JS では関数オブジェクト (関数) のみがクラスの概念を持っているため、オブジェクトを作成するには関数オブジェクトを使用する必要があります。関数オブジェクト内には [[Construct]] メソッドと [[Call]] メソッドがあり、 [[Construct]] はオブジェクトの構築に使用され、 [[Call]] は関数の呼び出しにのみ使用されます。 new 演算子を使用する場合。注: この例では、カスタム関数 HumanCloning は関数オブジェクトなので、Object、String、Number などのローカル オブジェクトは関数オブジェクトですか? ローカル オブジェクトは関数の派生型と見なすことができるため、答えは「はい」です。この意味では、ユーザー定義関数と同等に扱うことができます。 (「JavaScript_04_Data Model の理解」の「組み込みデータ型」セクションを繰り返します)
var obj=new Object(); は、組み込みオブジェクト関数オブジェクトを使用して、インスタンス化されたオブジェクト obj を作成します。 var obj={}; および var obj=[]; この種のコードは、JS エンジンによるオブジェクトと配列の構築プロセスをトリガーします。 function fn(){}; var myObj=new fn(); は、ユーザー定義型を使用してインスタンス化されたオブジェクトを作成します。
この例に基づいて、関数オブジェクトは HumanCloning であり、関数オブジェクトによって作成されるオブジェクト インスタンスは clone01 と clone02 です。次に、var clone01 = new HumanCloning(); の実装の詳細を見てみましょう。 [[ 関数オブジェクトの Construct]] メソッドは、オブジェクトの作成を実装するロジックを処理します):
1. 組み込みオブジェクト obj を作成し、それを初期化します。
3.1 内部 [[Call]] メソッドは、現在の実行コンテキストを作成します (注: 実行コンテキストについては、後続のブログ投稿で説明します。また、記事「」でも部分的に説明されています)。 Javascript Speed Up_01_Reference 変数の最適化")
が返されます。 4. [[Call]] の場合、戻り値が Object 型の場合はこの値が返され、それ以外の場合は obj が返されます。次のコードは
HumanCloning を理解するためにのみ使用されます。呼び出し(clone01);
Note that in step 2, prototype refers to the prototype property displayed by the object, and [[Prototype]] represents the internal prototype property of the object (implicit). What constitutes the object's prototype chain is the internal implicit [[Prototype]], not the object's explicit prototype attribute. The displayed prototype is only meaningful on the function object. As you can see from the above creation process, the prototype of the function is assigned to the implicit [[Prototype]] attribute of the derived object. In this way, according to the Prototype rules, the prototype objects of the derived object and the function Only the inheritance/sharing relationship of properties and methods exists between them. (That is, the implementation principle of prototypal inheritance is the content of "Understanding Javascript_05_Prototypal Inheritance Principle")
Pay attention to the description in step 3.4, let us look at a question from Yi Fei:
I think it should be easy to answer this question now.
Note: Original addresshttp://www.planabc.net/2008/02/20/javascript_new_function/
Memory Analysis
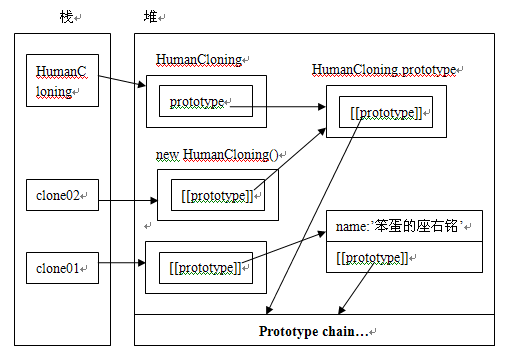
A simple memory diagram and the introduction of the concept of function objects also explain the above code (relatively speaking, the diagram is not very rigorous, but easy to understand). This also raises a question, the implementation principle of instanceof. I believe everyone has seen some signs. The judgment of instanceof relies on the prototype chain. For specific implementation details, please refer to the subsequent blog post.
Local attributes and inherited attributes
Objects can inherit attributes and methods through the implicit prototype chain, but prototype is also an ordinary object, that is to say, it is an ordinary instantiated object, not purely abstract data. Structural description. So there is this problem of local properties and inherited properties.
First, let’s take a look at the process of setting object properties. JS defines a set of attributes that are used to describe the properties of objects to indicate whether the properties can be set in JavaScript code, enumerated by for in, etc. The steps for processing the assignment statement of
obj.propName=value are as follows:
1. If the attribute of propName is set to cannot be set, return
2. If obj.propName does not exist, create an attribute for obj , the name is propName
3. Set the value of obj.propName to value
. You can see that the value setting process does not consider the Prototype chain. The reason is obvious. The internal [[Prototype]] of obj is an instance. It is an object that not only shares properties with obj, but may also share properties with other objects. Modifying it may affect other objects.
Let’s look at an example:
function HumanCloning( ){
}
HumanCloning.prototype ={
name:'Idiot's motto'
}
var clone01 = new HumanCloning();
clone01.name = 'jxl';
alert(clone01.name);//jxl
var clone02 = new HumanCloning();
alert(clone02.name);//Idiot’s motto
The result is great To be clear, the properties of an object cannot modify the property of the same name in its prototype, but will only create a property of the same name and assign a value to it.
Reference.
http://www.jb51.net/article/25008.htm