Article discusses lambda functions, their differences from regular functions, and their utility in programming scenarios. Not all languages support them.
What is a lambda function?
A lambda function, often referred to as an anonymous function, is a small, anonymous function that can be defined inline within a larger expression. It is typically used for short, one-time use functions that don't need a name. The term "lambda" originates from the Lambda Calculus, a system for expressing functions and computations using pure, untyped lambda terms.
In many programming languages, a lambda function has a concise syntax that allows it to be written in a single line. For example, in Python, a lambda function can be defined as follows:
lambda x: x + 10
This lambda function takes an argument x
and returns the value of x + 10
. Lambda functions are particularly useful in scenarios where you need a simple function for a short period and don't want to formally define it with a def
statement.
How is a lambda function different from a regular function?
Lambda functions and regular functions have several key differences:
-
Syntax: Lambda functions have a more compact syntax compared to regular functions. In Python, a regular function is defined using the
def
keyword, while a lambda function uses thelambda
keyword.Regular function example:
def add_ten(x): return x + 10
Lambda function example:
lambda x: x + 10
- Anonymity: Lambda functions are anonymous, meaning they don't have a declared name. This makes them suitable for use in situations where a function is needed for a short duration and does not need to be referenced later. Regular functions, on the other hand, must be named when they are defined.
- Scope and Reusability: Regular functions can be reused throughout a program and can contain multiple statements, including complex logic and control flow. Lambda functions, being designed for simplicity, are limited to a single expression and are typically used in the context where they are defined.
-
Use Cases: Lambda functions are often used as arguments to higher-order functions like
map()
,filter()
, andreduce()
, or as event handlers in GUI programming. Regular functions are used for more complex operations that may span multiple lines and require more comprehensive logic.
In what programming scenarios are lambda functions most useful?
Lambda functions are most useful in several specific programming scenarios:
-
Functional Programming: Lambda functions are integral to functional programming paradigms, where they are used to create small, reusable components. They are often passed as arguments to higher-order functions like
map()
,filter()
, andreduce()
. For example:numbers = [1, 2, 3, 4, 5] squared_numbers = list(map(lambda x: x**2, numbers))
-
Event Handling: In GUI programming, lambda functions can be used as event handlers for buttons, menus, or other interactive elements. They allow for inline definition of small functions that respond to user actions:
button = Button(root, text="Click me", command=lambda: print("Button clicked!"))
-
Sorting and Key Functions: Lambda functions can be used to customize sorting operations by providing a key function that defines how elements should be compared. For example:
students = [{"name": "Alice", "score": 85}, {"name": "Bob", "score": 92}, {"name": "Charlie", "score": 78}] sorted_students = sorted(students, key=lambda x: x["score"], reverse=True)
-
Data Processing: When working with data, lambda functions can be used to apply transformations or filters on-the-fly. For instance, in data analysis with Pandas:
import pandas as pd df = pd.DataFrame({"A": [1, 2, 3], "B": [4, 5, 6]}) df["C"] = df["A"].apply(lambda x: x * 2)
Can lambda functions be used in all programming languages?
No, lambda functions are not supported in all programming languages. Their availability depends on the language's design and support for functional programming concepts. Here are some examples:
-
Python: Supports lambda functions with the
lambda
keyword. -
JavaScript: Supports lambda functions through arrow functions (
=>
). - Java: Introduced lambda expressions in Java 8.
- C++: Supports lambda functions since C++11.
-
Ruby: Uses the
lambda
keyword or the->
operator for lambda functions. - Haskell: A functional programming language that heavily uses lambda functions.
However, some languages do not support lambda functions at all, or their support is limited:
- C: Does not have built-in support for lambda functions, though some compilers may offer extensions.
- PHP: Introduced limited support for anonymous functions in PHP 5.3, but they are not as flexible as lambda functions in other languages.
In summary, while lambda functions are a powerful tool in many modern programming languages, their availability and syntax can vary significantly depending on the language's design and capabilities.
以上がラムダの機能とは何ですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
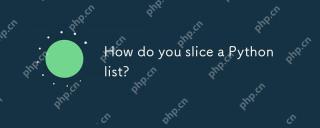
slicingapythonlistisdoneusingtheyntaxlist [start:stop:step] .hore'showitworks:1)startisthe indexofthefirstelementtoinclude.2)spotisthe indexofthefirmenttoeexclude.3)staptistheincrementbetbetinelements

