私は最近サムネイルに取り組んでおり、2 つの方法を皆さんと共有したいと思います
$source_path: 元の画像のパス
$NewImagePath: サムネイルのパスを生成します
$target_width: サムネイルの幅
$target_height : サムネイルの高さ
<?php function getCropper($source_path,$NewImagePath, $target_width, $target_height) { $source_info = getimagesize($source_path); $source_width = $source_info[0]; $source_height = $source_info[1]; $source_mime = $source_info['mime']; $source_ratio = $source_height / $source_width; $target_ratio = $target_height / $target_width; // 源图过高 if ($source_ratio > $target_ratio) { $cropped_width = $source_width; $cropped_height = $source_width * $target_ratio; $source_x = 0; $source_y = ($source_height - $cropped_height) / 2; } // 源图过宽 elseif ($source_ratio < $target_ratio) { $cropped_width = $source_height / $target_ratio; $cropped_height = $source_height; $source_x = ($source_width - $cropped_width) / 2; $source_y = 0; } // 源图适中 else { $cropped_width = $source_width; $cropped_height = $source_height; $source_x = 0; $source_y = 0; } switch ($source_mime) { case 'image/gif': $source_image = imagecreatefromgif($source_path); break; case 'image/jpeg': $source_image = imagecreatefromjpeg($source_path); break; case 'image/png': $source_image = imagecreatefrompng($source_path); break; default: return false; break; } $target_image = imagecreatetruecolor($target_width, $target_height); $cropped_image = imagecreatetruecolor($cropped_width, $cropped_height); // 图片裁剪 imagecopy($cropped_image, $source_image, 0, 0, $source_x, $source_y, $cropped_width, $cropped_height); // 图片缩放 imagecopyresampled($target_image, $cropped_image, 0, 0, 0, 0, $target_width, $target_height, $cropped_width, $cropped_height); header('Content-Type: image/jpeg'); imagejpeg($target_image,$NewImagePath,100); imagedestroy($source_image); imagedestroy($target_image); imagedestroy($cropped_image); }
次の方法は、サムネイルを生成し、白い端を埋めることです
<?php //生成缩略图,填充白边 function getCrops($src_path,$NewImagePath,$width,$height){ //源图对象 $src_image = imagecreatefromstring(file_get_contents($src_path)); $source_info = getimagesize($src_path); $source_mime = $source_info['mime']; $src_width = imagesx($src_image); $src_height = imagesy($src_image); switch ($source_mime) { case 'image/gif': $src_image = imagecreatefromgif($src_path); break; case 'image/jpeg': $src_image = imagecreatefromjpeg($src_path); break; case 'image/png': $src_image = imagecreatefrompng($src_path); break; default: return false; break; } //生成等比例的缩略图 //$tmp_image_width = 0; //$tmp_image_height = 0; if ($src_width / $src_height >= $width / $height) { $tmp_image_width = $width; $tmp_image_height = round($tmp_image_width * $src_height / $src_width); } else { $tmp_image_height = $height; $tmp_image_width = round($tmp_image_height * $src_width / $src_height); } $tmpImage = imagecreatetruecolor($tmp_image_width, $tmp_image_height); imagecopyresampled($tmpImage, $src_image, 0, 0, 0, 0, $tmp_image_width, $tmp_image_height, $src_width, $src_height); //添加白边 $final_image = imagecreatetruecolor($width, $height); $color = imagecolorallocate($final_image, 255, 255, 255); imagefill($final_image, 0, 0, $color); $x = round(($width - $tmp_image_width) / 2); $y = round(($height - $tmp_image_height) / 2); imagecopy($final_image, $tmpImage, $x, $y, 0, 0, $tmp_image_width, $tmp_image_height); //输出图片 header('Content-Type: image/jpeg'); imagejpeg($final_image,$NewImagePath,100); imagedestroy($src_image); imagedestroy($final_image); }
上記は、サムネイルを生成するための(実践的な)PHP メソッドの内容です。その他の関連コンテンツについては、注意してください。 PHP 中国語 Web サイト (www.php.cn) にアクセスしてください。
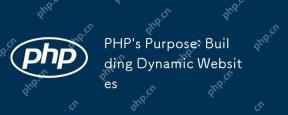
PHPは動的なWebサイトを構築するために使用され、そのコア関数には次のものが含まれます。1。データベースに接続することにより、動的コンテンツを生成し、リアルタイムでWebページを生成します。 2。ユーザーのインタラクションを処理し、提出をフォームし、入力を確認し、操作に応答します。 3.セッションとユーザー認証を管理して、パーソナライズされたエクスペリエンスを提供します。 4.パフォーマンスを最適化し、ベストプラクティスに従って、ウェブサイトの効率とセキュリティを改善します。

