この記事では、ThinkPhpのカスタム検証ルールの作成と使用を示しています。検証クラスを拡張して、ドメイン固有の電子メールチェックなどのルールを定義する詳細を示します。コード組織、エラー処理、テストのベストプラクティスが強調されています
ThinkPhpでカスタム検証ルールを作成および使用します
ThinkPHPは、組み込みオプションを超えてカスタム検証ルールを定義できる柔軟な検証システムを提供します。 This is achieved primarily through the Validate
class and its associated methods. You can create custom validation rules by extending the Think\Validate
class or by defining validation rules within your model or controller.
例で説明しましょう。 Suppose we need a rule to validate an email address against a specific domain, say example.com
.次のようなカスタム検証ルールを作成できます。
<code class="php"><?php namespace app\validate; use think\Validate; class UserValidate extends Validate { protected $rule = [ 'email' => 'require|email|domain:example.com', ]; protected $message = [ 'email' => [ 'require' => 'Email is required', 'email' => 'Invalid email format', 'domain:example.com' => 'Email must be from example.com', ], ]; protected function domain($value, $rule, $data = []) { return strpos($value, '@example.com') !== false; } }</code>
In this example, we define a domain
rule within the UserValidate
class. The domain
method checks if the email address contains @example.com
. This custom rule is then used in the rule
array alongside ThinkPHP's built-in require
and email
rules. The message
array provides custom error messages for each rule. To use this validation, you'd simply instantiate the UserValidate
class and run the check
method.
<code class="php">$validate = new \app\validate\UserValidate(); if ($validate->check(['email' => 'test@example.com'])) { // Validation passed } else { // Validation failed; $validate->getError() will return the error message. }</code>
カスタム検証ルールを実装するためのベストプラクティス
長期的なプロジェクトの成功には、クリーンで再利用可能なコードを維持することが重要です。 ThinkPhpにカスタム検証ルールを実装するためのベストプラクティスをいくつか紹介します。
- Separation of Concerns: Create separate validation classes for different models or groups of related models.これにより、組織と再利用性が向上します。すべての検証ロジックを単一のクラスに詰め込まないでください。
- Descriptive Naming: Use clear and descriptive names for your validation classes and methods.これにより、読みやすさと理解が向上します。 For example, instead of
validate_user
, useUserValidate
. - Consistent Error Handling: Always provide informative error messages for failed validations. Use the
message
array in yourValidate
class to define custom error messages. - Unit Testing: Write unit tests for your custom validation rules to ensure correctness and prevent regressions.これは、複雑な検証ロジックにとって特に重要です。
- Documentation: Document your custom validation rules, explaining their purpose, parameters, and expected behavior.これは、保守性とコラボレーションに役立ちます。
カスタム検証ルールをThinkPHPの組み込みシステムと統合します
カスタム検証ルールをThinkPHPの組み込みシステムと統合するのは簡単です。 You can seamlessly combine your custom rules with ThinkPHP's built-in rules within the rule
array of your Validate
class. ThinkPhpは、指定された順序でカスタムルールと組み込みルールの両方を実行します。これにより、柔軟で強力な検証アプローチが可能になります。
For example, you can combine our custom domain
rule with other rules:
<code class="php">protected $rule = [ 'email' => 'require|email|domain:example.com|unique:users', ];</code>
This validates that the email
field is required, a valid email address, belongs to the example.com
domain, and is unique within the users
table.
ThinkPHPの既存の検証ルールを拡張します
ThinkPHPの検証システムを使用すると、既存のルールを拡張して、より複雑なカスタム検証を作成できます。 This is done by overriding or extending the existing validation methods within your custom Validate
class.これは、ThinkPhpの検証機能を特定のニーズに適応させる強力なメカニズムを提供します。
For example, let's say you want to extend the length
rule to also check for the presence of specific characters.カスタムメソッドを作成できます。
<code class="php">protected function lengthWithChars($value, $rule, $data = []) { list($min, $max, $chars) = explode(',', $rule); $len = mb_strlen($value); if ($len $max) return false; foreach (str_split($chars) as $char) { if (strpos($value, $char) === false) return false; } return true; }</code>
Then you can use it in your rule
array:
<code class="php">protected $rule = [ 'password' => 'lengthWithChars:8,20,A,a,1', // Password must be 8-20 characters long and contain at least one uppercase A, one lowercase a, and one digit 1. ];</code>
これは、ThinkPhpのコア機能を拡張して、アプリケーションの要件に合わせて非常に具体的で複雑な検証ルールを作成する方法を示しています。常に潜在的なエラーを優雅に処理し、ユーザーに有益なフィードバックを提供することを忘れないでください。
以上がThinkPhpでカスタム検証ルールを作成および使用するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
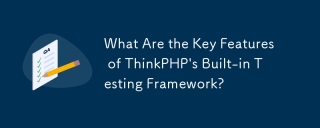
この記事では、ThinkPHPの組み込みテストフレームワークについて説明し、ユニットや統合テストなどの主要な機能と、早期のバグ検出とコード品質の向上を通じてアプリケーションの信頼性を高める方法について強調しています。
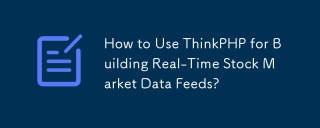
記事では、リアルタイムの株式市場データフィードにThinkPhpを使用して、セットアップ、データの正確性、最適化、セキュリティ対策に焦点を当てて説明します。

