<?php /** * 缩略图水印生成类 文字水印 字符编码为 utf-8 * 中文需要处理还字体的问题 * @name MakeMiniature * @see * @version 2.1.0 (2016-1-22) * @author sanshi0815 */ class MakeMiniature { //字体带目录 private $font; //水印内容 可以是 文字,可以是图片路径,也可以为为空 private $watermark; //源文件 private $srcFile; //目标文件 private $dstFile; //水印类别 0 无水印,1图片水印,2文字水印 private $watetType; //支持的图片类别当前支持2种 private $imgType=array("jpg","jpeg","png"); //图片打开资源句柄 private $im; //原始图片类型 private $fileType; private $errorMsg=""; public function __construct() { } /** * 获取失败信息 * @author sanshi0815 * @return string 失败信息 */ public function getErrorMsg() { return $this->errorMsg; } /** * 参数设置 * @author sanshi0815 * @param string $font 字体文件带目录文字水印使用 * @param string $watermark 水印文字或者是图片地址 * @param string $srcFile 原始图片地址 * @param string $dstFile 生成新图片地址 * @return null 无返回值 */ public function set($font,$watermark,$srcFile,$dstFile) { $this->font = $font; $this->watermark = $watermark; $this->srcFile = $srcFile; $this->dstFile = $dstFile; if(empty($this->watermark )) { //无水印 $this->watetType = 0; }elseif(is_file($this->watermark)){ //图片水印 $this->watetType = 1; }else{ //文字水印 $this->watetType = 2; } } /** * 图片资源获取 成功返回数组,失败返回false * @author sanshi0815 * @param string $fileName 图片地址 * @return array r{句柄},t{后缀},w{宽度},h{高度} */ private function getResource($fileName) { if(!is_file($fileName)) { $this->errorMsg = "{$fileName} 不存在 line:".__LINE__; } $temp = explode('.',$fileName); $fileType = strtolower(end($temp)); //判断后缀是否是否符合要求 if(!in_array($fileType,$this->imgType)) { //文件类型不支持 $this->errorMsg = "{$fileName}图片后缀类型不支持 line:".__LINE__; return false; } if($fileType=="jpg" || $fileType=="jpeg") { $im=imageCreateFromjpeg($fileName); }else{ $im=imagecreatefrompng($fileName); } if(!$im) { //图片初始化失败 $this->errorMsg = "{$fileName}图片初始化资源失败 line:".__LINE__; return false; } //源图片宽 $width=imagesx($im); //源图片高 $height=imagesy($im); if(empty($width) || empty($height)) { //图片高度宽度获取失败 $this->errorMsg = "{$fileName}图片高度或者宽度获取失败 line:".__LINE__; return false; } return array("r"=>$im,"t"=>$fileType,"w"=>$width,"h"=>$height); } /** * 原始图片全局变量设置 成功返回数组,失败返回false * @author sanshi0815 * @return array w{宽度},h{高度} */ private function initSrcImgWH() { $temp = $this->getResource($this->srcFile); if(empty($temp)) { $this->errorMsg = "图像资源不存在 line:".__LINE__; return false; } $this->fileType = $temp['t']; $this->im=$temp['r']; return array("w"=>$temp['w'],"h"=>$temp['h']); } /** * 固定宽度,高度 进行图片缩放 * @author sanshi0815 * @param int $width 生成图片宽度 * @param int $height 生成图片高度 * @return bool 成功为 true,失败为false */ public function resetImgWH($width,$height) { $temp = $this->initSrcImgWH(); if(empty($temp)) { $this->errorMsg = "图像资源不存在 line:".