numpyallows forvariousoperationsonarrays:1)basicarithmeticlikeaddition、減算、乗算、および分割; 2)AdvancedperationssuchasmatrixMultiplication;
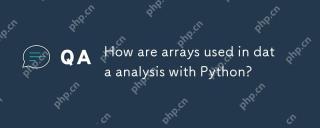
Arraysinpython、特にnumpyandpandas、aresentialfordataanalysis、offeringspeedandeficiency.1)numpyarraysenable numpyarraysenable handling forlaredatasents andcomplexoperationslikemoverages.2)Pandasextendsnumpy'scapabivitieswithdataframesfortruc
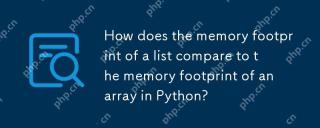
listsandnumpyarraysinpythonhavedifferentmemoryfootprints:listsaremoreflexiblellessmemory-efficient、whileenumpyarraysaraysareoptimizedfornumericaldata.1)listsstorereferencesto objects、with whowedaround64byteson64-bitedatigu
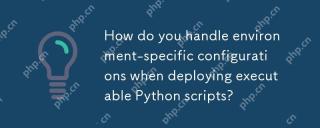
toensurepythonscriptsbehaveCorrectlyAcrossDevelosment、staging、and Production、usetheseStrategies:1)環境variablesforsimplestetings、2)configurationfilesforcomplexsetups、and3)dynamicloadingforadaptability.eachtododododododofersuniquebentandrequiresca
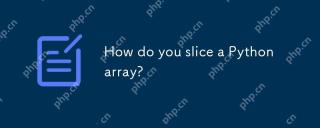
Pythonリストスライスの基本的な構文はリストです[start:stop:step]。 1.STARTは最初の要素インデックス、2。ストップは除外された最初の要素インデックスであり、3.ステップは要素間のステップサイズを決定します。スライスは、データを抽出するためだけでなく、リストを変更および反転させるためにも使用されます。
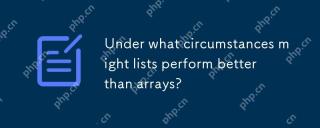
ListSoutPerformArraysIn:1)ダイナミシジョンアンドフレーケンティオン/削除、2)ストーリングヘテロゼンダタ、および3)メモリ効率の装飾、ButmayhaveslightPerformancostsinceNASOPERATIONS。
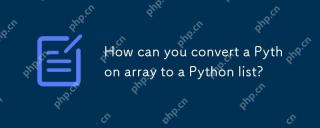
toconvertapythonarraytoalist、usetheList()constructororageneratorexpression.1)importhearraymoduleandcreateanarray.2)useList(arr)または[xforxinarr] toconvertoalistは、largedatatessを変えることを伴うものです。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、

Safe Exam Browser
Safe Exam Browser は、オンライン試験を安全に受験するための安全なブラウザ環境です。このソフトウェアは、あらゆるコンピュータを安全なワークステーションに変えます。あらゆるユーティリティへのアクセスを制御し、学生が無許可のリソースを使用するのを防ぎます。

mPDF
mPDF は、UTF-8 でエンコードされた HTML から PDF ファイルを生成できる PHP ライブラリです。オリジナルの作者である Ian Back は、Web サイトから「オンザフライ」で PDF ファイルを出力し、さまざまな言語を処理するために mPDF を作成しました。 HTML2FPDF などのオリジナルのスクリプトよりも遅く、Unicode フォントを使用すると生成されるファイルが大きくなりますが、CSS スタイルなどをサポートし、多くの機能強化が施されています。 RTL (アラビア語とヘブライ語) や CJK (中国語、日本語、韓国語) を含むほぼすべての言語をサポートします。ネストされたブロックレベル要素 (P、DIV など) をサポートします。
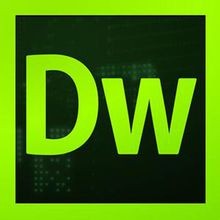
ドリームウィーバー CS6
ビジュアル Web 開発ツール

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。

ホットトピック