PHPはMySQLIおよびPDO拡張機能を使用して、データベース操作とサーバー側のロジック処理で対話し、セッション管理などの関数を介してサーバー側のロジックを処理します。 1)MySQLIまたはPDOを使用してデータベースに接続し、SQLクエリを実行します。 2)セッション管理およびその他の機能を通じて、HTTPリクエストとユーザーステータスを処理します。 3)トランザクションを使用して、データベース操作の原子性を確保します。 4)SQLインジェクションを防ぎ、例外処理とデバッグの閉鎖接続を使用します。 5)インデックスとキャッシュを通じてパフォーマンスを最適化し、読みやすいコードを書き、エラー処理を実行します。
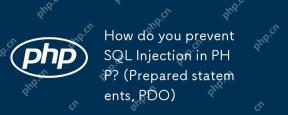
PHPで前処理ステートメントとPDOを使用すると、SQL注入攻撃を効果的に防ぐことができます。 1)PDOを使用してデータベースに接続し、エラーモードを設定します。 2)準備方法を使用して前処理ステートメントを作成し、プレースホルダーを使用してデータを渡し、メソッドを実行します。 3)結果のクエリを処理し、コードのセキュリティとパフォーマンスを確保します。
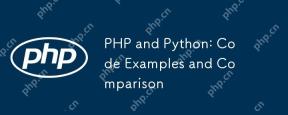
PHPとPythonには独自の利点と短所があり、選択はプロジェクトのニーズと個人的な好みに依存します。 1.PHPは、大規模なWebアプリケーションの迅速な開発とメンテナンスに適しています。 2。Pythonは、データサイエンスと機械学習の分野を支配しています。
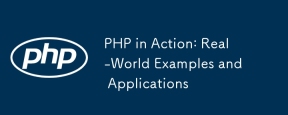
PHPは、電子商取引、コンテンツ管理システム、API開発で広く使用されています。 1)eコマース:ショッピングカート機能と支払い処理に使用。 2)コンテンツ管理システム:動的コンテンツの生成とユーザー管理に使用されます。 3)API開発:RESTFUL API開発とAPIセキュリティに使用されます。パフォーマンスの最適化とベストプラクティスを通じて、PHPアプリケーションの効率と保守性が向上します。
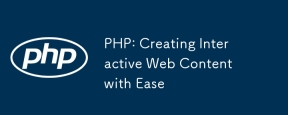
PHPにより、インタラクティブなWebコンテンツを簡単に作成できます。 1)HTMLを埋め込んでコンテンツを動的に生成し、ユーザー入力またはデータベースデータに基づいてリアルタイムで表示します。 2)プロセスフォームの提出と動的出力を生成して、XSSを防ぐためにHTMLSPECIALCHARSを使用していることを確認します。 3)MySQLを使用してユーザー登録システムを作成し、Password_HashおよびPreprocessingステートメントを使用してセキュリティを強化します。これらの手法を習得すると、Web開発の効率が向上します。
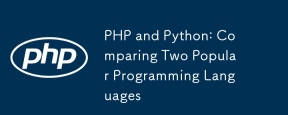
PHPとPythonにはそれぞれ独自の利点があり、プロジェクトの要件に従って選択します。 1.PHPは、特にWebサイトの迅速な開発とメンテナンスに適しています。 2。Pythonは、データサイエンス、機械学習、人工知能に適しており、簡潔な構文を備えており、初心者に適しています。
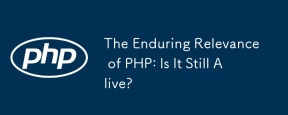
PHPは依然として動的であり、現代のプログラミングの分野で重要な位置を占めています。 1)PHPのシンプルさと強力なコミュニティサポートにより、Web開発で広く使用されています。 2)その柔軟性と安定性により、Webフォーム、データベース操作、ファイル処理の処理において顕著になります。 3)PHPは、初心者や経験豊富な開発者に適した、常に進化し、最適化しています。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール
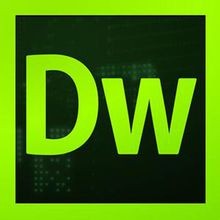
ドリームウィーバー CS6
ビジュアル Web 開発ツール

Safe Exam Browser
Safe Exam Browser は、オンライン試験を安全に受験するための安全なブラウザ環境です。このソフトウェアは、あらゆるコンピュータを安全なワークステーションに変えます。あらゆるユーティリティへのアクセスを制御し、学生が無許可のリソースを使用するのを防ぎます。

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

MantisBT
Mantis は、製品の欠陥追跡を支援するために設計された、導入が簡単な Web ベースの欠陥追跡ツールです。 PHP、MySQL、Web サーバーが必要です。デモおよびホスティング サービスをチェックしてください。
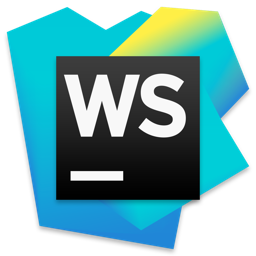
WebStorm Mac版
便利なJavaScript開発ツール