__LINE__; return false; } $srcW = $temp['w']; $srcH = $temp['h']; $detW = intval($width); $detH = intval($height); //生成新的图像资源 $om = $this->getNewImg($srcW,$srcH,$detW,$detH); $temp = empty($om) ? false : $this->createImg($om,$detW,$detH); return $temp; } /** * 根据最大高度 进行图片等比缩放 * @author sanshi0815 * @param int $maxHeight 生成图片高度 * @return bool 成功为 true,失败为false */ public function resetImgMaxH($maxHeight) { $maxHeight = intval($maxHeight); $temp = $this->initSrcImgWH(); if(empty($temp)) { $this->errorMsg = "图像资源不存在 line:".__LINE__; return false; } $srcW = $temp['w']; $srcH = $temp['h']; //计算缩放比 $scale = round($maxHeight/$srcH,4); $detW = round($srcW*$scale); $detH = round($srcH*$scale); //生成新的图像资源 $om = $this->getNewImg($srcW,$srcH,$detW,$detH); $temp = empty($om) ? false : $this->createImg($om,$detW,$detH); return $temp; } /** * 根据最大宽度 进行图片等比缩放 * @author sanshi0815 * @param int $maxWidth 生成图片宽度 * @return bool 成功为 true,失败为false */ public function resetImgMaxW($maxWidth) { $temp = $this->initSrcImgWH(); if(empty($temp)) { $this->errorMsg = "图像资源不存在 line:".__LINE__; return false; } $srcW = $temp['w']; $srcH = $temp['h']; //计算缩放比 $scale = round($maxWidth/$srcW,4); $detW = round($srcW*$scale); $detH = round($srcH*$scale); //生成新的图像资源 $om = $this->getNewImg($srcW,$srcH,$detW,$detH); //$om = $this->im; $temp = empty($om) ? false : $this->createImg($om,$detW,$detH); return $temp; } /** * 获得缩放后的图片资源句柄 * @author sanshi0815 * @param int $srcW 原始图片宽度 * @param int $srcH 原始图片高度 * @param int $detW 原始图片宽度 * @param int $detH 原始图片高度 * @return bool 成功为 true,失败为false */ private function getNewImg($srcW,$srcH,$detW,$detH) { $om=imagecreatetruecolor($detW,$detH);//真色彩对gb库有要求 if(empty($om)) { $this->errorMsg = "imagecreatetruecolor 函数失败 line:".__LINE__; return false; } //ImageCopyResized($om,$im,0,0,0,0,$detW,$detH,$srcW,$srcH); $temp = imagecopyresampled($om,$this->im,0,0,0,0,$detW,$detH,$srcW,$srcH); if(empty($temp)) { $this->errorMsg = "imagecopyresampled 函数失败 line:".__LINE__; return false; } return $om; } /** * 获得图片加文字水印后资源 * @author sanshi0815 * @param resource $im 原始资源 * @param int $detW 原始图片宽度 * @param int $detH 原始图片高度 * @return resource 成功为 水印后的图片资源,失败为false */ private function getWatermarkText($im,$detW,$detH) { if(!$is_file($this->font)) { $this->errorMsg = "{$this->font} 字体不存在 line:".