Base64 は、インターネット上で 8 ビット バイト コードを送信するための最も一般的なエンコード方式の 1 つであり、MIME の詳細な仕様が記載されている RFC2045 ~ RFC2049 を確認できます。 Base64 では、3 つの 8 ビット バイトごとに 4 つの 6 ビット バイト (3*8 = 4*6 = 24) に変換し、その後 2 つの上位ビット 0 を 6 ビットに追加して 4 つの 8 ビット バイトを形成する必要があります。つまり、変換された文字列は理論的には次のようになります。元のものより 1/3 長くなります
php 関数:base64_encode() とbase64_decode()
base64 エンコードとデコードの原理
Base64 エンコードは実際には 3 つの 8 ビット ワードです セクションは 4 つの 6 ビット バイトに変換されます (3) *8 = 4*6 = 24) これら 4 つの 6 ビット バイトは実際には 8 ビットのままですが、上位 2 ビットは 0 に設定されます。バイトが 6 のみの場合。ビットが有効な場合、その値空間は 0 から 0 までです。 2の6乗から1を引いた値、つまり63です。つまり、変換されたBase64コードの各コードの値空間は(0~63)となります。
実際には、ASCII コードには 0 ~ 63 の間に多くの目に見えない文字があるため、別のマッピングを行う必要があります。
'A' ~ 'Z' ? ASCII (0 ~ 25)
'a ' ~ 'z' ? ASCII (26 ~ 51)
' 0' ~ '9' ? ASCII (62)
'/'
3 つの 8 ビット バイトを 4 つの表示可能な文字に変換できます。
具体的なバイト分割方法は次のとおりです: (図 (絵は下手です、精神を理解してください:-))
aaaaaabb ccccdddd eeffffff //abcdef は実際には 1 または 0 です。明確に見るには、代わりに abcdef を使用してください
~ ~~~~~~~ ~~~~~~~~ ~~~~~~~~
バイト 1 バイト 2 バイト 3
||
/
00aaaaaa 00bbcccc 00ddddee 00ffffff
注: 上部3 バイトは元のテキストで、下位 4 バイトは Base64 でエンコードされ、最初の 2 ビットは 0 です。
このように分割する場合、元のテキストのバイト数は3の倍数である必要があります。この条件を満たさない場合は、変換時にBase64エンコードをすべて0バイトで補います。 Base64 エンコーディングが 1 つまたは 2 つの等号
で終わる理由は、次のような理由からです。F (origin) が元のテキストのバイト数を表し、F (remain) が表す場合は剰余を表すので、
F (剰余) = F(原点) MOD 3 が成り立ちます。
したがって、F(remain) の可能な値は 0,1,2 です。
n = [F(origin) – F(remain)] / 3 の場合
F(remain) = 0 の場合、これが起こります。 4*n バイトの Base64 エンコードに変換されます。
F(remain) = 1の場合、元のテキストの1バイトはBase64でエンコードされた2つのバイトに分割できるため、
Base64エンコードを4の倍数にするには、2つの等号を追加する必要があります。
F(remain) = 2の場合、元のテキストの2バイトはBase64でエンコードされた3バイトに分割できるため、同様に
に等号を追加する必要があります。
Base64 でエンコードされた文字列の末尾には 0 ~ 2 個の等号がありますが、これらの等号はデコードには必要ないため、削除できます。
ネットワークのGETおよびPOSTパラメータリストでは、「+」は通常は送信できません。「|」に置き換えることができます
この方法では、base64でエンコードされた文字列には「|」と「/」のみが含まれるため、base64はこのように処理され、エンコードされた文字列はパラメータリストのパラメータ値として送信できます
============================== ====== =======================================
以下は外国人によって書かれた実装:
package com.meterware.httpunit;
/******************************************************************************************************************** <br>* $Id: Base64.java,v 1.4 2002/12/24 15:17:17 russgold Exp $ <br>* <br>* Copyright (c) 2000-2002 by Russell Gold <br>* <br>* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated <br>* documentation files (the "Software "), to deal in the Software without restriction, including without limitation <br>* the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and <br>* to permit persons to whom the Software is furnished to do so, subject to the following conditions: <br>* <br>* The above copyright notice and this permission notice shall be included in all copies or substantial portions <br>* of the Software. <br>* <br>* THE SOFTWARE IS PROVIDED "AS IS ", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO <br>* THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE <br>* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF <br>* CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER <br>* DEALINGS IN THE SOFTWARE. <br>* <br>*******************************************************************************************************************/ <br><br>/** <br>* A utility class to convert to and from base 64 encoding. <br>* <br>* @author <a href= "mailto:russgold@httpunit.org "> Russell Gold </a> <br>**/ <br> public class Base64 { final static String encodingChar = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/ "; /** * Returns the base 64 encoded equivalent of a supplied string. * @param source the string to encode */ public static String encode( String source ) { char[] sourceBytes = getPaddedBytes( source ); int numGroups = (sourceBytes.length + 2) / 3; char[] targetBytes = new char[4]; char[] target = new char[ 4 * numGroups ]; for (int group = 0; group < numGroups; group++) { convert3To4( sourceBytes, group*3, targetBytes ); for (int i = 0; i < targetBytes.length; i++) { target[ i + 4*group ] = encodingChar.charAt( targetBytes[i] ); } } int numPadBytes = sourceBytes.length - source.length(); for (int i = target.length-numPadBytes; i < target.length; i++) target[i] = '= '; return new String( target ); } private static char[] getPaddedBytes( String source ) { char[] converted = source.toCharArray(); int requiredLength = 3 * ((converted.length+2) /3); char[] result = new char[ requiredLength ]; System.arraycopy( converted, 0, result, 0, converted.length ); return result; } private static void convert3To4( char[] source, int sourceIndex, char[] target ) { target[0] = (char) ( source[ sourceIndex ] > > > 2); target[1] = (char) (((source[ sourceIndex ] & 0x03) < < 4) | (source[ sourceIndex+1 ] > > > 4)); target[2] = (char) (((source[ sourceIndex+1 ] & 0x0f) < < 2) | (source[ sourceIndex+2 ] > > > 6)); target[3] = (char) ( source[ sourceIndex+2 ] & 0x3f); } /** * Returns the plaintext equivalent of a base 64-encoded string. * @param source a base 64 string (which must have a multiple of 4 characters) */ public static String decode( String source ) { if (source.length()%4 != 0) throw new RuntimeException( "valid Base64 codes have a multiple of 4 characters " ); int numGroups = source.length() / 4; int numExtraBytes = source.endsWith( "== " ) ? 2 : (source.endsWith( "= " ) ? 1 : 0); byte[] targetBytes = new byte[ 3*numGroups ]; byte[] sourceBytes = new byte[4]; for (int group = 0; group < numGroups; group++) { for (int i = 0; i < sourceBytes.length; i++) { sourceBytes[i] = (byte) Math.max( 0, encodingChar.indexOf( source.charAt( 4*group+i ) ) ); } convert4To3( sourceBytes, targetBytes, group*3 ); } return new String( targetBytes, 0, targetBytes.length - numExtraBytes ); } private static void convert4To3( byte[] source, byte[] target, int targetIndex ) { target[ targetIndex ] = (byte) (( source[0] < < 2) | (source[1] > > > 4)); target[ targetIndex+1 ] = (byte) (((source[1] & 0x0f) < < 4) | (source[2] > > > 2)); target[ targetIndex+2 ] = (byte) (((source[2] & 0x03) < < 6) | (source[3])); } }
