この記事では、Angular でモーダル ボックスをポップアップする 2 つの方法を主に紹介します。これは非常に優れており、必要な方は参考にしていただければ幸いです。
ブログを始める前に、まず ngx-bootstrap-modal をインストールする必要があります
npm install ngx-bootstrap-modal --save
そうしないと、モーダル ボックスの効果が見づらくなり、吐きたくなるでしょう
1. ポップアップ方法 1 (この方法は、 https://github .com/cipchk/ngx-bootstrap-modal)
1.アラートポップアップボックス
(1)デモディレクトリ
--------app.component.ts
- ------- app.component.html
--------app.module.ts
--------詳細(フォルダ)
------ -----detail .component.ts
---------------detail.component.html
(2)デモコード
app.module.tsは、必要なBootstrapModalModuleとModalModuleをインポートします。そして登録します
//app.module.ts import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; //这种模态框只需要导入下面这两个 import { BootstrapModalModule } from 'ngx-bootstrap-modal'; import { ModalModule } from 'ngx-bootstrap/modal'; import { AppComponent } from './app.component'; import { DetailComponent } from './detail/detail.component'; @NgModule({ declarations: [ AppComponent, DetailComponent ], imports: [ BrowserModule, BootstrapModalModule ], providers: [], entryComponents: [ DetailComponent ], bootstrap: [AppComponent] }) export class AppModule { }
app.component.html モーダルボックスをポップアップできるボタンを作成します
<p> </p><p> <button>alert模态框</button> </p>
app.component.ts このボタンのアクションを記述します showAlert()
import { Component } from '@angular/core'; import { DialogService } from "ngx-bootstrap-modal"; import { DetailComponent } from './detail/detail.component' @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'app'; constructor(public dialogService: DialogService) { } showAlert() { this.dialogService.addDialog(DetailComponent, { title: 'Alert title!', message: 'Alert message!!!' }); } }
detail.component.html レイアウトを記述しますアラート ポップアップ ボックスの
<p> </p><p> </p><p> <button>×</button> </p><h4 id="title">{{title}}</h4> <p> 这是alert弹框 </p> <p> <button>取消</button> <button>确定</button> </p>
detail.component .ts を使用してモーダル ボックス コンポーネントを作成するには、コンポーネントを作成する必要があります。その後、ngx-bootstrap-model が起動をガイドします
このコンポーネントについては、次のことを継承する必要がありますDialogComponent
T 外部パラメーターはモーダル ボックスのタイプに渡されます。
T1 モーダル ボックスの戻り値の型。
したがって、DialogService は DialogComponent のコンストラクター パラメーターである必要があります。
import { Component } from '@angular/core'; import { DialogComponent, DialogService } from 'ngx-bootstrap-modal'; export interface AlertModel { title: string; message: string; } @Component({ selector: 'alert', templateUrl: './detail.component.html', styleUrls: ['./detail.component.css'] }) export class DetailComponent extends DialogComponent<alertmodel> implements AlertModel { title: string; message: string; constructor(dialogService: DialogService) { super(dialogService); } }</alertmodel>
2.ポップアップボックスの確認
(1)デモディレクトリ
--------app.component.ts
--------app.component.html
--- -- ---app.module.ts
----------confirm(フォルダ)
-------------confirm.component.ts
-------- - ---confirm.component.html
(2)デモコード
app.module.ts は必要な BootstrapModalModule と ModalModule をインポートして登録します。これらはアラート ポップアップ ボックスと同じです。メソッド 1 のポップアップ メソッド
//app.module.ts import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; //这种模态框只需要导入下面这两个 import { BootstrapModalModule } from 'ngx-bootstrap-modal'; import { ModalModule } from 'ngx-bootstrap/modal'; import { AppComponent } from './app.component'; import { DetailComponent } from './detail/detail.component'; @NgModule({ declarations: [ AppComponent, DetailComponent ], imports: [ BrowserModule, BootstrapModalModule ], providers: [], entryComponents: [ DetailComponent ], bootstrap: [AppComponent] }) export class AppModule { }
