI *r; }
関連する推奨事項:
Node.js モジュール パスの紹介Node.js ファイルシステムでのファイル監視のコード実装
以上がNode.js カスタム モジュールのコンテンツの紹介 (コード付き)の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
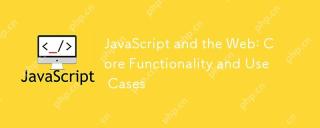
Web開発におけるJavaScriptの主な用途には、クライアントの相互作用、フォーム検証、非同期通信が含まれます。 1)DOM操作による動的なコンテンツの更新とユーザーインタラクション。 2)ユーザーエクスペリエンスを改善するためにデータを提出する前に、クライアントの検証が実行されます。 3)サーバーとのリフレッシュレス通信は、AJAXテクノロジーを通じて達成されます。
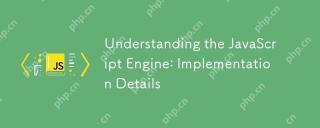
JavaScriptエンジンが内部的にどのように機能するかを理解することは、開発者にとってより効率的なコードの作成とパフォーマンスのボトルネックと最適化戦略の理解に役立つためです。 1)エンジンのワークフローには、3つの段階が含まれます。解析、コンパイル、実行。 2)実行プロセス中、エンジンはインラインキャッシュや非表示クラスなどの動的最適化を実行します。 3)ベストプラクティスには、グローバル変数の避け、ループの最適化、constとletsの使用、閉鎖の過度の使用の回避が含まれます。

Pythonは、スムーズな学習曲線と簡潔な構文を備えた初心者により適しています。 JavaScriptは、急な学習曲線と柔軟な構文を備えたフロントエンド開発に適しています。 1。Python構文は直感的で、データサイエンスやバックエンド開発に適しています。 2。JavaScriptは柔軟で、フロントエンドおよびサーバー側のプログラミングで広く使用されています。
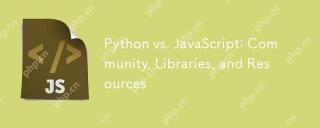
PythonとJavaScriptには、コミュニティ、ライブラリ、リソースの観点から、独自の利点と短所があります。 1)Pythonコミュニティはフレンドリーで初心者に適していますが、フロントエンドの開発リソースはJavaScriptほど豊富ではありません。 2)Pythonはデータサイエンスおよび機械学習ライブラリで強力ですが、JavaScriptはフロントエンド開発ライブラリとフレームワークで優れています。 3)どちらも豊富な学習リソースを持っていますが、Pythonは公式文書から始めるのに適していますが、JavaScriptはMDNWebDocsにより優れています。選択は、プロジェクトのニーズと個人的な関心に基づいている必要があります。
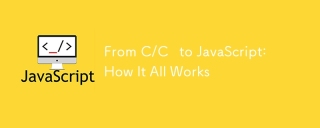
C/CからJavaScriptへのシフトには、動的なタイピング、ゴミ収集、非同期プログラミングへの適応が必要です。 1)C/Cは、手動メモリ管理を必要とする静的に型付けられた言語であり、JavaScriptは動的に型付けされ、ごみ収集が自動的に処理されます。 2)C/Cはマシンコードにコンパイルする必要がありますが、JavaScriptは解釈言語です。 3)JavaScriptは、閉鎖、プロトタイプチェーン、約束などの概念を導入します。これにより、柔軟性と非同期プログラミング機能が向上します。
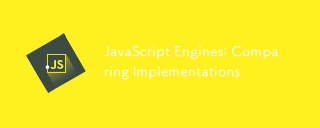
さまざまなJavaScriptエンジンは、各エンジンの実装原則と最適化戦略が異なるため、JavaScriptコードを解析および実行するときに異なる効果をもたらします。 1。語彙分析:ソースコードを語彙ユニットに変換します。 2。文法分析:抽象的な構文ツリーを生成します。 3。最適化とコンパイル:JITコンパイラを介してマシンコードを生成します。 4。実行:マシンコードを実行します。 V8エンジンはインスタントコンピレーションと非表示クラスを通じて最適化され、Spidermonkeyはタイプ推論システムを使用して、同じコードで異なるパフォーマンスパフォーマンスをもたらします。
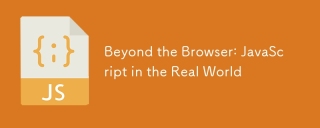
現実世界におけるJavaScriptのアプリケーションには、サーバー側のプログラミング、モバイルアプリケーション開発、モノのインターネット制御が含まれます。 2。モバイルアプリケーションの開発は、ReactNativeを通じて実行され、クロスプラットフォームの展開をサポートします。 3.ハードウェアの相互作用に適したJohnny-Fiveライブラリを介したIoTデバイス制御に使用されます。
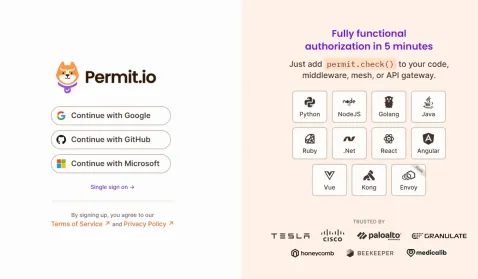
私はあなたの日常的な技術ツールを使用して機能的なマルチテナントSaaSアプリケーション(EDTECHアプリ)を作成しましたが、あなたは同じことをすることができます。 まず、マルチテナントSaaSアプリケーションとは何ですか? マルチテナントSaaSアプリケーションを使用すると、Singの複数の顧客にサービスを提供できます


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール
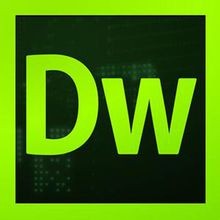
ドリームウィーバー CS6
ビジュアル Web 開発ツール

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
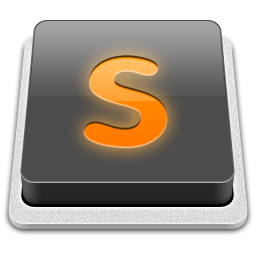
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、
