この記事では、例を示して Python スコープの使用法を分析します。参考のために皆さんと共有してください。詳細は次のとおりです:
すべてのプログラミング言語には変数スコープの概念があり、Python も例外ではありません。以下は Python スコープのコードのデモです:
def scope_test(): def do_local(): spam = "local spam" def do_nonlocal(): nonlocal spam spam = "nonlocal spam" def do_global(): global spam spam = "global spam" spam = "test spam" do_local() print("After local assignment:", spam) do_nonlocal() print("After nonlocal assignment:", spam) do_global() print("After global assignment:", spam) scope_test() print("In global scope:", spam)
。プログラムの出力 結果:
After local assignment: test spam After nonlocal assignment: nonlocal spam After global assignment: nonlocal spam In global scope: global spam
注: ローカル代入ステートメントは、scope_test のスパム バインディングを変更できません。非ローカル代入ステートメントは、scope_test のスパム バインディングを変更し、グローバル代入ステートメントは、モジュール レベルからスパム バインディングを変更します。
その中で、nonlocal は Python 3 の新しいキーワードです。
また、スパムがグローバル代入ステートメントの前に事前バインドされていないこともわかります。
概要:
プログラム内でグローバル変数にアクセスする状況に遭遇し、グローバル変数の値を変更したい場合は、以下を使用できます: global キーワード、この変数を関数内のグローバル変数として宣言します
nonlocal キーワードが関数で使用されています。または、他のスコープで外部 (非グローバル) 変数を使用します。
グローバル キーワードは理解しやすく、他の言語にも一般に同じことが当てはまります。別の非ローカルな例を次に示します:
def make_counter(): count = 0 def counter(): nonlocal count count += 1 return count return counter def make_counter_test(): mc = make_counter() print(mc()) print(mc()) print(mc())
実行結果:
1 2 3
Python スコープの使用例分析に関する詳細な記事については、PHP 中国語 Web サイトに注目してください。
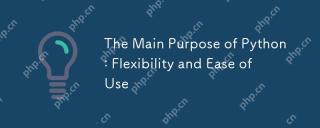
Pythonの柔軟性は、マルチパラダイムサポートと動的タイプシステムに反映されていますが、使いやすさはシンプルな構文とリッチ標準ライブラリに由来しています。 1。柔軟性:オブジェクト指向、機能的および手続き的プログラミングをサポートし、動的タイプシステムは開発効率を向上させます。 2。使いやすさ:文法は自然言語に近く、標準的なライブラリは幅広い機能をカバーし、開発プロセスを簡素化します。
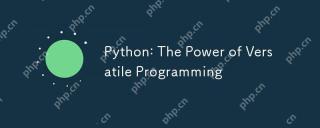
Pythonは、初心者から上級開発者までのすべてのニーズに適した、そのシンプルさとパワーに非常に好まれています。その汎用性は、次のことに反映されています。1)学習と使用が簡単、シンプルな構文。 2)Numpy、Pandasなどの豊富なライブラリとフレームワーク。 3)さまざまなオペレーティングシステムで実行できるクロスプラットフォームサポート。 4)作業効率を向上させるためのスクリプトおよび自動化タスクに適しています。
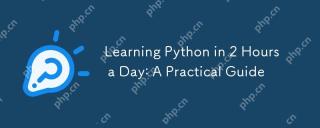
はい、1日2時間でPythonを学びます。 1.合理的な学習計画を作成します。2。適切な学習リソースを選択します。3。実践を通じて学んだ知識を統合します。これらの手順は、短時間でPythonをマスターするのに役立ちます。
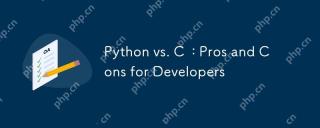
Pythonは迅速な開発とデータ処理に適していますが、Cは高性能および基礎となる制御に適しています。 1)Pythonは、簡潔な構文を備えた使いやすく、データサイエンスやWeb開発に適しています。 2)Cは高性能で正確な制御を持ち、ゲームやシステムのプログラミングでよく使用されます。
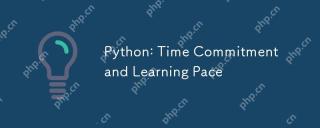
Pythonを学ぶのに必要な時間は、人によって異なり、主に以前のプログラミングの経験、学習の動機付け、学習リソースと方法、学習リズムの影響を受けます。現実的な学習目標を設定し、実用的なプロジェクトを通じて最善を尽くします。
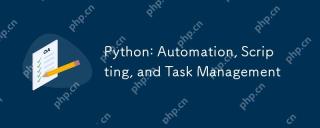
Pythonは、自動化、スクリプト、およびタスク管理に優れています。 1)自動化:OSやShutilなどの標準ライブラリを介してファイルバックアップが実現されます。 2)スクリプトの書き込み:Psutilライブラリを使用してシステムリソースを監視します。 3)タスク管理:スケジュールライブラリを使用してタスクをスケジュールします。 Pythonの使いやすさと豊富なライブラリサポートにより、これらの分野で優先ツールになります。
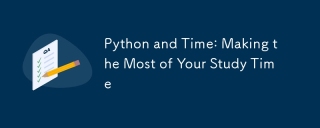
限られた時間でPythonの学習効率を最大化するには、PythonのDateTime、時間、およびスケジュールモジュールを使用できます。 1. DateTimeモジュールは、学習時間を記録および計画するために使用されます。 2。時間モジュールは、勉強と休息の時間を設定するのに役立ちます。 3.スケジュールモジュールは、毎週の学習タスクを自動的に配置します。
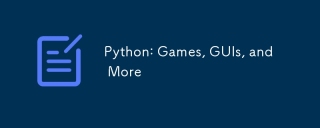
PythonはゲームとGUI開発に優れています。 1)ゲーム開発は、2Dゲームの作成に適した図面、オーディオ、その他の機能を提供し、Pygameを使用します。 2)GUI開発は、TKINTERまたはPYQTを選択できます。 TKINTERはシンプルで使いやすく、PYQTは豊富な機能を備えており、専門能力開発に適しています。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター
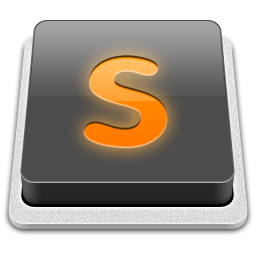
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)
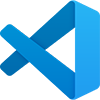
VSCode Windows 64 ビットのダウンロード
Microsoft によって発売された無料で強力な IDE エディター