app.component.html モーダル ボックスをポップアップできるボタンを作成します
<p> </p><p> <button>modal模态框</button> </p>
app.component.ts このボタンのアクション showconfirm() を記述します
import { Component } from '@angular/core'; import { DialogService } from "ngx-bootstrap-modal"; import { ConfirmComponent } from './confirm/confirm.component' @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'app'; constructor(public dialogService: DialogService,private modalService: BsModalService) { } showConfirm() { this.dialogService.addDialog(ConfirmComponent, { title: 'Confirmation', message: 'bla bla' }) .subscribe((isConfirmed) => { }); } }
confirm.component .html 確認ポップアップボックスのレイアウトを記述します
<p> </p><p> </p><p> <button>×</button> </p><h4 id="title">{{title}}</h4> <p> 这是confirm弹框 </p> <p> <button>取消</button> <button>确定</button> </p>
verify.component.ts はモーダルボックスコンポーネントを作成します
(1)デモディレクトリ
---- ----app.component.ts
------ -app.component.html
(2)デモコード
ポップアップ ボックスには 3 つの形式のアラート確認プロンプトも含まれており、すべていくつかの組み込みスタイルが含まれています
app .module.ts
import { Component } from '@angular/core'; import { DialogComponent, DialogService } from 'ngx-bootstrap-modal'; export interface ConfirmModel { title:string; message:any; } @Component({ selector: 'confirm', templateUrl: './confirm.component.html', styleUrls: ['./confirm.component.css'] }) export class ConfirmComponent extends DialogComponent<confirmmodel> implements ConfirmModel { title: string; message: any; constructor(dialogService: DialogService) { super(dialogService); } confirm() { // on click on confirm button we set dialog result as true, // ten we can get dialog result from caller code this.close(true); } cancel() { this.close(false); } }</confirmmodel>
app.component.html は非常にシンプルで、ボタン 1 つだけです
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { BootstrapModalModule } from 'ngx-bootstrap-modal'; import { ModalModule } from 'ngx-bootstrap/modal'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, BootstrapModalModule, ModalModule.forRoot() ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
app.component。 ts は非常にシンプルで、コンポーネントのレイアウトを記述する必要さえありません。アイコンやサイズなどのパラメーターを渡すだけです。
<p> </p><p> <button>内置</button> </p>
2. ポップアップ メソッド 2 (このメソッドは https:/ からのものです) /valor-software.com/ngx-bootstrap/#/modals)
前の方法と同じですが、最初に ngx-bootstrap-modal をインストールしてから、ブートストラップ スタイルの Table
1.demo ディレクトリをインポートします
-- ------app.component.ts
----------app.component.html--------app.module.ts
2.デモコード
app.module .ts は対応するモジュールをインポートして登録します
import { Component } from '@angular/core'; import { DialogService, BuiltInOptions } from "ngx-bootstrap-modal"; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'app'; constructor(public dialogService: DialogService) { } show(){ this.dialogService.show(<builtinoptions>{ content: '保存成功', icon: 'success', size: 'sm', showCancelButton: false }) } }</builtinoptions>
app.component.ts
//app.module.ts import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { ModalModule } from 'ngx-bootstrap/modal'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, ModalModule.forRoot() ], providers: [], entryComponents: [ ], bootstrap: [AppComponent] }) export class AppModule { }
app.component.html
