基本概念
Annotation(アノテーション)は、プログラムがリフレクションを通じて指定されたプログラム要素のAnnotationオブジェクトを取得し、Annotationオブジェクトを通じてアノテーション内のメタデータを取得することができるインターフェースです。
アノテーションの使用法と目的に応じて、アノテーションはシステム アノテーション、メタ アノテーション、カスタム アノテーションの 3 つのカテゴリに分類できます。
システム アノテーション
システム アノテーションは JDK の組み込みアノテーションであり、主に @Override、@Deprecated、@SuppressWarnings が含まれます。
1. @Override
メソッドを変更する場合、そのメソッドは親クラスのメソッド、またはインターフェースを実装するメソッドをオーバーライドすることを意味します
interface Demo{ public void print(); }public class Test implements Demo{ @Override public void print() { } }
2.@Deprecated
廃止されたメソッドを変更するMethod
3.@SuppressWarnings
コンパイラの警告を抑制します。つまり、警告を削除します。
共通のパラメータ値は次のとおりです:
Name | Function |
---|---|
rawtypes | は、パラメータを渡すときにジェネリックを持つパラメータも渡す必要があることを意味します |
非推奨 | は非推奨の警告を使用しましたクラスまたはメソッドの場合 |
unchecked | 保存されるコレクションの種類を指定するためにジェネリックを使用せずにコレクションを使用する場合など、チェックされていない変換が実行された場合の警告 |
fallthrough | Switch ブロックが次のブロックに直接つながる場合の警告Break なしの場合 |
クラスパス、ソースファイルパスなどに存在しないパスがある場合の警告 | |
finally 節が正常に完了しない場合の警告 | |
上記すべてに関する警告。 | |
// 抑制单类型@SuppressWarnings("unchecked")public void print() { @SuppressWarnings("rawtypes") List list = new ArrayList(); list.add("a"); }// 抑制多类型@SuppressWarnings({ "unchecked", "rawtypes" })public void print() { List list = new ArrayList(); list.add("a"); }// 抑制所有类型@SuppressWarnings({ "all" })public void print() { List list = new ArrayList(); list.add("a"); } |
メタアノテーション |
メタアノテーション
タイプが定義されています。定義されたメタアノテーションは次のとおりです: @Target、@Retention、@Documented、@Inherited。
1.@Target
@Target は、Annotation によって変更されるオブジェクトの範囲を定義します。具体的な変更範囲は次のとおりです。public enum ElementType { // 用于描述类、接口(包括注解类型) 或enum声明 TYPE, // 用于描述域(即变量) FIELD, // 用于描述方法 METHOD, // 用于描述参数 PARAMETER, // 用于描述构造器 CONSTRUCTOR, // 用于描述局部变量 LOCAL_VARIABLE, // 用于描述注解类型 ANNOTATION_TYPE, // 用于描述包 PACKAGE }
2.@Retention
@Retention は、注釈は保持されます。長さは注釈のライフサイクルを示します。public enum RetentionPolicy { // 在源文件中有效(编译器要丢弃的注解) SOURCE, // class 文件中有效(默认,编译器将把注解记录在类文件中,但在运行时 VM 不需要保留注解) CLASS, // 在运行时有效(编译器将把注解记录在类文件中,在运行时 VM 将保留注解,因此可以反射性地读取) RUNTIME }
3.@Documented
@Documented はアノテーションを定義し、特定のタイプのアノテーションが javadoc および同様のデフォルト ツールを通じて文書化されることを示します。 型宣言に Documented の注釈が付けられている場合、その注釈は注釈付き要素のパブリック API の一部になります。4.@Inherited
@Inherited は注釈を定義し、注釈タイプが自動的に継承されることを示します。つまり、 @Inherited で変更された注釈タイプがクラスに使用され、この注釈が子に使用されます。クラスの種類の。クラス以外のアノテーション型を使用した場合、@Inheritedは無効となります。
このメタアノテーションは、親クラスからのアノテーションの継承を容易にするだけであり、実装されたインターフェースのアノテーションは無効です。
-
@Inherited アノテーション タイプでアノテーションが付けられたアノテーションの Retention が RetentionPolicy.RUNTIME の場合、リフレクション API はこの継承を強化します。 java.lang.reflect を使用して @Inherited アノテーション タイプのアノテーションをクエリすると、リフレクティブ コード インスペクションが機能し始めます。指定されたアノテーション タイプが見つかるまで、またはクラス継承構造の最上位レベルが見つかるまで、クラスとその親クラスがチェックされます。に達しました
-
例は次のとおりです:
// 定义注解@Retention(RetentionPolicy.RUNTIME)@Target(ElementType.TYPE)@Inherited@interface MyAnotation{ public String name(); }// 作用在类上@MyAnotation(name="parent") class Parent{ }// 继承 Parent 类public class Test extends Parent{ public static void main(String[] args) { Class<?> cls = Test.class; // 通过 @Inherited 继承父类的注解 Annotation annotation = cls.getAnnotation(MyAnotation.class); MyAnotation myAnotation = (MyAnotation) annotation; System.out.println(myAnotation.name()); } }// 输出结果:parent(若注释掉注解,返回异常)
カスタムアノテーション
1. クラスアノテーション
// 定义注解@Retention(RetentionPolicy.RUNTIME)@Target(ElementType.TYPE)@interface MyAnnotation { public String name(); public String age(); }// 调用注解@MyAnnotation(name="cook",age="100")public class Test { public static void main(String[] args) { Class<?> cls = Test.class; // 1.取得所有注解 Annotation[] annotations =cls.getAnnotations(); // 2.取得指定注解 MyAnnotation annotation = (MyAnnotation)cls.getAnnotation(MyAnnotation.class); } }
2. メソッドアノテーション
// 定义注解@Retention(RetentionPolicy.RUNTIME)
// 修改作用范围@Target(ElementType.METHOD)@interface MyAnnotation { public String name(); public String age();
}
// 调用注解public class Test {
public static void main(String[] args) throws Exception {
Class cls = Test.class;
Method method = cls.getDeclaredMethod("print", null); // 1.取得所有注解
Annotation[] annotations = method.getDeclaredAnnotations(); // 2.取得指定注解
MyAnnotation annotation =
(MyAnnotation)method.getAnnotation(MyAnnotation.class);
}
3. パラメータの注釈// 定义注解@Retention(RetentionPolicy.RUNTIME)
// 修改作用范围@Target(ElementType.PARAMETER)@interface MyAnnotation { public String name(); public String age();
}public class Test {
public static void main(String[] args) throws Exception {
Class cls = Test.class;
Method method = cls.getDeclaredMethod("print", new Class[]{String.class,String.class});
getAllAnnotations(method);
} // 作用在参数上
public void print(@MyAnnotation(name = "cook", age = "100")
String name, String age) { } public static void getAllAnnotations(Method method) {
Annotation[][] parameterAnnotions = method.getParameterAnnotations();
// 通过反射只能取得所有参数类型,不能取得指定参数
Class[] paraemterTypes = method.getParameterTypes();
int i = 0;
for (Annotation[] annotations : parameterAnnotions) {
Class paraemterType = paraemterTypes[i++];
for (Annotation annotation : annotations) {
if (annotation instanceof MyAnnotation) {
MyAnnotation myAnnotation = (MyAnnotation) annotation;
System.out.println(paraemterType.getName());
System.out.println(myAnnotation.name());
System.out.println(myAnnotation.age());
}
}
}
}
}
4. 変数アノテーション // 定义注解@Retention(RetentionPolicy.RUNTIME)
// 修改作用范围@Target(ElementType.FIELD)@interface MyAnnotation { public String name(); public String age();
}public class Test {
// 作用在变量上
@MyAnnotation(name = "cook", age = "100")
private String name;
public static void main(String[] args) throws Exception {
Class cls = Test.class;
Field field = cls.getDeclaredField("name");
Annotation[] fieldAnnotions = field.getDeclaredAnnotations();
MyAnnotation annotation =
(MyAnnotation) field.getAnnotation(MyAnnotation.class);
}
}
上記は 09.Java Basics - Annotations の内容です。その他の関連コンテンツについては、PHP 中国語 Web サイト (www.php.cn) に注目してください。