base64_encode と Base64_decode の JAVA 実装に関連するその他の記事については、PHP 中国語 Web サイトに注目してください。
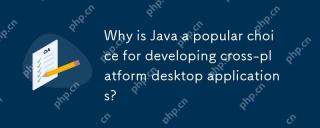
javaispopularforsoss-platformdesktopapplicationsduetoits "writeonce、runaynay" philosophy.1)itusesbytecodatiTatrunnanyjvm-adipplatform.2)ライブラリリケンディンガンドジャヴァフククレアティック - ルルクリス
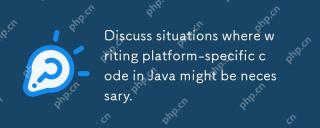
Javaでプラットフォーム固有のコードを作成する理由には、特定のオペレーティングシステム機能へのアクセス、特定のハードウェアとの対話、パフォーマンスの最適化が含まれます。 1)JNAまたはJNIを使用して、Windowsレジストリにアクセスします。 2)JNIを介してLinux固有のハードウェアドライバーと対話します。 3)金属を使用して、JNIを介してMacOSのゲームパフォーマンスを最適化します。それにもかかわらず、プラットフォーム固有のコードを書くことは、コードの移植性に影響を与え、複雑さを高め、パフォーマンスのオーバーヘッドとセキュリティのリスクをもたらす可能性があります。
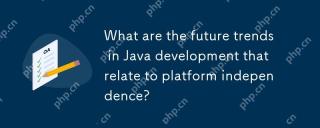
Javaは、クラウドネイティブアプリケーション、マルチプラットフォームの展開、および言語間の相互運用性を通じて、プラットフォームの独立性をさらに強化します。 1)クラウドネイティブアプリケーションは、GraalvmとQuarkusを使用してスタートアップ速度を向上させます。 2)Javaは、埋め込みデバイス、モバイルデバイス、量子コンピューターに拡張されます。 3)Graalvmを通じて、JavaはPythonやJavaScriptなどの言語とシームレスに統合して、言語間の相互運用性を高めます。
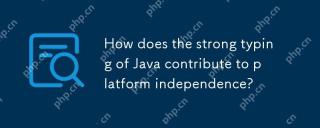
Javaの強力なタイプ化されたシステムは、タイプの安全性、統一タイプの変換、多型を通じてプラットフォームの独立性を保証します。 1)タイプの安全性は、コンパイル時間でタイプチェックを実行して、ランタイムエラーを回避します。 2)統一された型変換ルールは、すべてのプラットフォームで一貫しています。 3)多型とインターフェイスメカニズムにより、コードはさまざまなプラットフォームで一貫して動作します。
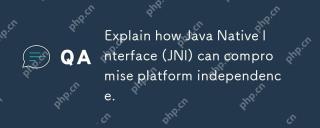
JNIはJavaのプラットフォームの独立を破壊します。 1)JNIは特定のプラットフォームにローカルライブラリを必要とします。2)ローカルコードをターゲットプラットフォームにコンパイルおよびリンクする必要があります。3)異なるバージョンのオペレーティングシステムまたはJVMは、異なるローカルライブラリバージョンを必要とする場合があります。
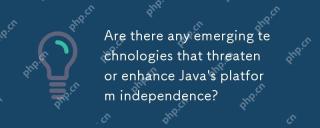
新しいテクノロジーは、両方の脅威をもたらし、Javaのプラットフォームの独立性を高めます。 1)Dockerなどのクラウドコンピューティングとコンテナ化テクノロジーは、Javaのプラットフォームの独立性を強化しますが、さまざまなクラウド環境に適応するために最適化する必要があります。 2)WebAssemblyは、Graalvmを介してJavaコードをコンパイルし、プラットフォームの独立性を拡張しますが、パフォーマンスのために他の言語と競合する必要があります。
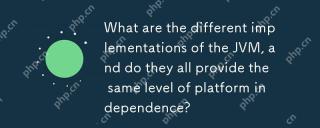
JVMの実装が異なると、プラットフォームの独立性が得られますが、パフォーマンスはわずかに異なります。 1。OracleHotspotとOpenJDKJVMは、プラットフォームの独立性で同様に機能しますが、OpenJDKは追加の構成が必要になる場合があります。 2。IBMJ9JVMは、特定のオペレーティングシステムで最適化を実行します。 3. Graalvmは複数の言語をサポートし、追加の構成が必要です。 4。AzulzingJVMには、特定のプラットフォーム調整が必要です。
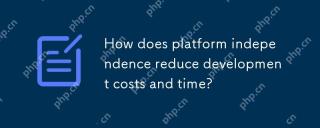
プラットフォームの独立性により、開発コストが削減され、複数のオペレーティングシステムで同じコードセットを実行することで開発時間を短縮します。具体的には、次のように表示されます。1。開発時間を短縮すると、1セットのコードのみが必要です。 2。メンテナンスコストを削減し、テストプロセスを統合します。 3.展開プロセスを簡素化するための迅速な反復とチームコラボレーション。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール
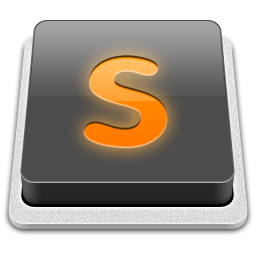
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。

SublimeText3 英語版
推奨: Win バージョン、コードプロンプトをサポート!

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ホットトピック