import { Component,TemplateRef } from '@angular/core'; import { BsModalService } from 'ngx-bootstrap/modal'; import { BsModalRef } from 'ngx-bootstrap/modal/modal-options.class'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'app'; public modalRef: BsModalRef; constructor(private modalService: BsModalService) { } showSecond(template: TemplateRef<any>){//传入的是一个组件 this.modalRef = this.modalService.show(template,{class: 'modal-lg'});//在这里通过BsModalService里面的show方法把它显示出来 }; }</any>
3. 最終的な効果
上記をすべて結合します。すべてのポップアップ ボックスを一緒に書き込みます。効果は次のとおりです
関連する推奨事項:
以上がAngular でモーダル ボックスをポップアップする 2 つの方法の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
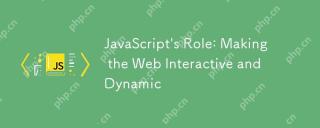
JavaScriptは、Webページのインタラクティブ性とダイナミズムを向上させるため、現代のWebサイトの中心にあります。 1)ページを更新せずにコンテンツを変更できます。2)Domapiを介してWebページを操作する、3)アニメーションやドラッグアンドドロップなどの複雑なインタラクティブ効果、4)ユーザーエクスペリエンスを改善するためのパフォーマンスとベストプラクティスを最適化します。
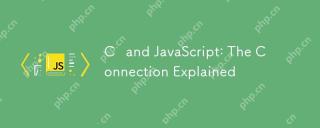
CおよびJavaScriptは、WebAssemblyを介して相互運用性を実現します。 1)CコードはWebAssemblyモジュールにコンパイルされ、JavaScript環境に導入され、コンピューティングパワーが強化されます。 2)ゲーム開発では、Cは物理エンジンとグラフィックスレンダリングを処理し、JavaScriptはゲームロジックとユーザーインターフェイスを担当します。
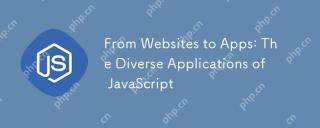
JavaScriptは、Webサイト、モバイルアプリケーション、デスクトップアプリケーション、サーバー側のプログラミングで広く使用されています。 1)Webサイト開発では、JavaScriptはHTMLおよびCSSと一緒にDOMを運用して、JQueryやReactなどのフレームワークをサポートします。 2)ReactNativeおよびIonicを通じて、JavaScriptはクロスプラットフォームモバイルアプリケーションを開発するために使用されます。 3)電子フレームワークにより、JavaScriptはデスクトップアプリケーションを構築できます。 4)node.jsを使用すると、JavaScriptがサーバー側で実行され、高い並行リクエストをサポートします。
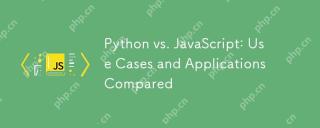
Pythonはデータサイエンスと自動化により適していますが、JavaScriptはフロントエンドとフルスタックの開発により適しています。 1. Pythonは、データ処理とモデリングのためにNumpyやPandasなどのライブラリを使用して、データサイエンスと機械学習でうまく機能します。 2。Pythonは、自動化とスクリプトにおいて簡潔で効率的です。 3. JavaScriptはフロントエンド開発に不可欠であり、動的なWebページと単一ページアプリケーションの構築に使用されます。 4. JavaScriptは、node.jsを通じてバックエンド開発において役割を果たし、フルスタック開発をサポートします。
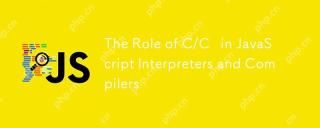
CとCは、主に通訳者とJITコンパイラを実装するために使用されるJavaScriptエンジンで重要な役割を果たします。 1)cは、JavaScriptソースコードを解析し、抽象的な構文ツリーを生成するために使用されます。 2)Cは、Bytecodeの生成と実行を担当します。 3)Cは、JITコンパイラを実装し、実行時にホットスポットコードを最適化およびコンパイルし、JavaScriptの実行効率を大幅に改善します。
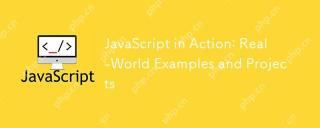
現実世界でのJavaScriptのアプリケーションには、フロントエンドとバックエンドの開発が含まれます。 1)DOM操作とイベント処理を含むTODOリストアプリケーションを構築して、フロントエンドアプリケーションを表示します。 2)node.jsを介してRestfulapiを構築し、バックエンドアプリケーションをデモンストレーションします。
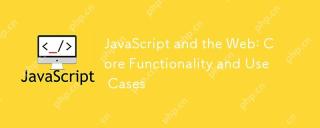
Web開発におけるJavaScriptの主な用途には、クライアントの相互作用、フォーム検証、非同期通信が含まれます。 1)DOM操作による動的なコンテンツの更新とユーザーインタラクション。 2)ユーザーエクスペリエンスを改善するためにデータを提出する前に、クライアントの検証が実行されます。 3)サーバーとのリフレッシュレス通信は、AJAXテクノロジーを通じて達成されます。
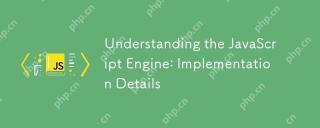
JavaScriptエンジンが内部的にどのように機能するかを理解することは、開発者にとってより効率的なコードの作成とパフォーマンスのボトルネックと最適化戦略の理解に役立つためです。 1)エンジンのワークフローには、3つの段階が含まれます。解析、コンパイル、実行。 2)実行プロセス中、エンジンはインラインキャッシュや非表示クラスなどの動的最適化を実行します。 3)ベストプラクティスには、グローバル変数の避け、ループの最適化、constとletsの使用、閉鎖の過度の使用の回避が含まれます。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール
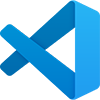
VSCode Windows 64 ビットのダウンロード
Microsoft によって発売された無料で強力な IDE エディター
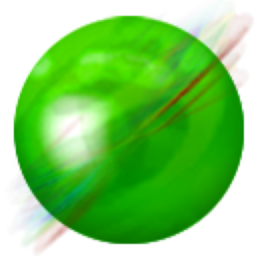
ZendStudio 13.5.1 Mac
強力な PHP 統合開発環境

MantisBT
Mantis は、製品の欠陥追跡を支援するために設計された、導入が簡単な Web ベースの欠陥追跡ツールです。 PHP、MySQL、Web サーバーが必要です。デモおよびホスティング サービスをチェックしてください。

メモ帳++7.3.1
使いやすく無料のコードエディター

mPDF
mPDF は、UTF-8 でエンコードされた HTML から PDF ファイルを生成できる PHP ライブラリです。オリジナルの作者である Ian Back は、Web サイトから「オンザフライ」で PDF ファイルを出力し、さまざまな言語を処理するために mPDF を作成しました。 HTML2FPDF などのオリジナルのスクリプトよりも遅く、Unicode フォントを使用すると生成されるファイルが大きくなりますが、CSS スタイルなどをサポートし、多くの機能強化が施されています。 RTL (アラビア語とヘブライ語) や CJK (中国語、日本語、韓国語) を含むほぼすべての言語をサポートします。ネストされたブロックレベル要素 (P、DIV など) をサポートします。

ホットトピック









