1. 基本的な
変数関数:
<?php $func = 'test'; function test(){ echo 'yes !'; } $func(); ?>
ランダム関数:
<?php $newfunc = create_function('$a,$b', 'return $a.$b;'); echo "New anonymous function: $newfunc<br>"; echo $newfunc('just', 'coding'); ?>
create_function — 匿名 (ラムダスタイル) 関数を作成します
匿名関数を作成します。この関数は主にunsortとarray_walkのコールバック関数で使用されます
$a,$bはパラメータ、'return $a,$b'は関数のコードです
コールバック関数:
<?php //5.3 以前 $array = array( 'asbc', 'ddd', 'tttt', 'qqq'); array_walk($array,create_function('&$item','$item=strtoupper($item);') ); //function(&$itm){$itm = strtoupper($itm);} print_r($array); //5.3 以后 $array = array( 'asbc', 'ddd', 'tttt', 'qqq'); array_walk($array,function(&$itm){$itm = strtoupper($itm);}); print_r($array); ?>
array_walk(array 、関数、ユーザーデータ ...)
array_walk() 関数は、配列内の各要素にコールバック関数を適用します。成功した場合は TRUE を返し、そうでない場合は FALSE を返します。
通常、関数は 2 つのパラメータを受け取ります。配列パラメータの値が最初のパラメータとして使用され、キー名が 2 番目のパラメータとして使用されます。オプションのパラメーター userdata が指定されている場合、それは 3 番目のパラメーターとしてコールバック関数に渡されます。
2. クラス関数のインスタンスの動的作成
<?php /* create class */ class Record { /* record information will be held in here */ private $info; /* constructor */ function Record($record_array) { $record_array['body'] = 'this is a new attribution'; $this->info = $record_array; } /* dynamic function server */ function __call($method,$arguments) { $meth = $this->from_case(substr($method,3,strlen($method)-3)); return array_key_exists($meth,$this->info) ? $this->info[$meth] : false; } function from_case($str) { $str[0] = strtolower($str[0]); $func = create_function('$c', 'return "_" . strtolower($c[1]);'); // function ($c) { return "_" . strtolower($c[1]); } return preg_replace_callback('/([A-Z])/', $func, $str); } } /* usage */ $Record = new Record( array( 'id' => 12, 'title' => 'Greatest Hits', 'description' => 'The greatest hits from the best band in the world!' ) ); /* proof it works! */ echo 'The ID is: '.$Record->getId().'<br>'; // returns 12 echo 'The Title is: '.$Record->getTitle().'<br>'; // returns "Greatest Hits" echo 'The Description is: '.$Record->getDescription().'<br>'; //returns "The greatest hits from the best band in the world!" echo 'The Body is: '.$Record->getBody(); //returns "The greatest hits from the best band in the world!" ?>
重要なポイントは: __call と create_function
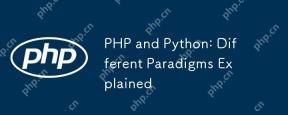
