html5のクライアント側データベースストレージにindexeddbを使用するにはどうすればよいですか?
indexedDBは、ファイル/ブロブを含む構造化データのクライアント側ストレージの低レベルAPIです。クライアント側のデータベースストレージにhtml5でindexedDBを使用するには、次の手順に従うことができます。
-
データベースを開きます:
IndexEdDBデータベースへの接続を開くことから始めます。これはindexedDB.open()
メソッドを使用して実行できます。データベースの名前とオプションでバージョン番号を指定します。onupgradeneeded
Event Handlerは、データベースの作成時またはそのバージョンの変更時にデータベーススキーマをセットアップするために使用されます。<code class="javascript">const request = indexedDB.open("MyDatabase", 1); request.onupgradeneeded = function(event) { const db = event.target.result; // Create an objectStore for this database const objectStore = db.createObjectStore("items", { keyPath: "id" }); // Create an index to search items by name objectStore.createIndex("name", "name", { unique: false }); };</code>
-
データの追加:
IndexEdDBデータベースにデータを追加するには、最初にトランザクションを開き、次にオブジェクトストアでadd()
またはput()
メソッドを使用します。<code class="javascript">request.onsuccess = function(event) { const db = event.target.result; const transaction = db.transaction(["items"], "readwrite"); const objectStore = transaction.objectStore("items"); const request = objectStore.add({ id: 1, name: "item1", price: 10 }); request.onsuccess = function(event) { console.log("Item added to the database"); }; };</code>
-
データを取得:
データを取得するには、キーを知っている場合はget()
メソッドを使用できます。より複雑なクエリには、カーソルまたはインデックスを使用できます。<code class="javascript">request.onsuccess = function(event) { const db = event.target.result; const transaction = db.transaction(["items"], "readonly"); const objectStore = transaction.objectStore("items"); const request = objectStore.get(1); request.onsuccess = function(event) { console.log("Item retrieved: ", event.target.result); }; };</code>
-
データを更新および削除します:
データの更新は、キーに基づいてデータを挿入または更新するput()
メソッドを使用して実行できます。データを削除するには、delete()
メソッドを使用します。<code class="javascript">request.onsuccess = function(event) { const db = event.target.result; const transaction = db.transaction(["items"], "readwrite"); const objectStore = transaction.objectStore("items"); const requestUpdate = objectStore.put({ id: 1, name: "item1 updated", price: 15 }); const requestDelete = objectStore.delete(2); };</code>
-
エラー処理:
onerror
イベントを使用して常にエラーを処理して、データベース操作中に発生する問題をキャッチします。<code class="javascript">request.onerror = function(event) { console.log("Database error: " event.target.errorCode); };</code>
クライアント側にデータを保存するためにindexedDBを使用することの利点は何ですか?
indexedDBは、クライアント側にデータを保存するためのいくつかの利点を提供します。
-
オフライン機能:
indexedDBを使用すると、ユーザーのデバイスにデータをローカルに保存することにより、Webアプリケーションがオフラインで動作することができます。これにより、インターネット接続なしで機能を有効にすることにより、ユーザーエクスペリエンスが向上します。 -
高い貯蔵容量:
LocalStorageなどの他のストレージオプションと比較して、IndexEdDBは大量のデータを処理でき、複雑なアプリケーションに適しています。 -
構造化されたデータストレージ:
IndexEdDBは、ファイルやブロブなどの構造化データの保存をサポートしているため、単純なキー価値ストアと比較して、より柔軟で効率的なデータ管理が可能になります。 -
効率的なデータ取得:
インデックスを使用すると、高速データの取得と複雑なクエリを実行する機能が可能になります。これは、データの検索と並べ替えが必要なアプリケーションに役立ちます。 -
非同期API:
IndexEdDBの非同期性は、UIのブロックを防ぎ、アプリケーションのパフォーマンスと応答性を改善します。 -
トランザクションサポート:
indexedDBは、ユニットとして成功または失敗する関連する操作をグループ化することにより、データの整合性を維持するのに役立つトランザクションをサポートします。
WebアプリケーションでIndexEdDBを使用するときに、データの永続性とセキュリティを確保するにはどうすればよいですか?
IndexEdDBのデータの持続性とセキュリティを確保するには、いくつかの重要なプラクティスが含まれます。
-
データの持続性:
- 通常のバックアップ:ユーザーデバイスの障害またはデータの破損の場合にデータの損失を防ぐために、indexedDBデータの定期的なバックアップを実装します。
- クォータ管理:ブラウザによって設定されたストレージクォータに注意し、データを超えることを避けるためにデータを効率的に管理します。これにより、データが自動的にクリアされる可能性があります。
- エラー処理:データ損失につながる可能性のある問題をキャッチおよび管理するために、堅牢なエラー処理を実装します。
-
データセキュリティ:
- 暗号化:クライアント側の暗号化を使用して、IndexEdDBに保存されている機密データを保護します。 Crypto-JSなどのライブラリを使用して、保存する前にデータを暗号化できます。
- ユーザー認証: IndexEdDBへのアクセスがユーザー認証によって制御されていることを確認してください。セッショントークンまたは同様のメカニズムを使用して、データベースへのアクセスを承認します。
- 安全なコンテキスト:アプリケーションがHTTPSを介して提供されていることを確認して、最新のブラウザでIndexEdDBにアクセスするための要件である安全なコンテキストを確保してください。
- データ検証:注入攻撃または不正なデータがデータベースに入るのを防ぐために、厳格なデータ検証を実装します。
- 分離:さまざまなユーザーに異なるデータベースまたはオブジェクトストアを使用して、データ露出を制限します。
html5にindexeddbを実装する際に避けるべき一般的な落とし穴は何ですか?
indexedDBを実装する場合、パフォーマンスの問題やアプリケーションの障害につながる可能性のある一般的な落とし穴を避けることが重要です。
-
エラー処理を無視する:
エラーを適切に処理できないと、静かな失敗につながる可能性があります。onerror
イベントハンドラーを常に使用して、エラーをキャッチおよびログにしてください。 -
同期操作:
IndexEdDB操作を同期として扱うことは、UIをブロックし、パフォーマンスの低下につながる可能性があります。常に非同期APIを使用し、コールバックまたは約束を使用して操作を管理します。 -
クォータの制限を無視する:
ストレージクォータを管理しないと、ブラウザが自動的にデータを削除する可能性があります。データサイズを監視および管理して、制限内にとどまります。 -
接続を閉じることを怠る:
データベース接続を開いたままにすると、リソースのリークが発生する可能性があります。それらがもはや必要ない場合は、常に接続を閉じてください。 -
過剰インデックス:
あまりにも多くのインデックスを作成すると、パフォーマンスが低下する可能性があります。必要なインデックスのみを作成し、クエリパフォーマンスへの影響を確認してください。 -
誤解バージョン:
データベースバージョンを誤って処理すると、データの損失や破損につながる可能性があります。アップグレード中に適切なバージョン管理を確認します。 -
データ検証の欠如:
データを保存する前にデータを検証しないと、データの破損やセキュリティの脆弱性につながる可能性があります。データをデータベースに挿入する前に、常にデータを検証およびサニタイズしてください。 -
ブラウザの互換性を無視する:
IndexEdDBの実装は、ブラウザによって異なる場合があります。複数のブラウザでアプリケーションをテストし、idb
などのポリフィルまたはライブラリを使用してブラウザの違いを抽象化することを検討してください。
以上がhtml5のクライアント側データベースストレージにindexeddbを使用するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