この記事では、パフォーマンスの最適化、ステートレス設計、セキュリティに焦点を当てたサーバーレスアーキテクチャでThinkPhpを使用するための重要な考慮事項について説明します。コスト効率やスケーラビリティなどの利点を強調しますが、課題にも対処します
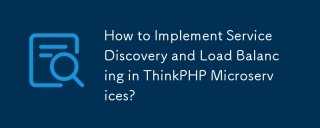
この記事では、セットアップ、ベストプラクティス、統合方法、および推奨ツールに焦点を当てたThinkPhpマイクロサービスにサービスの発見と負荷分散の実装について説明します。[159文字]。
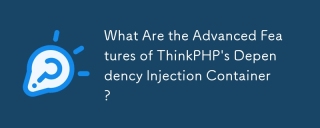
ThinkPHPのIOCコンテナは、PHPアプリで効率的な依存関係管理のための怠zyなロード、コンテキストバインディング、メソッドインジェクションなどの高度な機能を提供します。
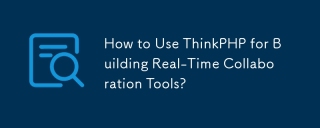
この記事では、ThinkPhpを使用してリアルタイムのコラボレーションツールを構築し、セットアップ、Websocket統合、セキュリティベストプラクティスに焦点を当てて説明します。
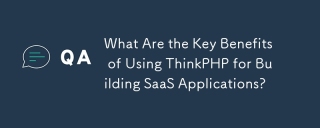
ThinkPhpは、軽量のデザイン、MVCアーキテクチャ、および拡張性を備えたSaaSアプリに利益をもたらします。スケーラビリティを向上させ、開発を速め、さまざまな機能を通じてセキュリティを改善します。
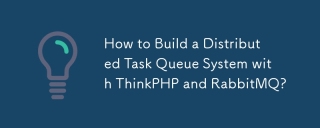
この記事では、ThinkPhpとRabbitMQを使用して分散タスクキューシステムの構築を概説し、インストール、構成、タスク管理、およびスケーラビリティに焦点を当てています。重要な問題には、Immedのような一般的な落とし穴を避けるための高可用性の確保が含まれます


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター

PhpStorm Mac バージョン
最新(2018.2.1)のプロフェッショナル向けPHP統合開発ツール

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
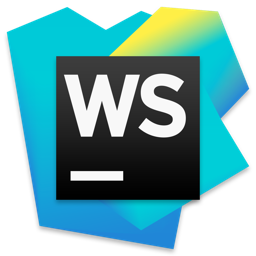
WebStorm Mac版
便利なJavaScript開発ツール
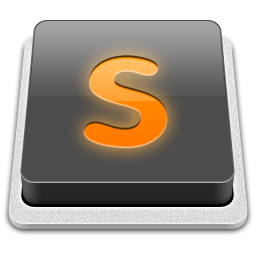
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)