__LINE__; return false; } //旋转角度 $angle = 20; $width = $detW/10; $size = $detW/8; $height = $detH; //echo $height; $black = imagecolorallocate($im, 0, 0, 0); $grey = imagecolorallocate($im, 180, 180, 180); //生成水印次数 $formax = 3; for($i=$formax;$i>=1;$i--) { $height =$height-$detH/($formax+2); //echo $height."<br>"; $temp = imagettftext($im, $size, $angle,$width,$height, $grey, $this->font,$this->watermark); if(empty($temp)) { $this->errorMsg = "imagettftext 函数失败 line:".__LINE__; return false; } } return $im; } /** * 获得图片加图片水印后资源 * @author sanshi0815 * @param resource $im 原始资源 * @param int $detW 原始图片宽度 * @param int $detH 原始图片高度 * @return resource 成功为 水印后的图片资源,失败为false */ private function getWatermarkPic($im,$detW,$detH) { $temp = $this->getResource($this->watermark); if(empty($temp)) return false; $wm = $temp['r']; $src_x = 0; $src_y = 0; $src_w = $temp['w']; $src_h = $temp['h']; $dst_x = $detW; $dst_y = $detH; $height = $dst_y > $src_h ? ($dst_y - $src_h)/2 :0; $width = $dst_x > $src_w ? ($dst_x - $src_w)/2 : 0; $temp = imagealphablending($im,true); if(empty($temp)) { $this->errorMsg = "imagealphablending 函数失败 line:".__LINE__; return false; } $temp = imagecopymerge($im,$wm,$width,$height,0,0,$src_w,$src_h,70); if(empty($temp)) { $this->errorMsg = "imagecopymerge 函数失败 line:".__LINE__; return false; } return $im; } /** * 新图片生成 * @author sanshi0815 * @param resource $im 原始资源 * @param int $detW 原始图片宽度 * @param int $detH 原始图片高度 * @return bool 成功为true,失败为false */ private function createImg($im,$detW,$detH) { //处理水印 if($this->watetType==2) { $om = $this->getWatermarkText($im,$detW,$detH); }elseif ($this->watetType==1) { $om = $this->getWatermarkPic($im,$detW,$detH); }else{ $om = $im; } if(empty($om)) { $this->errorMsg = "图片资源不存在 line:".__LINE__; return false; } $fileType = $this->fileType; if($fileType=="jpg" || $fileType=="jpeg") { $temp=imagejpeg($om,$this->dstFile); }else{ $temp=imagepng($om,$this->dstFile); } return $temp; } } $file=new MakeMiniature(); $file->set("./simhei.ttf","张磊专用","1_1453362028.png","s1_1453362028.png"); $file->resetImgMaxW(800); ?>
昔、ウォーターマーククラスについて書いていたのを思い出しました。 あまりにも長くなりすぎて、使いにくいものや、サムネイルなどの場面が変わってしまったものもあります。それらの多くは最大値に基づく必要があります。幅または高さは固定比率ではなく生成されます。これは、この方法で作成された画像が最高の表示効果を持ち、変形しないためです。したがって、このクラスは php7 のサポートのために再度改良され、実際には古いクラスもサポートされます。
前のクラス http://blog.csdn.net/sanshi0815/article/details/1604905
以上、PHP 7 をサポートするために 7 年ぶりに再構築された PHP gd ライブラリのウォーターマーク クラスについて、いくつかの側面を含めて紹介しましたが、PHP チュートリアルに興味のある友人の参考になれば幸いです。
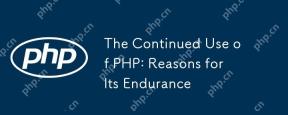
まだ人気があるのは、使いやすさ、柔軟性、強力なエコシステムです。 1)使いやすさとシンプルな構文により、初心者にとって最初の選択肢になります。 2)Web開発、HTTP要求とデータベースとの優れた相互作用と密接に統合されています。 3)巨大なエコシステムは、豊富なツールとライブラリを提供します。 4)アクティブなコミュニティとオープンソースの性質は、それらを新しいニーズとテクノロジーの傾向に適応させます。
