この記事では主に PHP のリフレクションの仕組みを紹介します。参考になるものがありますので、共有します。困っている友達は参考にしてください。
PHP5 は追加します新機能: リフレクション。この機能により、プログラマーは
リバース エンジニアリング[リバース エンジニアリング]、クラス、インターフェイス、関数、メソッド、および拡張機能[拡張ライブラリのサポート]を行うことができます。
PHP コードを通じて、オブジェクトのすべての情報を取得し、オブジェクトと対話できます。
次の Person クラスを想定します:
1 class Person { 2 /** 3 * For the sake of demonstration, we"re setting this private 4 */ 5 private $_allowDynamicAttributes = false; 6 7 /** 8 * type=primary_autoincrement 9 */ 10 protected $id = 0; 11 12 /** 13 * type=varchar length=255 null 14 */ 15 protected $name; 16 17 /** 18 * type=text null19 */ 20 protected $biography; 21 public function getId() { 22 return $this->id; 23 } 24 public function setId($v) { 25 $this->id = $v; 26 } 27 public function getName() { 28 return $this->name; 29 } 30 public function setName($v) { 31 $this->name = $v; 32 } 33 public function getBiography() { 34 return $this->biography; 35 } 36 public function setBiography($v) { 37 $this->biography = $v; 38 } 39 }
ReflectionClass を通じて、次の Person クラスの情報を取得できます:
-
定数コンテンツ
プロパティ プロパティ名
メソッド名
静的プロパティ静的プロパティ
-
NamespaceNamespace
Person クラスが Final か Abstract かどうか
クラス名「person」を ReflectionClass に渡すだけです:
1 $class = new ReflectionClass('Person');
* プロパティの取得 (Properties):
1 $properties = $class->getProperties();2 foreach($properties as $property) { 3 echo $property->getName()."\n";4 } 5 // 输出:6 // _allowDynamicAttributes7 // id8 // name9 // biography
デフォルトでは、ReflectionClass はプライベートおよび保護されたプロパティを含むすべてのプロパティを取得します。プライベート属性のみを取得したい場合は、追加のパラメーターを渡す必要があります:
1 $private_properties = $class->getProperties(ReflectionProperty::IS_PRIVATE);
使用可能なパラメーターのリスト:
- ##ReflectionProperty::IS_STATIC
- ReflectionProperty::IS_PUBLIC
- ReflectionProperty::IS_PROTECTED
- ReflectionProperty::IS_PRIVATE
1 foreach($properties as $property) { 2 if($property->isProtected()) { 3 $docblock = $property->getDocComment(); 4 preg_match('/ type\=([a-z_]*) /', $property->getDocComment(), $matches); 5 echo $matches[1]."\n"; 6 } 7 } 8 // Output: 9 // primary_autoincrement 10 // varchar 11 // textちょっと信じられないですね。コメントも取得できます。
## メソッドの取得: getMethods() を通じてクラスのすべてのメソッドを取得します。返されるのは、ReflectionMethod オブジェクトの配列です。
これ以上のデモはありません。
## 最後に、ReflectionMethod を通じてクラス内のメソッドを呼び出します。$data = array("id" => 1, "name" => "Chris", "biography" => "I am am a PHP developer"); foreach($data as $key => $value) { if(!$class->hasProperty($key)) { throw new Exception($key." is not a valid property"); } if(!$class->hasMethod("get".ucfirst($key))) { throw new Exception($key." is missing a getter"); } if(!$class->hasMethod("set".ucfirst($key))) { throw new Exception($key." is missing a setter"); } // Make a new object to interact with $object = new Person(); // Get the getter method and invoke it with the value in our data array $setter = $class->getMethod("set".ucfirst($key)); $ok = $setter->invoke($object, $value); // Get the setter method and invoke it $setter = $class->getMethod("get".ucfirst($key)); $objValue = $setter->invoke($object); // Now compare if($value == $objValue) { echo "Getter or Setter has modified the data.\n"; } else { echo "Getter and Setter does not modify the data.\n"; } }以上がこの記事の全内容です。皆様の学習に少しでもお役に立てれば幸いです。その他の関連コンテンツについては、PHP 中国語 Web サイトをご覧ください。 関連する推奨事項:
php で Web コンテンツと画像をクロールする方法
