コンストラクターとは
簡単に理解すると、コンストラクターはオブジェクトのコンストラクターを指します。次の例を参照してください。
function Foo( ){};
var foo = new Foo();
alert(foo.constructor);//Foo
alert(Foo.constructor);//関数
alert(Object.constructor) ;//Function
alert(Function.constructor);//Function
foo.constructor が Foo の場合、foo のコンストラクターが Foo なのでわかりやすいと思います。 Foo、Object、Function のコンストラクターが Function であるという事実については、何も議論の余地はないと思います。 (Foo、Object、Function はすべて関数オブジェクトであり、すべての関数オブジェクトは Function オブジェクトから構築されるため、コンストラクターは Function です。詳細は「js_Function オブジェクト」を参照してください。)
の関係プロトタイプとコンストラクター
function Dog( ) {}
alert(Dog === Dog.prototype.constructor);//true
JavaScript では、各関数には「prototype」という名前の属性があり、これは、プロトタイプオブジェクト。このプロトタイプ オブジェクトには、関数自体を参照する「コンストラクター」と呼ばれるプロパティがあります。これは図に示すように循環参照です:
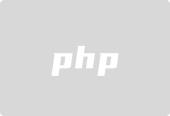
コンストラクター属性はどこから来たのでしょうか?
文字列を構築する関数の構築プロセスを見てみましょう:
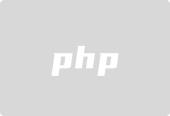
注: 関数コンストラクター どの関数オブジェクトのプロセスも同じであるため、文字列、ブール値、数値などの組み込みオブジェクトであっても、ユーザー定義オブジェクトであっても、構築プロセスは上図と同じです。ここでの文字列はあくまで代表です!
図からわかるように、コンストラクターは Function が関数オブジェクトを作成するときに生成されます。「プロトタイプとコンストラクターの関係」で説明したように、コンストラクターは関数オブジェクトのプロトタイプ チェーン内の属性です。それは String=== String.prototype.constructor です。
また、コードの一部を使用して、理論が正しいことを証明したいと思います。
function person(){}
var p = new Person();
alert(p.constructor);//person
alert( Person.prototype.constructor) ;//パーソン
alert(person.prototype.hasOwnProperty('constructor'));//true
alert(person.prototype.isPrototypeOf(p));//true
alert(Object.prototype .isPrototypeOf(p));//true
alert(person.prototype == Object.prototype);//false
今では、次のことがわかります。これは前の「プロトタイプ チェーン」と同じです。「実装原則」のデフォルトのプロトタイプは Object.prototype を指しますが、明らかに当時の理論はあまり包括的ではありませんでした。
特別なオブジェクト
注意深い読者はこの質問をするかもしれませんが、あなたの理論はオブジェクトには当てはまりません。次のコードは上記の理論と矛盾するためです:
alert(Object.prototype.hasOwnProperty('constructor'));//true
alert(Object.prototype.hasOwnProperty('isPrototypeOf'));//true、上記の理論によれば、ここでは false が返されるはずです
本当にそうですか?いいえ!次に、特別なオブジェクトがどのように処理されるかを見てみましょう:
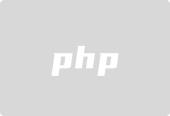
この図の原理は、上の図の原理と同じであることがわかります。これは、Object.prototype.hasOwnProperty('isPrototypeOf') が true であることを正しく説明できます。
コンストラクター探索
function Animal(){}
function person(){}
var person = new Person();
alert(person. constructor); //person
前のセクションの内容に基づいて、このコードの結果を正しく理解できますか?それについて考えた後、その記憶表現を見てください:
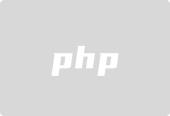
この図は、関数が動物と人を構築するプロセスを明確に示しています。同時にインスタンス人物と人物との関係も表示されます。
もう少し詳しく見てみると、コードは次のとおりです。
function Animal(){}
function Person(){}
Person.prototype = new Animal();
var person = new Person();
alert(person .constructor); //Animal
At this time, the constructor of person becomes Animal. How to explain?
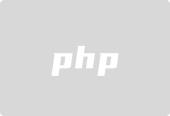
Note: The dotted line in the picture represents the default prototype pointer of Person (for reference only). But we pointed Person.prototype to new Animal.
At this time, the prototype of Person points to the instance of Animal, so the constructor of person is the constructor of Animal.
Conclusion: The principle of constructor is very simple, which is to look for the constructor attribute on the prototype chain of the object.
Note: If you cannot correctly understand the content of this article, please review the content of the previous chapters.