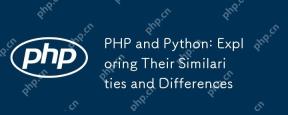
PHPとPythonはどちらも、Web開発、データ処理、自動化タスクで広く使用されている高レベルのプログラミング言語です。 1.PHPは、ダイナミックウェブサイトとコンテンツ管理システムの構築によく使用されますが、PythonはWebフレームワークとデータサイエンスの構築に使用されることがよくあります。 2.PHPはエコーを使用してコンテンツを出力し、Pythonは印刷を使用します。 3.両方ともオブジェクト指向プログラミングをサポートしますが、構文とキーワードは異なります。 4。PHPは弱いタイプの変換をサポートしますが、Pythonはより厳しくなります。 5. PHPパフォーマンスの最適化には、Opcacheおよび非同期プログラミングの使用が含まれますが、PythonはCprofileおよび非同期プログラミングを使用します。
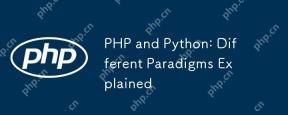
PHPは主に手順プログラミングですが、オブジェクト指向プログラミング(OOP)もサポートしています。 Pythonは、OOP、機能、手続き上のプログラミングなど、さまざまなパラダイムをサポートしています。 PHPはWeb開発に適しており、Pythonはデータ分析や機械学習などのさまざまなアプリケーションに適しています。
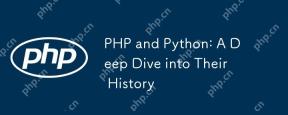
PHPは1994年に発信され、Rasmuslerdorfによって開発されました。もともとはウェブサイトの訪問者を追跡するために使用され、サーバー側のスクリプト言語に徐々に進化し、Web開発で広く使用されていました。 Pythonは、1980年代後半にGuidovan Rossumによって開発され、1991年に最初にリリースされました。コードの読みやすさとシンプルさを強調し、科学的コンピューティング、データ分析、その他の分野に適しています。
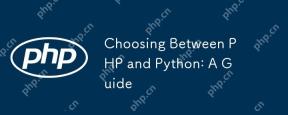
PHPはWeb開発と迅速なプロトタイピングに適しており、Pythonはデータサイエンスと機械学習に適しています。 1.PHPは、単純な構文と迅速な開発に適した動的なWeb開発に使用されます。 2。Pythonには簡潔な構文があり、複数のフィールドに適しており、強力なライブラリエコシステムがあります。
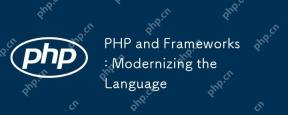
PHPは、多数のWebサイトとアプリケーションをサポートし、フレームワークを通じて開発ニーズに適応するため、近代化プロセスで依然として重要です。 1.PHP7はパフォーマンスを向上させ、新機能を紹介します。 2。Laravel、Symfony、Codeigniterなどの最新のフレームワークは、開発を簡素化し、コードの品質を向上させます。 3.パフォーマンスの最適化とベストプラクティスは、アプリケーションの効率をさらに改善します。
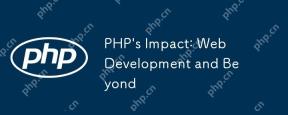
phphassiblasifly-impactedwebdevevermentandsbeyondit.1)itpowersmajorplatformslikewordpratsandexcelsindatabase interactions.2)php'sadaptableability allowsitale forlargeapplicationsusingframeworkslikelavel.3)
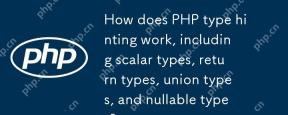
PHPタイプは、コードの品質と読みやすさを向上させるためのプロンプトがあります。 1)スカラータイプのヒント:php7.0であるため、基本データ型は、int、floatなどの関数パラメーターで指定できます。 3)ユニオンタイプのプロンプト:PHP8.0であるため、関数パラメーターまたは戻り値で複数のタイプを指定することができます。 4)Nullable Typeプロンプト:null値を含めることができ、null値を返す可能性のある機能を処理できます。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

SublimeText3 中国語版
中国語版、とても使いやすい
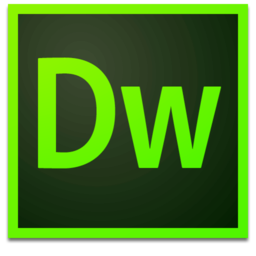
Dreamweaver Mac版
ビジュアル Web 開発ツール

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター
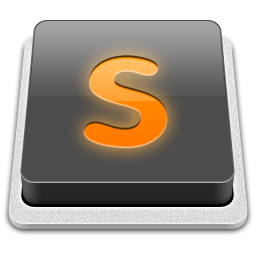
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

MinGW - Minimalist GNU for Windows
このプロジェクトは osdn.net/projects/mingw に移行中です。引き続きそこでフォローしていただけます。 MinGW: GNU Compiler Collection (GCC) のネイティブ Windows ポートであり、ネイティブ Windows アプリケーションを構築するための自由に配布可能なインポート ライブラリとヘッダー ファイルであり、C99 機能をサポートする MSVC ランタイムの拡張機能が含まれています。すべての MinGW ソフトウェアは 64 ビット Windows プラットフォームで実行できます。