この記事では、Javaプロジェクト管理、自動化の構築、依存関係の解像度にMavenとGradleを使用して、アプローチと最適化戦略を比較して説明します。
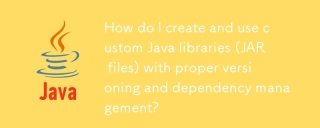
この記事では、MavenやGradleなどのツールを使用して、適切なバージョン化と依存関係管理を使用して、カスタムJavaライブラリ(JARファイル)の作成と使用について説明します。
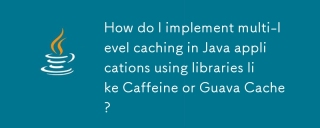
この記事では、カフェインとグアバキャッシュを使用してJavaでマルチレベルキャッシュを実装してアプリケーションのパフォーマンスを向上させています。セットアップ、統合、パフォーマンスの利点をカバーし、構成と立ち退きポリシー管理Best Pra

この記事では、キャッシュや怠zyなロードなどの高度な機能を備えたオブジェクトリレーショナルマッピングにJPAを使用することについて説明します。潜在的な落とし穴を強調しながら、パフォーマンスを最適化するためのセットアップ、エンティティマッピング、およびベストプラクティスをカバーしています。[159文字]
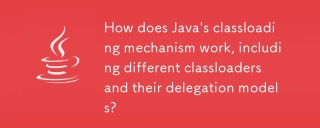
Javaのクラスロードには、ブートストラップ、拡張機能、およびアプリケーションクラスローダーを備えた階層システムを使用して、クラスの読み込み、リンク、および初期化が含まれます。親の委任モデルは、コアクラスが最初にロードされ、カスタムクラスのLOAに影響を与えることを保証します


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、
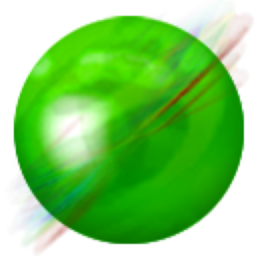
ZendStudio 13.5.1 Mac
強力な PHP 統合開発環境

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。

SublimeText3 中国語版
中国語版、とても使いやすい