PHPは主に手順プログラミングですが、オブジェクト指向プログラミング(OOP)もサポートしています。 Pythonは、OOP、機能、手続き上のプログラミングなど、さまざまなパラダイムをサポートしています。 PHPはWeb開発に適しており、Pythonはデータ分析や機械学習などのさまざまなアプリケーションに適しています。
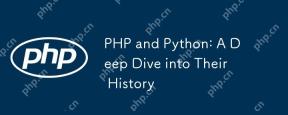
PHPは1994年に発信され、Rasmuslerdorfによって開発されました。もともとはウェブサイトの訪問者を追跡するために使用され、サーバー側のスクリプト言語に徐々に進化し、Web開発で広く使用されていました。 Pythonは、1980年代後半にGuidovan Rossumによって開発され、1991年に最初にリリースされました。コードの読みやすさとシンプルさを強調し、科学的コンピューティング、データ分析、その他の分野に適しています。
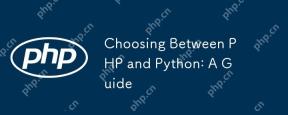
PHPはWeb開発と迅速なプロトタイピングに適しており、Pythonはデータサイエンスと機械学習に適しています。 1.PHPは、単純な構文と迅速な開発に適した動的なWeb開発に使用されます。 2。Pythonには簡潔な構文があり、複数のフィールドに適しており、強力なライブラリエコシステムがあります。
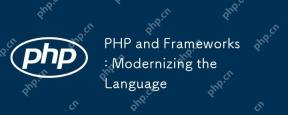
PHPは、多数のWebサイトとアプリケーションをサポートし、フレームワークを通じて開発ニーズに適応するため、近代化プロセスで依然として重要です。 1.PHP7はパフォーマンスを向上させ、新機能を紹介します。 2。Laravel、Symfony、Codeigniterなどの最新のフレームワークは、開発を簡素化し、コードの品質を向上させます。 3.パフォーマンスの最適化とベストプラクティスは、アプリケーションの効率をさらに改善します。
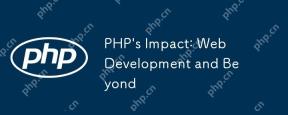
phphassiblasifly-impactedwebdevevermentandsbeyondit.1)itpowersmajorplatformslikewordpratsandexcelsindatabase interactions.2)php'sadaptableability allowsitale forlargeapplicationsusingframeworkslikelavel.3)
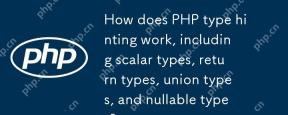
PHPタイプは、コードの品質と読みやすさを向上させるためのプロンプトがあります。 1)スカラータイプのヒント:php7.0であるため、基本データ型は、int、floatなどの関数パラメーターで指定できます。 3)ユニオンタイプのプロンプト:PHP8.0であるため、関数パラメーターまたは戻り値で複数のタイプを指定することができます。 4)Nullable Typeプロンプト:null値を含めることができ、null値を返す可能性のある機能を処理できます。
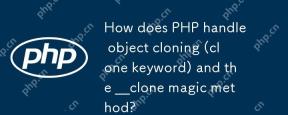
PHPでは、クローンキーワードを使用してオブジェクトのコピーを作成し、\ _ \ _クローンマジックメソッドを使用してクローン動作をカスタマイズします。 1.クローンキーワードを使用して浅いコピーを作成し、オブジェクトのプロパティをクローン化しますが、オブジェクトのプロパティはクローニングしません。 2。\ _ \ _クローン法は、浅いコピーの問題を避けるために、ネストされたオブジェクトを深くコピーできます。 3.クローニングにおける円形の参照とパフォーマンスの問題を避けるために注意し、クローニング操作を最適化して効率を向上させます。
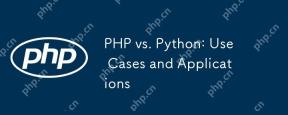
PHPはWeb開発およびコンテンツ管理システムに適しており、Pythonはデータサイエンス、機械学習、自動化スクリプトに適しています。 1.PHPは、高速でスケーラブルなWebサイトとアプリケーションの構築においてうまく機能し、WordPressなどのCMSで一般的に使用されます。 2。Pythonは、NumpyやTensorflowなどの豊富なライブラリを使用して、データサイエンスと機械学習の分野で驚くほどパフォーマンスを発揮しています。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール
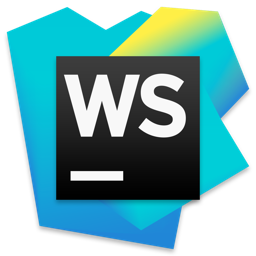
WebStorm Mac版
便利なJavaScript開発ツール

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター

SublimeText3 英語版
推奨: Win バージョン、コードプロンプトをサポート!

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。