以上がPHP の Reflection リフレクション メカニズムの概要の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
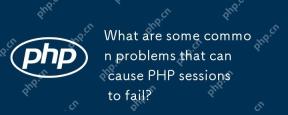
PHPSESSIONの障害の理由には、構成エラー、Cookieの問題、セッションの有効期限が含まれます。 1。構成エラー:正しいセッションをチェックして設定します。save_path。 2.Cookieの問題:Cookieが正しく設定されていることを確認してください。 3.セッションの有効期限:セッションを調整してください。GC_MAXLIFETIME値はセッション時間を延長します。
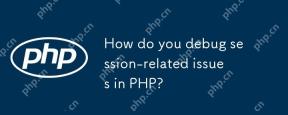
PHPでセッションの問題をデバッグする方法は次のとおりです。1。セッションが正しく開始されるかどうかを確認します。 2.セッションIDの配信を確認します。 3.セッションデータのストレージと読み取りを確認します。 4.サーバーの構成を確認します。セッションIDとデータを出力し、セッションファイルのコンテンツを表示するなど、セッション関連の問題を効果的に診断して解決できます。
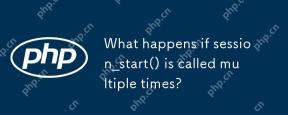
session_start()への複数の呼び出しにより、警告メッセージと可能なデータ上書きが行われます。 1)PHPは警告を発し、セッションが開始されたことを促します。 2)セッションデータの予期しない上書きを引き起こす可能性があります。 3)session_status()を使用してセッションステータスを確認して、繰り返しの呼び出しを避けます。
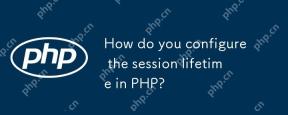
PHPでのセッションライフサイクルの構成は、session.gc_maxlifetimeとsession.cookie_lifetimeを設定することで達成できます。 1)session.gc_maxlifetimeサーバー側のセッションデータのサバイバル時間を制御します。 0に設定すると、ブラウザが閉じているとCookieが期限切れになります。
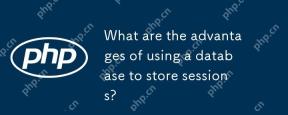
データベースストレージセッションを使用することの主な利点には、持続性、スケーラビリティ、セキュリティが含まれます。 1。永続性:サーバーが再起動しても、セッションデータは変更されないままになります。 2。スケーラビリティ:分散システムに適用され、セッションデータが複数のサーバー間で同期されるようにします。 3。セキュリティ:データベースは、機密情報を保護するための暗号化されたストレージを提供します。
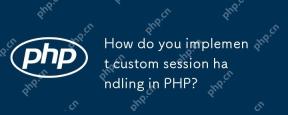
PHPでのカスタムセッション処理の実装は、SessionHandlerInterfaceインターフェイスを実装することで実行できます。具体的な手順には、次のものが含まれます。1)CussentsessionHandlerなどのSessionHandlerInterfaceを実装するクラスの作成。 2)セッションデータのライフサイクルとストレージ方法を定義するためのインターフェイス(オープン、クローズ、読み取り、書き込み、破壊、GCなど)の書き換え方法。 3)PHPスクリプトでカスタムセッションプロセッサを登録し、セッションを開始します。これにより、データをMySQLやRedisなどのメディアに保存して、パフォーマンス、セキュリティ、スケーラビリティを改善できます。
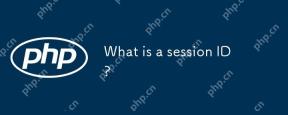
SessionIDは、ユーザーセッションのステータスを追跡するためにWebアプリケーションで使用されるメカニズムです。 1.ユーザーとサーバー間の複数のインタラクション中にユーザーのID情報を維持するために使用されるランダムに生成された文字列です。 2。サーバーは、ユーザーの複数のリクエストでこれらの要求を識別および関連付けるのに役立つCookieまたはURLパラメーターを介してクライアントに生成および送信します。 3.生成は通常、ランダムアルゴリズムを使用して、一意性と予測不可能性を確保します。 4.実際の開発では、Redisなどのメモリ内データベースを使用してセッションデータを保存してパフォーマンスとセキュリティを改善できます。
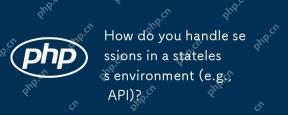
APIなどのステートレス環境でのセッションの管理は、JWTまたはCookieを使用して達成できます。 1。JWTは、無国籍とスケーラビリティに適していますが、ビッグデータに関してはサイズが大きいです。 2.cookiesはより伝統的で実装が簡単ですが、セキュリティを確保するために慎重に構成する必要があります。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール
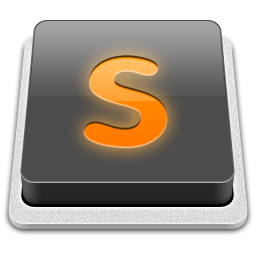
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

mPDF
mPDF は、UTF-8 でエンコードされた HTML から PDF ファイルを生成できる PHP ライブラリです。オリジナルの作者である Ian Back は、Web サイトから「オンザフライ」で PDF ファイルを出力し、さまざまな言語を処理するために mPDF を作成しました。 HTML2FPDF などのオリジナルのスクリプトよりも遅く、Unicode フォントを使用すると生成されるファイルが大きくなりますが、CSS スタイルなどをサポートし、多くの機能強化が施されています。 RTL (アラビア語とヘブライ語) や CJK (中国語、日本語、韓国語) を含むほぼすべての言語をサポートします。ネストされたブロックレベル要素 (P、DIV など) をサポートします。

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン
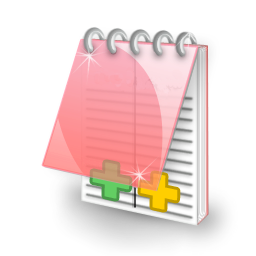
EditPlus 中国語クラック版
サイズが小さく、構文の強調表示、コード プロンプト機能はサポートされていません

ホットトピック