htmltagsareSterenceforwebdevelovementasyStheStructureanhandhancewebpages.1)theydefineLayout、semantics、and-interactivity.2)semanticagsimprovecessibility.3)opeusofusofagscanoptimizeperformanceandensurecross-brows-compativeation。

コードの読みやすさ、保守性、効率を向上させるため、一貫したHTMLエンコーディングスタイルは重要です。 1)低ケースタグと属性を使用します。2)一貫したインデントを保持し、3)シングルまたはダブルの引用符を選択して固執する、4)プロジェクトのさまざまなスタイルの混合を避け、5)きれいなスタイルやEslintなどの自動化ツールを使用して、スタイルの一貫性を確保します。
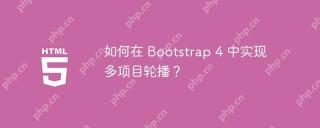
Bootstrap4にマルチプロジェクトカルーセルを実装するソリューションBootstrap4にマルチプロジェクトカルーセルを実装するのは簡単な作業ではありません。ブートストラップですが...
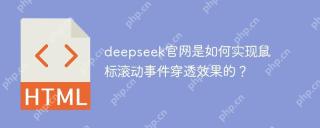
マウススクロールイベントの浸透の効果を実現する方法は? Webを閲覧すると、いくつかの特別なインタラクションデザインに遭遇することがよくあります。たとえば、DeepSeekの公式ウェブサイトでは、...
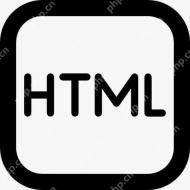
HTMLビデオのデフォルトの再生コントロールスタイルは、CSSを介して直接変更することはできません。 1. JavaScriptを使用してカスタムコントロールを作成します。 2。CSSを介してこれらのコントロールを美化します。 3. video.jsやPLYRなどのライブラリを使用すると、互換性、ユーザーエクスペリエンス、パフォーマンスを検討してください。プロセスを簡素化できます。
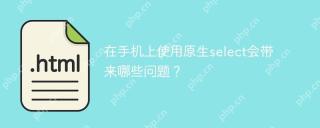
携帯電話でネイティブセレクトを使用する際の潜在的な問題は、モバイルアプリケーションを開発するときに、ボックスを選択する必要があることがよくあります。通常、開発者...
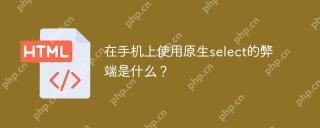
お使いの携帯電話でネイティブ選択を使用することの欠点は何ですか?モバイルデバイスでアプリケーションを開発する場合、適切なUIコンポーネントを選択することが非常に重要です。多くの開発者...
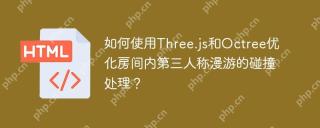
Three.JSとOctreeを使用して、部屋でのサードパーソンローミングの衝突処理を最適化します。 3つのjsでoctreeを使用して、部屋でサードパーソンローミングを実装し、衝突を追加してください...


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。

SublimeText3 英語版
推奨: Win バージョン、コードプロンプトをサポート!

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
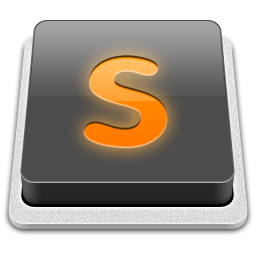
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

メモ帳++7.3.1
使いやすく無料のコードエディター

ホットトピック









